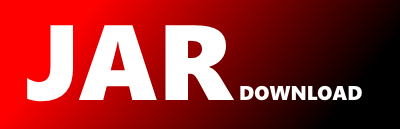
com.azure.resourcemanager.delegatednetwork.implementation.DncImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-delegatednetwork Show documentation
Show all versions of azure-resourcemanager-delegatednetwork Show documentation
This package contains Microsoft Azure SDK for DelegatedNetwork Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. DNC web api provides way to create, get and delete dnc controller. Package tag package-2021-03-15.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.delegatednetwork.implementation;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.http.HttpHeaders;
import com.azure.core.http.HttpPipeline;
import com.azure.core.http.HttpResponse;
import com.azure.core.http.rest.Response;
import com.azure.core.management.AzureEnvironment;
import com.azure.core.management.exception.ManagementError;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.management.polling.PollResult;
import com.azure.core.management.polling.PollerFactory;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.core.util.polling.AsyncPollResponse;
import com.azure.core.util.polling.LongRunningOperationStatus;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.serializer.SerializerAdapter;
import com.azure.core.util.serializer.SerializerEncoding;
import com.azure.resourcemanager.delegatednetwork.fluent.ControllersClient;
import com.azure.resourcemanager.delegatednetwork.fluent.DelegatedNetworksClient;
import com.azure.resourcemanager.delegatednetwork.fluent.DelegatedSubnetServicesClient;
import com.azure.resourcemanager.delegatednetwork.fluent.Dnc;
import com.azure.resourcemanager.delegatednetwork.fluent.OperationsClient;
import com.azure.resourcemanager.delegatednetwork.fluent.OrchestratorInstanceServicesClient;
import java.io.IOException;
import java.lang.reflect.Type;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.time.Duration;
import java.util.Map;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/** Initializes a new instance of the DncImpl type. */
@ServiceClient(builder = DncBuilder.class)
public final class DncImpl implements Dnc {
private final ClientLogger logger = new ClientLogger(DncImpl.class);
/** The ID of the target subscription. */
private final String subscriptionId;
/**
* Gets The ID of the target subscription.
*
* @return the subscriptionId value.
*/
public String getSubscriptionId() {
return this.subscriptionId;
}
/** server parameter. */
private final String endpoint;
/**
* Gets server parameter.
*
* @return the endpoint value.
*/
public String getEndpoint() {
return this.endpoint;
}
/** Api Version. */
private final String apiVersion;
/**
* Gets Api Version.
*
* @return the apiVersion value.
*/
public String getApiVersion() {
return this.apiVersion;
}
/** The HTTP pipeline to send requests through. */
private final HttpPipeline httpPipeline;
/**
* Gets The HTTP pipeline to send requests through.
*
* @return the httpPipeline value.
*/
public HttpPipeline getHttpPipeline() {
return this.httpPipeline;
}
/** The serializer to serialize an object into a string. */
private final SerializerAdapter serializerAdapter;
/**
* Gets The serializer to serialize an object into a string.
*
* @return the serializerAdapter value.
*/
SerializerAdapter getSerializerAdapter() {
return this.serializerAdapter;
}
/** The default poll interval for long-running operation. */
private final Duration defaultPollInterval;
/**
* Gets The default poll interval for long-running operation.
*
* @return the defaultPollInterval value.
*/
public Duration getDefaultPollInterval() {
return this.defaultPollInterval;
}
/** The ControllersClient object to access its operations. */
private final ControllersClient controllers;
/**
* Gets the ControllersClient object to access its operations.
*
* @return the ControllersClient object.
*/
public ControllersClient getControllers() {
return this.controllers;
}
/** The DelegatedNetworksClient object to access its operations. */
private final DelegatedNetworksClient delegatedNetworks;
/**
* Gets the DelegatedNetworksClient object to access its operations.
*
* @return the DelegatedNetworksClient object.
*/
public DelegatedNetworksClient getDelegatedNetworks() {
return this.delegatedNetworks;
}
/** The OrchestratorInstanceServicesClient object to access its operations. */
private final OrchestratorInstanceServicesClient orchestratorInstanceServices;
/**
* Gets the OrchestratorInstanceServicesClient object to access its operations.
*
* @return the OrchestratorInstanceServicesClient object.
*/
public OrchestratorInstanceServicesClient getOrchestratorInstanceServices() {
return this.orchestratorInstanceServices;
}
/** The DelegatedSubnetServicesClient object to access its operations. */
private final DelegatedSubnetServicesClient delegatedSubnetServices;
/**
* Gets the DelegatedSubnetServicesClient object to access its operations.
*
* @return the DelegatedSubnetServicesClient object.
*/
public DelegatedSubnetServicesClient getDelegatedSubnetServices() {
return this.delegatedSubnetServices;
}
/** The OperationsClient object to access its operations. */
private final OperationsClient operations;
/**
* Gets the OperationsClient object to access its operations.
*
* @return the OperationsClient object.
*/
public OperationsClient getOperations() {
return this.operations;
}
/**
* Initializes an instance of Dnc client.
*
* @param httpPipeline The HTTP pipeline to send requests through.
* @param serializerAdapter The serializer to serialize an object into a string.
* @param defaultPollInterval The default poll interval for long-running operation.
* @param environment The Azure environment.
* @param subscriptionId The ID of the target subscription.
* @param endpoint server parameter.
*/
DncImpl(
HttpPipeline httpPipeline,
SerializerAdapter serializerAdapter,
Duration defaultPollInterval,
AzureEnvironment environment,
String subscriptionId,
String endpoint) {
this.httpPipeline = httpPipeline;
this.serializerAdapter = serializerAdapter;
this.defaultPollInterval = defaultPollInterval;
this.subscriptionId = subscriptionId;
this.endpoint = endpoint;
this.apiVersion = "2021-03-15";
this.controllers = new ControllersClientImpl(this);
this.delegatedNetworks = new DelegatedNetworksClientImpl(this);
this.orchestratorInstanceServices = new OrchestratorInstanceServicesClientImpl(this);
this.delegatedSubnetServices = new DelegatedSubnetServicesClientImpl(this);
this.operations = new OperationsClientImpl(this);
}
/**
* Gets default client context.
*
* @return the default client context.
*/
public Context getContext() {
return Context.NONE;
}
/**
* Merges default client context with provided context.
*
* @param context the context to be merged with default client context.
* @return the merged context.
*/
public Context mergeContext(Context context) {
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy