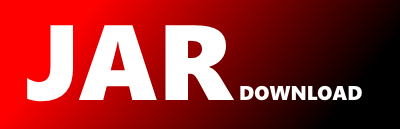
com.azure.resourcemanager.databoxedge.fluent.models.StorageAccountCredentialProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.databoxedge.models.AccountType;
import com.azure.resourcemanager.databoxedge.models.AsymmetricEncryptedSecret;
import com.azure.resourcemanager.databoxedge.models.SslStatus;
import java.io.IOException;
/**
* The storage account credential properties.
*/
@Fluent
public final class StorageAccountCredentialProperties implements JsonSerializable {
/*
* Alias for the storage account.
*/
private String alias;
/*
* Username for the storage account.
*/
private String username;
/*
* Encrypted storage key.
*/
private AsymmetricEncryptedSecret accountKey;
/*
* Connection string for the storage account. Use this string if username and account key are not specified.
*/
private String connectionString;
/*
* Signifies whether SSL needs to be enabled or not.
*/
private SslStatus sslStatus;
/*
* Blob end point for private clouds.
*/
private String blobDomainName;
/*
* Type of storage accessed on the storage account.
*/
private AccountType accountType;
/*
* Id of the storage account.
*/
private String storageAccountId;
/**
* Creates an instance of StorageAccountCredentialProperties class.
*/
public StorageAccountCredentialProperties() {
}
/**
* Get the alias property: Alias for the storage account.
*
* @return the alias value.
*/
public String alias() {
return this.alias;
}
/**
* Set the alias property: Alias for the storage account.
*
* @param alias the alias value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withAlias(String alias) {
this.alias = alias;
return this;
}
/**
* Get the username property: Username for the storage account.
*
* @return the username value.
*/
public String username() {
return this.username;
}
/**
* Set the username property: Username for the storage account.
*
* @param username the username value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withUsername(String username) {
this.username = username;
return this;
}
/**
* Get the accountKey property: Encrypted storage key.
*
* @return the accountKey value.
*/
public AsymmetricEncryptedSecret accountKey() {
return this.accountKey;
}
/**
* Set the accountKey property: Encrypted storage key.
*
* @param accountKey the accountKey value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withAccountKey(AsymmetricEncryptedSecret accountKey) {
this.accountKey = accountKey;
return this;
}
/**
* Get the connectionString property: Connection string for the storage account. Use this string if username and
* account key are not specified.
*
* @return the connectionString value.
*/
public String connectionString() {
return this.connectionString;
}
/**
* Set the connectionString property: Connection string for the storage account. Use this string if username and
* account key are not specified.
*
* @param connectionString the connectionString value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withConnectionString(String connectionString) {
this.connectionString = connectionString;
return this;
}
/**
* Get the sslStatus property: Signifies whether SSL needs to be enabled or not.
*
* @return the sslStatus value.
*/
public SslStatus sslStatus() {
return this.sslStatus;
}
/**
* Set the sslStatus property: Signifies whether SSL needs to be enabled or not.
*
* @param sslStatus the sslStatus value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withSslStatus(SslStatus sslStatus) {
this.sslStatus = sslStatus;
return this;
}
/**
* Get the blobDomainName property: Blob end point for private clouds.
*
* @return the blobDomainName value.
*/
public String blobDomainName() {
return this.blobDomainName;
}
/**
* Set the blobDomainName property: Blob end point for private clouds.
*
* @param blobDomainName the blobDomainName value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withBlobDomainName(String blobDomainName) {
this.blobDomainName = blobDomainName;
return this;
}
/**
* Get the accountType property: Type of storage accessed on the storage account.
*
* @return the accountType value.
*/
public AccountType accountType() {
return this.accountType;
}
/**
* Set the accountType property: Type of storage accessed on the storage account.
*
* @param accountType the accountType value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withAccountType(AccountType accountType) {
this.accountType = accountType;
return this;
}
/**
* Get the storageAccountId property: Id of the storage account.
*
* @return the storageAccountId value.
*/
public String storageAccountId() {
return this.storageAccountId;
}
/**
* Set the storageAccountId property: Id of the storage account.
*
* @param storageAccountId the storageAccountId value to set.
* @return the StorageAccountCredentialProperties object itself.
*/
public StorageAccountCredentialProperties withStorageAccountId(String storageAccountId) {
this.storageAccountId = storageAccountId;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (alias() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property alias in model StorageAccountCredentialProperties"));
}
if (accountKey() != null) {
accountKey().validate();
}
if (sslStatus() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property sslStatus in model StorageAccountCredentialProperties"));
}
if (accountType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property accountType in model StorageAccountCredentialProperties"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(StorageAccountCredentialProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("alias", this.alias);
jsonWriter.writeStringField("sslStatus", this.sslStatus == null ? null : this.sslStatus.toString());
jsonWriter.writeStringField("accountType", this.accountType == null ? null : this.accountType.toString());
jsonWriter.writeStringField("userName", this.username);
jsonWriter.writeJsonField("accountKey", this.accountKey);
jsonWriter.writeStringField("connectionString", this.connectionString);
jsonWriter.writeStringField("blobDomainName", this.blobDomainName);
jsonWriter.writeStringField("storageAccountId", this.storageAccountId);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StorageAccountCredentialProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StorageAccountCredentialProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the StorageAccountCredentialProperties.
*/
public static StorageAccountCredentialProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StorageAccountCredentialProperties deserializedStorageAccountCredentialProperties
= new StorageAccountCredentialProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("alias".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.alias = reader.getString();
} else if ("sslStatus".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.sslStatus = SslStatus.fromString(reader.getString());
} else if ("accountType".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.accountType
= AccountType.fromString(reader.getString());
} else if ("userName".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.username = reader.getString();
} else if ("accountKey".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.accountKey
= AsymmetricEncryptedSecret.fromJson(reader);
} else if ("connectionString".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.connectionString = reader.getString();
} else if ("blobDomainName".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.blobDomainName = reader.getString();
} else if ("storageAccountId".equals(fieldName)) {
deserializedStorageAccountCredentialProperties.storageAccountId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedStorageAccountCredentialProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy