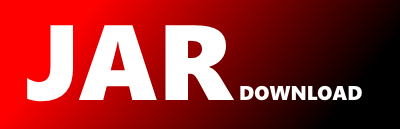
com.azure.resourcemanager.databoxedge.models.NetworkAdapter Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Represents the networkAdapter on a device.
*/
@Fluent
public final class NetworkAdapter implements JsonSerializable {
/*
* Instance ID of network adapter.
*/
private String adapterId;
/*
* Hardware position of network adapter.
*/
private NetworkAdapterPosition adapterPosition;
/*
* Logical index of the adapter.
*/
private Integer index;
/*
* Node ID of the network adapter.
*/
private String nodeId;
/*
* Network adapter name.
*/
private String networkAdapterName;
/*
* Hardware label for the adapter.
*/
private String label;
/*
* MAC address.
*/
private String macAddress;
/*
* Link speed.
*/
private Long linkSpeed;
/*
* Value indicating whether this adapter is valid.
*/
private NetworkAdapterStatus status;
/*
* Value indicating whether this adapter is RDMA capable.
*/
private NetworkAdapterRdmaStatus rdmaStatus;
/*
* Value indicating whether this adapter has DHCP enabled.
*/
private NetworkAdapterDhcpStatus dhcpStatus;
/*
* The IPv4 configuration of the network adapter.
*/
private Ipv4Config ipv4Configuration;
/*
* The IPv6 configuration of the network adapter.
*/
private Ipv6Config ipv6Configuration;
/*
* The IPv6 local address.
*/
private String ipv6LinkLocalAddress;
/*
* The list of DNS Servers of the device.
*/
private List dnsServers;
/**
* Creates an instance of NetworkAdapter class.
*/
public NetworkAdapter() {
}
/**
* Get the adapterId property: Instance ID of network adapter.
*
* @return the adapterId value.
*/
public String adapterId() {
return this.adapterId;
}
/**
* Get the adapterPosition property: Hardware position of network adapter.
*
* @return the adapterPosition value.
*/
public NetworkAdapterPosition adapterPosition() {
return this.adapterPosition;
}
/**
* Get the index property: Logical index of the adapter.
*
* @return the index value.
*/
public Integer index() {
return this.index;
}
/**
* Get the nodeId property: Node ID of the network adapter.
*
* @return the nodeId value.
*/
public String nodeId() {
return this.nodeId;
}
/**
* Get the networkAdapterName property: Network adapter name.
*
* @return the networkAdapterName value.
*/
public String networkAdapterName() {
return this.networkAdapterName;
}
/**
* Get the label property: Hardware label for the adapter.
*
* @return the label value.
*/
public String label() {
return this.label;
}
/**
* Get the macAddress property: MAC address.
*
* @return the macAddress value.
*/
public String macAddress() {
return this.macAddress;
}
/**
* Get the linkSpeed property: Link speed.
*
* @return the linkSpeed value.
*/
public Long linkSpeed() {
return this.linkSpeed;
}
/**
* Get the status property: Value indicating whether this adapter is valid.
*
* @return the status value.
*/
public NetworkAdapterStatus status() {
return this.status;
}
/**
* Get the rdmaStatus property: Value indicating whether this adapter is RDMA capable.
*
* @return the rdmaStatus value.
*/
public NetworkAdapterRdmaStatus rdmaStatus() {
return this.rdmaStatus;
}
/**
* Set the rdmaStatus property: Value indicating whether this adapter is RDMA capable.
*
* @param rdmaStatus the rdmaStatus value to set.
* @return the NetworkAdapter object itself.
*/
public NetworkAdapter withRdmaStatus(NetworkAdapterRdmaStatus rdmaStatus) {
this.rdmaStatus = rdmaStatus;
return this;
}
/**
* Get the dhcpStatus property: Value indicating whether this adapter has DHCP enabled.
*
* @return the dhcpStatus value.
*/
public NetworkAdapterDhcpStatus dhcpStatus() {
return this.dhcpStatus;
}
/**
* Set the dhcpStatus property: Value indicating whether this adapter has DHCP enabled.
*
* @param dhcpStatus the dhcpStatus value to set.
* @return the NetworkAdapter object itself.
*/
public NetworkAdapter withDhcpStatus(NetworkAdapterDhcpStatus dhcpStatus) {
this.dhcpStatus = dhcpStatus;
return this;
}
/**
* Get the ipv4Configuration property: The IPv4 configuration of the network adapter.
*
* @return the ipv4Configuration value.
*/
public Ipv4Config ipv4Configuration() {
return this.ipv4Configuration;
}
/**
* Get the ipv6Configuration property: The IPv6 configuration of the network adapter.
*
* @return the ipv6Configuration value.
*/
public Ipv6Config ipv6Configuration() {
return this.ipv6Configuration;
}
/**
* Get the ipv6LinkLocalAddress property: The IPv6 local address.
*
* @return the ipv6LinkLocalAddress value.
*/
public String ipv6LinkLocalAddress() {
return this.ipv6LinkLocalAddress;
}
/**
* Get the dnsServers property: The list of DNS Servers of the device.
*
* @return the dnsServers value.
*/
public List dnsServers() {
return this.dnsServers;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (adapterPosition() != null) {
adapterPosition().validate();
}
if (ipv4Configuration() != null) {
ipv4Configuration().validate();
}
if (ipv6Configuration() != null) {
ipv6Configuration().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("rdmaStatus", this.rdmaStatus == null ? null : this.rdmaStatus.toString());
jsonWriter.writeStringField("dhcpStatus", this.dhcpStatus == null ? null : this.dhcpStatus.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of NetworkAdapter from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of NetworkAdapter if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the NetworkAdapter.
*/
public static NetworkAdapter fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
NetworkAdapter deserializedNetworkAdapter = new NetworkAdapter();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("adapterId".equals(fieldName)) {
deserializedNetworkAdapter.adapterId = reader.getString();
} else if ("adapterPosition".equals(fieldName)) {
deserializedNetworkAdapter.adapterPosition = NetworkAdapterPosition.fromJson(reader);
} else if ("index".equals(fieldName)) {
deserializedNetworkAdapter.index = reader.getNullable(JsonReader::getInt);
} else if ("nodeId".equals(fieldName)) {
deserializedNetworkAdapter.nodeId = reader.getString();
} else if ("networkAdapterName".equals(fieldName)) {
deserializedNetworkAdapter.networkAdapterName = reader.getString();
} else if ("label".equals(fieldName)) {
deserializedNetworkAdapter.label = reader.getString();
} else if ("macAddress".equals(fieldName)) {
deserializedNetworkAdapter.macAddress = reader.getString();
} else if ("linkSpeed".equals(fieldName)) {
deserializedNetworkAdapter.linkSpeed = reader.getNullable(JsonReader::getLong);
} else if ("status".equals(fieldName)) {
deserializedNetworkAdapter.status = NetworkAdapterStatus.fromString(reader.getString());
} else if ("rdmaStatus".equals(fieldName)) {
deserializedNetworkAdapter.rdmaStatus = NetworkAdapterRdmaStatus.fromString(reader.getString());
} else if ("dhcpStatus".equals(fieldName)) {
deserializedNetworkAdapter.dhcpStatus = NetworkAdapterDhcpStatus.fromString(reader.getString());
} else if ("ipv4Configuration".equals(fieldName)) {
deserializedNetworkAdapter.ipv4Configuration = Ipv4Config.fromJson(reader);
} else if ("ipv6Configuration".equals(fieldName)) {
deserializedNetworkAdapter.ipv6Configuration = Ipv6Config.fromJson(reader);
} else if ("ipv6LinkLocalAddress".equals(fieldName)) {
deserializedNetworkAdapter.ipv6LinkLocalAddress = reader.getString();
} else if ("dnsServers".equals(fieldName)) {
List dnsServers = reader.readArray(reader1 -> reader1.getString());
deserializedNetworkAdapter.dnsServers = dnsServers;
} else {
reader.skipChildren();
}
}
return deserializedNetworkAdapter;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy