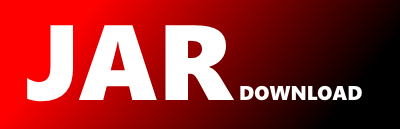
com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPooledScheduleProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.desktopvirtualization.models.DayOfWeek;
import com.azure.resourcemanager.desktopvirtualization.models.SessionHostLoadBalancingAlgorithm;
import com.azure.resourcemanager.desktopvirtualization.models.StopHostsWhen;
import com.azure.resourcemanager.desktopvirtualization.models.Time;
import java.io.IOException;
import java.util.List;
/**
* A ScalingPlanPooledSchedule.
*/
@Fluent
public final class ScalingPlanPooledScheduleProperties
implements JsonSerializable {
/*
* Set of days of the week on which this schedule is active.
*/
private List daysOfWeek;
/*
* Starting time for ramp up period.
*/
private Time rampUpStartTime;
/*
* Load balancing algorithm for ramp up period.
*/
private SessionHostLoadBalancingAlgorithm rampUpLoadBalancingAlgorithm;
/*
* Minimum host percentage for ramp up period.
*/
private Integer rampUpMinimumHostsPct;
/*
* Capacity threshold for ramp up period.
*/
private Integer rampUpCapacityThresholdPct;
/*
* Starting time for peak period.
*/
private Time peakStartTime;
/*
* Load balancing algorithm for peak period.
*/
private SessionHostLoadBalancingAlgorithm peakLoadBalancingAlgorithm;
/*
* Starting time for ramp down period.
*/
private Time rampDownStartTime;
/*
* Load balancing algorithm for ramp down period.
*/
private SessionHostLoadBalancingAlgorithm rampDownLoadBalancingAlgorithm;
/*
* Minimum host percentage for ramp down period.
*/
private Integer rampDownMinimumHostsPct;
/*
* Capacity threshold for ramp down period.
*/
private Integer rampDownCapacityThresholdPct;
/*
* Should users be logged off forcefully from hosts.
*/
private Boolean rampDownForceLogoffUsers;
/*
* Specifies when to stop hosts during ramp down period.
*/
private StopHostsWhen rampDownStopHostsWhen;
/*
* Number of minutes to wait to stop hosts during ramp down period.
*/
private Integer rampDownWaitTimeMinutes;
/*
* Notification message for users during ramp down period.
*/
private String rampDownNotificationMessage;
/*
* Starting time for off-peak period.
*/
private Time offPeakStartTime;
/*
* Load balancing algorithm for off-peak period.
*/
private SessionHostLoadBalancingAlgorithm offPeakLoadBalancingAlgorithm;
/**
* Creates an instance of ScalingPlanPooledScheduleProperties class.
*/
public ScalingPlanPooledScheduleProperties() {
}
/**
* Get the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @return the daysOfWeek value.
*/
public List daysOfWeek() {
return this.daysOfWeek;
}
/**
* Set the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @param daysOfWeek the daysOfWeek value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withDaysOfWeek(List daysOfWeek) {
this.daysOfWeek = daysOfWeek;
return this;
}
/**
* Get the rampUpStartTime property: Starting time for ramp up period.
*
* @return the rampUpStartTime value.
*/
public Time rampUpStartTime() {
return this.rampUpStartTime;
}
/**
* Set the rampUpStartTime property: Starting time for ramp up period.
*
* @param rampUpStartTime the rampUpStartTime value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampUpStartTime(Time rampUpStartTime) {
this.rampUpStartTime = rampUpStartTime;
return this;
}
/**
* Get the rampUpLoadBalancingAlgorithm property: Load balancing algorithm for ramp up period.
*
* @return the rampUpLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm rampUpLoadBalancingAlgorithm() {
return this.rampUpLoadBalancingAlgorithm;
}
/**
* Set the rampUpLoadBalancingAlgorithm property: Load balancing algorithm for ramp up period.
*
* @param rampUpLoadBalancingAlgorithm the rampUpLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties
withRampUpLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm rampUpLoadBalancingAlgorithm) {
this.rampUpLoadBalancingAlgorithm = rampUpLoadBalancingAlgorithm;
return this;
}
/**
* Get the rampUpMinimumHostsPct property: Minimum host percentage for ramp up period.
*
* @return the rampUpMinimumHostsPct value.
*/
public Integer rampUpMinimumHostsPct() {
return this.rampUpMinimumHostsPct;
}
/**
* Set the rampUpMinimumHostsPct property: Minimum host percentage for ramp up period.
*
* @param rampUpMinimumHostsPct the rampUpMinimumHostsPct value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampUpMinimumHostsPct(Integer rampUpMinimumHostsPct) {
this.rampUpMinimumHostsPct = rampUpMinimumHostsPct;
return this;
}
/**
* Get the rampUpCapacityThresholdPct property: Capacity threshold for ramp up period.
*
* @return the rampUpCapacityThresholdPct value.
*/
public Integer rampUpCapacityThresholdPct() {
return this.rampUpCapacityThresholdPct;
}
/**
* Set the rampUpCapacityThresholdPct property: Capacity threshold for ramp up period.
*
* @param rampUpCapacityThresholdPct the rampUpCapacityThresholdPct value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampUpCapacityThresholdPct(Integer rampUpCapacityThresholdPct) {
this.rampUpCapacityThresholdPct = rampUpCapacityThresholdPct;
return this;
}
/**
* Get the peakStartTime property: Starting time for peak period.
*
* @return the peakStartTime value.
*/
public Time peakStartTime() {
return this.peakStartTime;
}
/**
* Set the peakStartTime property: Starting time for peak period.
*
* @param peakStartTime the peakStartTime value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withPeakStartTime(Time peakStartTime) {
this.peakStartTime = peakStartTime;
return this;
}
/**
* Get the peakLoadBalancingAlgorithm property: Load balancing algorithm for peak period.
*
* @return the peakLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm peakLoadBalancingAlgorithm() {
return this.peakLoadBalancingAlgorithm;
}
/**
* Set the peakLoadBalancingAlgorithm property: Load balancing algorithm for peak period.
*
* @param peakLoadBalancingAlgorithm the peakLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties
withPeakLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm peakLoadBalancingAlgorithm) {
this.peakLoadBalancingAlgorithm = peakLoadBalancingAlgorithm;
return this;
}
/**
* Get the rampDownStartTime property: Starting time for ramp down period.
*
* @return the rampDownStartTime value.
*/
public Time rampDownStartTime() {
return this.rampDownStartTime;
}
/**
* Set the rampDownStartTime property: Starting time for ramp down period.
*
* @param rampDownStartTime the rampDownStartTime value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampDownStartTime(Time rampDownStartTime) {
this.rampDownStartTime = rampDownStartTime;
return this;
}
/**
* Get the rampDownLoadBalancingAlgorithm property: Load balancing algorithm for ramp down period.
*
* @return the rampDownLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm rampDownLoadBalancingAlgorithm() {
return this.rampDownLoadBalancingAlgorithm;
}
/**
* Set the rampDownLoadBalancingAlgorithm property: Load balancing algorithm for ramp down period.
*
* @param rampDownLoadBalancingAlgorithm the rampDownLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties
withRampDownLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm rampDownLoadBalancingAlgorithm) {
this.rampDownLoadBalancingAlgorithm = rampDownLoadBalancingAlgorithm;
return this;
}
/**
* Get the rampDownMinimumHostsPct property: Minimum host percentage for ramp down period.
*
* @return the rampDownMinimumHostsPct value.
*/
public Integer rampDownMinimumHostsPct() {
return this.rampDownMinimumHostsPct;
}
/**
* Set the rampDownMinimumHostsPct property: Minimum host percentage for ramp down period.
*
* @param rampDownMinimumHostsPct the rampDownMinimumHostsPct value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampDownMinimumHostsPct(Integer rampDownMinimumHostsPct) {
this.rampDownMinimumHostsPct = rampDownMinimumHostsPct;
return this;
}
/**
* Get the rampDownCapacityThresholdPct property: Capacity threshold for ramp down period.
*
* @return the rampDownCapacityThresholdPct value.
*/
public Integer rampDownCapacityThresholdPct() {
return this.rampDownCapacityThresholdPct;
}
/**
* Set the rampDownCapacityThresholdPct property: Capacity threshold for ramp down period.
*
* @param rampDownCapacityThresholdPct the rampDownCapacityThresholdPct value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampDownCapacityThresholdPct(Integer rampDownCapacityThresholdPct) {
this.rampDownCapacityThresholdPct = rampDownCapacityThresholdPct;
return this;
}
/**
* Get the rampDownForceLogoffUsers property: Should users be logged off forcefully from hosts.
*
* @return the rampDownForceLogoffUsers value.
*/
public Boolean rampDownForceLogoffUsers() {
return this.rampDownForceLogoffUsers;
}
/**
* Set the rampDownForceLogoffUsers property: Should users be logged off forcefully from hosts.
*
* @param rampDownForceLogoffUsers the rampDownForceLogoffUsers value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampDownForceLogoffUsers(Boolean rampDownForceLogoffUsers) {
this.rampDownForceLogoffUsers = rampDownForceLogoffUsers;
return this;
}
/**
* Get the rampDownStopHostsWhen property: Specifies when to stop hosts during ramp down period.
*
* @return the rampDownStopHostsWhen value.
*/
public StopHostsWhen rampDownStopHostsWhen() {
return this.rampDownStopHostsWhen;
}
/**
* Set the rampDownStopHostsWhen property: Specifies when to stop hosts during ramp down period.
*
* @param rampDownStopHostsWhen the rampDownStopHostsWhen value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampDownStopHostsWhen(StopHostsWhen rampDownStopHostsWhen) {
this.rampDownStopHostsWhen = rampDownStopHostsWhen;
return this;
}
/**
* Get the rampDownWaitTimeMinutes property: Number of minutes to wait to stop hosts during ramp down period.
*
* @return the rampDownWaitTimeMinutes value.
*/
public Integer rampDownWaitTimeMinutes() {
return this.rampDownWaitTimeMinutes;
}
/**
* Set the rampDownWaitTimeMinutes property: Number of minutes to wait to stop hosts during ramp down period.
*
* @param rampDownWaitTimeMinutes the rampDownWaitTimeMinutes value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampDownWaitTimeMinutes(Integer rampDownWaitTimeMinutes) {
this.rampDownWaitTimeMinutes = rampDownWaitTimeMinutes;
return this;
}
/**
* Get the rampDownNotificationMessage property: Notification message for users during ramp down period.
*
* @return the rampDownNotificationMessage value.
*/
public String rampDownNotificationMessage() {
return this.rampDownNotificationMessage;
}
/**
* Set the rampDownNotificationMessage property: Notification message for users during ramp down period.
*
* @param rampDownNotificationMessage the rampDownNotificationMessage value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withRampDownNotificationMessage(String rampDownNotificationMessage) {
this.rampDownNotificationMessage = rampDownNotificationMessage;
return this;
}
/**
* Get the offPeakStartTime property: Starting time for off-peak period.
*
* @return the offPeakStartTime value.
*/
public Time offPeakStartTime() {
return this.offPeakStartTime;
}
/**
* Set the offPeakStartTime property: Starting time for off-peak period.
*
* @param offPeakStartTime the offPeakStartTime value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties withOffPeakStartTime(Time offPeakStartTime) {
this.offPeakStartTime = offPeakStartTime;
return this;
}
/**
* Get the offPeakLoadBalancingAlgorithm property: Load balancing algorithm for off-peak period.
*
* @return the offPeakLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm offPeakLoadBalancingAlgorithm() {
return this.offPeakLoadBalancingAlgorithm;
}
/**
* Set the offPeakLoadBalancingAlgorithm property: Load balancing algorithm for off-peak period.
*
* @param offPeakLoadBalancingAlgorithm the offPeakLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledScheduleProperties object itself.
*/
public ScalingPlanPooledScheduleProperties
withOffPeakLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm offPeakLoadBalancingAlgorithm) {
this.offPeakLoadBalancingAlgorithm = offPeakLoadBalancingAlgorithm;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (rampUpStartTime() != null) {
rampUpStartTime().validate();
}
if (peakStartTime() != null) {
peakStartTime().validate();
}
if (rampDownStartTime() != null) {
rampDownStartTime().validate();
}
if (offPeakStartTime() != null) {
offPeakStartTime().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("daysOfWeek", this.daysOfWeek,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeJsonField("rampUpStartTime", this.rampUpStartTime);
jsonWriter.writeStringField("rampUpLoadBalancingAlgorithm",
this.rampUpLoadBalancingAlgorithm == null ? null : this.rampUpLoadBalancingAlgorithm.toString());
jsonWriter.writeNumberField("rampUpMinimumHostsPct", this.rampUpMinimumHostsPct);
jsonWriter.writeNumberField("rampUpCapacityThresholdPct", this.rampUpCapacityThresholdPct);
jsonWriter.writeJsonField("peakStartTime", this.peakStartTime);
jsonWriter.writeStringField("peakLoadBalancingAlgorithm",
this.peakLoadBalancingAlgorithm == null ? null : this.peakLoadBalancingAlgorithm.toString());
jsonWriter.writeJsonField("rampDownStartTime", this.rampDownStartTime);
jsonWriter.writeStringField("rampDownLoadBalancingAlgorithm",
this.rampDownLoadBalancingAlgorithm == null ? null : this.rampDownLoadBalancingAlgorithm.toString());
jsonWriter.writeNumberField("rampDownMinimumHostsPct", this.rampDownMinimumHostsPct);
jsonWriter.writeNumberField("rampDownCapacityThresholdPct", this.rampDownCapacityThresholdPct);
jsonWriter.writeBooleanField("rampDownForceLogoffUsers", this.rampDownForceLogoffUsers);
jsonWriter.writeStringField("rampDownStopHostsWhen",
this.rampDownStopHostsWhen == null ? null : this.rampDownStopHostsWhen.toString());
jsonWriter.writeNumberField("rampDownWaitTimeMinutes", this.rampDownWaitTimeMinutes);
jsonWriter.writeStringField("rampDownNotificationMessage", this.rampDownNotificationMessage);
jsonWriter.writeJsonField("offPeakStartTime", this.offPeakStartTime);
jsonWriter.writeStringField("offPeakLoadBalancingAlgorithm",
this.offPeakLoadBalancingAlgorithm == null ? null : this.offPeakLoadBalancingAlgorithm.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScalingPlanPooledScheduleProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScalingPlanPooledScheduleProperties if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ScalingPlanPooledScheduleProperties.
*/
public static ScalingPlanPooledScheduleProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScalingPlanPooledScheduleProperties deserializedScalingPlanPooledScheduleProperties
= new ScalingPlanPooledScheduleProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("daysOfWeek".equals(fieldName)) {
List daysOfWeek = reader.readArray(reader1 -> DayOfWeek.fromString(reader1.getString()));
deserializedScalingPlanPooledScheduleProperties.daysOfWeek = daysOfWeek;
} else if ("rampUpStartTime".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampUpStartTime = Time.fromJson(reader);
} else if ("rampUpLoadBalancingAlgorithm".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampUpLoadBalancingAlgorithm
= SessionHostLoadBalancingAlgorithm.fromString(reader.getString());
} else if ("rampUpMinimumHostsPct".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampUpMinimumHostsPct
= reader.getNullable(JsonReader::getInt);
} else if ("rampUpCapacityThresholdPct".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampUpCapacityThresholdPct
= reader.getNullable(JsonReader::getInt);
} else if ("peakStartTime".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.peakStartTime = Time.fromJson(reader);
} else if ("peakLoadBalancingAlgorithm".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.peakLoadBalancingAlgorithm
= SessionHostLoadBalancingAlgorithm.fromString(reader.getString());
} else if ("rampDownStartTime".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownStartTime = Time.fromJson(reader);
} else if ("rampDownLoadBalancingAlgorithm".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownLoadBalancingAlgorithm
= SessionHostLoadBalancingAlgorithm.fromString(reader.getString());
} else if ("rampDownMinimumHostsPct".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownMinimumHostsPct
= reader.getNullable(JsonReader::getInt);
} else if ("rampDownCapacityThresholdPct".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownCapacityThresholdPct
= reader.getNullable(JsonReader::getInt);
} else if ("rampDownForceLogoffUsers".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownForceLogoffUsers
= reader.getNullable(JsonReader::getBoolean);
} else if ("rampDownStopHostsWhen".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownStopHostsWhen
= StopHostsWhen.fromString(reader.getString());
} else if ("rampDownWaitTimeMinutes".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownWaitTimeMinutes
= reader.getNullable(JsonReader::getInt);
} else if ("rampDownNotificationMessage".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.rampDownNotificationMessage = reader.getString();
} else if ("offPeakStartTime".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.offPeakStartTime = Time.fromJson(reader);
} else if ("offPeakLoadBalancingAlgorithm".equals(fieldName)) {
deserializedScalingPlanPooledScheduleProperties.offPeakLoadBalancingAlgorithm
= SessionHostLoadBalancingAlgorithm.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedScalingPlanPooledScheduleProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy