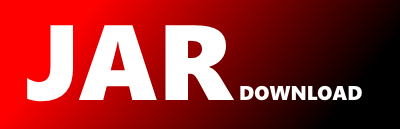
com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPersonalScheduleProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.desktopvirtualization.models.DayOfWeek;
import com.azure.resourcemanager.desktopvirtualization.models.SessionHandlingOperation;
import com.azure.resourcemanager.desktopvirtualization.models.SetStartVMOnConnect;
import com.azure.resourcemanager.desktopvirtualization.models.StartupBehavior;
import com.azure.resourcemanager.desktopvirtualization.models.Time;
import java.io.IOException;
import java.util.List;
/**
* A ScalingPlanPersonalSchedule.
*/
@Fluent
public final class ScalingPlanPersonalScheduleProperties
implements JsonSerializable {
/*
* Set of days of the week on which this schedule is active.
*/
private List daysOfWeek;
/*
* Starting time for ramp up period.
*/
private Time rampUpStartTime;
/*
* The desired startup behavior during the ramp up period for personal vms in the hostpool.
*/
private StartupBehavior rampUpAutoStartHosts;
/*
* The desired configuration of Start VM On Connect for the hostpool during the ramp up phase. If this is disabled,
* session hosts must be turned on using rampUpAutoStartHosts or by turning them on manually.
*/
private SetStartVMOnConnect rampUpStartVMOnConnect;
/*
* Action to be taken after a user disconnect during the ramp up period.
*/
private SessionHandlingOperation rampUpActionOnDisconnect;
/*
* The time in minutes to wait before performing the desired session handling action when a user disconnects during
* the ramp up period.
*/
private Integer rampUpMinutesToWaitOnDisconnect;
/*
* Action to be taken after a logoff during the ramp up period.
*/
private SessionHandlingOperation rampUpActionOnLogoff;
/*
* The time in minutes to wait before performing the desired session handling action when a user logs off during the
* ramp up period.
*/
private Integer rampUpMinutesToWaitOnLogoff;
/*
* Starting time for peak period.
*/
private Time peakStartTime;
/*
* The desired configuration of Start VM On Connect for the hostpool during the peak phase.
*/
private SetStartVMOnConnect peakStartVMOnConnect;
/*
* Action to be taken after a user disconnect during the peak period.
*/
private SessionHandlingOperation peakActionOnDisconnect;
/*
* The time in minutes to wait before performing the desired session handling action when a user disconnects during
* the peak period.
*/
private Integer peakMinutesToWaitOnDisconnect;
/*
* Action to be taken after a logoff during the peak period.
*/
private SessionHandlingOperation peakActionOnLogoff;
/*
* The time in minutes to wait before performing the desired session handling action when a user logs off during the
* peak period.
*/
private Integer peakMinutesToWaitOnLogoff;
/*
* Starting time for ramp down period.
*/
private Time rampDownStartTime;
/*
* The desired configuration of Start VM On Connect for the hostpool during the ramp down phase.
*/
private SetStartVMOnConnect rampDownStartVMOnConnect;
/*
* Action to be taken after a user disconnect during the ramp down period.
*/
private SessionHandlingOperation rampDownActionOnDisconnect;
/*
* The time in minutes to wait before performing the desired session handling action when a user disconnects during
* the ramp down period.
*/
private Integer rampDownMinutesToWaitOnDisconnect;
/*
* Action to be taken after a logoff during the ramp down period.
*/
private SessionHandlingOperation rampDownActionOnLogoff;
/*
* The time in minutes to wait before performing the desired session handling action when a user logs off during the
* ramp down period.
*/
private Integer rampDownMinutesToWaitOnLogoff;
/*
* Starting time for off-peak period.
*/
private Time offPeakStartTime;
/*
* The desired configuration of Start VM On Connect for the hostpool during the off-peak phase.
*/
private SetStartVMOnConnect offPeakStartVMOnConnect;
/*
* Action to be taken after a user disconnect during the off-peak period.
*/
private SessionHandlingOperation offPeakActionOnDisconnect;
/*
* The time in minutes to wait before performing the desired session handling action when a user disconnects during
* the off-peak period.
*/
private Integer offPeakMinutesToWaitOnDisconnect;
/*
* Action to be taken after a logoff during the off-peak period.
*/
private SessionHandlingOperation offPeakActionOnLogoff;
/*
* The time in minutes to wait before performing the desired session handling action when a user logs off during the
* off-peak period.
*/
private Integer offPeakMinutesToWaitOnLogoff;
/**
* Creates an instance of ScalingPlanPersonalScheduleProperties class.
*/
public ScalingPlanPersonalScheduleProperties() {
}
/**
* Get the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @return the daysOfWeek value.
*/
public List daysOfWeek() {
return this.daysOfWeek;
}
/**
* Set the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @param daysOfWeek the daysOfWeek value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withDaysOfWeek(List daysOfWeek) {
this.daysOfWeek = daysOfWeek;
return this;
}
/**
* Get the rampUpStartTime property: Starting time for ramp up period.
*
* @return the rampUpStartTime value.
*/
public Time rampUpStartTime() {
return this.rampUpStartTime;
}
/**
* Set the rampUpStartTime property: Starting time for ramp up period.
*
* @param rampUpStartTime the rampUpStartTime value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withRampUpStartTime(Time rampUpStartTime) {
this.rampUpStartTime = rampUpStartTime;
return this;
}
/**
* Get the rampUpAutoStartHosts property: The desired startup behavior during the ramp up period for personal vms in
* the hostpool.
*
* @return the rampUpAutoStartHosts value.
*/
public StartupBehavior rampUpAutoStartHosts() {
return this.rampUpAutoStartHosts;
}
/**
* Set the rampUpAutoStartHosts property: The desired startup behavior during the ramp up period for personal vms in
* the hostpool.
*
* @param rampUpAutoStartHosts the rampUpAutoStartHosts value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withRampUpAutoStartHosts(StartupBehavior rampUpAutoStartHosts) {
this.rampUpAutoStartHosts = rampUpAutoStartHosts;
return this;
}
/**
* Get the rampUpStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by turning
* them on manually.
*
* @return the rampUpStartVMOnConnect value.
*/
public SetStartVMOnConnect rampUpStartVMOnConnect() {
return this.rampUpStartVMOnConnect;
}
/**
* Set the rampUpStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by turning
* them on manually.
*
* @param rampUpStartVMOnConnect the rampUpStartVMOnConnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampUpStartVMOnConnect(SetStartVMOnConnect rampUpStartVMOnConnect) {
this.rampUpStartVMOnConnect = rampUpStartVMOnConnect;
return this;
}
/**
* Get the rampUpActionOnDisconnect property: Action to be taken after a user disconnect during the ramp up period.
*
* @return the rampUpActionOnDisconnect value.
*/
public SessionHandlingOperation rampUpActionOnDisconnect() {
return this.rampUpActionOnDisconnect;
}
/**
* Set the rampUpActionOnDisconnect property: Action to be taken after a user disconnect during the ramp up period.
*
* @param rampUpActionOnDisconnect the rampUpActionOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampUpActionOnDisconnect(SessionHandlingOperation rampUpActionOnDisconnect) {
this.rampUpActionOnDisconnect = rampUpActionOnDisconnect;
return this;
}
/**
* Get the rampUpMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp up period.
*
* @return the rampUpMinutesToWaitOnDisconnect value.
*/
public Integer rampUpMinutesToWaitOnDisconnect() {
return this.rampUpMinutesToWaitOnDisconnect;
}
/**
* Set the rampUpMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp up period.
*
* @param rampUpMinutesToWaitOnDisconnect the rampUpMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampUpMinutesToWaitOnDisconnect(Integer rampUpMinutesToWaitOnDisconnect) {
this.rampUpMinutesToWaitOnDisconnect = rampUpMinutesToWaitOnDisconnect;
return this;
}
/**
* Get the rampUpActionOnLogoff property: Action to be taken after a logoff during the ramp up period.
*
* @return the rampUpActionOnLogoff value.
*/
public SessionHandlingOperation rampUpActionOnLogoff() {
return this.rampUpActionOnLogoff;
}
/**
* Set the rampUpActionOnLogoff property: Action to be taken after a logoff during the ramp up period.
*
* @param rampUpActionOnLogoff the rampUpActionOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampUpActionOnLogoff(SessionHandlingOperation rampUpActionOnLogoff) {
this.rampUpActionOnLogoff = rampUpActionOnLogoff;
return this;
}
/**
* Get the rampUpMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp up period.
*
* @return the rampUpMinutesToWaitOnLogoff value.
*/
public Integer rampUpMinutesToWaitOnLogoff() {
return this.rampUpMinutesToWaitOnLogoff;
}
/**
* Set the rampUpMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp up period.
*
* @param rampUpMinutesToWaitOnLogoff the rampUpMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withRampUpMinutesToWaitOnLogoff(Integer rampUpMinutesToWaitOnLogoff) {
this.rampUpMinutesToWaitOnLogoff = rampUpMinutesToWaitOnLogoff;
return this;
}
/**
* Get the peakStartTime property: Starting time for peak period.
*
* @return the peakStartTime value.
*/
public Time peakStartTime() {
return this.peakStartTime;
}
/**
* Set the peakStartTime property: Starting time for peak period.
*
* @param peakStartTime the peakStartTime value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withPeakStartTime(Time peakStartTime) {
this.peakStartTime = peakStartTime;
return this;
}
/**
* Get the peakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the peak phase.
*
* @return the peakStartVMOnConnect value.
*/
public SetStartVMOnConnect peakStartVMOnConnect() {
return this.peakStartVMOnConnect;
}
/**
* Set the peakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the peak phase.
*
* @param peakStartVMOnConnect the peakStartVMOnConnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withPeakStartVMOnConnect(SetStartVMOnConnect peakStartVMOnConnect) {
this.peakStartVMOnConnect = peakStartVMOnConnect;
return this;
}
/**
* Get the peakActionOnDisconnect property: Action to be taken after a user disconnect during the peak period.
*
* @return the peakActionOnDisconnect value.
*/
public SessionHandlingOperation peakActionOnDisconnect() {
return this.peakActionOnDisconnect;
}
/**
* Set the peakActionOnDisconnect property: Action to be taken after a user disconnect during the peak period.
*
* @param peakActionOnDisconnect the peakActionOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withPeakActionOnDisconnect(SessionHandlingOperation peakActionOnDisconnect) {
this.peakActionOnDisconnect = peakActionOnDisconnect;
return this;
}
/**
* Get the peakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the peak period.
*
* @return the peakMinutesToWaitOnDisconnect value.
*/
public Integer peakMinutesToWaitOnDisconnect() {
return this.peakMinutesToWaitOnDisconnect;
}
/**
* Set the peakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the peak period.
*
* @param peakMinutesToWaitOnDisconnect the peakMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withPeakMinutesToWaitOnDisconnect(Integer peakMinutesToWaitOnDisconnect) {
this.peakMinutesToWaitOnDisconnect = peakMinutesToWaitOnDisconnect;
return this;
}
/**
* Get the peakActionOnLogoff property: Action to be taken after a logoff during the peak period.
*
* @return the peakActionOnLogoff value.
*/
public SessionHandlingOperation peakActionOnLogoff() {
return this.peakActionOnLogoff;
}
/**
* Set the peakActionOnLogoff property: Action to be taken after a logoff during the peak period.
*
* @param peakActionOnLogoff the peakActionOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withPeakActionOnLogoff(SessionHandlingOperation peakActionOnLogoff) {
this.peakActionOnLogoff = peakActionOnLogoff;
return this;
}
/**
* Get the peakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the peak period.
*
* @return the peakMinutesToWaitOnLogoff value.
*/
public Integer peakMinutesToWaitOnLogoff() {
return this.peakMinutesToWaitOnLogoff;
}
/**
* Set the peakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the peak period.
*
* @param peakMinutesToWaitOnLogoff the peakMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withPeakMinutesToWaitOnLogoff(Integer peakMinutesToWaitOnLogoff) {
this.peakMinutesToWaitOnLogoff = peakMinutesToWaitOnLogoff;
return this;
}
/**
* Get the rampDownStartTime property: Starting time for ramp down period.
*
* @return the rampDownStartTime value.
*/
public Time rampDownStartTime() {
return this.rampDownStartTime;
}
/**
* Set the rampDownStartTime property: Starting time for ramp down period.
*
* @param rampDownStartTime the rampDownStartTime value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withRampDownStartTime(Time rampDownStartTime) {
this.rampDownStartTime = rampDownStartTime;
return this;
}
/**
* Get the rampDownStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the ramp down phase.
*
* @return the rampDownStartVMOnConnect value.
*/
public SetStartVMOnConnect rampDownStartVMOnConnect() {
return this.rampDownStartVMOnConnect;
}
/**
* Set the rampDownStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the ramp down phase.
*
* @param rampDownStartVMOnConnect the rampDownStartVMOnConnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampDownStartVMOnConnect(SetStartVMOnConnect rampDownStartVMOnConnect) {
this.rampDownStartVMOnConnect = rampDownStartVMOnConnect;
return this;
}
/**
* Get the rampDownActionOnDisconnect property: Action to be taken after a user disconnect during the ramp down
* period.
*
* @return the rampDownActionOnDisconnect value.
*/
public SessionHandlingOperation rampDownActionOnDisconnect() {
return this.rampDownActionOnDisconnect;
}
/**
* Set the rampDownActionOnDisconnect property: Action to be taken after a user disconnect during the ramp down
* period.
*
* @param rampDownActionOnDisconnect the rampDownActionOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampDownActionOnDisconnect(SessionHandlingOperation rampDownActionOnDisconnect) {
this.rampDownActionOnDisconnect = rampDownActionOnDisconnect;
return this;
}
/**
* Get the rampDownMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp down period.
*
* @return the rampDownMinutesToWaitOnDisconnect value.
*/
public Integer rampDownMinutesToWaitOnDisconnect() {
return this.rampDownMinutesToWaitOnDisconnect;
}
/**
* Set the rampDownMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp down period.
*
* @param rampDownMinutesToWaitOnDisconnect the rampDownMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampDownMinutesToWaitOnDisconnect(Integer rampDownMinutesToWaitOnDisconnect) {
this.rampDownMinutesToWaitOnDisconnect = rampDownMinutesToWaitOnDisconnect;
return this;
}
/**
* Get the rampDownActionOnLogoff property: Action to be taken after a logoff during the ramp down period.
*
* @return the rampDownActionOnLogoff value.
*/
public SessionHandlingOperation rampDownActionOnLogoff() {
return this.rampDownActionOnLogoff;
}
/**
* Set the rampDownActionOnLogoff property: Action to be taken after a logoff during the ramp down period.
*
* @param rampDownActionOnLogoff the rampDownActionOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampDownActionOnLogoff(SessionHandlingOperation rampDownActionOnLogoff) {
this.rampDownActionOnLogoff = rampDownActionOnLogoff;
return this;
}
/**
* Get the rampDownMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp down period.
*
* @return the rampDownMinutesToWaitOnLogoff value.
*/
public Integer rampDownMinutesToWaitOnLogoff() {
return this.rampDownMinutesToWaitOnLogoff;
}
/**
* Set the rampDownMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp down period.
*
* @param rampDownMinutesToWaitOnLogoff the rampDownMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withRampDownMinutesToWaitOnLogoff(Integer rampDownMinutesToWaitOnLogoff) {
this.rampDownMinutesToWaitOnLogoff = rampDownMinutesToWaitOnLogoff;
return this;
}
/**
* Get the offPeakStartTime property: Starting time for off-peak period.
*
* @return the offPeakStartTime value.
*/
public Time offPeakStartTime() {
return this.offPeakStartTime;
}
/**
* Set the offPeakStartTime property: Starting time for off-peak period.
*
* @param offPeakStartTime the offPeakStartTime value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties withOffPeakStartTime(Time offPeakStartTime) {
this.offPeakStartTime = offPeakStartTime;
return this;
}
/**
* Get the offPeakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the off-peak phase.
*
* @return the offPeakStartVMOnConnect value.
*/
public SetStartVMOnConnect offPeakStartVMOnConnect() {
return this.offPeakStartVMOnConnect;
}
/**
* Set the offPeakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the off-peak phase.
*
* @param offPeakStartVMOnConnect the offPeakStartVMOnConnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withOffPeakStartVMOnConnect(SetStartVMOnConnect offPeakStartVMOnConnect) {
this.offPeakStartVMOnConnect = offPeakStartVMOnConnect;
return this;
}
/**
* Get the offPeakActionOnDisconnect property: Action to be taken after a user disconnect during the off-peak
* period.
*
* @return the offPeakActionOnDisconnect value.
*/
public SessionHandlingOperation offPeakActionOnDisconnect() {
return this.offPeakActionOnDisconnect;
}
/**
* Set the offPeakActionOnDisconnect property: Action to be taken after a user disconnect during the off-peak
* period.
*
* @param offPeakActionOnDisconnect the offPeakActionOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withOffPeakActionOnDisconnect(SessionHandlingOperation offPeakActionOnDisconnect) {
this.offPeakActionOnDisconnect = offPeakActionOnDisconnect;
return this;
}
/**
* Get the offPeakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the off-peak period.
*
* @return the offPeakMinutesToWaitOnDisconnect value.
*/
public Integer offPeakMinutesToWaitOnDisconnect() {
return this.offPeakMinutesToWaitOnDisconnect;
}
/**
* Set the offPeakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the off-peak period.
*
* @param offPeakMinutesToWaitOnDisconnect the offPeakMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withOffPeakMinutesToWaitOnDisconnect(Integer offPeakMinutesToWaitOnDisconnect) {
this.offPeakMinutesToWaitOnDisconnect = offPeakMinutesToWaitOnDisconnect;
return this;
}
/**
* Get the offPeakActionOnLogoff property: Action to be taken after a logoff during the off-peak period.
*
* @return the offPeakActionOnLogoff value.
*/
public SessionHandlingOperation offPeakActionOnLogoff() {
return this.offPeakActionOnLogoff;
}
/**
* Set the offPeakActionOnLogoff property: Action to be taken after a logoff during the off-peak period.
*
* @param offPeakActionOnLogoff the offPeakActionOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withOffPeakActionOnLogoff(SessionHandlingOperation offPeakActionOnLogoff) {
this.offPeakActionOnLogoff = offPeakActionOnLogoff;
return this;
}
/**
* Get the offPeakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the off-peak period.
*
* @return the offPeakMinutesToWaitOnLogoff value.
*/
public Integer offPeakMinutesToWaitOnLogoff() {
return this.offPeakMinutesToWaitOnLogoff;
}
/**
* Set the offPeakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the off-peak period.
*
* @param offPeakMinutesToWaitOnLogoff the offPeakMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalScheduleProperties object itself.
*/
public ScalingPlanPersonalScheduleProperties
withOffPeakMinutesToWaitOnLogoff(Integer offPeakMinutesToWaitOnLogoff) {
this.offPeakMinutesToWaitOnLogoff = offPeakMinutesToWaitOnLogoff;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (rampUpStartTime() != null) {
rampUpStartTime().validate();
}
if (peakStartTime() != null) {
peakStartTime().validate();
}
if (rampDownStartTime() != null) {
rampDownStartTime().validate();
}
if (offPeakStartTime() != null) {
offPeakStartTime().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("daysOfWeek", this.daysOfWeek,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeJsonField("rampUpStartTime", this.rampUpStartTime);
jsonWriter.writeStringField("rampUpAutoStartHosts",
this.rampUpAutoStartHosts == null ? null : this.rampUpAutoStartHosts.toString());
jsonWriter.writeStringField("rampUpStartVMOnConnect",
this.rampUpStartVMOnConnect == null ? null : this.rampUpStartVMOnConnect.toString());
jsonWriter.writeStringField("rampUpActionOnDisconnect",
this.rampUpActionOnDisconnect == null ? null : this.rampUpActionOnDisconnect.toString());
jsonWriter.writeNumberField("rampUpMinutesToWaitOnDisconnect", this.rampUpMinutesToWaitOnDisconnect);
jsonWriter.writeStringField("rampUpActionOnLogoff",
this.rampUpActionOnLogoff == null ? null : this.rampUpActionOnLogoff.toString());
jsonWriter.writeNumberField("rampUpMinutesToWaitOnLogoff", this.rampUpMinutesToWaitOnLogoff);
jsonWriter.writeJsonField("peakStartTime", this.peakStartTime);
jsonWriter.writeStringField("peakStartVMOnConnect",
this.peakStartVMOnConnect == null ? null : this.peakStartVMOnConnect.toString());
jsonWriter.writeStringField("peakActionOnDisconnect",
this.peakActionOnDisconnect == null ? null : this.peakActionOnDisconnect.toString());
jsonWriter.writeNumberField("peakMinutesToWaitOnDisconnect", this.peakMinutesToWaitOnDisconnect);
jsonWriter.writeStringField("peakActionOnLogoff",
this.peakActionOnLogoff == null ? null : this.peakActionOnLogoff.toString());
jsonWriter.writeNumberField("peakMinutesToWaitOnLogoff", this.peakMinutesToWaitOnLogoff);
jsonWriter.writeJsonField("rampDownStartTime", this.rampDownStartTime);
jsonWriter.writeStringField("rampDownStartVMOnConnect",
this.rampDownStartVMOnConnect == null ? null : this.rampDownStartVMOnConnect.toString());
jsonWriter.writeStringField("rampDownActionOnDisconnect",
this.rampDownActionOnDisconnect == null ? null : this.rampDownActionOnDisconnect.toString());
jsonWriter.writeNumberField("rampDownMinutesToWaitOnDisconnect", this.rampDownMinutesToWaitOnDisconnect);
jsonWriter.writeStringField("rampDownActionOnLogoff",
this.rampDownActionOnLogoff == null ? null : this.rampDownActionOnLogoff.toString());
jsonWriter.writeNumberField("rampDownMinutesToWaitOnLogoff", this.rampDownMinutesToWaitOnLogoff);
jsonWriter.writeJsonField("offPeakStartTime", this.offPeakStartTime);
jsonWriter.writeStringField("offPeakStartVMOnConnect",
this.offPeakStartVMOnConnect == null ? null : this.offPeakStartVMOnConnect.toString());
jsonWriter.writeStringField("offPeakActionOnDisconnect",
this.offPeakActionOnDisconnect == null ? null : this.offPeakActionOnDisconnect.toString());
jsonWriter.writeNumberField("offPeakMinutesToWaitOnDisconnect", this.offPeakMinutesToWaitOnDisconnect);
jsonWriter.writeStringField("offPeakActionOnLogoff",
this.offPeakActionOnLogoff == null ? null : this.offPeakActionOnLogoff.toString());
jsonWriter.writeNumberField("offPeakMinutesToWaitOnLogoff", this.offPeakMinutesToWaitOnLogoff);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScalingPlanPersonalScheduleProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScalingPlanPersonalScheduleProperties if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ScalingPlanPersonalScheduleProperties.
*/
public static ScalingPlanPersonalScheduleProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScalingPlanPersonalScheduleProperties deserializedScalingPlanPersonalScheduleProperties
= new ScalingPlanPersonalScheduleProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("daysOfWeek".equals(fieldName)) {
List daysOfWeek = reader.readArray(reader1 -> DayOfWeek.fromString(reader1.getString()));
deserializedScalingPlanPersonalScheduleProperties.daysOfWeek = daysOfWeek;
} else if ("rampUpStartTime".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampUpStartTime = Time.fromJson(reader);
} else if ("rampUpAutoStartHosts".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampUpAutoStartHosts
= StartupBehavior.fromString(reader.getString());
} else if ("rampUpStartVMOnConnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampUpStartVMOnConnect
= SetStartVMOnConnect.fromString(reader.getString());
} else if ("rampUpActionOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampUpActionOnDisconnect
= SessionHandlingOperation.fromString(reader.getString());
} else if ("rampUpMinutesToWaitOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampUpMinutesToWaitOnDisconnect
= reader.getNullable(JsonReader::getInt);
} else if ("rampUpActionOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampUpActionOnLogoff
= SessionHandlingOperation.fromString(reader.getString());
} else if ("rampUpMinutesToWaitOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampUpMinutesToWaitOnLogoff
= reader.getNullable(JsonReader::getInt);
} else if ("peakStartTime".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.peakStartTime = Time.fromJson(reader);
} else if ("peakStartVMOnConnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.peakStartVMOnConnect
= SetStartVMOnConnect.fromString(reader.getString());
} else if ("peakActionOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.peakActionOnDisconnect
= SessionHandlingOperation.fromString(reader.getString());
} else if ("peakMinutesToWaitOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.peakMinutesToWaitOnDisconnect
= reader.getNullable(JsonReader::getInt);
} else if ("peakActionOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.peakActionOnLogoff
= SessionHandlingOperation.fromString(reader.getString());
} else if ("peakMinutesToWaitOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.peakMinutesToWaitOnLogoff
= reader.getNullable(JsonReader::getInt);
} else if ("rampDownStartTime".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampDownStartTime = Time.fromJson(reader);
} else if ("rampDownStartVMOnConnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampDownStartVMOnConnect
= SetStartVMOnConnect.fromString(reader.getString());
} else if ("rampDownActionOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampDownActionOnDisconnect
= SessionHandlingOperation.fromString(reader.getString());
} else if ("rampDownMinutesToWaitOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampDownMinutesToWaitOnDisconnect
= reader.getNullable(JsonReader::getInt);
} else if ("rampDownActionOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampDownActionOnLogoff
= SessionHandlingOperation.fromString(reader.getString());
} else if ("rampDownMinutesToWaitOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.rampDownMinutesToWaitOnLogoff
= reader.getNullable(JsonReader::getInt);
} else if ("offPeakStartTime".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.offPeakStartTime = Time.fromJson(reader);
} else if ("offPeakStartVMOnConnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.offPeakStartVMOnConnect
= SetStartVMOnConnect.fromString(reader.getString());
} else if ("offPeakActionOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.offPeakActionOnDisconnect
= SessionHandlingOperation.fromString(reader.getString());
} else if ("offPeakMinutesToWaitOnDisconnect".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.offPeakMinutesToWaitOnDisconnect
= reader.getNullable(JsonReader::getInt);
} else if ("offPeakActionOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.offPeakActionOnLogoff
= SessionHandlingOperation.fromString(reader.getString());
} else if ("offPeakMinutesToWaitOnLogoff".equals(fieldName)) {
deserializedScalingPlanPersonalScheduleProperties.offPeakMinutesToWaitOnLogoff
= reader.getNullable(JsonReader::getInt);
} else {
reader.skipChildren();
}
}
return deserializedScalingPlanPersonalScheduleProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy