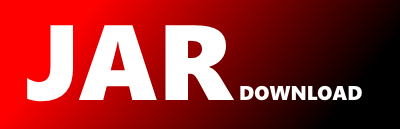
com.azure.resourcemanager.desktopvirtualization.fluent.models.ApplicationPatchProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.desktopvirtualization.models.CommandLineSetting;
import com.azure.resourcemanager.desktopvirtualization.models.RemoteApplicationType;
import java.io.IOException;
/**
* Application properties that can be patched.
*/
@Fluent
public final class ApplicationPatchProperties implements JsonSerializable {
/*
* Description of Application.
*/
private String description;
/*
* Friendly name of Application.
*/
private String friendlyName;
/*
* Specifies a path for the executable file for the application.
*/
private String filePath;
/*
* Specifies whether this published application can be launched with command line arguments provided by the client,
* command line arguments specified at publish time, or no command line arguments at all.
*/
private CommandLineSetting commandLineSetting;
/*
* Command Line Arguments for Application.
*/
private String commandLineArguments;
/*
* Specifies whether to show the RemoteApp program in the RD Web Access server.
*/
private Boolean showInPortal;
/*
* Path to icon.
*/
private String iconPath;
/*
* Index of the icon.
*/
private Integer iconIndex;
/*
* Specifies the package family name for MSIX applications
*/
private String msixPackageFamilyName;
/*
* Specifies the package application Id for MSIX applications
*/
private String msixPackageApplicationId;
/*
* Resource Type of Application.
*/
private RemoteApplicationType applicationType;
/**
* Creates an instance of ApplicationPatchProperties class.
*/
public ApplicationPatchProperties() {
}
/**
* Get the description property: Description of Application.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: Description of Application.
*
* @param description the description value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the friendlyName property: Friendly name of Application.
*
* @return the friendlyName value.
*/
public String friendlyName() {
return this.friendlyName;
}
/**
* Set the friendlyName property: Friendly name of Application.
*
* @param friendlyName the friendlyName value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withFriendlyName(String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* Get the filePath property: Specifies a path for the executable file for the application.
*
* @return the filePath value.
*/
public String filePath() {
return this.filePath;
}
/**
* Set the filePath property: Specifies a path for the executable file for the application.
*
* @param filePath the filePath value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withFilePath(String filePath) {
this.filePath = filePath;
return this;
}
/**
* Get the commandLineSetting property: Specifies whether this published application can be launched with command
* line arguments provided by the client, command line arguments specified at publish time, or no command line
* arguments at all.
*
* @return the commandLineSetting value.
*/
public CommandLineSetting commandLineSetting() {
return this.commandLineSetting;
}
/**
* Set the commandLineSetting property: Specifies whether this published application can be launched with command
* line arguments provided by the client, command line arguments specified at publish time, or no command line
* arguments at all.
*
* @param commandLineSetting the commandLineSetting value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withCommandLineSetting(CommandLineSetting commandLineSetting) {
this.commandLineSetting = commandLineSetting;
return this;
}
/**
* Get the commandLineArguments property: Command Line Arguments for Application.
*
* @return the commandLineArguments value.
*/
public String commandLineArguments() {
return this.commandLineArguments;
}
/**
* Set the commandLineArguments property: Command Line Arguments for Application.
*
* @param commandLineArguments the commandLineArguments value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withCommandLineArguments(String commandLineArguments) {
this.commandLineArguments = commandLineArguments;
return this;
}
/**
* Get the showInPortal property: Specifies whether to show the RemoteApp program in the RD Web Access server.
*
* @return the showInPortal value.
*/
public Boolean showInPortal() {
return this.showInPortal;
}
/**
* Set the showInPortal property: Specifies whether to show the RemoteApp program in the RD Web Access server.
*
* @param showInPortal the showInPortal value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withShowInPortal(Boolean showInPortal) {
this.showInPortal = showInPortal;
return this;
}
/**
* Get the iconPath property: Path to icon.
*
* @return the iconPath value.
*/
public String iconPath() {
return this.iconPath;
}
/**
* Set the iconPath property: Path to icon.
*
* @param iconPath the iconPath value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withIconPath(String iconPath) {
this.iconPath = iconPath;
return this;
}
/**
* Get the iconIndex property: Index of the icon.
*
* @return the iconIndex value.
