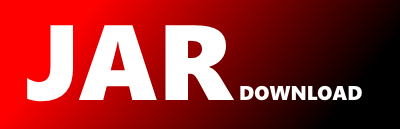
com.azure.resourcemanager.desktopvirtualization.models.MsixPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.models;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.desktopvirtualization.fluent.models.MsixPackageInner;
import java.time.OffsetDateTime;
import java.util.List;
/**
* An immutable client-side representation of MsixPackage.
*/
public interface MsixPackage {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the imagePath property: VHD/CIM image path on Network Share.
*
* @return the imagePath value.
*/
String imagePath();
/**
* Gets the packageName property: Package Name from appxmanifest.xml.
*
* @return the packageName value.
*/
String packageName();
/**
* Gets the packageFamilyName property: Package Family Name from appxmanifest.xml. Contains Package Name and
* Publisher name.
*
* @return the packageFamilyName value.
*/
String packageFamilyName();
/**
* Gets the displayName property: User friendly Name to be displayed in the portal.
*
* @return the displayName value.
*/
String displayName();
/**
* Gets the packageRelativePath property: Relative Path to the package inside the image.
*
* @return the packageRelativePath value.
*/
String packageRelativePath();
/**
* Gets the isRegularRegistration property: Specifies how to register Package in feed.
*
* @return the isRegularRegistration value.
*/
Boolean isRegularRegistration();
/**
* Gets the isActive property: Make this version of the package the active one across the hostpool.
*
* @return the isActive value.
*/
Boolean isActive();
/**
* Gets the packageDependencies property: List of package dependencies.
*
* @return the packageDependencies value.
*/
List packageDependencies();
/**
* Gets the version property: Package version found in the appxmanifest.xml.
*
* @return the version value.
*/
String version();
/**
* Gets the lastUpdated property: Date Package was last updated, found in the appxmanifest.xml.
*
* @return the lastUpdated value.
*/
OffsetDateTime lastUpdated();
/**
* Gets the packageApplications property: List of package applications.
*
* @return the packageApplications value.
*/
List packageApplications();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.desktopvirtualization.fluent.models.MsixPackageInner object.
*
* @return the inner object.
*/
MsixPackageInner innerModel();
/**
* The entirety of the MsixPackage definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The MsixPackage definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the MsixPackage definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the MsixPackage definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, hostPoolName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param hostPoolName The name of the host pool within the specified resource group.
* @return the next definition stage.
*/
WithCreate withExistingHostPool(String resourceGroupName, String hostPoolName);
}
/**
* The stage of the MsixPackage definition which contains all the minimum required properties for the resource
* to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithImagePath, DefinitionStages.WithPackageName,
DefinitionStages.WithPackageFamilyName, DefinitionStages.WithDisplayName,
DefinitionStages.WithPackageRelativePath, DefinitionStages.WithIsRegularRegistration,
DefinitionStages.WithIsActive, DefinitionStages.WithPackageDependencies, DefinitionStages.WithVersion,
DefinitionStages.WithLastUpdated, DefinitionStages.WithPackageApplications {
/**
* Executes the create request.
*
* @return the created resource.
*/
MsixPackage create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
MsixPackage create(Context context);
}
/**
* The stage of the MsixPackage definition allowing to specify imagePath.
*/
interface WithImagePath {
/**
* Specifies the imagePath property: VHD/CIM image path on Network Share..
*
* @param imagePath VHD/CIM image path on Network Share.
* @return the next definition stage.
*/
WithCreate withImagePath(String imagePath);
}
/**
* The stage of the MsixPackage definition allowing to specify packageName.
*/
interface WithPackageName {
/**
* Specifies the packageName property: Package Name from appxmanifest.xml. .
*
* @param packageName Package Name from appxmanifest.xml.
* @return the next definition stage.
*/
WithCreate withPackageName(String packageName);
}
/**
* The stage of the MsixPackage definition allowing to specify packageFamilyName.
*/
interface WithPackageFamilyName {
/**
* Specifies the packageFamilyName property: Package Family Name from appxmanifest.xml. Contains Package
* Name and Publisher name. .
*
* @param packageFamilyName Package Family Name from appxmanifest.xml. Contains Package Name and Publisher
* name.
* @return the next definition stage.
*/
WithCreate withPackageFamilyName(String packageFamilyName);
}
/**
* The stage of the MsixPackage definition allowing to specify displayName.
*/
interface WithDisplayName {
/**
* Specifies the displayName property: User friendly Name to be displayed in the portal. .
*
* @param displayName User friendly Name to be displayed in the portal.
* @return the next definition stage.
*/
WithCreate withDisplayName(String displayName);
}
/**
* The stage of the MsixPackage definition allowing to specify packageRelativePath.
*/
interface WithPackageRelativePath {
/**
* Specifies the packageRelativePath property: Relative Path to the package inside the image. .
*
* @param packageRelativePath Relative Path to the package inside the image.
* @return the next definition stage.
*/
WithCreate withPackageRelativePath(String packageRelativePath);
}
/**
* The stage of the MsixPackage definition allowing to specify isRegularRegistration.
*/
interface WithIsRegularRegistration {
/**
* Specifies the isRegularRegistration property: Specifies how to register Package in feed..
*
* @param isRegularRegistration Specifies how to register Package in feed.
* @return the next definition stage.
*/
WithCreate withIsRegularRegistration(Boolean isRegularRegistration);
}
/**
* The stage of the MsixPackage definition allowing to specify isActive.
*/
interface WithIsActive {
/**
* Specifies the isActive property: Make this version of the package the active one across the hostpool. .
*
* @param isActive Make this version of the package the active one across the hostpool.
* @return the next definition stage.
*/
WithCreate withIsActive(Boolean isActive);
}
/**
* The stage of the MsixPackage definition allowing to specify packageDependencies.
*/
interface WithPackageDependencies {
/**
* Specifies the packageDependencies property: List of package dependencies. .
*
* @param packageDependencies List of package dependencies.
* @return the next definition stage.
*/
WithCreate withPackageDependencies(List packageDependencies);
}
/**
* The stage of the MsixPackage definition allowing to specify version.
*/
interface WithVersion {
/**
* Specifies the version property: Package version found in the appxmanifest.xml. .
*
* @param version Package version found in the appxmanifest.xml.
* @return the next definition stage.
*/
WithCreate withVersion(String version);
}
/**
* The stage of the MsixPackage definition allowing to specify lastUpdated.
*/
interface WithLastUpdated {
/**
* Specifies the lastUpdated property: Date Package was last updated, found in the appxmanifest.xml. .
*
* @param lastUpdated Date Package was last updated, found in the appxmanifest.xml.
* @return the next definition stage.
*/
WithCreate withLastUpdated(OffsetDateTime lastUpdated);
}
/**
* The stage of the MsixPackage definition allowing to specify packageApplications.
*/
interface WithPackageApplications {
/**
* Specifies the packageApplications property: List of package applications. .
*
* @param packageApplications List of package applications.
* @return the next definition stage.
*/
WithCreate withPackageApplications(List packageApplications);
}
}
/**
* Begins update for the MsixPackage resource.
*
* @return the stage of resource update.
*/
MsixPackage.Update update();
/**
* The template for MsixPackage update.
*/
interface Update
extends UpdateStages.WithIsActive, UpdateStages.WithIsRegularRegistration, UpdateStages.WithDisplayName {
/**
* Executes the update request.
*
* @return the updated resource.
*/
MsixPackage apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
MsixPackage apply(Context context);
}
/**
* The MsixPackage update stages.
*/
interface UpdateStages {
/**
* The stage of the MsixPackage update allowing to specify isActive.
*/
interface WithIsActive {
/**
* Specifies the isActive property: Set a version of the package to be active across hostpool. .
*
* @param isActive Set a version of the package to be active across hostpool.
* @return the next definition stage.
*/
Update withIsActive(Boolean isActive);
}
/**
* The stage of the MsixPackage update allowing to specify isRegularRegistration.
*/
interface WithIsRegularRegistration {
/**
* Specifies the isRegularRegistration property: Set Registration mode. Regular or Delayed..
*
* @param isRegularRegistration Set Registration mode. Regular or Delayed.
* @return the next definition stage.
*/
Update withIsRegularRegistration(Boolean isRegularRegistration);
}
/**
* The stage of the MsixPackage update allowing to specify displayName.
*/
interface WithDisplayName {
/**
* Specifies the displayName property: Display name for MSIX Package..
*
* @param displayName Display name for MSIX Package.
* @return the next definition stage.
*/
Update withDisplayName(String displayName);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
MsixPackage refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
MsixPackage refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy