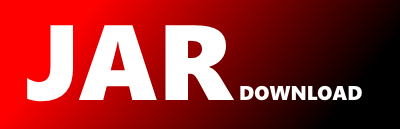
com.azure.resourcemanager.databoxedge.models.UpdateSummary Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.databoxedge.models;
import com.azure.resourcemanager.databoxedge.fluent.models.UpdateSummaryInner;
import java.time.OffsetDateTime;
import java.util.List;
/**
* An immutable client-side representation of UpdateSummary.
*/
public interface UpdateSummary {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the deviceVersionNumber property: The current version of the device in format: 1.2.17312.13.",.
*
* @return the deviceVersionNumber value.
*/
String deviceVersionNumber();
/**
* Gets the friendlyDeviceVersionName property: The current version of the device in text format.
*
* @return the friendlyDeviceVersionName value.
*/
String friendlyDeviceVersionName();
/**
* Gets the deviceLastScannedDateTime property: The last time when a scan was done on the device.
*
* @return the deviceLastScannedDateTime value.
*/
OffsetDateTime deviceLastScannedDateTime();
/**
* Gets the lastCompletedScanJobDateTime property: The time when the last scan job was completed
* (success/cancelled/failed) on the appliance.
*
* @return the lastCompletedScanJobDateTime value.
*/
OffsetDateTime lastCompletedScanJobDateTime();
/**
* Gets the lastCompletedDownloadJobDateTime property: The time when the last Download job was completed
* (success/cancelled/failed) on the appliance.
*
* @return the lastCompletedDownloadJobDateTime value.
*/
OffsetDateTime lastCompletedDownloadJobDateTime();
/**
* Gets the lastCompletedInstallJobDateTime property: The time when the last Install job was completed
* (success/cancelled/failed) on the appliance.
*
* @return the lastCompletedInstallJobDateTime value.
*/
OffsetDateTime lastCompletedInstallJobDateTime();
/**
* Gets the totalNumberOfUpdatesAvailable property: The number of updates available for the current device version
* as per the last device scan.
*
* @return the totalNumberOfUpdatesAvailable value.
*/
Integer totalNumberOfUpdatesAvailable();
/**
* Gets the totalNumberOfUpdatesPendingDownload property: The total number of items pending download.
*
* @return the totalNumberOfUpdatesPendingDownload value.
*/
Integer totalNumberOfUpdatesPendingDownload();
/**
* Gets the totalNumberOfUpdatesPendingInstall property: The total number of items pending install.
*
* @return the totalNumberOfUpdatesPendingInstall value.
*/
Integer totalNumberOfUpdatesPendingInstall();
/**
* Gets the rebootBehavior property: Indicates if updates are available and at least one of the updates needs a
* reboot.
*
* @return the rebootBehavior value.
*/
InstallRebootBehavior rebootBehavior();
/**
* Gets the ongoingUpdateOperation property: The current update operation.
*
* @return the ongoingUpdateOperation value.
*/
UpdateOperation ongoingUpdateOperation();
/**
* Gets the inProgressDownloadJobId property: The job ID of the download job in progress.
*
* @return the inProgressDownloadJobId value.
*/
String inProgressDownloadJobId();
/**
* Gets the inProgressInstallJobId property: The job ID of the install job in progress.
*
* @return the inProgressInstallJobId value.
*/
String inProgressInstallJobId();
/**
* Gets the inProgressDownloadJobStartedDateTime property: The time when the currently running download (if any)
* started.
*
* @return the inProgressDownloadJobStartedDateTime value.
*/
OffsetDateTime inProgressDownloadJobStartedDateTime();
/**
* Gets the inProgressInstallJobStartedDateTime property: The time when the currently running install (if any)
* started.
*
* @return the inProgressInstallJobStartedDateTime value.
*/
OffsetDateTime inProgressInstallJobStartedDateTime();
/**
* Gets the updateTitles property: The list of updates available for install.
*
* @return the updateTitles value.
*/
List updateTitles();
/**
* Gets the totalUpdateSizeInBytes property: The total size of updates available for download in bytes.
*
* @return the totalUpdateSizeInBytes value.
*/
Double totalUpdateSizeInBytes();
/**
* Gets the inner com.azure.resourcemanager.databoxedge.fluent.models.UpdateSummaryInner object.
*
* @return the inner object.
*/
UpdateSummaryInner innerModel();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy