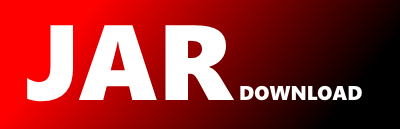
com.azure.resourcemanager.databoxedge.implementation.DevicesClientImpl Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.Delete;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Headers;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.Patch;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.Put;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.databoxedge.fluent.DevicesClient;
import com.azure.resourcemanager.databoxedge.fluent.models.DataBoxEdgeDeviceExtendedInfoInner;
import com.azure.resourcemanager.databoxedge.fluent.models.DataBoxEdgeDeviceInner;
import com.azure.resourcemanager.databoxedge.fluent.models.NetworkSettingsInner;
import com.azure.resourcemanager.databoxedge.fluent.models.UpdateSummaryInner;
import com.azure.resourcemanager.databoxedge.fluent.models.UploadCertificateResponseInner;
import com.azure.resourcemanager.databoxedge.models.DataBoxEdgeDeviceList;
import com.azure.resourcemanager.databoxedge.models.DataBoxEdgeDevicePatch;
import com.azure.resourcemanager.databoxedge.models.SecuritySettings;
import com.azure.resourcemanager.databoxedge.models.UploadCertificateRequest;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in DevicesClient.
*/
public final class DevicesClientImpl implements DevicesClient {
/**
* The proxy service used to perform REST calls.
*/
private final DevicesService service;
/**
* The service client containing this operation class.
*/
private final DataBoxEdgeManagementClientImpl client;
/**
* Initializes an instance of DevicesClientImpl.
*
* @param client the instance of the service client containing this operation class.
*/
DevicesClientImpl(DataBoxEdgeManagementClientImpl client) {
this.service = RestProxy.create(DevicesService.class, client.getHttpPipeline(), client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for DataBoxEdgeManagementClientDevices to be used by the proxy service to
* perform REST calls.
*/
@Host("{$host}")
@ServiceInterface(name = "DataBoxEdgeManagemen")
public interface DevicesService {
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> list(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId, @QueryParam("api-version") String apiVersion,
@QueryParam("$expand") String expand, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByResourceGroup(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@QueryParam("$expand") String expand, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getByResourceGroup(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName,
@PathParam("subscriptionId") String subscriptionId, @PathParam("deviceName") String deviceName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createOrUpdate(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@BodyParam("application/json") DataBoxEdgeDeviceInner dataBoxEdgeDevice,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}")
@ExpectedResponses({ 200, 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> delete(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName,
@PathParam("subscriptionId") String subscriptionId, @PathParam("deviceName") String deviceName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Patch("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> update(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@BodyParam("application/json") DataBoxEdgeDevicePatch parameters, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/downloadUpdates")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> downloadUpdates(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/getExtendedInformation")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getExtendedInformation(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/installUpdates")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> installUpdates(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/networkSettings/default")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getNetworkSettings(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/scanForUpdates")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> scanForUpdates(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/securitySettings/default/update")
@ExpectedResponses({ 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createOrUpdateSecuritySettings(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@BodyParam("application/json") SecuritySettings securitySettings, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/updateSummary/default")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getUpdateSummary(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataBoxEdge/dataBoxEdgeDevices/{deviceName}/uploadCertificate")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> uploadCertificate(@HostParam("$host") String endpoint,
@PathParam("deviceName") String deviceName, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("api-version") String apiVersion,
@BodyParam("application/json") UploadCertificateRequest parameters, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listBySubscriptionNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByResourceGroupNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a subscription.
*
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a subscription along with {@link PagedResponse} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync(String expand) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.list(this.client.getEndpoint(), this.client.getSubscriptionId(),
this.client.getApiVersion(), expand, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a subscription.
*
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a subscription along with {@link PagedResponse} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync(String expand, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.list(this.client.getEndpoint(), this.client.getSubscriptionId(), this.client.getApiVersion(), expand,
accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a subscription.
*
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a subscription as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync(String expand) {
return new PagedFlux<>(() -> listSinglePageAsync(expand),
nextLink -> listBySubscriptionNextSinglePageAsync(nextLink));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a subscription.
*
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a subscription as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync() {
final String expand = null;
return new PagedFlux<>(() -> listSinglePageAsync(expand),
nextLink -> listBySubscriptionNextSinglePageAsync(nextLink));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a subscription.
*
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a subscription as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync(String expand, Context context) {
return new PagedFlux<>(() -> listSinglePageAsync(expand, context),
nextLink -> listBySubscriptionNextSinglePageAsync(nextLink, context));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a subscription.
*
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a subscription as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list() {
final String expand = null;
return new PagedIterable<>(listAsync(expand));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a subscription.
*
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a subscription as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list(String expand, Context context) {
return new PagedIterable<>(listAsync(expand, context));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a resource group.
*
* @param resourceGroupName The resource group name.
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a resource group along with {@link PagedResponse} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupSinglePageAsync(String resourceGroupName,
String expand) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listByResourceGroup(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), expand, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a resource group.
*
* @param resourceGroupName The resource group name.
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a resource group along with {@link PagedResponse} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupSinglePageAsync(String resourceGroupName,
String expand, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listByResourceGroup(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
this.client.getApiVersion(), expand, accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a resource group.
*
* @param resourceGroupName The resource group name.
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a resource group as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByResourceGroupAsync(String resourceGroupName, String expand) {
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName, expand),
nextLink -> listByResourceGroupNextSinglePageAsync(nextLink));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a resource group.
*
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a resource group as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByResourceGroupAsync(String resourceGroupName) {
final String expand = null;
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName, expand),
nextLink -> listByResourceGroupNextSinglePageAsync(nextLink));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a resource group.
*
* @param resourceGroupName The resource group name.
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a resource group as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByResourceGroupAsync(String resourceGroupName, String expand,
Context context) {
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName, expand, context),
nextLink -> listByResourceGroupNextSinglePageAsync(nextLink, context));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a resource group.
*
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a resource group as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByResourceGroup(String resourceGroupName) {
final String expand = null;
return new PagedIterable<>(listByResourceGroupAsync(resourceGroupName, expand));
}
/**
* Gets all the Data Box Edge/Data Box Gateway devices in a resource group.
*
* @param resourceGroupName The resource group name.
* @param expand Specify $expand=details to populate additional fields related to the resource or Specify
* $skipToken=<token> to populate the next page in the list.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Data Box Edge/Data Box Gateway devices in a resource group as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByResourceGroup(String resourceGroupName, String expand,
Context context) {
return new PagedIterable<>(listByResourceGroupAsync(resourceGroupName, expand, context));
}
/**
* Gets the properties of the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of the Data Box Edge/Data Box Gateway device along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getByResourceGroupWithResponseAsync(String resourceGroupName,
String deviceName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getByResourceGroup(this.client.getEndpoint(), resourceGroupName,
this.client.getSubscriptionId(), deviceName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets the properties of the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of the Data Box Edge/Data Box Gateway device along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getByResourceGroupWithResponseAsync(String resourceGroupName,
String deviceName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getByResourceGroup(this.client.getEndpoint(), resourceGroupName, this.client.getSubscriptionId(),
deviceName, this.client.getApiVersion(), accept, context);
}
/**
* Gets the properties of the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of the Data Box Edge/Data Box Gateway device on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getByResourceGroupAsync(String resourceGroupName, String deviceName) {
return getByResourceGroupWithResponseAsync(resourceGroupName, deviceName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets the properties of the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of the Data Box Edge/Data Box Gateway device along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getByResourceGroupWithResponse(String resourceGroupName, String deviceName,
Context context) {
return getByResourceGroupWithResponseAsync(resourceGroupName, deviceName, context).block();
}
/**
* Gets the properties of the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of the Data Box Edge/Data Box Gateway device.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataBoxEdgeDeviceInner getByResourceGroup(String resourceGroupName, String deviceName) {
return getByResourceGroupWithResponse(resourceGroupName, deviceName, Context.NONE).getValue();
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateWithResponseAsync(String deviceName,
String resourceGroupName, DataBoxEdgeDeviceInner dataBoxEdgeDevice) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (dataBoxEdgeDevice == null) {
return Mono
.error(new IllegalArgumentException("Parameter dataBoxEdgeDevice is required and cannot be null."));
} else {
dataBoxEdgeDevice.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createOrUpdate(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), dataBoxEdgeDevice,
accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateWithResponseAsync(String deviceName,
String resourceGroupName, DataBoxEdgeDeviceInner dataBoxEdgeDevice, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (dataBoxEdgeDevice == null) {
return Mono
.error(new IllegalArgumentException("Parameter dataBoxEdgeDevice is required and cannot be null."));
} else {
dataBoxEdgeDevice.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createOrUpdate(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), dataBoxEdgeDevice, accept, context);
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of the Data Box Edge/Gateway device.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, DataBoxEdgeDeviceInner> beginCreateOrUpdateAsync(
String deviceName, String resourceGroupName, DataBoxEdgeDeviceInner dataBoxEdgeDevice) {
Mono>> mono
= createOrUpdateWithResponseAsync(deviceName, resourceGroupName, dataBoxEdgeDevice);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), DataBoxEdgeDeviceInner.class, DataBoxEdgeDeviceInner.class,
this.client.getContext());
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of the Data Box Edge/Gateway device.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, DataBoxEdgeDeviceInner> beginCreateOrUpdateAsync(
String deviceName, String resourceGroupName, DataBoxEdgeDeviceInner dataBoxEdgeDevice, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= createOrUpdateWithResponseAsync(deviceName, resourceGroupName, dataBoxEdgeDevice, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), DataBoxEdgeDeviceInner.class, DataBoxEdgeDeviceInner.class, context);
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of the Data Box Edge/Gateway device.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, DataBoxEdgeDeviceInner> beginCreateOrUpdate(String deviceName,
String resourceGroupName, DataBoxEdgeDeviceInner dataBoxEdgeDevice) {
return this.beginCreateOrUpdateAsync(deviceName, resourceGroupName, dataBoxEdgeDevice).getSyncPoller();
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of the Data Box Edge/Gateway device.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, DataBoxEdgeDeviceInner> beginCreateOrUpdate(String deviceName,
String resourceGroupName, DataBoxEdgeDeviceInner dataBoxEdgeDevice, Context context) {
return this.beginCreateOrUpdateAsync(deviceName, resourceGroupName, dataBoxEdgeDevice, context).getSyncPoller();
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateAsync(String deviceName, String resourceGroupName,
DataBoxEdgeDeviceInner dataBoxEdgeDevice) {
return beginCreateOrUpdateAsync(deviceName, resourceGroupName, dataBoxEdgeDevice).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateAsync(String deviceName, String resourceGroupName,
DataBoxEdgeDeviceInner dataBoxEdgeDevice, Context context) {
return beginCreateOrUpdateAsync(deviceName, resourceGroupName, dataBoxEdgeDevice, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataBoxEdgeDeviceInner createOrUpdate(String deviceName, String resourceGroupName,
DataBoxEdgeDeviceInner dataBoxEdgeDevice) {
return createOrUpdateAsync(deviceName, resourceGroupName, dataBoxEdgeDevice).block();
}
/**
* Creates or updates a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param dataBoxEdgeDevice The resource object.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataBoxEdgeDeviceInner createOrUpdate(String deviceName, String resourceGroupName,
DataBoxEdgeDeviceInner dataBoxEdgeDevice, Context context) {
return createOrUpdateAsync(deviceName, resourceGroupName, dataBoxEdgeDevice, context).block();
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteWithResponseAsync(String resourceGroupName, String deviceName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.delete(this.client.getEndpoint(), resourceGroupName,
this.client.getSubscriptionId(), deviceName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteWithResponseAsync(String resourceGroupName, String deviceName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.delete(this.client.getEndpoint(), resourceGroupName, this.client.getSubscriptionId(), deviceName,
this.client.getApiVersion(), accept, context);
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String deviceName) {
Mono>> mono = deleteWithResponseAsync(resourceGroupName, deviceName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String deviceName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = deleteWithResponseAsync(resourceGroupName, deviceName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String deviceName) {
return this.beginDeleteAsync(resourceGroupName, deviceName).getSyncPoller();
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String deviceName,
Context context) {
return this.beginDeleteAsync(resourceGroupName, deviceName, context).getSyncPoller();
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String deviceName) {
return beginDeleteAsync(resourceGroupName, deviceName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String deviceName, Context context) {
return beginDeleteAsync(resourceGroupName, deviceName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String deviceName) {
deleteAsync(resourceGroupName, deviceName).block();
}
/**
* Deletes the Data Box Edge/Data Box Gateway device.
*
* @param resourceGroupName The resource group name.
* @param deviceName The device name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String deviceName, Context context) {
deleteAsync(resourceGroupName, deviceName, context).block();
}
/**
* Modifies a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The resource parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> updateWithResponseAsync(String deviceName, String resourceGroupName,
DataBoxEdgeDevicePatch parameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.update(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), parameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Modifies a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The resource parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> updateWithResponseAsync(String deviceName, String resourceGroupName,
DataBoxEdgeDevicePatch parameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.update(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(), resourceGroupName,
this.client.getApiVersion(), parameters, accept, context);
}
/**
* Modifies a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The resource parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono updateAsync(String deviceName, String resourceGroupName,
DataBoxEdgeDevicePatch parameters) {
return updateWithResponseAsync(deviceName, resourceGroupName, parameters)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Modifies a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The resource parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response updateWithResponse(String deviceName, String resourceGroupName,
DataBoxEdgeDevicePatch parameters, Context context) {
return updateWithResponseAsync(deviceName, resourceGroupName, parameters, context).block();
}
/**
* Modifies a Data Box Edge/Data Box Gateway resource.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The resource parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Data Box Edge/Gateway device.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataBoxEdgeDeviceInner update(String deviceName, String resourceGroupName,
DataBoxEdgeDevicePatch parameters) {
return updateWithResponse(deviceName, resourceGroupName, parameters, Context.NONE).getValue();
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> downloadUpdatesWithResponseAsync(String deviceName,
String resourceGroupName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.downloadUpdates(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> downloadUpdatesWithResponseAsync(String deviceName,
String resourceGroupName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.downloadUpdates(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), accept, context);
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDownloadUpdatesAsync(String deviceName, String resourceGroupName) {
Mono>> mono = downloadUpdatesWithResponseAsync(deviceName, resourceGroupName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDownloadUpdatesAsync(String deviceName, String resourceGroupName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= downloadUpdatesWithResponseAsync(deviceName, resourceGroupName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDownloadUpdates(String deviceName, String resourceGroupName) {
return this.beginDownloadUpdatesAsync(deviceName, resourceGroupName).getSyncPoller();
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDownloadUpdates(String deviceName, String resourceGroupName,
Context context) {
return this.beginDownloadUpdatesAsync(deviceName, resourceGroupName, context).getSyncPoller();
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono downloadUpdatesAsync(String deviceName, String resourceGroupName) {
return beginDownloadUpdatesAsync(deviceName, resourceGroupName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono downloadUpdatesAsync(String deviceName, String resourceGroupName, Context context) {
return beginDownloadUpdatesAsync(deviceName, resourceGroupName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void downloadUpdates(String deviceName, String resourceGroupName) {
downloadUpdatesAsync(deviceName, resourceGroupName).block();
}
/**
* Downloads the updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void downloadUpdates(String deviceName, String resourceGroupName, Context context) {
downloadUpdatesAsync(deviceName, resourceGroupName, context).block();
}
/**
* Gets additional information for the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return additional information for the specified Data Box Edge/Data Box Gateway device along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
getExtendedInformationWithResponseAsync(String deviceName, String resourceGroupName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getExtendedInformation(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets additional information for the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return additional information for the specified Data Box Edge/Data Box Gateway device along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
getExtendedInformationWithResponseAsync(String deviceName, String resourceGroupName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getExtendedInformation(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), accept, context);
}
/**
* Gets additional information for the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return additional information for the specified Data Box Edge/Data Box Gateway device on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getExtendedInformationAsync(String deviceName,
String resourceGroupName) {
return getExtendedInformationWithResponseAsync(deviceName, resourceGroupName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets additional information for the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return additional information for the specified Data Box Edge/Data Box Gateway device along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getExtendedInformationWithResponse(String deviceName,
String resourceGroupName, Context context) {
return getExtendedInformationWithResponseAsync(deviceName, resourceGroupName, context).block();
}
/**
* Gets additional information for the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return additional information for the specified Data Box Edge/Data Box Gateway device.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataBoxEdgeDeviceExtendedInfoInner getExtendedInformation(String deviceName, String resourceGroupName) {
return getExtendedInformationWithResponse(deviceName, resourceGroupName, Context.NONE).getValue();
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> installUpdatesWithResponseAsync(String deviceName,
String resourceGroupName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.installUpdates(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> installUpdatesWithResponseAsync(String deviceName,
String resourceGroupName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.installUpdates(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), accept, context);
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginInstallUpdatesAsync(String deviceName, String resourceGroupName) {
Mono>> mono = installUpdatesWithResponseAsync(deviceName, resourceGroupName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginInstallUpdatesAsync(String deviceName, String resourceGroupName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = installUpdatesWithResponseAsync(deviceName, resourceGroupName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginInstallUpdates(String deviceName, String resourceGroupName) {
return this.beginInstallUpdatesAsync(deviceName, resourceGroupName).getSyncPoller();
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginInstallUpdates(String deviceName, String resourceGroupName,
Context context) {
return this.beginInstallUpdatesAsync(deviceName, resourceGroupName, context).getSyncPoller();
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono installUpdatesAsync(String deviceName, String resourceGroupName) {
return beginInstallUpdatesAsync(deviceName, resourceGroupName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono installUpdatesAsync(String deviceName, String resourceGroupName, Context context) {
return beginInstallUpdatesAsync(deviceName, resourceGroupName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void installUpdates(String deviceName, String resourceGroupName) {
installUpdatesAsync(deviceName, resourceGroupName).block();
}
/**
* Installs the updates on the Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void installUpdates(String deviceName, String resourceGroupName, Context context) {
installUpdatesAsync(deviceName, resourceGroupName, context).block();
}
/**
* Gets the network settings of the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the network settings of the specified Data Box Edge/Data Box Gateway device along with {@link Response}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getNetworkSettingsWithResponseAsync(String deviceName,
String resourceGroupName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getNetworkSettings(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets the network settings of the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the network settings of the specified Data Box Edge/Data Box Gateway device along with {@link Response}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getNetworkSettingsWithResponseAsync(String deviceName,
String resourceGroupName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getNetworkSettings(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), accept, context);
}
/**
* Gets the network settings of the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the network settings of the specified Data Box Edge/Data Box Gateway device on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getNetworkSettingsAsync(String deviceName, String resourceGroupName) {
return getNetworkSettingsWithResponseAsync(deviceName, resourceGroupName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets the network settings of the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the network settings of the specified Data Box Edge/Data Box Gateway device along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getNetworkSettingsWithResponse(String deviceName, String resourceGroupName,
Context context) {
return getNetworkSettingsWithResponseAsync(deviceName, resourceGroupName, context).block();
}
/**
* Gets the network settings of the specified Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the network settings of the specified Data Box Edge/Data Box Gateway device.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public NetworkSettingsInner getNetworkSettings(String deviceName, String resourceGroupName) {
return getNetworkSettingsWithResponse(deviceName, resourceGroupName, Context.NONE).getValue();
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> scanForUpdatesWithResponseAsync(String deviceName,
String resourceGroupName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.scanForUpdates(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> scanForUpdatesWithResponseAsync(String deviceName,
String resourceGroupName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.scanForUpdates(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), accept, context);
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginScanForUpdatesAsync(String deviceName, String resourceGroupName) {
Mono>> mono = scanForUpdatesWithResponseAsync(deviceName, resourceGroupName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginScanForUpdatesAsync(String deviceName, String resourceGroupName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = scanForUpdatesWithResponseAsync(deviceName, resourceGroupName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginScanForUpdates(String deviceName, String resourceGroupName) {
return this.beginScanForUpdatesAsync(deviceName, resourceGroupName).getSyncPoller();
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginScanForUpdates(String deviceName, String resourceGroupName,
Context context) {
return this.beginScanForUpdatesAsync(deviceName, resourceGroupName, context).getSyncPoller();
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono scanForUpdatesAsync(String deviceName, String resourceGroupName) {
return beginScanForUpdatesAsync(deviceName, resourceGroupName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono scanForUpdatesAsync(String deviceName, String resourceGroupName, Context context) {
return beginScanForUpdatesAsync(deviceName, resourceGroupName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void scanForUpdates(String deviceName, String resourceGroupName) {
scanForUpdatesAsync(deviceName, resourceGroupName).block();
}
/**
* Scans for updates on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void scanForUpdates(String deviceName, String resourceGroupName, Context context) {
scanForUpdatesAsync(deviceName, resourceGroupName, context).block();
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateSecuritySettingsWithResponseAsync(String deviceName,
String resourceGroupName, SecuritySettings securitySettings) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (securitySettings == null) {
return Mono
.error(new IllegalArgumentException("Parameter securitySettings is required and cannot be null."));
} else {
securitySettings.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createOrUpdateSecuritySettings(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), securitySettings,
accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateSecuritySettingsWithResponseAsync(String deviceName,
String resourceGroupName, SecuritySettings securitySettings, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (securitySettings == null) {
return Mono
.error(new IllegalArgumentException("Parameter securitySettings is required and cannot be null."));
} else {
securitySettings.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createOrUpdateSecuritySettings(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), securitySettings, accept,
context);
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginCreateOrUpdateSecuritySettingsAsync(String deviceName,
String resourceGroupName, SecuritySettings securitySettings) {
Mono>> mono
= createOrUpdateSecuritySettingsWithResponseAsync(deviceName, resourceGroupName, securitySettings);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginCreateOrUpdateSecuritySettingsAsync(String deviceName,
String resourceGroupName, SecuritySettings securitySettings, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= createOrUpdateSecuritySettingsWithResponseAsync(deviceName, resourceGroupName, securitySettings, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginCreateOrUpdateSecuritySettings(String deviceName,
String resourceGroupName, SecuritySettings securitySettings) {
return this.beginCreateOrUpdateSecuritySettingsAsync(deviceName, resourceGroupName, securitySettings)
.getSyncPoller();
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginCreateOrUpdateSecuritySettings(String deviceName,
String resourceGroupName, SecuritySettings securitySettings, Context context) {
return this.beginCreateOrUpdateSecuritySettingsAsync(deviceName, resourceGroupName, securitySettings, context)
.getSyncPoller();
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateSecuritySettingsAsync(String deviceName, String resourceGroupName,
SecuritySettings securitySettings) {
return beginCreateOrUpdateSecuritySettingsAsync(deviceName, resourceGroupName, securitySettings).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateSecuritySettingsAsync(String deviceName, String resourceGroupName,
SecuritySettings securitySettings, Context context) {
return beginCreateOrUpdateSecuritySettingsAsync(deviceName, resourceGroupName, securitySettings, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void createOrUpdateSecuritySettings(String deviceName, String resourceGroupName,
SecuritySettings securitySettings) {
createOrUpdateSecuritySettingsAsync(deviceName, resourceGroupName, securitySettings).block();
}
/**
* Updates the security settings on a Data Box Edge/Data Box Gateway device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param securitySettings The security settings.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void createOrUpdateSecuritySettings(String deviceName, String resourceGroupName,
SecuritySettings securitySettings, Context context) {
createOrUpdateSecuritySettingsAsync(deviceName, resourceGroupName, securitySettings, context).block();
}
/**
* Gets information about the availability of updates based on the last scan of the device. It also gets information
* about any ongoing download or install jobs on the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return information about the availability of updates based on the last scan of the device along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getUpdateSummaryWithResponseAsync(String deviceName,
String resourceGroupName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getUpdateSummary(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets information about the availability of updates based on the last scan of the device. It also gets information
* about any ongoing download or install jobs on the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return information about the availability of updates based on the last scan of the device along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getUpdateSummaryWithResponseAsync(String deviceName,
String resourceGroupName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getUpdateSummary(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), accept, context);
}
/**
* Gets information about the availability of updates based on the last scan of the device. It also gets information
* about any ongoing download or install jobs on the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return information about the availability of updates based on the last scan of the device on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getUpdateSummaryAsync(String deviceName, String resourceGroupName) {
return getUpdateSummaryWithResponseAsync(deviceName, resourceGroupName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets information about the availability of updates based on the last scan of the device. It also gets information
* about any ongoing download or install jobs on the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return information about the availability of updates based on the last scan of the device along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getUpdateSummaryWithResponse(String deviceName, String resourceGroupName,
Context context) {
return getUpdateSummaryWithResponseAsync(deviceName, resourceGroupName, context).block();
}
/**
* Gets information about the availability of updates based on the last scan of the device. It also gets information
* about any ongoing download or install jobs on the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return information about the availability of updates based on the last scan of the device.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public UpdateSummaryInner getUpdateSummary(String deviceName, String resourceGroupName) {
return getUpdateSummaryWithResponse(deviceName, resourceGroupName, Context.NONE).getValue();
}
/**
* Uploads registration certificate for the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The upload certificate request.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the upload registration certificate response along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> uploadCertificateWithResponseAsync(String deviceName,
String resourceGroupName, UploadCertificateRequest parameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.uploadCertificate(this.client.getEndpoint(), deviceName,
this.client.getSubscriptionId(), resourceGroupName, this.client.getApiVersion(), parameters, accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Uploads registration certificate for the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The upload certificate request.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the upload registration certificate response along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> uploadCertificateWithResponseAsync(String deviceName,
String resourceGroupName, UploadCertificateRequest parameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (deviceName == null) {
return Mono.error(new IllegalArgumentException("Parameter deviceName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.uploadCertificate(this.client.getEndpoint(), deviceName, this.client.getSubscriptionId(),
resourceGroupName, this.client.getApiVersion(), parameters, accept, context);
}
/**
* Uploads registration certificate for the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The upload certificate request.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the upload registration certificate response on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono uploadCertificateAsync(String deviceName, String resourceGroupName,
UploadCertificateRequest parameters) {
return uploadCertificateWithResponseAsync(deviceName, resourceGroupName, parameters)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Uploads registration certificate for the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The upload certificate request.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the upload registration certificate response along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response uploadCertificateWithResponse(String deviceName,
String resourceGroupName, UploadCertificateRequest parameters, Context context) {
return uploadCertificateWithResponseAsync(deviceName, resourceGroupName, parameters, context).block();
}
/**
* Uploads registration certificate for the device.
*
* @param deviceName The device name.
* @param resourceGroupName The resource group name.
* @param parameters The upload certificate request.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the upload registration certificate response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public UploadCertificateResponseInner uploadCertificate(String deviceName, String resourceGroupName,
UploadCertificateRequest parameters) {
return uploadCertificateWithResponse(deviceName, resourceGroupName, parameters, Context.NONE).getValue();
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the collection of Data Box Edge/Gateway devices along with {@link PagedResponse} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listBySubscriptionNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listBySubscriptionNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the collection of Data Box Edge/Gateway devices along with {@link PagedResponse} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listBySubscriptionNextSinglePageAsync(String nextLink,
Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listBySubscriptionNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the collection of Data Box Edge/Gateway devices along with {@link PagedResponse} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listByResourceGroupNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the collection of Data Box Edge/Gateway devices along with {@link PagedResponse} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupNextSinglePageAsync(String nextLink,
Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listByResourceGroupNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy