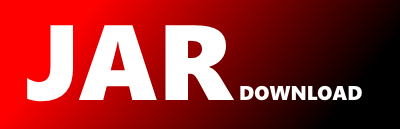
com.azure.resourcemanager.delegatednetwork.models.Orchestrator Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.delegatednetwork.models;
import com.azure.core.management.Region;
import com.azure.core.util.Context;
import com.azure.resourcemanager.delegatednetwork.fluent.models.OrchestratorInner;
import java.util.Map;
/** An immutable client-side representation of Orchestrator. */
public interface Orchestrator {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the location property: The geo-location where the resource lives.
*
* @return the location value.
*/
String location();
/**
* Gets the tags property: Resource tags.
*
* @return the tags value.
*/
Map tags();
/**
* Gets the kind property: The kind of workbook. Choices are user and shared.
*
* @return the kind value.
*/
OrchestratorKind kind();
/**
* Gets the identity property: The identity of the orchestrator.
*
* @return the identity value.
*/
OrchestratorIdentity identity();
/**
* Gets the resourceGuid property: Resource guid.
*
* @return the resourceGuid value.
*/
String resourceGuid();
/**
* Gets the provisioningState property: The current state of orchestratorInstance resource.
*
* @return the provisioningState value.
*/
OrchestratorInstanceState provisioningState();
/**
* Gets the orchestratorAppId property: AAD ID used with apiserver.
*
* @return the orchestratorAppId value.
*/
String orchestratorAppId();
/**
* Gets the orchestratorTenantId property: TenantID of server App ID.
*
* @return the orchestratorTenantId value.
*/
String orchestratorTenantId();
/**
* Gets the clusterRootCA property: RootCA certificate of kubernetes cluster base64 encoded.
*
* @return the clusterRootCA value.
*/
String clusterRootCA();
/**
* Gets the apiServerEndpoint property: K8s APIServer url. Either one of apiServerEndpoint or privateLinkResourceId
* can be specified.
*
* @return the apiServerEndpoint value.
*/
String apiServerEndpoint();
/**
* Gets the privateLinkResourceId property: private link arm resource id. Either one of apiServerEndpoint or
* privateLinkResourceId can be specified.
*
* @return the privateLinkResourceId value.
*/
String privateLinkResourceId();
/**
* Gets the controllerDetails property: Properties of the controller.
*
* @return the controllerDetails value.
*/
ControllerDetails controllerDetails();
/**
* Gets the region of the resource.
*
* @return the region of the resource.
*/
Region region();
/**
* Gets the name of the resource region.
*
* @return the name of the resource region.
*/
String regionName();
/**
* Gets the inner com.azure.resourcemanager.delegatednetwork.fluent.models.OrchestratorInner object.
*
* @return the inner object.
*/
OrchestratorInner innerModel();
/** The entirety of the Orchestrator definition. */
interface Definition
extends DefinitionStages.Blank,
DefinitionStages.WithLocation,
DefinitionStages.WithResourceGroup,
DefinitionStages.WithKind,
DefinitionStages.WithCreate {
}
/** The Orchestrator definition stages. */
interface DefinitionStages {
/** The first stage of the Orchestrator definition. */
interface Blank extends WithLocation {
}
/** The stage of the Orchestrator definition allowing to specify location. */
interface WithLocation {
/**
* Specifies the region for the resource.
*
* @param location The geo-location where the resource lives.
* @return the next definition stage.
*/
WithResourceGroup withRegion(Region location);
/**
* Specifies the region for the resource.
*
* @param location The geo-location where the resource lives.
* @return the next definition stage.
*/
WithResourceGroup withRegion(String location);
}
/** The stage of the Orchestrator definition allowing to specify parent resource. */
interface WithResourceGroup {
/**
* Specifies resourceGroupName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return the next definition stage.
*/
WithKind withExistingResourceGroup(String resourceGroupName);
}
/** The stage of the Orchestrator definition allowing to specify kind. */
interface WithKind {
/**
* Specifies the kind property: The kind of workbook. Choices are user and shared..
*
* @param kind The kind of workbook. Choices are user and shared.
* @return the next definition stage.
*/
WithCreate withKind(OrchestratorKind kind);
}
/**
* The stage of the Orchestrator definition which contains all the minimum required properties for the resource
* to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate
extends DefinitionStages.WithTags,
DefinitionStages.WithIdentity,
DefinitionStages.WithOrchestratorAppId,
DefinitionStages.WithOrchestratorTenantId,
DefinitionStages.WithClusterRootCA,
DefinitionStages.WithApiServerEndpoint,
DefinitionStages.WithPrivateLinkResourceId,
DefinitionStages.WithControllerDetails {
/**
* Executes the create request.
*
* @return the created resource.
*/
Orchestrator create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
Orchestrator create(Context context);
}
/** The stage of the Orchestrator definition allowing to specify tags. */
interface WithTags {
/**
* Specifies the tags property: Resource tags..
*
* @param tags Resource tags.
* @return the next definition stage.
*/
WithCreate withTags(Map tags);
}
/** The stage of the Orchestrator definition allowing to specify identity. */
interface WithIdentity {
/**
* Specifies the identity property: The identity of the orchestrator.
*
* @param identity The identity of the orchestrator.
* @return the next definition stage.
*/
WithCreate withIdentity(OrchestratorIdentity identity);
}
/** The stage of the Orchestrator definition allowing to specify orchestratorAppId. */
interface WithOrchestratorAppId {
/**
* Specifies the orchestratorAppId property: AAD ID used with apiserver.
*
* @param orchestratorAppId AAD ID used with apiserver.
* @return the next definition stage.
*/
WithCreate withOrchestratorAppId(String orchestratorAppId);
}
/** The stage of the Orchestrator definition allowing to specify orchestratorTenantId. */
interface WithOrchestratorTenantId {
/**
* Specifies the orchestratorTenantId property: TenantID of server App ID.
*
* @param orchestratorTenantId TenantID of server App ID.
* @return the next definition stage.
*/
WithCreate withOrchestratorTenantId(String orchestratorTenantId);
}
/** The stage of the Orchestrator definition allowing to specify clusterRootCA. */
interface WithClusterRootCA {
/**
* Specifies the clusterRootCA property: RootCA certificate of kubernetes cluster base64 encoded.
*
* @param clusterRootCA RootCA certificate of kubernetes cluster base64 encoded.
* @return the next definition stage.
*/
WithCreate withClusterRootCA(String clusterRootCA);
}
/** The stage of the Orchestrator definition allowing to specify apiServerEndpoint. */
interface WithApiServerEndpoint {
/**
* Specifies the apiServerEndpoint property: K8s APIServer url. Either one of apiServerEndpoint or
* privateLinkResourceId can be specified.
*
* @param apiServerEndpoint K8s APIServer url. Either one of apiServerEndpoint or privateLinkResourceId can
* be specified.
* @return the next definition stage.
*/
WithCreate withApiServerEndpoint(String apiServerEndpoint);
}
/** The stage of the Orchestrator definition allowing to specify privateLinkResourceId. */
interface WithPrivateLinkResourceId {
/**
* Specifies the privateLinkResourceId property: private link arm resource id. Either one of
* apiServerEndpoint or privateLinkResourceId can be specified.
*
* @param privateLinkResourceId private link arm resource id. Either one of apiServerEndpoint or
* privateLinkResourceId can be specified.
* @return the next definition stage.
*/
WithCreate withPrivateLinkResourceId(String privateLinkResourceId);
}
/** The stage of the Orchestrator definition allowing to specify controllerDetails. */
interface WithControllerDetails {
/**
* Specifies the controllerDetails property: Properties of the controller..
*
* @param controllerDetails Properties of the controller.
* @return the next definition stage.
*/
WithCreate withControllerDetails(ControllerDetails controllerDetails);
}
}
/**
* Begins update for the Orchestrator resource.
*
* @return the stage of resource update.
*/
Orchestrator.Update update();
/** The template for Orchestrator update. */
interface Update extends UpdateStages.WithTags {
/**
* Executes the update request.
*
* @return the updated resource.
*/
Orchestrator apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
Orchestrator apply(Context context);
}
/** The Orchestrator update stages. */
interface UpdateStages {
/** The stage of the Orchestrator update allowing to specify tags. */
interface WithTags {
/**
* Specifies the tags property: The resource tags..
*
* @param tags The resource tags.
* @return the next definition stage.
*/
Update withTags(Map tags);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
Orchestrator refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
Orchestrator refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy