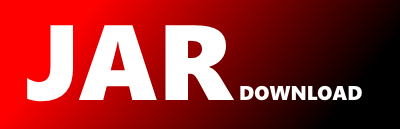
com.azure.resourcemanager.costmanagement.models.ScheduledAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-costmanagement Show documentation
Show all versions of azure-resourcemanager-costmanagement Show documentation
This package contains Microsoft Azure SDK for CostManagement Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. CostManagement management client provides access to CostManagement resources for Azure Enterprise Subscriptions. Package tag package-2022-10.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.costmanagement.models;
import com.azure.core.http.rest.Response;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.costmanagement.fluent.models.ScheduledActionInner;
/** An immutable client-side representation of ScheduledAction. */
public interface ScheduledAction {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the etag property: Resource Etag. For update calls, eTag is optional and can be specified to achieve
* optimistic concurrency. Fetch the resource's eTag by doing a 'GET' call first and then including the latest eTag
* as part of the request body or 'If-Match' header while performing the update. For create calls, eTag is not
* required.
*
* @return the etag value.
*/
String etag();
/**
* Gets the kind property: Kind of the scheduled action.
*
* @return the kind value.
*/
ScheduledActionKind kind();
/**
* Gets the systemData property: Kind of the scheduled action.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the displayName property: Scheduled action name.
*
* @return the displayName value.
*/
String displayName();
/**
* Gets the fileDestination property: Destination format of the view data. This is optional.
*
* @return the fileDestination value.
*/
FileDestination fileDestination();
/**
* Gets the notification property: Notification properties based on scheduled action kind.
*
* @return the notification value.
*/
NotificationProperties notification();
/**
* Gets the notificationEmail property: Email address of the point of contact that should get the unsubscribe
* requests and notification emails.
*
* @return the notificationEmail value.
*/
String notificationEmail();
/**
* Gets the schedule property: Schedule of the scheduled action.
*
* @return the schedule value.
*/
ScheduleProperties schedule();
/**
* Gets the scope property: Cost Management scope like 'subscriptions/{subscriptionId}' for subscription scope,
* 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for
* EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for
* InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}'
* for ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription
* scope.
*
* @return the scope value.
*/
String scope();
/**
* Gets the status property: Status of the scheduled action.
*
* @return the status value.
*/
ScheduledActionStatus status();
/**
* Gets the viewId property: Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'.
*
* @return the viewId value.
*/
String viewId();
/**
* Gets the inner com.azure.resourcemanager.costmanagement.fluent.models.ScheduledActionInner object.
*
* @return the inner object.
*/
ScheduledActionInner innerModel();
/** The entirety of the ScheduledAction definition. */
interface Definition extends DefinitionStages.Blank, DefinitionStages.WithScopeStage, DefinitionStages.WithCreate {
}
/** The ScheduledAction definition stages. */
interface DefinitionStages {
/** The first stage of the ScheduledAction definition. */
interface Blank extends WithScopeStage {
}
/** The stage of the ScheduledAction definition allowing to specify parent resource. */
interface WithScopeStage {
/**
* Specifies scope.
*
* @param scope The scope associated with scheduled action operations. This includes
* 'subscriptions/{subscriptionId}' for subscription scope,
* 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for
* Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}'
* for BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}'
* for InvoiceSection scope,
* 'providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for
* External Billing Account scope and
* 'providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for External
* Subscription scope. Note: Insight Alerts are only available on subscription scope.
* @return the next definition stage.
*/
WithCreate withExistingScope(String scope);
}
/**
* The stage of the ScheduledAction definition which contains all the minimum required properties for the
* resource to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate
extends DefinitionStages.WithKind,
DefinitionStages.WithDisplayName,
DefinitionStages.WithFileDestination,
DefinitionStages.WithNotification,
DefinitionStages.WithNotificationEmail,
DefinitionStages.WithSchedule,
DefinitionStages.WithScope,
DefinitionStages.WithStatus,
DefinitionStages.WithViewId,
DefinitionStages.WithIfMatch {
/**
* Executes the create request.
*
* @return the created resource.
*/
ScheduledAction create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
ScheduledAction create(Context context);
}
/** The stage of the ScheduledAction definition allowing to specify kind. */
interface WithKind {
/**
* Specifies the kind property: Kind of the scheduled action..
*
* @param kind Kind of the scheduled action.
* @return the next definition stage.
*/
WithCreate withKind(ScheduledActionKind kind);
}
/** The stage of the ScheduledAction definition allowing to specify displayName. */
interface WithDisplayName {
/**
* Specifies the displayName property: Scheduled action name..
*
* @param displayName Scheduled action name.
* @return the next definition stage.
*/
WithCreate withDisplayName(String displayName);
}
/** The stage of the ScheduledAction definition allowing to specify fileDestination. */
interface WithFileDestination {
/**
* Specifies the fileDestination property: Destination format of the view data. This is optional..
*
* @param fileDestination Destination format of the view data. This is optional.
* @return the next definition stage.
*/
WithCreate withFileDestination(FileDestination fileDestination);
}
/** The stage of the ScheduledAction definition allowing to specify notification. */
interface WithNotification {
/**
* Specifies the notification property: Notification properties based on scheduled action kind..
*
* @param notification Notification properties based on scheduled action kind.
* @return the next definition stage.
*/
WithCreate withNotification(NotificationProperties notification);
}
/** The stage of the ScheduledAction definition allowing to specify notificationEmail. */
interface WithNotificationEmail {
/**
* Specifies the notificationEmail property: Email address of the point of contact that should get the
* unsubscribe requests and notification emails..
*
* @param notificationEmail Email address of the point of contact that should get the unsubscribe requests
* and notification emails.
* @return the next definition stage.
*/
WithCreate withNotificationEmail(String notificationEmail);
}
/** The stage of the ScheduledAction definition allowing to specify schedule. */
interface WithSchedule {
/**
* Specifies the schedule property: Schedule of the scheduled action..
*
* @param schedule Schedule of the scheduled action.
* @return the next definition stage.
*/
WithCreate withSchedule(ScheduleProperties schedule);
}
/** The stage of the ScheduledAction definition allowing to specify scope. */
interface WithScope {
/**
* Specifies the scope property: Cost Management scope like 'subscriptions/{subscriptionId}' for
* subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for
* Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for
* InvoiceSection scope,
* '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for
* ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for
* ExternalSubscription scope..
*
* @param scope Cost Management scope like 'subscriptions/{subscriptionId}' for subscription scope,
* 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for
* Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}'
* for BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}'
* for InvoiceSection scope,
* '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for
* ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for
* ExternalSubscription scope.
* @return the next definition stage.
*/
WithCreate withScope(String scope);
}
/** The stage of the ScheduledAction definition allowing to specify status. */
interface WithStatus {
/**
* Specifies the status property: Status of the scheduled action..
*
* @param status Status of the scheduled action.
* @return the next definition stage.
*/
WithCreate withStatus(ScheduledActionStatus status);
}
/** The stage of the ScheduledAction definition allowing to specify viewId. */
interface WithViewId {
/**
* Specifies the viewId property: Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'.
*
* @param viewId Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'.
* @return the next definition stage.
*/
WithCreate withViewId(String viewId);
}
/** The stage of the ScheduledAction definition allowing to specify ifMatch. */
interface WithIfMatch {
/**
* Specifies the ifMatch property: ETag of the Entity. Not required when creating an entity. Optional when
* updating an entity and can be specified to achieve optimistic concurrency..
*
* @param ifMatch ETag of the Entity. Not required when creating an entity. Optional when updating an entity
* and can be specified to achieve optimistic concurrency.
* @return the next definition stage.
*/
WithCreate withIfMatch(String ifMatch);
}
}
/**
* Begins update for the ScheduledAction resource.
*
* @return the stage of resource update.
*/
ScheduledAction.Update update();
/** The template for ScheduledAction update. */
interface Update
extends UpdateStages.WithKind,
UpdateStages.WithDisplayName,
UpdateStages.WithFileDestination,
UpdateStages.WithNotification,
UpdateStages.WithNotificationEmail,
UpdateStages.WithSchedule,
UpdateStages.WithScope,
UpdateStages.WithStatus,
UpdateStages.WithViewId,
UpdateStages.WithIfMatch {
/**
* Executes the update request.
*
* @return the updated resource.
*/
ScheduledAction apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
ScheduledAction apply(Context context);
}
/** The ScheduledAction update stages. */
interface UpdateStages {
/** The stage of the ScheduledAction update allowing to specify kind. */
interface WithKind {
/**
* Specifies the kind property: Kind of the scheduled action..
*
* @param kind Kind of the scheduled action.
* @return the next definition stage.
*/
Update withKind(ScheduledActionKind kind);
}
/** The stage of the ScheduledAction update allowing to specify displayName. */
interface WithDisplayName {
/**
* Specifies the displayName property: Scheduled action name..
*
* @param displayName Scheduled action name.
* @return the next definition stage.
*/
Update withDisplayName(String displayName);
}
/** The stage of the ScheduledAction update allowing to specify fileDestination. */
interface WithFileDestination {
/**
* Specifies the fileDestination property: Destination format of the view data. This is optional..
*
* @param fileDestination Destination format of the view data. This is optional.
* @return the next definition stage.
*/
Update withFileDestination(FileDestination fileDestination);
}
/** The stage of the ScheduledAction update allowing to specify notification. */
interface WithNotification {
/**
* Specifies the notification property: Notification properties based on scheduled action kind..
*
* @param notification Notification properties based on scheduled action kind.
* @return the next definition stage.
*/
Update withNotification(NotificationProperties notification);
}
/** The stage of the ScheduledAction update allowing to specify notificationEmail. */
interface WithNotificationEmail {
/**
* Specifies the notificationEmail property: Email address of the point of contact that should get the
* unsubscribe requests and notification emails..
*
* @param notificationEmail Email address of the point of contact that should get the unsubscribe requests
* and notification emails.
* @return the next definition stage.
*/
Update withNotificationEmail(String notificationEmail);
}
/** The stage of the ScheduledAction update allowing to specify schedule. */
interface WithSchedule {
/**
* Specifies the schedule property: Schedule of the scheduled action..
*
* @param schedule Schedule of the scheduled action.
* @return the next definition stage.
*/
Update withSchedule(ScheduleProperties schedule);
}
/** The stage of the ScheduledAction update allowing to specify scope. */
interface WithScope {
/**
* Specifies the scope property: Cost Management scope like 'subscriptions/{subscriptionId}' for
* subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup
* scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for
* Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for
* BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for
* InvoiceSection scope,
* '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for
* ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for
* ExternalSubscription scope..
*
* @param scope Cost Management scope like 'subscriptions/{subscriptionId}' for subscription scope,
* 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for
* Department scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}'
* for EnrollmentAccount scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}'
* for BillingProfile scope,
* 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}'
* for InvoiceSection scope,
* '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for
* ExternalBillingAccount scope, and
* '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for
* ExternalSubscription scope.
* @return the next definition stage.
*/
Update withScope(String scope);
}
/** The stage of the ScheduledAction update allowing to specify status. */
interface WithStatus {
/**
* Specifies the status property: Status of the scheduled action..
*
* @param status Status of the scheduled action.
* @return the next definition stage.
*/
Update withStatus(ScheduledActionStatus status);
}
/** The stage of the ScheduledAction update allowing to specify viewId. */
interface WithViewId {
/**
* Specifies the viewId property: Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'.
*
* @param viewId Cost analysis viewId used for scheduled action. For example,
* '/providers/Microsoft.CostManagement/views/swaggerExample'.
* @return the next definition stage.
*/
Update withViewId(String viewId);
}
/** The stage of the ScheduledAction update allowing to specify ifMatch. */
interface WithIfMatch {
/**
* Specifies the ifMatch property: ETag of the Entity. Not required when creating an entity. Optional when
* updating an entity and can be specified to achieve optimistic concurrency..
*
* @param ifMatch ETag of the Entity. Not required when creating an entity. Optional when updating an entity
* and can be specified to achieve optimistic concurrency.
* @return the next definition stage.
*/
Update withIfMatch(String ifMatch);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
ScheduledAction refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
ScheduledAction refresh(Context context);
/**
* Runs a shared scheduled action within the given scope.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
Response runByScopeWithResponse(Context context);
/**
* Runs a shared scheduled action within the given scope.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void runByScope();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy