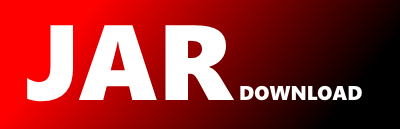
com.azure.resourcemanager.desktopvirtualization.fluent.models.HostPoolPropertiesInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.desktopvirtualization.models.AgentUpdateProperties;
import com.azure.resourcemanager.desktopvirtualization.models.HostpoolPublicNetworkAccess;
import com.azure.resourcemanager.desktopvirtualization.models.HostPoolType;
import com.azure.resourcemanager.desktopvirtualization.models.LoadBalancerType;
import com.azure.resourcemanager.desktopvirtualization.models.PersonalDesktopAssignmentType;
import com.azure.resourcemanager.desktopvirtualization.models.PreferredAppGroupType;
import com.azure.resourcemanager.desktopvirtualization.models.PrivateEndpointConnection;
import com.azure.resourcemanager.desktopvirtualization.models.SsoSecretType;
import java.io.IOException;
import java.util.List;
/**
* Properties of HostPool.
*/
@Fluent
public final class HostPoolPropertiesInner implements JsonSerializable {
/*
* ObjectId of HostPool. (internal use)
*/
private String objectId;
/*
* Friendly name of HostPool.
*/
private String friendlyName;
/*
* Description of HostPool.
*/
private String description;
/*
* HostPool type for desktop.
*/
private HostPoolType hostPoolType;
/*
* PersonalDesktopAssignment type for HostPool.
*/
private PersonalDesktopAssignmentType personalDesktopAssignmentType;
/*
* Custom rdp property of HostPool.
*/
private String customRdpProperty;
/*
* The max session limit of HostPool.
*/
private Integer maxSessionLimit;
/*
* The type of the load balancer.
*/
private LoadBalancerType loadBalancerType;
/*
* The ring number of HostPool.
*/
private Integer ring;
/*
* Is validation environment.
*/
private Boolean validationEnvironment;
/*
* The registration info of HostPool.
*/
private RegistrationInfoInner registrationInfo;
/*
* VM template for sessionhosts configuration within hostpool.
*/
private String vmTemplate;
/*
* List of applicationGroup links.
*/
private List applicationGroupReferences;
/*
* List of App Attach Package links.
*/
private List appAttachPackageReferences;
/*
* URL to customer ADFS server for signing WVD SSO certificates.
*/
private String ssoadfsAuthority;
/*
* ClientId for the registered Relying Party used to issue WVD SSO certificates.
*/
private String ssoClientId;
/*
* Path to Azure KeyVault storing the secret used for communication to ADFS.
*/
private String ssoClientSecretKeyVaultPath;
/*
* The type of single sign on Secret Type.
*/
private SsoSecretType ssoSecretType;
/*
* The type of preferred application group type, default to Desktop Application Group
*/
private PreferredAppGroupType preferredAppGroupType;
/*
* The flag to turn on/off StartVMOnConnect feature.
*/
private Boolean startVMOnConnect;
/*
* Is cloud pc resource.
*/
private Boolean cloudPcResource;
/*
* Enabled allows this resource to be accessed from both public and private networks, Disabled allows this resource
* to only be accessed via private endpoints
*/
private HostpoolPublicNetworkAccess publicNetworkAccess;
/*
* The session host configuration for updating agent, monitoring agent, and stack component.
*/
private AgentUpdateProperties agentUpdate;
/*
* List of private endpoint connection associated with the specified resource
*/
private List privateEndpointConnections;
/**
* Creates an instance of HostPoolPropertiesInner class.
*/
public HostPoolPropertiesInner() {
}
/**
* Get the objectId property: ObjectId of HostPool. (internal use).
*
* @return the objectId value.
*/
public String objectId() {
return this.objectId;
}
/**
* Get the friendlyName property: Friendly name of HostPool.
*
* @return the friendlyName value.
*/
public String friendlyName() {
return this.friendlyName;
}
/**
* Set the friendlyName property: Friendly name of HostPool.
*
* @param friendlyName the friendlyName value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withFriendlyName(String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* Get the description property: Description of HostPool.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: Description of HostPool.
*
* @param description the description value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the hostPoolType property: HostPool type for desktop.
*
* @return the hostPoolType value.
*/
public HostPoolType hostPoolType() {
return this.hostPoolType;
}
/**
* Set the hostPoolType property: HostPool type for desktop.
*
* @param hostPoolType the hostPoolType value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withHostPoolType(HostPoolType hostPoolType) {
this.hostPoolType = hostPoolType;
return this;
}
/**
* Get the personalDesktopAssignmentType property: PersonalDesktopAssignment type for HostPool.
*
* @return the personalDesktopAssignmentType value.
*/
public PersonalDesktopAssignmentType personalDesktopAssignmentType() {
return this.personalDesktopAssignmentType;
}
/**
* Set the personalDesktopAssignmentType property: PersonalDesktopAssignment type for HostPool.
*
* @param personalDesktopAssignmentType the personalDesktopAssignmentType value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner
withPersonalDesktopAssignmentType(PersonalDesktopAssignmentType personalDesktopAssignmentType) {
this.personalDesktopAssignmentType = personalDesktopAssignmentType;
return this;
}
/**
* Get the customRdpProperty property: Custom rdp property of HostPool.
*
* @return the customRdpProperty value.
*/
public String customRdpProperty() {
return this.customRdpProperty;
}
/**
* Set the customRdpProperty property: Custom rdp property of HostPool.
*
* @param customRdpProperty the customRdpProperty value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withCustomRdpProperty(String customRdpProperty) {
this.customRdpProperty = customRdpProperty;
return this;
}
/**
* Get the maxSessionLimit property: The max session limit of HostPool.
*
* @return the maxSessionLimit value.
*/
public Integer maxSessionLimit() {
return this.maxSessionLimit;
}
/**
* Set the maxSessionLimit property: The max session limit of HostPool.
*
* @param maxSessionLimit the maxSessionLimit value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withMaxSessionLimit(Integer maxSessionLimit) {
this.maxSessionLimit = maxSessionLimit;
return this;
}
/**
* Get the loadBalancerType property: The type of the load balancer.
*
* @return the loadBalancerType value.
*/
public LoadBalancerType loadBalancerType() {
return this.loadBalancerType;
}
/**
* Set the loadBalancerType property: The type of the load balancer.
*
* @param loadBalancerType the loadBalancerType value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withLoadBalancerType(LoadBalancerType loadBalancerType) {
this.loadBalancerType = loadBalancerType;
return this;
}
/**
* Get the ring property: The ring number of HostPool.
*
* @return the ring value.
*/
public Integer ring() {
return this.ring;
}
/**
* Set the ring property: The ring number of HostPool.
*
* @param ring the ring value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withRing(Integer ring) {
this.ring = ring;
return this;
}
/**
* Get the validationEnvironment property: Is validation environment.
*
* @return the validationEnvironment value.
*/
public Boolean validationEnvironment() {
return this.validationEnvironment;
}
/**
* Set the validationEnvironment property: Is validation environment.
*
* @param validationEnvironment the validationEnvironment value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withValidationEnvironment(Boolean validationEnvironment) {
this.validationEnvironment = validationEnvironment;
return this;
}
/**
* Get the registrationInfo property: The registration info of HostPool.
*
* @return the registrationInfo value.
*/
public RegistrationInfoInner registrationInfo() {
return this.registrationInfo;
}
/**
* Set the registrationInfo property: The registration info of HostPool.
*
* @param registrationInfo the registrationInfo value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withRegistrationInfo(RegistrationInfoInner registrationInfo) {
this.registrationInfo = registrationInfo;
return this;
}
/**
* Get the vmTemplate property: VM template for sessionhosts configuration within hostpool.
*
* @return the vmTemplate value.
*/
public String vmTemplate() {
return this.vmTemplate;
}
/**
* Set the vmTemplate property: VM template for sessionhosts configuration within hostpool.
*
* @param vmTemplate the vmTemplate value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withVmTemplate(String vmTemplate) {
this.vmTemplate = vmTemplate;
return this;
}
/**
* Get the applicationGroupReferences property: List of applicationGroup links.
*
* @return the applicationGroupReferences value.
*/
public List applicationGroupReferences() {
return this.applicationGroupReferences;
}
/**
* Get the appAttachPackageReferences property: List of App Attach Package links.
*
* @return the appAttachPackageReferences value.
*/
public List appAttachPackageReferences() {
return this.appAttachPackageReferences;
}
/**
* Get the ssoadfsAuthority property: URL to customer ADFS server for signing WVD SSO certificates.
*
* @return the ssoadfsAuthority value.
*/
public String ssoadfsAuthority() {
return this.ssoadfsAuthority;
}
/**
* Set the ssoadfsAuthority property: URL to customer ADFS server for signing WVD SSO certificates.
*
* @param ssoadfsAuthority the ssoadfsAuthority value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withSsoadfsAuthority(String ssoadfsAuthority) {
this.ssoadfsAuthority = ssoadfsAuthority;
return this;
}
/**
* Get the ssoClientId property: ClientId for the registered Relying Party used to issue WVD SSO certificates.
*
* @return the ssoClientId value.
*/
public String ssoClientId() {
return this.ssoClientId;
}
/**
* Set the ssoClientId property: ClientId for the registered Relying Party used to issue WVD SSO certificates.
*
* @param ssoClientId the ssoClientId value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withSsoClientId(String ssoClientId) {
this.ssoClientId = ssoClientId;
return this;
}
/**
* Get the ssoClientSecretKeyVaultPath property: Path to Azure KeyVault storing the secret used for communication to
* ADFS.
*
* @return the ssoClientSecretKeyVaultPath value.
*/
public String ssoClientSecretKeyVaultPath() {
return this.ssoClientSecretKeyVaultPath;
}
/**
* Set the ssoClientSecretKeyVaultPath property: Path to Azure KeyVault storing the secret used for communication to
* ADFS.
*
* @param ssoClientSecretKeyVaultPath the ssoClientSecretKeyVaultPath value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withSsoClientSecretKeyVaultPath(String ssoClientSecretKeyVaultPath) {
this.ssoClientSecretKeyVaultPath = ssoClientSecretKeyVaultPath;
return this;
}
/**
* Get the ssoSecretType property: The type of single sign on Secret Type.
*
* @return the ssoSecretType value.
*/
public SsoSecretType ssoSecretType() {
return this.ssoSecretType;
}
/**
* Set the ssoSecretType property: The type of single sign on Secret Type.
*
* @param ssoSecretType the ssoSecretType value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withSsoSecretType(SsoSecretType ssoSecretType) {
this.ssoSecretType = ssoSecretType;
return this;
}
/**
* Get the preferredAppGroupType property: The type of preferred application group type, default to Desktop
* Application Group.
*
* @return the preferredAppGroupType value.
*/
public PreferredAppGroupType preferredAppGroupType() {
return this.preferredAppGroupType;
}
/**
* Set the preferredAppGroupType property: The type of preferred application group type, default to Desktop
* Application Group.
*
* @param preferredAppGroupType the preferredAppGroupType value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withPreferredAppGroupType(PreferredAppGroupType preferredAppGroupType) {
this.preferredAppGroupType = preferredAppGroupType;
return this;
}
/**
* Get the startVMOnConnect property: The flag to turn on/off StartVMOnConnect feature.
*
* @return the startVMOnConnect value.
*/
public Boolean startVMOnConnect() {
return this.startVMOnConnect;
}
/**
* Set the startVMOnConnect property: The flag to turn on/off StartVMOnConnect feature.
*
* @param startVMOnConnect the startVMOnConnect value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withStartVMOnConnect(Boolean startVMOnConnect) {
this.startVMOnConnect = startVMOnConnect;
return this;
}
/**
* Get the cloudPcResource property: Is cloud pc resource.
*
* @return the cloudPcResource value.
*/
public Boolean cloudPcResource() {
return this.cloudPcResource;
}
/**
* Get the publicNetworkAccess property: Enabled allows this resource to be accessed from both public and private
* networks, Disabled allows this resource to only be accessed via private endpoints.
*
* @return the publicNetworkAccess value.
*/
public HostpoolPublicNetworkAccess publicNetworkAccess() {
return this.publicNetworkAccess;
}
/**
* Set the publicNetworkAccess property: Enabled allows this resource to be accessed from both public and private
* networks, Disabled allows this resource to only be accessed via private endpoints.
*
* @param publicNetworkAccess the publicNetworkAccess value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withPublicNetworkAccess(HostpoolPublicNetworkAccess publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
/**
* Get the agentUpdate property: The session host configuration for updating agent, monitoring agent, and stack
* component.
*
* @return the agentUpdate value.
*/
public AgentUpdateProperties agentUpdate() {
return this.agentUpdate;
}
/**
* Set the agentUpdate property: The session host configuration for updating agent, monitoring agent, and stack
* component.
*
* @param agentUpdate the agentUpdate value to set.
* @return the HostPoolPropertiesInner object itself.
*/
public HostPoolPropertiesInner withAgentUpdate(AgentUpdateProperties agentUpdate) {
this.agentUpdate = agentUpdate;
return this;
}
/**
* Get the privateEndpointConnections property: List of private endpoint connection associated with the specified
* resource.
*
* @return the privateEndpointConnections value.
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (hostPoolType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property hostPoolType in model HostPoolPropertiesInner"));
}
if (loadBalancerType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property loadBalancerType in model HostPoolPropertiesInner"));
}
if (registrationInfo() != null) {
registrationInfo().validate();
}
if (preferredAppGroupType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property preferredAppGroupType in model HostPoolPropertiesInner"));
}
if (agentUpdate() != null) {
agentUpdate().validate();
}
if (privateEndpointConnections() != null) {
privateEndpointConnections().forEach(e -> e.validate());
}
}
private static final ClientLogger LOGGER = new ClientLogger(HostPoolPropertiesInner.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("hostPoolType", this.hostPoolType == null ? null : this.hostPoolType.toString());
jsonWriter.writeStringField("loadBalancerType",
this.loadBalancerType == null ? null : this.loadBalancerType.toString());
jsonWriter.writeStringField("preferredAppGroupType",
this.preferredAppGroupType == null ? null : this.preferredAppGroupType.toString());
jsonWriter.writeStringField("friendlyName", this.friendlyName);
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeStringField("personalDesktopAssignmentType",
this.personalDesktopAssignmentType == null ? null : this.personalDesktopAssignmentType.toString());
jsonWriter.writeStringField("customRdpProperty", this.customRdpProperty);
jsonWriter.writeNumberField("maxSessionLimit", this.maxSessionLimit);
jsonWriter.writeNumberField("ring", this.ring);
jsonWriter.writeBooleanField("validationEnvironment", this.validationEnvironment);
jsonWriter.writeJsonField("registrationInfo", this.registrationInfo);
jsonWriter.writeStringField("vmTemplate", this.vmTemplate);
jsonWriter.writeStringField("ssoadfsAuthority", this.ssoadfsAuthority);
jsonWriter.writeStringField("ssoClientId", this.ssoClientId);
jsonWriter.writeStringField("ssoClientSecretKeyVaultPath", this.ssoClientSecretKeyVaultPath);
jsonWriter.writeStringField("ssoSecretType", this.ssoSecretType == null ? null : this.ssoSecretType.toString());
jsonWriter.writeBooleanField("startVMOnConnect", this.startVMOnConnect);
jsonWriter.writeStringField("publicNetworkAccess",
this.publicNetworkAccess == null ? null : this.publicNetworkAccess.toString());
jsonWriter.writeJsonField("agentUpdate", this.agentUpdate);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of HostPoolPropertiesInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of HostPoolPropertiesInner if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the HostPoolPropertiesInner.
*/
public static HostPoolPropertiesInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
HostPoolPropertiesInner deserializedHostPoolPropertiesInner = new HostPoolPropertiesInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("hostPoolType".equals(fieldName)) {
deserializedHostPoolPropertiesInner.hostPoolType = HostPoolType.fromString(reader.getString());
} else if ("loadBalancerType".equals(fieldName)) {
deserializedHostPoolPropertiesInner.loadBalancerType
= LoadBalancerType.fromString(reader.getString());
} else if ("preferredAppGroupType".equals(fieldName)) {
deserializedHostPoolPropertiesInner.preferredAppGroupType
= PreferredAppGroupType.fromString(reader.getString());
} else if ("objectId".equals(fieldName)) {
deserializedHostPoolPropertiesInner.objectId = reader.getString();
} else if ("friendlyName".equals(fieldName)) {
deserializedHostPoolPropertiesInner.friendlyName = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedHostPoolPropertiesInner.description = reader.getString();
} else if ("personalDesktopAssignmentType".equals(fieldName)) {
deserializedHostPoolPropertiesInner.personalDesktopAssignmentType
= PersonalDesktopAssignmentType.fromString(reader.getString());
} else if ("customRdpProperty".equals(fieldName)) {
deserializedHostPoolPropertiesInner.customRdpProperty = reader.getString();
} else if ("maxSessionLimit".equals(fieldName)) {
deserializedHostPoolPropertiesInner.maxSessionLimit = reader.getNullable(JsonReader::getInt);
} else if ("ring".equals(fieldName)) {
deserializedHostPoolPropertiesInner.ring = reader.getNullable(JsonReader::getInt);
} else if ("validationEnvironment".equals(fieldName)) {
deserializedHostPoolPropertiesInner.validationEnvironment
= reader.getNullable(JsonReader::getBoolean);
} else if ("registrationInfo".equals(fieldName)) {
deserializedHostPoolPropertiesInner.registrationInfo = RegistrationInfoInner.fromJson(reader);
} else if ("vmTemplate".equals(fieldName)) {
deserializedHostPoolPropertiesInner.vmTemplate = reader.getString();
} else if ("applicationGroupReferences".equals(fieldName)) {
List applicationGroupReferences = reader.readArray(reader1 -> reader1.getString());
deserializedHostPoolPropertiesInner.applicationGroupReferences = applicationGroupReferences;
} else if ("appAttachPackageReferences".equals(fieldName)) {
List appAttachPackageReferences = reader.readArray(reader1 -> reader1.getString());
deserializedHostPoolPropertiesInner.appAttachPackageReferences = appAttachPackageReferences;
} else if ("ssoadfsAuthority".equals(fieldName)) {
deserializedHostPoolPropertiesInner.ssoadfsAuthority = reader.getString();
} else if ("ssoClientId".equals(fieldName)) {
deserializedHostPoolPropertiesInner.ssoClientId = reader.getString();
} else if ("ssoClientSecretKeyVaultPath".equals(fieldName)) {
deserializedHostPoolPropertiesInner.ssoClientSecretKeyVaultPath = reader.getString();
} else if ("ssoSecretType".equals(fieldName)) {
deserializedHostPoolPropertiesInner.ssoSecretType = SsoSecretType.fromString(reader.getString());
} else if ("startVMOnConnect".equals(fieldName)) {
deserializedHostPoolPropertiesInner.startVMOnConnect = reader.getNullable(JsonReader::getBoolean);
} else if ("cloudPcResource".equals(fieldName)) {
deserializedHostPoolPropertiesInner.cloudPcResource = reader.getNullable(JsonReader::getBoolean);
} else if ("publicNetworkAccess".equals(fieldName)) {
deserializedHostPoolPropertiesInner.publicNetworkAccess
= HostpoolPublicNetworkAccess.fromString(reader.getString());
} else if ("agentUpdate".equals(fieldName)) {
deserializedHostPoolPropertiesInner.agentUpdate = AgentUpdateProperties.fromJson(reader);
} else if ("privateEndpointConnections".equals(fieldName)) {
List privateEndpointConnections
= reader.readArray(reader1 -> PrivateEndpointConnection.fromJson(reader1));
deserializedHostPoolPropertiesInner.privateEndpointConnections = privateEndpointConnections;
} else {
reader.skipChildren();
}
}
return deserializedHostPoolPropertiesInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy