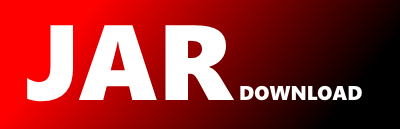
com.azure.resourcemanager.databoxedge.fluent.models.UploadCertificateResponseInner Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.databoxedge.models.AuthenticationType;
import java.io.IOException;
/**
* The upload registration certificate response.
*/
@Fluent
public final class UploadCertificateResponseInner implements JsonSerializable {
/*
* Specifies authentication type.
*/
private AuthenticationType authType;
/*
* The resource ID of the Data Box Edge/Gateway device.
*/
private String resourceId;
/*
* Azure Active Directory tenant authority.
*/
private String aadAuthority;
/*
* Azure Active Directory tenant ID.
*/
private String aadTenantId;
/*
* Azure Active Directory service principal client ID.
*/
private String servicePrincipalClientId;
/*
* Azure Active Directory service principal object ID.
*/
private String servicePrincipalObjectId;
/*
* The azure management endpoint audience.
*/
private String azureManagementEndpointAudience;
/*
* Identifier of the target resource that is the recipient of the requested token.
*/
private String aadAudience;
/**
* Creates an instance of UploadCertificateResponseInner class.
*/
public UploadCertificateResponseInner() {
}
/**
* Get the authType property: Specifies authentication type.
*
* @return the authType value.
*/
public AuthenticationType authType() {
return this.authType;
}
/**
* Set the authType property: Specifies authentication type.
*
* @param authType the authType value to set.
* @return the UploadCertificateResponseInner object itself.
*/
public UploadCertificateResponseInner withAuthType(AuthenticationType authType) {
this.authType = authType;
return this;
}
/**
* Get the resourceId property: The resource ID of the Data Box Edge/Gateway device.
*
* @return the resourceId value.
*/
public String resourceId() {
return this.resourceId;
}
/**
* Get the aadAuthority property: Azure Active Directory tenant authority.
*
* @return the aadAuthority value.
*/
public String aadAuthority() {
return this.aadAuthority;
}
/**
* Get the aadTenantId property: Azure Active Directory tenant ID.
*
* @return the aadTenantId value.
*/
public String aadTenantId() {
return this.aadTenantId;
}
/**
* Get the servicePrincipalClientId property: Azure Active Directory service principal client ID.
*
* @return the servicePrincipalClientId value.
*/
public String servicePrincipalClientId() {
return this.servicePrincipalClientId;
}
/**
* Get the servicePrincipalObjectId property: Azure Active Directory service principal object ID.
*
* @return the servicePrincipalObjectId value.
*/
public String servicePrincipalObjectId() {
return this.servicePrincipalObjectId;
}
/**
* Get the azureManagementEndpointAudience property: The azure management endpoint audience.
*
* @return the azureManagementEndpointAudience value.
*/
public String azureManagementEndpointAudience() {
return this.azureManagementEndpointAudience;
}
/**
* Get the aadAudience property: Identifier of the target resource that is the recipient of the requested token.
*
* @return the aadAudience value.
*/
public String aadAudience() {
return this.aadAudience;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("authType", this.authType == null ? null : this.authType.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of UploadCertificateResponseInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of UploadCertificateResponseInner if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the UploadCertificateResponseInner.
*/
public static UploadCertificateResponseInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
UploadCertificateResponseInner deserializedUploadCertificateResponseInner
= new UploadCertificateResponseInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("authType".equals(fieldName)) {
deserializedUploadCertificateResponseInner.authType
= AuthenticationType.fromString(reader.getString());
} else if ("resourceId".equals(fieldName)) {
deserializedUploadCertificateResponseInner.resourceId = reader.getString();
} else if ("aadAuthority".equals(fieldName)) {
deserializedUploadCertificateResponseInner.aadAuthority = reader.getString();
} else if ("aadTenantId".equals(fieldName)) {
deserializedUploadCertificateResponseInner.aadTenantId = reader.getString();
} else if ("servicePrincipalClientId".equals(fieldName)) {
deserializedUploadCertificateResponseInner.servicePrincipalClientId = reader.getString();
} else if ("servicePrincipalObjectId".equals(fieldName)) {
deserializedUploadCertificateResponseInner.servicePrincipalObjectId = reader.getString();
} else if ("azureManagementEndpointAudience".equals(fieldName)) {
deserializedUploadCertificateResponseInner.azureManagementEndpointAudience = reader.getString();
} else if ("aadAudience".equals(fieldName)) {
deserializedUploadCertificateResponseInner.aadAudience = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedUploadCertificateResponseInner;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy