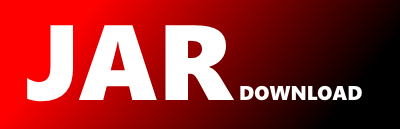
com.azure.resourcemanager.desktopvirtualization.implementation.ScalingPlanPersonalScheduleImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.implementation;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPersonalScheduleInner;
import com.azure.resourcemanager.desktopvirtualization.models.DayOfWeek;
import com.azure.resourcemanager.desktopvirtualization.models.ScalingPlanPersonalSchedule;
import com.azure.resourcemanager.desktopvirtualization.models.ScalingPlanPersonalSchedulePatch;
import com.azure.resourcemanager.desktopvirtualization.models.SessionHandlingOperation;
import com.azure.resourcemanager.desktopvirtualization.models.SetStartVMOnConnect;
import com.azure.resourcemanager.desktopvirtualization.models.StartupBehavior;
import com.azure.resourcemanager.desktopvirtualization.models.Time;
import java.util.Collections;
import java.util.List;
public final class ScalingPlanPersonalScheduleImpl
implements ScalingPlanPersonalSchedule, ScalingPlanPersonalSchedule.Definition, ScalingPlanPersonalSchedule.Update {
private ScalingPlanPersonalScheduleInner innerObject;
private final com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager serviceManager;
public String id() {
return this.innerModel().id();
}
public String name() {
return this.innerModel().name();
}
public String type() {
return this.innerModel().type();
}
public SystemData systemData() {
return this.innerModel().systemData();
}
public List daysOfWeek() {
List inner = this.innerModel().daysOfWeek();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public Time rampUpStartTime() {
return this.innerModel().rampUpStartTime();
}
public StartupBehavior rampUpAutoStartHosts() {
return this.innerModel().rampUpAutoStartHosts();
}
public SetStartVMOnConnect rampUpStartVMOnConnect() {
return this.innerModel().rampUpStartVMOnConnect();
}
public SessionHandlingOperation rampUpActionOnDisconnect() {
return this.innerModel().rampUpActionOnDisconnect();
}
public Integer rampUpMinutesToWaitOnDisconnect() {
return this.innerModel().rampUpMinutesToWaitOnDisconnect();
}
public SessionHandlingOperation rampUpActionOnLogoff() {
return this.innerModel().rampUpActionOnLogoff();
}
public Integer rampUpMinutesToWaitOnLogoff() {
return this.innerModel().rampUpMinutesToWaitOnLogoff();
}
public Time peakStartTime() {
return this.innerModel().peakStartTime();
}
public SetStartVMOnConnect peakStartVMOnConnect() {
return this.innerModel().peakStartVMOnConnect();
}
public SessionHandlingOperation peakActionOnDisconnect() {
return this.innerModel().peakActionOnDisconnect();
}
public Integer peakMinutesToWaitOnDisconnect() {
return this.innerModel().peakMinutesToWaitOnDisconnect();
}
public SessionHandlingOperation peakActionOnLogoff() {
return this.innerModel().peakActionOnLogoff();
}
public Integer peakMinutesToWaitOnLogoff() {
return this.innerModel().peakMinutesToWaitOnLogoff();
}
public Time rampDownStartTime() {
return this.innerModel().rampDownStartTime();
}
public SetStartVMOnConnect rampDownStartVMOnConnect() {
return this.innerModel().rampDownStartVMOnConnect();
}
public SessionHandlingOperation rampDownActionOnDisconnect() {
return this.innerModel().rampDownActionOnDisconnect();
}
public Integer rampDownMinutesToWaitOnDisconnect() {
return this.innerModel().rampDownMinutesToWaitOnDisconnect();
}
public SessionHandlingOperation rampDownActionOnLogoff() {
return this.innerModel().rampDownActionOnLogoff();
}
public Integer rampDownMinutesToWaitOnLogoff() {
return this.innerModel().rampDownMinutesToWaitOnLogoff();
}
public Time offPeakStartTime() {
return this.innerModel().offPeakStartTime();
}
public SetStartVMOnConnect offPeakStartVMOnConnect() {
return this.innerModel().offPeakStartVMOnConnect();
}
public SessionHandlingOperation offPeakActionOnDisconnect() {
return this.innerModel().offPeakActionOnDisconnect();
}
public Integer offPeakMinutesToWaitOnDisconnect() {
return this.innerModel().offPeakMinutesToWaitOnDisconnect();
}
public SessionHandlingOperation offPeakActionOnLogoff() {
return this.innerModel().offPeakActionOnLogoff();
}
public Integer offPeakMinutesToWaitOnLogoff() {
return this.innerModel().offPeakMinutesToWaitOnLogoff();
}
public String resourceGroupName() {
return resourceGroupName;
}
public ScalingPlanPersonalScheduleInner innerModel() {
return this.innerObject;
}
private com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager manager() {
return this.serviceManager;
}
private String resourceGroupName;
private String scalingPlanName;
private String scalingPlanScheduleName;
private ScalingPlanPersonalSchedulePatch updateScalingPlanSchedule;
public ScalingPlanPersonalScheduleImpl withExistingScalingPlan(String resourceGroupName, String scalingPlanName) {
this.resourceGroupName = resourceGroupName;
this.scalingPlanName = scalingPlanName;
return this;
}
public ScalingPlanPersonalSchedule create() {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPersonalSchedules()
.createWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, this.innerModel(),
Context.NONE)
.getValue();
return this;
}
public ScalingPlanPersonalSchedule create(Context context) {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPersonalSchedules()
.createWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, this.innerModel(), context)
.getValue();
return this;
}
ScalingPlanPersonalScheduleImpl(String name,
com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager serviceManager) {
this.innerObject = new ScalingPlanPersonalScheduleInner();
this.serviceManager = serviceManager;
this.scalingPlanScheduleName = name;
}
public ScalingPlanPersonalScheduleImpl update() {
this.updateScalingPlanSchedule = new ScalingPlanPersonalSchedulePatch();
return this;
}
public ScalingPlanPersonalSchedule apply() {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPersonalSchedules()
.updateWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, updateScalingPlanSchedule,
Context.NONE)
.getValue();
return this;
}
public ScalingPlanPersonalSchedule apply(Context context) {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPersonalSchedules()
.updateWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, updateScalingPlanSchedule,
context)
.getValue();
return this;
}
ScalingPlanPersonalScheduleImpl(ScalingPlanPersonalScheduleInner innerObject,
com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager serviceManager) {
this.innerObject = innerObject;
this.serviceManager = serviceManager;
this.resourceGroupName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "resourceGroups");
this.scalingPlanName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "scalingPlans");
this.scalingPlanScheduleName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "personalSchedules");
}
public ScalingPlanPersonalSchedule refresh() {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPersonalSchedules()
.getWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, Context.NONE)
.getValue();
return this;
}
public ScalingPlanPersonalSchedule refresh(Context context) {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPersonalSchedules()
.getWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, context)
.getValue();
return this;
}
public ScalingPlanPersonalScheduleImpl withDaysOfWeek(List daysOfWeek) {
if (isInCreateMode()) {
this.innerModel().withDaysOfWeek(daysOfWeek);
return this;
} else {
this.updateScalingPlanSchedule.withDaysOfWeek(daysOfWeek);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampUpStartTime(Time rampUpStartTime) {
if (isInCreateMode()) {
this.innerModel().withRampUpStartTime(rampUpStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpStartTime(rampUpStartTime);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampUpAutoStartHosts(StartupBehavior rampUpAutoStartHosts) {
if (isInCreateMode()) {
this.innerModel().withRampUpAutoStartHosts(rampUpAutoStartHosts);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpAutoStartHosts(rampUpAutoStartHosts);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampUpStartVMOnConnect(SetStartVMOnConnect rampUpStartVMOnConnect) {
if (isInCreateMode()) {
this.innerModel().withRampUpStartVMOnConnect(rampUpStartVMOnConnect);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpStartVMOnConnect(rampUpStartVMOnConnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl
withRampUpActionOnDisconnect(SessionHandlingOperation rampUpActionOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withRampUpActionOnDisconnect(rampUpActionOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpActionOnDisconnect(rampUpActionOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl
withRampUpMinutesToWaitOnDisconnect(Integer rampUpMinutesToWaitOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withRampUpMinutesToWaitOnDisconnect(rampUpMinutesToWaitOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpMinutesToWaitOnDisconnect(rampUpMinutesToWaitOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampUpActionOnLogoff(SessionHandlingOperation rampUpActionOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withRampUpActionOnLogoff(rampUpActionOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpActionOnLogoff(rampUpActionOnLogoff);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampUpMinutesToWaitOnLogoff(Integer rampUpMinutesToWaitOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withRampUpMinutesToWaitOnLogoff(rampUpMinutesToWaitOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpMinutesToWaitOnLogoff(rampUpMinutesToWaitOnLogoff);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withPeakStartTime(Time peakStartTime) {
if (isInCreateMode()) {
this.innerModel().withPeakStartTime(peakStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withPeakStartTime(peakStartTime);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withPeakStartVMOnConnect(SetStartVMOnConnect peakStartVMOnConnect) {
if (isInCreateMode()) {
this.innerModel().withPeakStartVMOnConnect(peakStartVMOnConnect);
return this;
} else {
this.updateScalingPlanSchedule.withPeakStartVMOnConnect(peakStartVMOnConnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withPeakActionOnDisconnect(SessionHandlingOperation peakActionOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withPeakActionOnDisconnect(peakActionOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withPeakActionOnDisconnect(peakActionOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withPeakMinutesToWaitOnDisconnect(Integer peakMinutesToWaitOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withPeakMinutesToWaitOnDisconnect(peakMinutesToWaitOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withPeakMinutesToWaitOnDisconnect(peakMinutesToWaitOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withPeakActionOnLogoff(SessionHandlingOperation peakActionOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withPeakActionOnLogoff(peakActionOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withPeakActionOnLogoff(peakActionOnLogoff);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withPeakMinutesToWaitOnLogoff(Integer peakMinutesToWaitOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withPeakMinutesToWaitOnLogoff(peakMinutesToWaitOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withPeakMinutesToWaitOnLogoff(peakMinutesToWaitOnLogoff);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampDownStartTime(Time rampDownStartTime) {
if (isInCreateMode()) {
this.innerModel().withRampDownStartTime(rampDownStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownStartTime(rampDownStartTime);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampDownStartVMOnConnect(SetStartVMOnConnect rampDownStartVMOnConnect) {
if (isInCreateMode()) {
this.innerModel().withRampDownStartVMOnConnect(rampDownStartVMOnConnect);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownStartVMOnConnect(rampDownStartVMOnConnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl
withRampDownActionOnDisconnect(SessionHandlingOperation rampDownActionOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withRampDownActionOnDisconnect(rampDownActionOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownActionOnDisconnect(rampDownActionOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl
withRampDownMinutesToWaitOnDisconnect(Integer rampDownMinutesToWaitOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withRampDownMinutesToWaitOnDisconnect(rampDownMinutesToWaitOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownMinutesToWaitOnDisconnect(rampDownMinutesToWaitOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampDownActionOnLogoff(SessionHandlingOperation rampDownActionOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withRampDownActionOnLogoff(rampDownActionOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownActionOnLogoff(rampDownActionOnLogoff);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withRampDownMinutesToWaitOnLogoff(Integer rampDownMinutesToWaitOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withRampDownMinutesToWaitOnLogoff(rampDownMinutesToWaitOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownMinutesToWaitOnLogoff(rampDownMinutesToWaitOnLogoff);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withOffPeakStartTime(Time offPeakStartTime) {
if (isInCreateMode()) {
this.innerModel().withOffPeakStartTime(offPeakStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakStartTime(offPeakStartTime);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withOffPeakStartVMOnConnect(SetStartVMOnConnect offPeakStartVMOnConnect) {
if (isInCreateMode()) {
this.innerModel().withOffPeakStartVMOnConnect(offPeakStartVMOnConnect);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakStartVMOnConnect(offPeakStartVMOnConnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl
withOffPeakActionOnDisconnect(SessionHandlingOperation offPeakActionOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withOffPeakActionOnDisconnect(offPeakActionOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakActionOnDisconnect(offPeakActionOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl
withOffPeakMinutesToWaitOnDisconnect(Integer offPeakMinutesToWaitOnDisconnect) {
if (isInCreateMode()) {
this.innerModel().withOffPeakMinutesToWaitOnDisconnect(offPeakMinutesToWaitOnDisconnect);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakMinutesToWaitOnDisconnect(offPeakMinutesToWaitOnDisconnect);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withOffPeakActionOnLogoff(SessionHandlingOperation offPeakActionOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withOffPeakActionOnLogoff(offPeakActionOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakActionOnLogoff(offPeakActionOnLogoff);
return this;
}
}
public ScalingPlanPersonalScheduleImpl withOffPeakMinutesToWaitOnLogoff(Integer offPeakMinutesToWaitOnLogoff) {
if (isInCreateMode()) {
this.innerModel().withOffPeakMinutesToWaitOnLogoff(offPeakMinutesToWaitOnLogoff);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakMinutesToWaitOnLogoff(offPeakMinutesToWaitOnLogoff);
return this;
}
}
private boolean isInCreateMode() {
return this.innerModel().id() == null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy