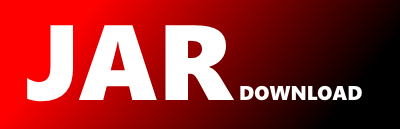
com.azure.resourcemanager.costmanagement.fluent.AlertsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-costmanagement Show documentation
Show all versions of azure-resourcemanager-costmanagement Show documentation
This package contains Microsoft Azure SDK for CostManagement Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. CostManagement management client provides access to CostManagement resources for Azure Enterprise Subscriptions. Package tag package-2022-10.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.costmanagement.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.Response;
import com.azure.core.util.Context;
import com.azure.resourcemanager.costmanagement.fluent.models.AlertInner;
import com.azure.resourcemanager.costmanagement.fluent.models.AlertsResultInner;
import com.azure.resourcemanager.costmanagement.models.DismissAlertPayload;
import com.azure.resourcemanager.costmanagement.models.ExternalCloudProviderType;
/** An instance of this class provides access to all the operations defined in AlertsClient. */
public interface AlertsClient {
/**
* Lists the alerts for scope defined.
*
* @param scope The scope associated with alerts operations. This includes '/subscriptions/{subscriptionId}/' for
* subscription scope, '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department
* scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope, '/providers/Microsoft.Management/managementGroups/{managementGroupId} for
* Management Group scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* billingProfile scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}/invoiceSections/{invoiceSectionId}'
* for invoiceSection scope, and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/customers/{customerId}' specific for
* partners.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return result of alerts along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response listWithResponse(String scope, Context context);
/**
* Lists the alerts for scope defined.
*
* @param scope The scope associated with alerts operations. This includes '/subscriptions/{subscriptionId}/' for
* subscription scope, '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department
* scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope, '/providers/Microsoft.Management/managementGroups/{managementGroupId} for
* Management Group scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* billingProfile scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}/invoiceSections/{invoiceSectionId}'
* for invoiceSection scope, and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/customers/{customerId}' specific for
* partners.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return result of alerts.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
AlertsResultInner list(String scope);
/**
* Gets the alert for the scope by alert ID.
*
* @param scope The scope associated with alerts operations. This includes '/subscriptions/{subscriptionId}/' for
* subscription scope, '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department
* scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope, '/providers/Microsoft.Management/managementGroups/{managementGroupId} for
* Management Group scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* billingProfile scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}/invoiceSections/{invoiceSectionId}'
* for invoiceSection scope, and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/customers/{customerId}' specific for
* partners.
* @param alertId Alert ID.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the alert for the scope by alert ID along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getWithResponse(String scope, String alertId, Context context);
/**
* Gets the alert for the scope by alert ID.
*
* @param scope The scope associated with alerts operations. This includes '/subscriptions/{subscriptionId}/' for
* subscription scope, '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department
* scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope, '/providers/Microsoft.Management/managementGroups/{managementGroupId} for
* Management Group scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* billingProfile scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}/invoiceSections/{invoiceSectionId}'
* for invoiceSection scope, and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/customers/{customerId}' specific for
* partners.
* @param alertId Alert ID.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the alert for the scope by alert ID.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
AlertInner get(String scope, String alertId);
/**
* Dismisses the specified alert.
*
* @param scope The scope associated with alerts operations. This includes '/subscriptions/{subscriptionId}/' for
* subscription scope, '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department
* scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope, '/providers/Microsoft.Management/managementGroups/{managementGroupId} for
* Management Group scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* billingProfile scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}/invoiceSections/{invoiceSectionId}'
* for invoiceSection scope, and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/customers/{customerId}' specific for
* partners.
* @param alertId Alert ID.
* @param parameters Parameters supplied to the Dismiss Alert operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an individual alert along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response dismissWithResponse(
String scope, String alertId, DismissAlertPayload parameters, Context context);
/**
* Dismisses the specified alert.
*
* @param scope The scope associated with alerts operations. This includes '/subscriptions/{subscriptionId}/' for
* subscription scope, '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department
* scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope, '/providers/Microsoft.Management/managementGroups/{managementGroupId} for
* Management Group scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* billingProfile scope,
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}/invoiceSections/{invoiceSectionId}'
* for invoiceSection scope, and
* '/providers/Microsoft.Billing/billingAccounts/{billingAccountId}/customers/{customerId}' specific for
* partners.
* @param alertId Alert ID.
* @param parameters Parameters supplied to the Dismiss Alert operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an individual alert.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
AlertInner dismiss(String scope, String alertId, DismissAlertPayload parameters);
/**
* Lists the Alerts for external cloud provider type defined.
*
* @param externalCloudProviderType The external cloud provider type associated with dimension/query operations.
* This includes 'externalSubscriptions' for linked account and 'externalBillingAccounts' for consolidated
* account.
* @param externalCloudProviderId This can be '{externalSubscriptionId}' for linked account or
* '{externalBillingAccountId}' for consolidated account used with dimension/query operations.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return result of alerts along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response listExternalWithResponse(
ExternalCloudProviderType externalCloudProviderType, String externalCloudProviderId, Context context);
/**
* Lists the Alerts for external cloud provider type defined.
*
* @param externalCloudProviderType The external cloud provider type associated with dimension/query operations.
* This includes 'externalSubscriptions' for linked account and 'externalBillingAccounts' for consolidated
* account.
* @param externalCloudProviderId This can be '{externalSubscriptionId}' for linked account or
* '{externalBillingAccountId}' for consolidated account used with dimension/query operations.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return result of alerts.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
AlertsResultInner listExternal(ExternalCloudProviderType externalCloudProviderType, String externalCloudProviderId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy