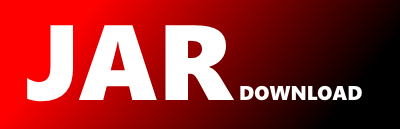
com.azure.resourcemanager.delegatednetwork.fluent.models.DelegatedSubnetInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-delegatednetwork Show documentation
Show all versions of azure-resourcemanager-delegatednetwork Show documentation
This package contains Microsoft Azure SDK for DelegatedNetwork Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. DNC web api provides way to create, get and delete dnc controller. Package tag package-2021-03-15.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.delegatednetwork.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.JsonFlatten;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.delegatednetwork.models.ControllerDetails;
import com.azure.resourcemanager.delegatednetwork.models.DelegatedSubnetResource;
import com.azure.resourcemanager.delegatednetwork.models.DelegatedSubnetState;
import com.azure.resourcemanager.delegatednetwork.models.SubnetDetails;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Map;
/** Represents an instance of a orchestrator. */
@JsonFlatten
@Fluent
public class DelegatedSubnetInner extends DelegatedSubnetResource {
@JsonIgnore private final ClientLogger logger = new ClientLogger(DelegatedSubnetInner.class);
/*
* Resource guid.
*/
@JsonProperty(value = "properties.resourceGuid", access = JsonProperty.Access.WRITE_ONLY)
private String resourceGuid;
/*
* The current state of dnc delegated subnet resource.
*/
@JsonProperty(value = "properties.provisioningState", access = JsonProperty.Access.WRITE_ONLY)
private DelegatedSubnetState provisioningState;
/*
* subnet details
*/
@JsonProperty(value = "properties.subnetDetails")
private SubnetDetails subnetDetails;
/*
* Properties of the controller.
*/
@JsonProperty(value = "properties.controllerDetails")
private ControllerDetails controllerDetails;
/**
* Get the resourceGuid property: Resource guid.
*
* @return the resourceGuid value.
*/
public String resourceGuid() {
return this.resourceGuid;
}
/**
* Get the provisioningState property: The current state of dnc delegated subnet resource.
*
* @return the provisioningState value.
*/
public DelegatedSubnetState provisioningState() {
return this.provisioningState;
}
/**
* Get the subnetDetails property: subnet details.
*
* @return the subnetDetails value.
*/
public SubnetDetails subnetDetails() {
return this.subnetDetails;
}
/**
* Set the subnetDetails property: subnet details.
*
* @param subnetDetails the subnetDetails value to set.
* @return the DelegatedSubnetInner object itself.
*/
public DelegatedSubnetInner withSubnetDetails(SubnetDetails subnetDetails) {
this.subnetDetails = subnetDetails;
return this;
}
/**
* Get the controllerDetails property: Properties of the controller.
*
* @return the controllerDetails value.
*/
public ControllerDetails controllerDetails() {
return this.controllerDetails;
}
/**
* Set the controllerDetails property: Properties of the controller.
*
* @param controllerDetails the controllerDetails value to set.
* @return the DelegatedSubnetInner object itself.
*/
public DelegatedSubnetInner withControllerDetails(ControllerDetails controllerDetails) {
this.controllerDetails = controllerDetails;
return this;
}
/** {@inheritDoc} */
@Override
public DelegatedSubnetInner withLocation(String location) {
super.withLocation(location);
return this;
}
/** {@inheritDoc} */
@Override
public DelegatedSubnetInner withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (subnetDetails() != null) {
subnetDetails().validate();
}
if (controllerDetails() != null) {
controllerDetails().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy