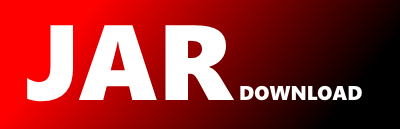
com.azure.resourcemanager.costmanagement.fluent.models.ScheduledActionProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-costmanagement Show documentation
Show all versions of azure-resourcemanager-costmanagement Show documentation
This package contains Microsoft Azure SDK for CostManagement Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. CostManagement management client provides access to CostManagement resources for Azure Enterprise Subscriptions. Package tag package-2022-10.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.costmanagement.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.costmanagement.models.FileDestination;
import com.azure.resourcemanager.costmanagement.models.NotificationProperties;
import com.azure.resourcemanager.costmanagement.models.ScheduleProperties;
import com.azure.resourcemanager.costmanagement.models.ScheduledActionStatus;
import com.fasterxml.jackson.annotation.JsonProperty;
/** The properties of the scheduled action. */
@Fluent
public final class ScheduledActionProperties {
/*
* Scheduled action name.
*/
@JsonProperty(value = "displayName", required = true)
private String displayName;
/*
* Destination format of the view data. This is optional.
*/
@JsonProperty(value = "fileDestination")
private FileDestination fileDestination;
/*
* Notification properties based on scheduled action kind.
*/
@JsonProperty(value = "notification", required = true)
private NotificationProperties notification;
/*
* Email address of the point of contact that should get the unsubscribe requests and notification emails.
*/
@JsonProperty(value = "notificationEmail")
private String notificationEmail;
/*
* Schedule of the scheduled action.
*/
@JsonProperty(value = "schedule", required = true)
private ScheduleProperties schedule;
/*
* Cost Management scope like 'subscriptions/{subscriptionId}' for subscription scope,
* 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department
* scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for
* InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}'
* for ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription
* scope.
*/
@JsonProperty(value = "scope")
private String scope;
/*
* Status of the scheduled action.
*/
@JsonProperty(value = "status", required = true)
private ScheduledActionStatus status;
/*
* Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'
*/
@JsonProperty(value = "viewId", required = true)
private String viewId;
/** Creates an instance of ScheduledActionProperties class. */
public ScheduledActionProperties() {
}
/**
* Get the displayName property: Scheduled action name.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Set the displayName property: Scheduled action name.
*
* @param displayName the displayName value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get the fileDestination property: Destination format of the view data. This is optional.
*
* @return the fileDestination value.
*/
public FileDestination fileDestination() {
return this.fileDestination;
}
/**
* Set the fileDestination property: Destination format of the view data. This is optional.
*
* @param fileDestination the fileDestination value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withFileDestination(FileDestination fileDestination) {
this.fileDestination = fileDestination;
return this;
}
/**
* Get the notification property: Notification properties based on scheduled action kind.
*
* @return the notification value.
*/
public NotificationProperties notification() {
return this.notification;
}
/**
* Set the notification property: Notification properties based on scheduled action kind.
*
* @param notification the notification value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withNotification(NotificationProperties notification) {
this.notification = notification;
return this;
}
/**
* Get the notificationEmail property: Email address of the point of contact that should get the unsubscribe
* requests and notification emails.
*
* @return the notificationEmail value.
*/
public String notificationEmail() {
return this.notificationEmail;
}
/**
* Set the notificationEmail property: Email address of the point of contact that should get the unsubscribe
* requests and notification emails.
*
* @param notificationEmail the notificationEmail value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withNotificationEmail(String notificationEmail) {
this.notificationEmail = notificationEmail;
return this;
}
/**
* Get the schedule property: Schedule of the scheduled action.
*
* @return the schedule value.
*/
public ScheduleProperties schedule() {
return this.schedule;
}
/**
* Set the schedule property: Schedule of the scheduled action.
*
* @param schedule the schedule value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withSchedule(ScheduleProperties schedule) {
this.schedule = schedule;
return this;
}
/**
* Get the scope property: Cost Management scope like 'subscriptions/{subscriptionId}' for subscription scope,
* 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for
* EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for
* InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}'
* for ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription
* scope.
*
* @return the scope value.
*/
public String scope() {
return this.scope;
}
/**
* Set the scope property: Cost Management scope like 'subscriptions/{subscriptionId}' for subscription scope,
* 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for
* EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for
* InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}'
* for ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription
* scope.
*
* @param scope the scope value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withScope(String scope) {
this.scope = scope;
return this;
}
/**
* Get the status property: Status of the scheduled action.
*
* @return the status value.
*/
public ScheduledActionStatus status() {
return this.status;
}
/**
* Set the status property: Status of the scheduled action.
*
* @param status the status value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withStatus(ScheduledActionStatus status) {
this.status = status;
return this;
}
/**
* Get the viewId property: Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'.
*
* @return the viewId value.
*/
public String viewId() {
return this.viewId;
}
/**
* Set the viewId property: Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'.
*
* @param viewId the viewId value to set.
* @return the ScheduledActionProperties object itself.
*/
public ScheduledActionProperties withViewId(String viewId) {
this.viewId = viewId;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (displayName() == null) {
throw LOGGER
.logExceptionAsError(
new IllegalArgumentException(
"Missing required property displayName in model ScheduledActionProperties"));
}
if (fileDestination() != null) {
fileDestination().validate();
}
if (notification() == null) {
throw LOGGER
.logExceptionAsError(
new IllegalArgumentException(
"Missing required property notification in model ScheduledActionProperties"));
} else {
notification().validate();
}
if (schedule() == null) {
throw LOGGER
.logExceptionAsError(
new IllegalArgumentException(
"Missing required property schedule in model ScheduledActionProperties"));
} else {
schedule().validate();
}
if (status() == null) {
throw LOGGER
.logExceptionAsError(
new IllegalArgumentException(
"Missing required property status in model ScheduledActionProperties"));
}
if (viewId() == null) {
throw LOGGER
.logExceptionAsError(
new IllegalArgumentException(
"Missing required property viewId in model ScheduledActionProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(ScheduledActionProperties.class);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy