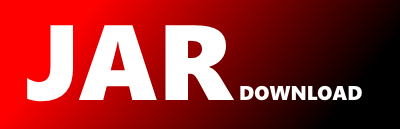
com.azure.resourcemanager.desktopvirtualization.models.AppAttachPackageInfoProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.List;
/**
* Schema for Import Package Information properties.
*/
@Fluent
public final class AppAttachPackageInfoProperties implements JsonSerializable {
/*
* Alias of App Attach Package. Assigned at import time
*/
private String packageAlias;
/*
* VHD/CIM image path on Network Share.
*/
private String imagePath;
/*
* Package Name from appxmanifest.xml.
*/
private String packageName;
/*
* Package Family Name from appxmanifest.xml. Contains Package Name and Publisher name.
*/
private String packageFamilyName;
/*
* Package Full Name from appxmanifest.xml.
*/
private String packageFullName;
/*
* User friendly Name to be displayed in the portal.
*/
private String displayName;
/*
* Relative Path to the package inside the image.
*/
private String packageRelativePath;
/*
* Specifies how to register Package in feed.
*/
private Boolean isRegularRegistration;
/*
* Make this version of the package the active one across the hostpool.
*/
private Boolean isActive;
/*
* List of package dependencies.
*/
private List packageDependencies;
/*
* Package version found in the appxmanifest.xml.
*/
private String version;
/*
* Date Package was last updated, found in the appxmanifest.xml.
*/
private OffsetDateTime lastUpdated;
/*
* List of package applications.
*/
private List packageApplications;
/*
* Certificate name found in the appxmanifest.xml.
*/
private String certificateName;
/*
* Date certificate expires, found in the appxmanifest.xml.
*/
private OffsetDateTime certificateExpiry;
/*
* Is package timestamped so it can ignore the certificate expiry date
*/
private PackageTimestamped isPackageTimestamped;
/**
* Creates an instance of AppAttachPackageInfoProperties class.
*/
public AppAttachPackageInfoProperties() {
}
/**
* Get the packageAlias property: Alias of App Attach Package. Assigned at import time.
*
* @return the packageAlias value.
*/
public String packageAlias() {
return this.packageAlias;
}
/**
* Set the packageAlias property: Alias of App Attach Package. Assigned at import time.
*
* @param packageAlias the packageAlias value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withPackageAlias(String packageAlias) {
this.packageAlias = packageAlias;
return this;
}
/**
* Get the imagePath property: VHD/CIM image path on Network Share.
*
* @return the imagePath value.
*/
public String imagePath() {
return this.imagePath;
}
/**
* Set the imagePath property: VHD/CIM image path on Network Share.
*
* @param imagePath the imagePath value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withImagePath(String imagePath) {
this.imagePath = imagePath;
return this;
}
/**
* Get the packageName property: Package Name from appxmanifest.xml.
*
* @return the packageName value.
*/
public String packageName() {
return this.packageName;
}
/**
* Set the packageName property: Package Name from appxmanifest.xml.
*
* @param packageName the packageName value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withPackageName(String packageName) {
this.packageName = packageName;
return this;
}
/**
* Get the packageFamilyName property: Package Family Name from appxmanifest.xml. Contains Package Name and
* Publisher name.
*
* @return the packageFamilyName value.
*/
public String packageFamilyName() {
return this.packageFamilyName;
}
/**
* Set the packageFamilyName property: Package Family Name from appxmanifest.xml. Contains Package Name and
* Publisher name.
*
* @param packageFamilyName the packageFamilyName value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withPackageFamilyName(String packageFamilyName) {
this.packageFamilyName = packageFamilyName;
return this;
}
/**
* Get the packageFullName property: Package Full Name from appxmanifest.xml.
*
* @return the packageFullName value.
*/
public String packageFullName() {
return this.packageFullName;
}
/**
* Set the packageFullName property: Package Full Name from appxmanifest.xml.
*
* @param packageFullName the packageFullName value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withPackageFullName(String packageFullName) {
this.packageFullName = packageFullName;
return this;
}
/**
* Get the displayName property: User friendly Name to be displayed in the portal.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Set the displayName property: User friendly Name to be displayed in the portal.
*
* @param displayName the displayName value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get the packageRelativePath property: Relative Path to the package inside the image.
*
* @return the packageRelativePath value.
*/
public String packageRelativePath() {
return this.packageRelativePath;
}
/**
* Set the packageRelativePath property: Relative Path to the package inside the image.
*
* @param packageRelativePath the packageRelativePath value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withPackageRelativePath(String packageRelativePath) {
this.packageRelativePath = packageRelativePath;
return this;
}
/**
* Get the isRegularRegistration property: Specifies how to register Package in feed.
*
* @return the isRegularRegistration value.
*/
public Boolean isRegularRegistration() {
return this.isRegularRegistration;
}
/**
* Set the isRegularRegistration property: Specifies how to register Package in feed.
*
* @param isRegularRegistration the isRegularRegistration value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withIsRegularRegistration(Boolean isRegularRegistration) {
this.isRegularRegistration = isRegularRegistration;
return this;
}
/**
* Get the isActive property: Make this version of the package the active one across the hostpool.
*
* @return the isActive value.
*/
public Boolean isActive() {
return this.isActive;
}
/**
* Set the isActive property: Make this version of the package the active one across the hostpool.
*
* @param isActive the isActive value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withIsActive(Boolean isActive) {
this.isActive = isActive;
return this;
}
/**
* Get the packageDependencies property: List of package dependencies.
*
* @return the packageDependencies value.
*/
public List packageDependencies() {
return this.packageDependencies;
}
/**
* Set the packageDependencies property: List of package dependencies.
*
* @param packageDependencies the packageDependencies value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withPackageDependencies(List packageDependencies) {
this.packageDependencies = packageDependencies;
return this;
}
/**
* Get the version property: Package version found in the appxmanifest.xml.
*
* @return the version value.
*/
public String version() {
return this.version;
}
/**
* Set the version property: Package version found in the appxmanifest.xml.
*
* @param version the version value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withVersion(String version) {
this.version = version;
return this;
}
/**
* Get the lastUpdated property: Date Package was last updated, found in the appxmanifest.xml.
*
* @return the lastUpdated value.
*/
public OffsetDateTime lastUpdated() {
return this.lastUpdated;
}
/**
* Set the lastUpdated property: Date Package was last updated, found in the appxmanifest.xml.
*
* @param lastUpdated the lastUpdated value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withLastUpdated(OffsetDateTime lastUpdated) {
this.lastUpdated = lastUpdated;
return this;
}
/**
* Get the packageApplications property: List of package applications.
*
* @return the packageApplications value.
*/
public List packageApplications() {
return this.packageApplications;
}
/**
* Set the packageApplications property: List of package applications.
*
* @param packageApplications the packageApplications value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withPackageApplications(List packageApplications) {
this.packageApplications = packageApplications;
return this;
}
/**
* Get the certificateName property: Certificate name found in the appxmanifest.xml.
*
* @return the certificateName value.
*/
public String certificateName() {
return this.certificateName;
}
/**
* Set the certificateName property: Certificate name found in the appxmanifest.xml.
*
* @param certificateName the certificateName value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withCertificateName(String certificateName) {
this.certificateName = certificateName;
return this;
}
/**
* Get the certificateExpiry property: Date certificate expires, found in the appxmanifest.xml.
*
* @return the certificateExpiry value.
*/
public OffsetDateTime certificateExpiry() {
return this.certificateExpiry;
}
/**
* Set the certificateExpiry property: Date certificate expires, found in the appxmanifest.xml.
*
* @param certificateExpiry the certificateExpiry value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withCertificateExpiry(OffsetDateTime certificateExpiry) {
this.certificateExpiry = certificateExpiry;
return this;
}
/**
* Get the isPackageTimestamped property: Is package timestamped so it can ignore the certificate expiry date.
*
* @return the isPackageTimestamped value.
*/
public PackageTimestamped isPackageTimestamped() {
return this.isPackageTimestamped;
}
/**
* Set the isPackageTimestamped property: Is package timestamped so it can ignore the certificate expiry date.
*
* @param isPackageTimestamped the isPackageTimestamped value to set.
* @return the AppAttachPackageInfoProperties object itself.
*/
public AppAttachPackageInfoProperties withIsPackageTimestamped(PackageTimestamped isPackageTimestamped) {
this.isPackageTimestamped = isPackageTimestamped;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (packageDependencies() != null) {
packageDependencies().forEach(e -> e.validate());
}
if (packageApplications() != null) {
packageApplications().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("packageAlias", this.packageAlias);
jsonWriter.writeStringField("imagePath", this.imagePath);
jsonWriter.writeStringField("packageName", this.packageName);
jsonWriter.writeStringField("packageFamilyName", this.packageFamilyName);
jsonWriter.writeStringField("packageFullName", this.packageFullName);
jsonWriter.writeStringField("displayName", this.displayName);
jsonWriter.writeStringField("packageRelativePath", this.packageRelativePath);
jsonWriter.writeBooleanField("isRegularRegistration", this.isRegularRegistration);
jsonWriter.writeBooleanField("isActive", this.isActive);
jsonWriter.writeArrayField("packageDependencies", this.packageDependencies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("version", this.version);
jsonWriter.writeStringField("lastUpdated",
this.lastUpdated == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.lastUpdated));
jsonWriter.writeArrayField("packageApplications", this.packageApplications,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("certificateName", this.certificateName);
jsonWriter.writeStringField("certificateExpiry",
this.certificateExpiry == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.certificateExpiry));
jsonWriter.writeStringField("isPackageTimestamped",
this.isPackageTimestamped == null ? null : this.isPackageTimestamped.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AppAttachPackageInfoProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AppAttachPackageInfoProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AppAttachPackageInfoProperties.
*/
public static AppAttachPackageInfoProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AppAttachPackageInfoProperties deserializedAppAttachPackageInfoProperties
= new AppAttachPackageInfoProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("packageAlias".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.packageAlias = reader.getString();
} else if ("imagePath".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.imagePath = reader.getString();
} else if ("packageName".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.packageName = reader.getString();
} else if ("packageFamilyName".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.packageFamilyName = reader.getString();
} else if ("packageFullName".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.packageFullName = reader.getString();
} else if ("displayName".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.displayName = reader.getString();
} else if ("packageRelativePath".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.packageRelativePath = reader.getString();
} else if ("isRegularRegistration".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.isRegularRegistration
= reader.getNullable(JsonReader::getBoolean);
} else if ("isActive".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.isActive = reader.getNullable(JsonReader::getBoolean);
} else if ("packageDependencies".equals(fieldName)) {
List packageDependencies
= reader.readArray(reader1 -> MsixPackageDependencies.fromJson(reader1));
deserializedAppAttachPackageInfoProperties.packageDependencies = packageDependencies;
} else if ("version".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.version = reader.getString();
} else if ("lastUpdated".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.lastUpdated = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("packageApplications".equals(fieldName)) {
List packageApplications
= reader.readArray(reader1 -> MsixPackageApplications.fromJson(reader1));
deserializedAppAttachPackageInfoProperties.packageApplications = packageApplications;
} else if ("certificateName".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.certificateName = reader.getString();
} else if ("certificateExpiry".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.certificateExpiry = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("isPackageTimestamped".equals(fieldName)) {
deserializedAppAttachPackageInfoProperties.isPackageTimestamped
= PackageTimestamped.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedAppAttachPackageInfoProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy