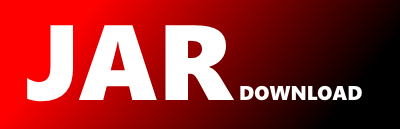
com.azure.resourcemanager.databoxedge.models.UpdateDownloadProgress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-databoxedge Show documentation
Show all versions of azure-resourcemanager-databoxedge Show documentation
This package contains Microsoft Azure SDK for DataBoxEdge Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2019-08.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.models;
import com.azure.core.annotation.Immutable;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Details about the download progress of update.
*/
@Immutable
public final class UpdateDownloadProgress implements JsonSerializable {
/*
* The download phase.
*/
private DownloadPhase downloadPhase;
/*
* Percentage of completion.
*/
private Integer percentComplete;
/*
* Total bytes to download.
*/
private Double totalBytesToDownload;
/*
* Total bytes downloaded.
*/
private Double totalBytesDownloaded;
/*
* Number of updates to download.
*/
private Integer numberOfUpdatesToDownload;
/*
* Number of updates downloaded.
*/
private Integer numberOfUpdatesDownloaded;
/**
* Creates an instance of UpdateDownloadProgress class.
*/
public UpdateDownloadProgress() {
}
/**
* Get the downloadPhase property: The download phase.
*
* @return the downloadPhase value.
*/
public DownloadPhase downloadPhase() {
return this.downloadPhase;
}
/**
* Get the percentComplete property: Percentage of completion.
*
* @return the percentComplete value.
*/
public Integer percentComplete() {
return this.percentComplete;
}
/**
* Get the totalBytesToDownload property: Total bytes to download.
*
* @return the totalBytesToDownload value.
*/
public Double totalBytesToDownload() {
return this.totalBytesToDownload;
}
/**
* Get the totalBytesDownloaded property: Total bytes downloaded.
*
* @return the totalBytesDownloaded value.
*/
public Double totalBytesDownloaded() {
return this.totalBytesDownloaded;
}
/**
* Get the numberOfUpdatesToDownload property: Number of updates to download.
*
* @return the numberOfUpdatesToDownload value.
*/
public Integer numberOfUpdatesToDownload() {
return this.numberOfUpdatesToDownload;
}
/**
* Get the numberOfUpdatesDownloaded property: Number of updates downloaded.
*
* @return the numberOfUpdatesDownloaded value.
*/
public Integer numberOfUpdatesDownloaded() {
return this.numberOfUpdatesDownloaded;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of UpdateDownloadProgress from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of UpdateDownloadProgress if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the UpdateDownloadProgress.
*/
public static UpdateDownloadProgress fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
UpdateDownloadProgress deserializedUpdateDownloadProgress = new UpdateDownloadProgress();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("downloadPhase".equals(fieldName)) {
deserializedUpdateDownloadProgress.downloadPhase = DownloadPhase.fromString(reader.getString());
} else if ("percentComplete".equals(fieldName)) {
deserializedUpdateDownloadProgress.percentComplete = reader.getNullable(JsonReader::getInt);
} else if ("totalBytesToDownload".equals(fieldName)) {
deserializedUpdateDownloadProgress.totalBytesToDownload = reader.getNullable(JsonReader::getDouble);
} else if ("totalBytesDownloaded".equals(fieldName)) {
deserializedUpdateDownloadProgress.totalBytesDownloaded = reader.getNullable(JsonReader::getDouble);
} else if ("numberOfUpdatesToDownload".equals(fieldName)) {
deserializedUpdateDownloadProgress.numberOfUpdatesToDownload
= reader.getNullable(JsonReader::getInt);
} else if ("numberOfUpdatesDownloaded".equals(fieldName)) {
deserializedUpdateDownloadProgress.numberOfUpdatesDownloaded
= reader.getNullable(JsonReader::getInt);
} else {
reader.skipChildren();
}
}
return deserializedUpdateDownloadProgress;
});
}
}