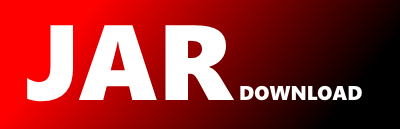
com.azure.resourcemanager.databoxedge.models.Address Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The shipping address of the customer.
*/
@Fluent
public final class Address implements JsonSerializable {
/*
* The address line1.
*/
private String addressLine1;
/*
* The address line2.
*/
private String addressLine2;
/*
* The address line3.
*/
private String addressLine3;
/*
* The postal code.
*/
private String postalCode;
/*
* The city name.
*/
private String city;
/*
* The state name.
*/
private String state;
/*
* The country name.
*/
private String country;
/**
* Creates an instance of Address class.
*/
public Address() {
}
/**
* Get the addressLine1 property: The address line1.
*
* @return the addressLine1 value.
*/
public String addressLine1() {
return this.addressLine1;
}
/**
* Set the addressLine1 property: The address line1.
*
* @param addressLine1 the addressLine1 value to set.
* @return the Address object itself.
*/
public Address withAddressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
return this;
}
/**
* Get the addressLine2 property: The address line2.
*
* @return the addressLine2 value.
*/
public String addressLine2() {
return this.addressLine2;
}
/**
* Set the addressLine2 property: The address line2.
*
* @param addressLine2 the addressLine2 value to set.
* @return the Address object itself.
*/
public Address withAddressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
return this;
}
/**
* Get the addressLine3 property: The address line3.
*
* @return the addressLine3 value.
*/
public String addressLine3() {
return this.addressLine3;
}
/**
* Set the addressLine3 property: The address line3.
*
* @param addressLine3 the addressLine3 value to set.
* @return the Address object itself.
*/
public Address withAddressLine3(String addressLine3) {
this.addressLine3 = addressLine3;
return this;
}
/**
* Get the postalCode property: The postal code.
*
* @return the postalCode value.
*/
public String postalCode() {
return this.postalCode;
}
/**
* Set the postalCode property: The postal code.
*
* @param postalCode the postalCode value to set.
* @return the Address object itself.
*/
public Address withPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
/**
* Get the city property: The city name.
*
* @return the city value.
*/
public String city() {
return this.city;
}
/**
* Set the city property: The city name.
*
* @param city the city value to set.
* @return the Address object itself.
*/
public Address withCity(String city) {
this.city = city;
return this;
}
/**
* Get the state property: The state name.
*
* @return the state value.
*/
public String state() {
return this.state;
}
/**
* Set the state property: The state name.
*
* @param state the state value to set.
* @return the Address object itself.
*/
public Address withState(String state) {
this.state = state;
return this;
}
/**
* Get the country property: The country name.
*
* @return the country value.
*/
public String country() {
return this.country;
}
/**
* Set the country property: The country name.
*
* @param country the country value to set.
* @return the Address object itself.
*/
public Address withCountry(String country) {
this.country = country;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (addressLine1() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property addressLine1 in model Address"));
}
if (postalCode() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property postalCode in model Address"));
}
if (city() == null) {
throw LOGGER.atError().log(new IllegalArgumentException("Missing required property city in model Address"));
}
if (state() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property state in model Address"));
}
if (country() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property country in model Address"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(Address.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("addressLine1", this.addressLine1);
jsonWriter.writeStringField("postalCode", this.postalCode);
jsonWriter.writeStringField("city", this.city);
jsonWriter.writeStringField("state", this.state);
jsonWriter.writeStringField("country", this.country);
jsonWriter.writeStringField("addressLine2", this.addressLine2);
jsonWriter.writeStringField("addressLine3", this.addressLine3);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Address from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Address if the JsonReader was pointing to an instance of it, or null if it was pointing to
* JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the Address.
*/
public static Address fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Address deserializedAddress = new Address();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("addressLine1".equals(fieldName)) {
deserializedAddress.addressLine1 = reader.getString();
} else if ("postalCode".equals(fieldName)) {
deserializedAddress.postalCode = reader.getString();
} else if ("city".equals(fieldName)) {
deserializedAddress.city = reader.getString();
} else if ("state".equals(fieldName)) {
deserializedAddress.state = reader.getString();
} else if ("country".equals(fieldName)) {
deserializedAddress.country = reader.getString();
} else if ("addressLine2".equals(fieldName)) {
deserializedAddress.addressLine2 = reader.getString();
} else if ("addressLine3".equals(fieldName)) {
deserializedAddress.addressLine3 = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedAddress;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy