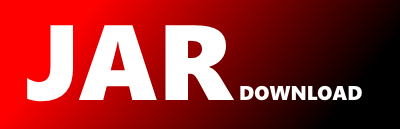
com.azure.resourcemanager.cosmos.models.CosmosDBAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.cosmos.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.http.rest.PagedFlux;
import com.azure.resourcemanager.cosmos.CosmosManager;
import com.azure.resourcemanager.cosmos.fluent.models.DatabaseAccountGetResultsInner;
import com.azure.core.management.Region;
import com.azure.resourcemanager.resources.fluentcore.arm.models.GroupableResource;
import com.azure.resourcemanager.resources.fluentcore.arm.models.Resource;
import com.azure.resourcemanager.resources.fluentcore.collection.SupportsUpdatingPrivateEndpointConnection;
import com.azure.resourcemanager.resources.fluentcore.model.Appliable;
import com.azure.resourcemanager.resources.fluentcore.model.Creatable;
import com.azure.resourcemanager.resources.fluentcore.model.Refreshable;
import com.azure.resourcemanager.resources.fluentcore.model.Updatable;
import reactor.core.publisher.Mono;
import java.util.List;
import java.util.Map;
/** An immutable client-side representation of an Azure Cosmos DB. */
@Fluent
public interface CosmosDBAccount
extends GroupableResource,
Refreshable,
Updatable,
SupportsUpdatingPrivateEndpointConnection {
/** @return indicates the type of database account */
DatabaseAccountKind kind();
/** @return the connection endpoint for the CosmosDB database account */
String documentEndpoint();
/** @return the offer type for the CosmosDB database account */
DatabaseAccountOfferType databaseAccountOfferType();
/**
* Whether the CosmosDB account can be accessed from public network.
*
* @return whether the CosmosDB account can be accessed from public network.
*/
PublicNetworkAccess publicNetworkAccess();
/**
* @return specifies the set of IP addresses or IP address ranges in CIDR form.
* @deprecated use {@link #ipRules()}
*/
@Deprecated
String ipRangeFilter();
/** @return specifies the set of IP addresses or IP address ranges in CIDR form. */
List ipRules();
/** @return the consistency policy for the CosmosDB database account */
ConsistencyPolicy consistencyPolicy();
/** @return the default consistency level for the CosmosDB database account */
DefaultConsistencyLevel defaultConsistencyLevel();
/** @return an array that contains the writable georeplication locations enabled for the CosmosDB account */
List writableReplications();
/** @return an array that contains the readable georeplication locations enabled for the CosmosDB account */
List readableReplications();
/** @return the access keys for the specified Azure CosmosDB database account */
DatabaseAccountListKeysResult listKeys();
/** @return the access keys for the specified Azure CosmosDB database account */
Mono listKeysAsync();
/** @return the read-only access keys for the specified Azure CosmosDB database account */
DatabaseAccountListReadOnlyKeysResult listReadOnlyKeys();
/** @return the read-only access keys for the specified Azure CosmosDB database account */
Mono listReadOnlyKeysAsync();
/** @return the connection strings for the specified Azure CosmosDB database account */
DatabaseAccountListConnectionStringsResult listConnectionStrings();
/** @return the connection strings for the specified Azure CosmosDB database account */
Mono listConnectionStringsAsync();
/** @return the list of Azure Cosmos DB SQL databases */
List listSqlDatabases();
/** @return the list of Azure Cosmos DB SQL databases */
PagedFlux listSqlDatabasesAsync();
/** @return whether write is enabled for multiple locations or not */
boolean multipleWriteLocationsEnabled();
/** @return whether cassandra connector is enabled or not. */
boolean cassandraConnectorEnabled();
/** @return the current cassandra connector offer. */
ConnectorOffer cassandraConnectorOffer();
/** @return whether metadata write access is disabled or not. */
boolean keyBasedMetadataWriteAccessDisabled();
/** @return all private link resources in the account. */
PagedFlux listPrivateLinkResourcesAsync();
/** @return all private link resources in the account. */
List listPrivateLinkResources();
/**
* @param groupName group name of private link resource
* @return the specific private link resource group
*/
Mono getPrivateLinkResourceAsync(String groupName);
/**
* @param groupName group name of private link resource
* @return the specific private link resource group
*/
PrivateLinkResource getPrivateLinkResource(String groupName);
/** @return all private endpoint connection in the account. */
Mono
© 2015 - 2024 Weber Informatics LLC | Privacy Policy