*/
public Integer iconIndex() {
return this.iconIndex;
}
/**
* Set the iconIndex property: Index of the icon.
*
* @param iconIndex the iconIndex value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withIconIndex(Integer iconIndex) {
this.iconIndex = iconIndex;
return this;
}
/**
* Get the msixPackageFamilyName property: Specifies the package family name for MSIX applications.
*
* @return the msixPackageFamilyName value.
*/
public String msixPackageFamilyName() {
return this.msixPackageFamilyName;
}
/**
* Set the msixPackageFamilyName property: Specifies the package family name for MSIX applications.
*
* @param msixPackageFamilyName the msixPackageFamilyName value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withMsixPackageFamilyName(String msixPackageFamilyName) {
this.msixPackageFamilyName = msixPackageFamilyName;
return this;
}
/**
* Get the msixPackageApplicationId property: Specifies the package application Id for MSIX applications.
*
* @return the msixPackageApplicationId value.
*/
public String msixPackageApplicationId() {
return this.msixPackageApplicationId;
}
/**
* Set the msixPackageApplicationId property: Specifies the package application Id for MSIX applications.
*
* @param msixPackageApplicationId the msixPackageApplicationId value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withMsixPackageApplicationId(String msixPackageApplicationId) {
this.msixPackageApplicationId = msixPackageApplicationId;
return this;
}
/**
* Get the applicationType property: Resource Type of Application.
*
* @return the applicationType value.
*/
public RemoteApplicationType applicationType() {
return this.applicationType;
}
/**
* Set the applicationType property: Resource Type of Application.
*
* @param applicationType the applicationType value to set.
* @return the ApplicationPatchProperties object itself.
*/
public ApplicationPatchProperties withApplicationType(RemoteApplicationType applicationType) {
this.applicationType = applicationType;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeStringField("friendlyName", this.friendlyName);
jsonWriter.writeStringField("filePath", this.filePath);
jsonWriter.writeStringField("commandLineSetting",
this.commandLineSetting == null ? null : this.commandLineSetting.toString());
jsonWriter.writeStringField("commandLineArguments", this.commandLineArguments);
jsonWriter.writeBooleanField("showInPortal", this.showInPortal);
jsonWriter.writeStringField("iconPath", this.iconPath);
jsonWriter.writeNumberField("iconIndex", this.iconIndex);
jsonWriter.writeStringField("msixPackageFamilyName", this.msixPackageFamilyName);
jsonWriter.writeStringField("msixPackageApplicationId", this.msixPackageApplicationId);
jsonWriter.writeStringField("applicationType",
this.applicationType == null ? null : this.applicationType.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ApplicationPatchProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ApplicationPatchProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ApplicationPatchProperties.
*/
public static ApplicationPatchProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ApplicationPatchProperties deserializedApplicationPatchProperties = new ApplicationPatchProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("description".equals(fieldName)) {
deserializedApplicationPatchProperties.description = reader.getString();
} else if ("friendlyName".equals(fieldName)) {
deserializedApplicationPatchProperties.friendlyName = reader.getString();
} else if ("filePath".equals(fieldName)) {
deserializedApplicationPatchProperties.filePath = reader.getString();
} else if ("commandLineSetting".equals(fieldName)) {
deserializedApplicationPatchProperties.commandLineSetting
= CommandLineSetting.fromString(reader.getString());
} else if ("commandLineArguments".equals(fieldName)) {
deserializedApplicationPatchProperties.commandLineArguments = reader.getString();
} else if ("showInPortal".equals(fieldName)) {
deserializedApplicationPatchProperties.showInPortal = reader.getNullable(JsonReader::getBoolean);
} else if ("iconPath".equals(fieldName)) {
deserializedApplicationPatchProperties.iconPath = reader.getString();
} else if ("iconIndex".equals(fieldName)) {
deserializedApplicationPatchProperties.iconIndex = reader.getNullable(JsonReader::getInt);
} else if ("msixPackageFamilyName".equals(fieldName)) {
deserializedApplicationPatchProperties.msixPackageFamilyName = reader.getString();
} else if ("msixPackageApplicationId".equals(fieldName)) {
deserializedApplicationPatchProperties.msixPackageApplicationId = reader.getString();
} else if ("applicationType".equals(fieldName)) {
deserializedApplicationPatchProperties.applicationType
= RemoteApplicationType.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedApplicationPatchProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy