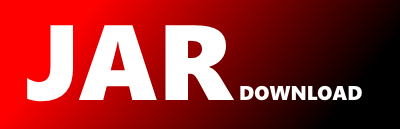
com.azure.resourcemanager.databoxedge.fluent.models.OrderProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.databoxedge.models.Address;
import com.azure.resourcemanager.databoxedge.models.ContactDetails;
import com.azure.resourcemanager.databoxedge.models.OrderStatus;
import com.azure.resourcemanager.databoxedge.models.TrackingInfo;
import java.io.IOException;
import java.util.List;
/**
* Order properties.
*/
@Fluent
public final class OrderProperties implements JsonSerializable {
/*
* The contact details.
*/
private ContactDetails contactInformation;
/*
* The shipping address.
*/
private Address shippingAddress;
/*
* Current status of the order.
*/
private OrderStatus currentStatus;
/*
* List of status changes in the order.
*/
private List orderHistory;
/*
* Serial number of the device.
*/
private String serialNumber;
/*
* Tracking information for the package delivered to the customer whether it has an original or a replacement
* device.
*/
private List deliveryTrackingInfo;
/*
* Tracking information for the package returned from the customer whether it has an original or a replacement
* device.
*/
private List returnTrackingInfo;
/**
* Creates an instance of OrderProperties class.
*/
public OrderProperties() {
}
/**
* Get the contactInformation property: The contact details.
*
* @return the contactInformation value.
*/
public ContactDetails contactInformation() {
return this.contactInformation;
}
/**
* Set the contactInformation property: The contact details.
*
* @param contactInformation the contactInformation value to set.
* @return the OrderProperties object itself.
*/
public OrderProperties withContactInformation(ContactDetails contactInformation) {
this.contactInformation = contactInformation;
return this;
}
/**
* Get the shippingAddress property: The shipping address.
*
* @return the shippingAddress value.
*/
public Address shippingAddress() {
return this.shippingAddress;
}
/**
* Set the shippingAddress property: The shipping address.
*
* @param shippingAddress the shippingAddress value to set.
* @return the OrderProperties object itself.
*/
public OrderProperties withShippingAddress(Address shippingAddress) {
this.shippingAddress = shippingAddress;
return this;
}
/**
* Get the currentStatus property: Current status of the order.
*
* @return the currentStatus value.
*/
public OrderStatus currentStatus() {
return this.currentStatus;
}
/**
* Set the currentStatus property: Current status of the order.
*
* @param currentStatus the currentStatus value to set.
* @return the OrderProperties object itself.
*/
public OrderProperties withCurrentStatus(OrderStatus currentStatus) {
this.currentStatus = currentStatus;
return this;
}
/**
* Get the orderHistory property: List of status changes in the order.
*
* @return the orderHistory value.
*/
public List orderHistory() {
return this.orderHistory;
}
/**
* Get the serialNumber property: Serial number of the device.
*
* @return the serialNumber value.
*/
public String serialNumber() {
return this.serialNumber;
}
/**
* Get the deliveryTrackingInfo property: Tracking information for the package delivered to the customer whether it
* has an original or a replacement device.
*
* @return the deliveryTrackingInfo value.
*/
public List deliveryTrackingInfo() {
return this.deliveryTrackingInfo;
}
/**
* Get the returnTrackingInfo property: Tracking information for the package returned from the customer whether it
* has an original or a replacement device.
*
* @return the returnTrackingInfo value.
*/
public List returnTrackingInfo() {
return this.returnTrackingInfo;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (contactInformation() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property contactInformation in model OrderProperties"));
} else {
contactInformation().validate();
}
if (shippingAddress() == null) {
throw LOGGER.atError()
.log(
new IllegalArgumentException("Missing required property shippingAddress in model OrderProperties"));
} else {
shippingAddress().validate();
}
if (currentStatus() != null) {
currentStatus().validate();
}
if (orderHistory() != null) {
orderHistory().forEach(e -> e.validate());
}
if (deliveryTrackingInfo() != null) {
deliveryTrackingInfo().forEach(e -> e.validate());
}
if (returnTrackingInfo() != null) {
returnTrackingInfo().forEach(e -> e.validate());
}
}
private static final ClientLogger LOGGER = new ClientLogger(OrderProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("contactInformation", this.contactInformation);
jsonWriter.writeJsonField("shippingAddress", this.shippingAddress);
jsonWriter.writeJsonField("currentStatus", this.currentStatus);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of OrderProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of OrderProperties if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the OrderProperties.
*/
public static OrderProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
OrderProperties deserializedOrderProperties = new OrderProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("contactInformation".equals(fieldName)) {
deserializedOrderProperties.contactInformation = ContactDetails.fromJson(reader);
} else if ("shippingAddress".equals(fieldName)) {
deserializedOrderProperties.shippingAddress = Address.fromJson(reader);
} else if ("currentStatus".equals(fieldName)) {
deserializedOrderProperties.currentStatus = OrderStatus.fromJson(reader);
} else if ("orderHistory".equals(fieldName)) {
List orderHistory = reader.readArray(reader1 -> OrderStatus.fromJson(reader1));
deserializedOrderProperties.orderHistory = orderHistory;
} else if ("serialNumber".equals(fieldName)) {
deserializedOrderProperties.serialNumber = reader.getString();
} else if ("deliveryTrackingInfo".equals(fieldName)) {
List deliveryTrackingInfo
= reader.readArray(reader1 -> TrackingInfo.fromJson(reader1));
deserializedOrderProperties.deliveryTrackingInfo = deliveryTrackingInfo;
} else if ("returnTrackingInfo".equals(fieldName)) {
List returnTrackingInfo = reader.readArray(reader1 -> TrackingInfo.fromJson(reader1));
deserializedOrderProperties.returnTrackingInfo = returnTrackingInfo;
} else {
reader.skipChildren();
}
}
return deserializedOrderProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy