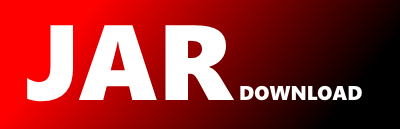
com.azure.resourcemanager.desktopvirtualization.fluent.models.ExpandMsixImageInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.ProxyResource;
import com.azure.core.management.SystemData;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.desktopvirtualization.models.MsixPackageApplications;
import com.azure.resourcemanager.desktopvirtualization.models.MsixPackageDependencies;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.List;
/**
* Represents the definition of contents retrieved after expanding the MSIX Image.
*/
@Fluent
public final class ExpandMsixImageInner extends ProxyResource {
/*
* Detailed properties for ExpandMsixImage
*/
private ExpandMsixImageProperties innerProperties;
/*
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*/
private SystemData systemData;
/*
* The type of the resource.
*/
private String type;
/*
* The name of the resource.
*/
private String name;
/*
* Fully qualified resource Id for the resource.
*/
private String id;
/**
* Creates an instance of ExpandMsixImageInner class.
*/
public ExpandMsixImageInner() {
}
/**
* Get the innerProperties property: Detailed properties for ExpandMsixImage.
*
* @return the innerProperties value.
*/
private ExpandMsixImageProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* Get the type property: The type of the resource.
*
* @return the type value.
*/
@Override
public String type() {
return this.type;
}
/**
* Get the name property: The name of the resource.
*
* @return the name value.
*/
@Override
public String name() {
return this.name;
}
/**
* Get the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
@Override
public String id() {
return this.id;
}
/**
* Get the packageAlias property: Alias of MSIX Package.
*
* @return the packageAlias value.
*/
public String packageAlias() {
return this.innerProperties() == null ? null : this.innerProperties().packageAlias();
}
/**
* Set the packageAlias property: Alias of MSIX Package.
*
* @param packageAlias the packageAlias value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withPackageAlias(String packageAlias) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withPackageAlias(packageAlias);
return this;
}
/**
* Get the imagePath property: VHD/CIM image path on Network Share.
*
* @return the imagePath value.
*/
public String imagePath() {
return this.innerProperties() == null ? null : this.innerProperties().imagePath();
}
/**
* Set the imagePath property: VHD/CIM image path on Network Share.
*
* @param imagePath the imagePath value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withImagePath(String imagePath) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withImagePath(imagePath);
return this;
}
/**
* Get the packageName property: Package Name from appxmanifest.xml.
*
* @return the packageName value.
*/
public String packageName() {
return this.innerProperties() == null ? null : this.innerProperties().packageName();
}
/**
* Set the packageName property: Package Name from appxmanifest.xml.
*
* @param packageName the packageName value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withPackageName(String packageName) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withPackageName(packageName);
return this;
}
/**
* Get the packageFamilyName property: Package Family Name from appxmanifest.xml. Contains Package Name and
* Publisher name.
*
* @return the packageFamilyName value.
*/
public String packageFamilyName() {
return this.innerProperties() == null ? null : this.innerProperties().packageFamilyName();
}
/**
* Set the packageFamilyName property: Package Family Name from appxmanifest.xml. Contains Package Name and
* Publisher name.
*
* @param packageFamilyName the packageFamilyName value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withPackageFamilyName(String packageFamilyName) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withPackageFamilyName(packageFamilyName);
return this;
}
/**
* Get the packageFullName property: Package Full Name from appxmanifest.xml.
*
* @return the packageFullName value.
*/
public String packageFullName() {
return this.innerProperties() == null ? null : this.innerProperties().packageFullName();
}
/**
* Set the packageFullName property: Package Full Name from appxmanifest.xml.
*
* @param packageFullName the packageFullName value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withPackageFullName(String packageFullName) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withPackageFullName(packageFullName);
return this;
}
/**
* Get the displayName property: User friendly Name to be displayed in the portal.
*
* @return the displayName value.
*/
public String displayName() {
return this.innerProperties() == null ? null : this.innerProperties().displayName();
}
/**
* Set the displayName property: User friendly Name to be displayed in the portal.
*
* @param displayName the displayName value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withDisplayName(String displayName) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withDisplayName(displayName);
return this;
}
/**
* Get the packageRelativePath property: Relative Path to the package inside the image.
*
* @return the packageRelativePath value.
*/
public String packageRelativePath() {
return this.innerProperties() == null ? null : this.innerProperties().packageRelativePath();
}
/**
* Set the packageRelativePath property: Relative Path to the package inside the image.
*
* @param packageRelativePath the packageRelativePath value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withPackageRelativePath(String packageRelativePath) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withPackageRelativePath(packageRelativePath);
return this;
}
/**
* Get the isRegularRegistration property: Specifies how to register Package in feed.
*
* @return the isRegularRegistration value.
*/
public Boolean isRegularRegistration() {
return this.innerProperties() == null ? null : this.innerProperties().isRegularRegistration();
}
/**
* Set the isRegularRegistration property: Specifies how to register Package in feed.
*
* @param isRegularRegistration the isRegularRegistration value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withIsRegularRegistration(Boolean isRegularRegistration) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withIsRegularRegistration(isRegularRegistration);
return this;
}
/**
* Get the isActive property: Make this version of the package the active one across the hostpool.
*
* @return the isActive value.
*/
public Boolean isActive() {
return this.innerProperties() == null ? null : this.innerProperties().isActive();
}
/**
* Set the isActive property: Make this version of the package the active one across the hostpool.
*
* @param isActive the isActive value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withIsActive(Boolean isActive) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withIsActive(isActive);
return this;
}
/**
* Get the packageDependencies property: List of package dependencies.
*
* @return the packageDependencies value.
*/
public List packageDependencies() {
return this.innerProperties() == null ? null : this.innerProperties().packageDependencies();
}
/**
* Set the packageDependencies property: List of package dependencies.
*
* @param packageDependencies the packageDependencies value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withPackageDependencies(List packageDependencies) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withPackageDependencies(packageDependencies);
return this;
}
/**
* Get the version property: Package version found in the appxmanifest.xml.
*
* @return the version value.
*/
public String version() {
return this.innerProperties() == null ? null : this.innerProperties().version();
}
/**
* Set the version property: Package version found in the appxmanifest.xml.
*
* @param version the version value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withVersion(String version) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withVersion(version);
return this;
}
/**
* Get the lastUpdated property: Date Package was last updated, found in the appxmanifest.xml.
*
* @return the lastUpdated value.
*/
public OffsetDateTime lastUpdated() {
return this.innerProperties() == null ? null : this.innerProperties().lastUpdated();
}
/**
* Set the lastUpdated property: Date Package was last updated, found in the appxmanifest.xml.
*
* @param lastUpdated the lastUpdated value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withLastUpdated(OffsetDateTime lastUpdated) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withLastUpdated(lastUpdated);
return this;
}
/**
* Get the packageApplications property: List of package applications.
*
* @return the packageApplications value.
*/
public List packageApplications() {
return this.innerProperties() == null ? null : this.innerProperties().packageApplications();
}
/**
* Set the packageApplications property: List of package applications.
*
* @param packageApplications the packageApplications value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withPackageApplications(List packageApplications) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withPackageApplications(packageApplications);
return this;
}
/**
* Get the certificateName property: Certificate name found in the appxmanifest.xml.
*
* @return the certificateName value.
*/
public String certificateName() {
return this.innerProperties() == null ? null : this.innerProperties().certificateName();
}
/**
* Set the certificateName property: Certificate name found in the appxmanifest.xml.
*
* @param certificateName the certificateName value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withCertificateName(String certificateName) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withCertificateName(certificateName);
return this;
}
/**
* Get the certificateExpiry property: Date certificate expires, found in the appxmanifest.xml.
*
* @return the certificateExpiry value.
*/
public OffsetDateTime certificateExpiry() {
return this.innerProperties() == null ? null : this.innerProperties().certificateExpiry();
}
/**
* Set the certificateExpiry property: Date certificate expires, found in the appxmanifest.xml.
*
* @param certificateExpiry the certificateExpiry value to set.
* @return the ExpandMsixImageInner object itself.
*/
public ExpandMsixImageInner withCertificateExpiry(OffsetDateTime certificateExpiry) {
if (this.innerProperties() == null) {
this.innerProperties = new ExpandMsixImageProperties();
}
this.innerProperties().withCertificateExpiry(certificateExpiry);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("properties", this.innerProperties);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ExpandMsixImageInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ExpandMsixImageInner if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ExpandMsixImageInner.
*/
public static ExpandMsixImageInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ExpandMsixImageInner deserializedExpandMsixImageInner = new ExpandMsixImageInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedExpandMsixImageInner.id = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedExpandMsixImageInner.name = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedExpandMsixImageInner.type = reader.getString();
} else if ("properties".equals(fieldName)) {
deserializedExpandMsixImageInner.innerProperties = ExpandMsixImageProperties.fromJson(reader);
} else if ("systemData".equals(fieldName)) {
deserializedExpandMsixImageInner.systemData = SystemData.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedExpandMsixImageInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy