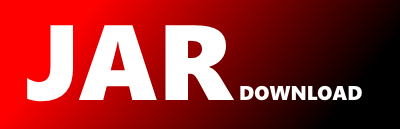
com.azure.resourcemanager.cosmos.models.IndexingPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Cosmos DB indexing policy.
*/
@Fluent
public final class IndexingPolicy implements JsonSerializable {
/*
* Indicates if the indexing policy is automatic
*/
private Boolean automatic;
/*
* Indicates the indexing mode.
*/
private IndexingMode indexingMode;
/*
* List of paths to include in the indexing
*/
private List includedPaths;
/*
* List of paths to exclude from indexing
*/
private List excludedPaths;
/*
* List of composite path list
*/
private List> compositeIndexes;
/*
* List of spatial specifics
*/
private List spatialIndexes;
/**
* Creates an instance of IndexingPolicy class.
*/
public IndexingPolicy() {
}
/**
* Get the automatic property: Indicates if the indexing policy is automatic.
*
* @return the automatic value.
*/
public Boolean automatic() {
return this.automatic;
}
/**
* Set the automatic property: Indicates if the indexing policy is automatic.
*
* @param automatic the automatic value to set.
* @return the IndexingPolicy object itself.
*/
public IndexingPolicy withAutomatic(Boolean automatic) {
this.automatic = automatic;
return this;
}
/**
* Get the indexingMode property: Indicates the indexing mode.
*
* @return the indexingMode value.
*/
public IndexingMode indexingMode() {
return this.indexingMode;
}
/**
* Set the indexingMode property: Indicates the indexing mode.
*
* @param indexingMode the indexingMode value to set.
* @return the IndexingPolicy object itself.
*/
public IndexingPolicy withIndexingMode(IndexingMode indexingMode) {
this.indexingMode = indexingMode;
return this;
}
/**
* Get the includedPaths property: List of paths to include in the indexing.
*
* @return the includedPaths value.
*/
public List includedPaths() {
return this.includedPaths;
}
/**
* Set the includedPaths property: List of paths to include in the indexing.
*
* @param includedPaths the includedPaths value to set.
* @return the IndexingPolicy object itself.
*/
public IndexingPolicy withIncludedPaths(List includedPaths) {
this.includedPaths = includedPaths;
return this;
}
/**
* Get the excludedPaths property: List of paths to exclude from indexing.
*
* @return the excludedPaths value.
*/
public List excludedPaths() {
return this.excludedPaths;
}
/**
* Set the excludedPaths property: List of paths to exclude from indexing.
*
* @param excludedPaths the excludedPaths value to set.
* @return the IndexingPolicy object itself.
*/
public IndexingPolicy withExcludedPaths(List excludedPaths) {
this.excludedPaths = excludedPaths;
return this;
}
/**
* Get the compositeIndexes property: List of composite path list.
*
* @return the compositeIndexes value.
*/
public List> compositeIndexes() {
return this.compositeIndexes;
}
/**
* Set the compositeIndexes property: List of composite path list.
*
* @param compositeIndexes the compositeIndexes value to set.
* @return the IndexingPolicy object itself.
*/
public IndexingPolicy withCompositeIndexes(List> compositeIndexes) {
this.compositeIndexes = compositeIndexes;
return this;
}
/**
* Get the spatialIndexes property: List of spatial specifics.
*
* @return the spatialIndexes value.
*/
public List spatialIndexes() {
return this.spatialIndexes;
}
/**
* Set the spatialIndexes property: List of spatial specifics.
*
* @param spatialIndexes the spatialIndexes value to set.
* @return the IndexingPolicy object itself.
*/
public IndexingPolicy withSpatialIndexes(List spatialIndexes) {
this.spatialIndexes = spatialIndexes;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (includedPaths() != null) {
includedPaths().forEach(e -> e.validate());
}
if (excludedPaths() != null) {
excludedPaths().forEach(e -> e.validate());
}
if (compositeIndexes() != null) {
compositeIndexes().forEach(e -> e.forEach(e1 -> e1.validate()));
}
if (spatialIndexes() != null) {
spatialIndexes().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeBooleanField("automatic", this.automatic);
jsonWriter.writeStringField("indexingMode", this.indexingMode == null ? null : this.indexingMode.toString());
jsonWriter.writeArrayField("includedPaths", this.includedPaths, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("excludedPaths", this.excludedPaths, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("compositeIndexes", this.compositeIndexes,
(writer, element) -> writer.writeArray(element, (writer1, element1) -> writer1.writeJson(element1)));
jsonWriter.writeArrayField("spatialIndexes", this.spatialIndexes,
(writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of IndexingPolicy from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of IndexingPolicy if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the IndexingPolicy.
*/
public static IndexingPolicy fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
IndexingPolicy deserializedIndexingPolicy = new IndexingPolicy();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("automatic".equals(fieldName)) {
deserializedIndexingPolicy.automatic = reader.getNullable(JsonReader::getBoolean);
} else if ("indexingMode".equals(fieldName)) {
deserializedIndexingPolicy.indexingMode = IndexingMode.fromString(reader.getString());
} else if ("includedPaths".equals(fieldName)) {
List includedPaths = reader.readArray(reader1 -> IncludedPath.fromJson(reader1));
deserializedIndexingPolicy.includedPaths = includedPaths;
} else if ("excludedPaths".equals(fieldName)) {
List excludedPaths = reader.readArray(reader1 -> ExcludedPath.fromJson(reader1));
deserializedIndexingPolicy.excludedPaths = excludedPaths;
} else if ("compositeIndexes".equals(fieldName)) {
List> compositeIndexes
= reader.readArray(reader1 -> reader1.readArray(reader2 -> CompositePath.fromJson(reader2)));
deserializedIndexingPolicy.compositeIndexes = compositeIndexes;
} else if ("spatialIndexes".equals(fieldName)) {
List spatialIndexes = reader.readArray(reader1 -> SpatialSpec.fromJson(reader1));
deserializedIndexingPolicy.spatialIndexes = spatialIndexes;
} else {
reader.skipChildren();
}
}
return deserializedIndexingPolicy;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy