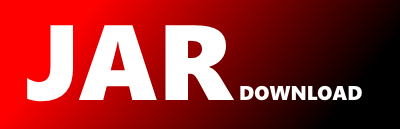
com.azure.resourcemanager.desktopvirtualization.implementation.ScalingPlanPooledScheduleImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.implementation;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPooledScheduleInner;
import com.azure.resourcemanager.desktopvirtualization.models.DayOfWeek;
import com.azure.resourcemanager.desktopvirtualization.models.ScalingPlanPooledSchedule;
import com.azure.resourcemanager.desktopvirtualization.models.ScalingPlanPooledSchedulePatch;
import com.azure.resourcemanager.desktopvirtualization.models.SessionHostLoadBalancingAlgorithm;
import com.azure.resourcemanager.desktopvirtualization.models.StopHostsWhen;
import com.azure.resourcemanager.desktopvirtualization.models.Time;
import java.util.Collections;
import java.util.List;
public final class ScalingPlanPooledScheduleImpl
implements ScalingPlanPooledSchedule, ScalingPlanPooledSchedule.Definition, ScalingPlanPooledSchedule.Update {
private ScalingPlanPooledScheduleInner innerObject;
private final com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager serviceManager;
public String id() {
return this.innerModel().id();
}
public String name() {
return this.innerModel().name();
}
public String type() {
return this.innerModel().type();
}
public SystemData systemData() {
return this.innerModel().systemData();
}
public List daysOfWeek() {
List inner = this.innerModel().daysOfWeek();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public Time rampUpStartTime() {
return this.innerModel().rampUpStartTime();
}
public SessionHostLoadBalancingAlgorithm rampUpLoadBalancingAlgorithm() {
return this.innerModel().rampUpLoadBalancingAlgorithm();
}
public Integer rampUpMinimumHostsPct() {
return this.innerModel().rampUpMinimumHostsPct();
}
public Integer rampUpCapacityThresholdPct() {
return this.innerModel().rampUpCapacityThresholdPct();
}
public Time peakStartTime() {
return this.innerModel().peakStartTime();
}
public SessionHostLoadBalancingAlgorithm peakLoadBalancingAlgorithm() {
return this.innerModel().peakLoadBalancingAlgorithm();
}
public Time rampDownStartTime() {
return this.innerModel().rampDownStartTime();
}
public SessionHostLoadBalancingAlgorithm rampDownLoadBalancingAlgorithm() {
return this.innerModel().rampDownLoadBalancingAlgorithm();
}
public Integer rampDownMinimumHostsPct() {
return this.innerModel().rampDownMinimumHostsPct();
}
public Integer rampDownCapacityThresholdPct() {
return this.innerModel().rampDownCapacityThresholdPct();
}
public Boolean rampDownForceLogoffUsers() {
return this.innerModel().rampDownForceLogoffUsers();
}
public StopHostsWhen rampDownStopHostsWhen() {
return this.innerModel().rampDownStopHostsWhen();
}
public Integer rampDownWaitTimeMinutes() {
return this.innerModel().rampDownWaitTimeMinutes();
}
public String rampDownNotificationMessage() {
return this.innerModel().rampDownNotificationMessage();
}
public Time offPeakStartTime() {
return this.innerModel().offPeakStartTime();
}
public SessionHostLoadBalancingAlgorithm offPeakLoadBalancingAlgorithm() {
return this.innerModel().offPeakLoadBalancingAlgorithm();
}
public String resourceGroupName() {
return resourceGroupName;
}
public ScalingPlanPooledScheduleInner innerModel() {
return this.innerObject;
}
private com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager manager() {
return this.serviceManager;
}
private String resourceGroupName;
private String scalingPlanName;
private String scalingPlanScheduleName;
private ScalingPlanPooledSchedulePatch updateScalingPlanSchedule;
public ScalingPlanPooledScheduleImpl withExistingScalingPlan(String resourceGroupName, String scalingPlanName) {
this.resourceGroupName = resourceGroupName;
this.scalingPlanName = scalingPlanName;
return this;
}
public ScalingPlanPooledSchedule create() {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPooledSchedules()
.createWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, this.innerModel(),
Context.NONE)
.getValue();
return this;
}
public ScalingPlanPooledSchedule create(Context context) {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPooledSchedules()
.createWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, this.innerModel(), context)
.getValue();
return this;
}
ScalingPlanPooledScheduleImpl(String name,
com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager serviceManager) {
this.innerObject = new ScalingPlanPooledScheduleInner();
this.serviceManager = serviceManager;
this.scalingPlanScheduleName = name;
}
public ScalingPlanPooledScheduleImpl update() {
this.updateScalingPlanSchedule = new ScalingPlanPooledSchedulePatch();
return this;
}
public ScalingPlanPooledSchedule apply() {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPooledSchedules()
.updateWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, updateScalingPlanSchedule,
Context.NONE)
.getValue();
return this;
}
public ScalingPlanPooledSchedule apply(Context context) {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPooledSchedules()
.updateWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, updateScalingPlanSchedule,
context)
.getValue();
return this;
}
ScalingPlanPooledScheduleImpl(ScalingPlanPooledScheduleInner innerObject,
com.azure.resourcemanager.desktopvirtualization.DesktopVirtualizationManager serviceManager) {
this.innerObject = innerObject;
this.serviceManager = serviceManager;
this.resourceGroupName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "resourceGroups");
this.scalingPlanName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "scalingPlans");
this.scalingPlanScheduleName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "pooledSchedules");
}
public ScalingPlanPooledSchedule refresh() {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPooledSchedules()
.getWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, Context.NONE)
.getValue();
return this;
}
public ScalingPlanPooledSchedule refresh(Context context) {
this.innerObject = serviceManager.serviceClient()
.getScalingPlanPooledSchedules()
.getWithResponse(resourceGroupName, scalingPlanName, scalingPlanScheduleName, context)
.getValue();
return this;
}
public ScalingPlanPooledScheduleImpl withDaysOfWeek(List daysOfWeek) {
if (isInCreateMode()) {
this.innerModel().withDaysOfWeek(daysOfWeek);
return this;
} else {
this.updateScalingPlanSchedule.withDaysOfWeek(daysOfWeek);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampUpStartTime(Time rampUpStartTime) {
if (isInCreateMode()) {
this.innerModel().withRampUpStartTime(rampUpStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpStartTime(rampUpStartTime);
return this;
}
}
public ScalingPlanPooledScheduleImpl
withRampUpLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm rampUpLoadBalancingAlgorithm) {
if (isInCreateMode()) {
this.innerModel().withRampUpLoadBalancingAlgorithm(rampUpLoadBalancingAlgorithm);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpLoadBalancingAlgorithm(rampUpLoadBalancingAlgorithm);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampUpMinimumHostsPct(Integer rampUpMinimumHostsPct) {
if (isInCreateMode()) {
this.innerModel().withRampUpMinimumHostsPct(rampUpMinimumHostsPct);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpMinimumHostsPct(rampUpMinimumHostsPct);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampUpCapacityThresholdPct(Integer rampUpCapacityThresholdPct) {
if (isInCreateMode()) {
this.innerModel().withRampUpCapacityThresholdPct(rampUpCapacityThresholdPct);
return this;
} else {
this.updateScalingPlanSchedule.withRampUpCapacityThresholdPct(rampUpCapacityThresholdPct);
return this;
}
}
public ScalingPlanPooledScheduleImpl withPeakStartTime(Time peakStartTime) {
if (isInCreateMode()) {
this.innerModel().withPeakStartTime(peakStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withPeakStartTime(peakStartTime);
return this;
}
}
public ScalingPlanPooledScheduleImpl
withPeakLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm peakLoadBalancingAlgorithm) {
if (isInCreateMode()) {
this.innerModel().withPeakLoadBalancingAlgorithm(peakLoadBalancingAlgorithm);
return this;
} else {
this.updateScalingPlanSchedule.withPeakLoadBalancingAlgorithm(peakLoadBalancingAlgorithm);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampDownStartTime(Time rampDownStartTime) {
if (isInCreateMode()) {
this.innerModel().withRampDownStartTime(rampDownStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownStartTime(rampDownStartTime);
return this;
}
}
public ScalingPlanPooledScheduleImpl
withRampDownLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm rampDownLoadBalancingAlgorithm) {
if (isInCreateMode()) {
this.innerModel().withRampDownLoadBalancingAlgorithm(rampDownLoadBalancingAlgorithm);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownLoadBalancingAlgorithm(rampDownLoadBalancingAlgorithm);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampDownMinimumHostsPct(Integer rampDownMinimumHostsPct) {
if (isInCreateMode()) {
this.innerModel().withRampDownMinimumHostsPct(rampDownMinimumHostsPct);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownMinimumHostsPct(rampDownMinimumHostsPct);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampDownCapacityThresholdPct(Integer rampDownCapacityThresholdPct) {
if (isInCreateMode()) {
this.innerModel().withRampDownCapacityThresholdPct(rampDownCapacityThresholdPct);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownCapacityThresholdPct(rampDownCapacityThresholdPct);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampDownForceLogoffUsers(Boolean rampDownForceLogoffUsers) {
if (isInCreateMode()) {
this.innerModel().withRampDownForceLogoffUsers(rampDownForceLogoffUsers);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownForceLogoffUsers(rampDownForceLogoffUsers);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampDownStopHostsWhen(StopHostsWhen rampDownStopHostsWhen) {
if (isInCreateMode()) {
this.innerModel().withRampDownStopHostsWhen(rampDownStopHostsWhen);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownStopHostsWhen(rampDownStopHostsWhen);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampDownWaitTimeMinutes(Integer rampDownWaitTimeMinutes) {
if (isInCreateMode()) {
this.innerModel().withRampDownWaitTimeMinutes(rampDownWaitTimeMinutes);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownWaitTimeMinutes(rampDownWaitTimeMinutes);
return this;
}
}
public ScalingPlanPooledScheduleImpl withRampDownNotificationMessage(String rampDownNotificationMessage) {
if (isInCreateMode()) {
this.innerModel().withRampDownNotificationMessage(rampDownNotificationMessage);
return this;
} else {
this.updateScalingPlanSchedule.withRampDownNotificationMessage(rampDownNotificationMessage);
return this;
}
}
public ScalingPlanPooledScheduleImpl withOffPeakStartTime(Time offPeakStartTime) {
if (isInCreateMode()) {
this.innerModel().withOffPeakStartTime(offPeakStartTime);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakStartTime(offPeakStartTime);
return this;
}
}
public ScalingPlanPooledScheduleImpl
withOffPeakLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm offPeakLoadBalancingAlgorithm) {
if (isInCreateMode()) {
this.innerModel().withOffPeakLoadBalancingAlgorithm(offPeakLoadBalancingAlgorithm);
return this;
} else {
this.updateScalingPlanSchedule.withOffPeakLoadBalancingAlgorithm(offPeakLoadBalancingAlgorithm);
return this;
}
}
private boolean isInCreateMode() {
return this.innerModel().id() == null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy