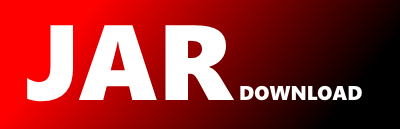
com.azure.resourcemanager.cosmos.models.ContainerPartitionKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* The configuration of the partition key to be used for partitioning data into multiple partitions.
*/
@Fluent
public final class ContainerPartitionKey implements JsonSerializable {
/*
* List of paths using which data within the container can be partitioned
*/
private List paths;
/*
* Indicates the kind of algorithm used for partitioning. For MultiHash, multiple partition keys (upto three
* maximum) are supported for container create
*/
private PartitionKind kind;
/*
* Indicates the version of the partition key definition
*/
private Integer version;
/*
* Indicates if the container is using a system generated partition key
*/
private Boolean systemKey;
/**
* Creates an instance of ContainerPartitionKey class.
*/
public ContainerPartitionKey() {
}
/**
* Get the paths property: List of paths using which data within the container can be partitioned.
*
* @return the paths value.
*/
public List paths() {
return this.paths;
}
/**
* Set the paths property: List of paths using which data within the container can be partitioned.
*
* @param paths the paths value to set.
* @return the ContainerPartitionKey object itself.
*/
public ContainerPartitionKey withPaths(List paths) {
this.paths = paths;
return this;
}
/**
* Get the kind property: Indicates the kind of algorithm used for partitioning. For MultiHash, multiple partition
* keys (upto three maximum) are supported for container create.
*
* @return the kind value.
*/
public PartitionKind kind() {
return this.kind;
}
/**
* Set the kind property: Indicates the kind of algorithm used for partitioning. For MultiHash, multiple partition
* keys (upto three maximum) are supported for container create.
*
* @param kind the kind value to set.
* @return the ContainerPartitionKey object itself.
*/
public ContainerPartitionKey withKind(PartitionKind kind) {
this.kind = kind;
return this;
}
/**
* Get the version property: Indicates the version of the partition key definition.
*
* @return the version value.
*/
public Integer version() {
return this.version;
}
/**
* Set the version property: Indicates the version of the partition key definition.
*
* @param version the version value to set.
* @return the ContainerPartitionKey object itself.
*/
public ContainerPartitionKey withVersion(Integer version) {
this.version = version;
return this;
}
/**
* Get the systemKey property: Indicates if the container is using a system generated partition key.
*
* @return the systemKey value.
*/
public Boolean systemKey() {
return this.systemKey;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("paths", this.paths, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("kind", this.kind == null ? null : this.kind.toString());
jsonWriter.writeNumberField("version", this.version);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ContainerPartitionKey from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ContainerPartitionKey if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the ContainerPartitionKey.
*/
public static ContainerPartitionKey fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ContainerPartitionKey deserializedContainerPartitionKey = new ContainerPartitionKey();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("paths".equals(fieldName)) {
List paths = reader.readArray(reader1 -> reader1.getString());
deserializedContainerPartitionKey.paths = paths;
} else if ("kind".equals(fieldName)) {
deserializedContainerPartitionKey.kind = PartitionKind.fromString(reader.getString());
} else if ("version".equals(fieldName)) {
deserializedContainerPartitionKey.version = reader.getNullable(JsonReader::getInt);
} else if ("systemKey".equals(fieldName)) {
deserializedContainerPartitionKey.systemKey = reader.getNullable(JsonReader::getBoolean);
} else {
reader.skipChildren();
}
}
return deserializedContainerPartitionKey;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy