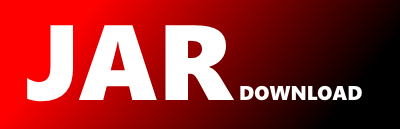
com.azure.resourcemanager.desktopvirtualization.models.ScalingPlanPersonalSchedule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.models;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPersonalScheduleInner;
import java.util.List;
/**
* An immutable client-side representation of ScalingPlanPersonalSchedule.
*/
public interface ScalingPlanPersonalSchedule {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @return the daysOfWeek value.
*/
List daysOfWeek();
/**
* Gets the rampUpStartTime property: Starting time for ramp up period.
*
* @return the rampUpStartTime value.
*/
Time rampUpStartTime();
/**
* Gets the rampUpAutoStartHosts property: The desired startup behavior during the ramp up period for personal vms
* in the hostpool.
*
* @return the rampUpAutoStartHosts value.
*/
StartupBehavior rampUpAutoStartHosts();
/**
* Gets the rampUpStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by
* turning them on manually.
*
* @return the rampUpStartVMOnConnect value.
*/
SetStartVMOnConnect rampUpStartVMOnConnect();
/**
* Gets the rampUpActionOnDisconnect property: Action to be taken after a user disconnect during the ramp up period.
*
* @return the rampUpActionOnDisconnect value.
*/
SessionHandlingOperation rampUpActionOnDisconnect();
/**
* Gets the rampUpMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp up period.
*
* @return the rampUpMinutesToWaitOnDisconnect value.
*/
Integer rampUpMinutesToWaitOnDisconnect();
/**
* Gets the rampUpActionOnLogoff property: Action to be taken after a logoff during the ramp up period.
*
* @return the rampUpActionOnLogoff value.
*/
SessionHandlingOperation rampUpActionOnLogoff();
/**
* Gets the rampUpMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp up period.
*
* @return the rampUpMinutesToWaitOnLogoff value.
*/
Integer rampUpMinutesToWaitOnLogoff();
/**
* Gets the peakStartTime property: Starting time for peak period.
*
* @return the peakStartTime value.
*/
Time peakStartTime();
/**
* Gets the peakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the peak phase.
*
* @return the peakStartVMOnConnect value.
*/
SetStartVMOnConnect peakStartVMOnConnect();
/**
* Gets the peakActionOnDisconnect property: Action to be taken after a user disconnect during the peak period.
*
* @return the peakActionOnDisconnect value.
*/
SessionHandlingOperation peakActionOnDisconnect();
/**
* Gets the peakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the peak period.
*
* @return the peakMinutesToWaitOnDisconnect value.
*/
Integer peakMinutesToWaitOnDisconnect();
/**
* Gets the peakActionOnLogoff property: Action to be taken after a logoff during the peak period.
*
* @return the peakActionOnLogoff value.
*/
SessionHandlingOperation peakActionOnLogoff();
/**
* Gets the peakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the peak period.
*
* @return the peakMinutesToWaitOnLogoff value.
*/
Integer peakMinutesToWaitOnLogoff();
/**
* Gets the rampDownStartTime property: Starting time for ramp down period.
*
* @return the rampDownStartTime value.
*/
Time rampDownStartTime();
/**
* Gets the rampDownStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the ramp down phase.
*
* @return the rampDownStartVMOnConnect value.
*/
SetStartVMOnConnect rampDownStartVMOnConnect();
/**
* Gets the rampDownActionOnDisconnect property: Action to be taken after a user disconnect during the ramp down
* period.
*
* @return the rampDownActionOnDisconnect value.
*/
SessionHandlingOperation rampDownActionOnDisconnect();
/**
* Gets the rampDownMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp down period.
*
* @return the rampDownMinutesToWaitOnDisconnect value.
*/
Integer rampDownMinutesToWaitOnDisconnect();
/**
* Gets the rampDownActionOnLogoff property: Action to be taken after a logoff during the ramp down period.
*
* @return the rampDownActionOnLogoff value.
*/
SessionHandlingOperation rampDownActionOnLogoff();
/**
* Gets the rampDownMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired
* session handling action when a user logs off during the ramp down period.
*
* @return the rampDownMinutesToWaitOnLogoff value.
*/
Integer rampDownMinutesToWaitOnLogoff();
/**
* Gets the offPeakStartTime property: Starting time for off-peak period.
*
* @return the offPeakStartTime value.
*/
Time offPeakStartTime();
/**
* Gets the offPeakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the off-peak phase.
*
* @return the offPeakStartVMOnConnect value.
*/
SetStartVMOnConnect offPeakStartVMOnConnect();
/**
* Gets the offPeakActionOnDisconnect property: Action to be taken after a user disconnect during the off-peak
* period.
*
* @return the offPeakActionOnDisconnect value.
*/
SessionHandlingOperation offPeakActionOnDisconnect();
/**
* Gets the offPeakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the off-peak period.
*
* @return the offPeakMinutesToWaitOnDisconnect value.
*/
Integer offPeakMinutesToWaitOnDisconnect();
/**
* Gets the offPeakActionOnLogoff property: Action to be taken after a logoff during the off-peak period.
*
* @return the offPeakActionOnLogoff value.
*/
SessionHandlingOperation offPeakActionOnLogoff();
/**
* Gets the offPeakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the off-peak period.
*
* @return the offPeakMinutesToWaitOnLogoff value.
*/
Integer offPeakMinutesToWaitOnLogoff();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPersonalScheduleInner
* object.
*
* @return the inner object.
*/
ScalingPlanPersonalScheduleInner innerModel();
/**
* The entirety of the ScalingPlanPersonalSchedule definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The ScalingPlanPersonalSchedule definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the ScalingPlanPersonalSchedule definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, scalingPlanName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param scalingPlanName The name of the scaling plan.
* @return the next definition stage.
*/
WithCreate withExistingScalingPlan(String resourceGroupName, String scalingPlanName);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition which contains all the minimum required properties
* for the resource to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithDaysOfWeek, DefinitionStages.WithRampUpStartTime,
DefinitionStages.WithRampUpAutoStartHosts, DefinitionStages.WithRampUpStartVMOnConnect,
DefinitionStages.WithRampUpActionOnDisconnect, DefinitionStages.WithRampUpMinutesToWaitOnDisconnect,
DefinitionStages.WithRampUpActionOnLogoff, DefinitionStages.WithRampUpMinutesToWaitOnLogoff,
DefinitionStages.WithPeakStartTime, DefinitionStages.WithPeakStartVMOnConnect,
DefinitionStages.WithPeakActionOnDisconnect, DefinitionStages.WithPeakMinutesToWaitOnDisconnect,
DefinitionStages.WithPeakActionOnLogoff, DefinitionStages.WithPeakMinutesToWaitOnLogoff,
DefinitionStages.WithRampDownStartTime, DefinitionStages.WithRampDownStartVMOnConnect,
DefinitionStages.WithRampDownActionOnDisconnect, DefinitionStages.WithRampDownMinutesToWaitOnDisconnect,
DefinitionStages.WithRampDownActionOnLogoff, DefinitionStages.WithRampDownMinutesToWaitOnLogoff,
DefinitionStages.WithOffPeakStartTime, DefinitionStages.WithOffPeakStartVMOnConnect,
DefinitionStages.WithOffPeakActionOnDisconnect, DefinitionStages.WithOffPeakMinutesToWaitOnDisconnect,
DefinitionStages.WithOffPeakActionOnLogoff, DefinitionStages.WithOffPeakMinutesToWaitOnLogoff {
/**
* Executes the create request.
*
* @return the created resource.
*/
ScalingPlanPersonalSchedule create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
ScalingPlanPersonalSchedule create(Context context);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify daysOfWeek.
*/
interface WithDaysOfWeek {
/**
* Specifies the daysOfWeek property: Set of days of the week on which this schedule is active..
*
* @param daysOfWeek Set of days of the week on which this schedule is active.
* @return the next definition stage.
*/
WithCreate withDaysOfWeek(List daysOfWeek);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampUpStartTime.
*/
interface WithRampUpStartTime {
/**
* Specifies the rampUpStartTime property: Starting time for ramp up period..
*
* @param rampUpStartTime Starting time for ramp up period.
* @return the next definition stage.
*/
WithCreate withRampUpStartTime(Time rampUpStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampUpAutoStartHosts.
*/
interface WithRampUpAutoStartHosts {
/**
* Specifies the rampUpAutoStartHosts property: The desired startup behavior during the ramp up period for
* personal vms in the hostpool..
*
* @param rampUpAutoStartHosts The desired startup behavior during the ramp up period for personal vms in
* the hostpool.
* @return the next definition stage.
*/
WithCreate withRampUpAutoStartHosts(StartupBehavior rampUpAutoStartHosts);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampUpStartVMOnConnect.
*/
interface WithRampUpStartVMOnConnect {
/**
* Specifies the rampUpStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the ramp up phase. If this is disabled, session hosts must be turned on using
* rampUpAutoStartHosts or by turning them on manually..
*
* @param rampUpStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during
* the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by
* turning them on manually.
* @return the next definition stage.
*/
WithCreate withRampUpStartVMOnConnect(SetStartVMOnConnect rampUpStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampUpActionOnDisconnect.
*/
interface WithRampUpActionOnDisconnect {
/**
* Specifies the rampUpActionOnDisconnect property: Action to be taken after a user disconnect during the
* ramp up period..
*
* @param rampUpActionOnDisconnect Action to be taken after a user disconnect during the ramp up period.
* @return the next definition stage.
*/
WithCreate withRampUpActionOnDisconnect(SessionHandlingOperation rampUpActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampUpMinutesToWaitOnDisconnect.
*/
interface WithRampUpMinutesToWaitOnDisconnect {
/**
* Specifies the rampUpMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the
* desired session handling action when a user disconnects during the ramp up period..
*
* @param rampUpMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the ramp up period.
* @return the next definition stage.
*/
WithCreate withRampUpMinutesToWaitOnDisconnect(Integer rampUpMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampUpActionOnLogoff.
*/
interface WithRampUpActionOnLogoff {
/**
* Specifies the rampUpActionOnLogoff property: Action to be taken after a logoff during the ramp up
* period..
*
* @param rampUpActionOnLogoff Action to be taken after a logoff during the ramp up period.
* @return the next definition stage.
*/
WithCreate withRampUpActionOnLogoff(SessionHandlingOperation rampUpActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampUpMinutesToWaitOnLogoff.
*/
interface WithRampUpMinutesToWaitOnLogoff {
/**
* Specifies the rampUpMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the ramp up period..
*
* @param rampUpMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp up period.
* @return the next definition stage.
*/
WithCreate withRampUpMinutesToWaitOnLogoff(Integer rampUpMinutesToWaitOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify peakStartTime.
*/
interface WithPeakStartTime {
/**
* Specifies the peakStartTime property: Starting time for peak period..
*
* @param peakStartTime Starting time for peak period.
* @return the next definition stage.
*/
WithCreate withPeakStartTime(Time peakStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify peakStartVMOnConnect.
*/
interface WithPeakStartVMOnConnect {
/**
* Specifies the peakStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the peak phase..
*
* @param peakStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during the
* peak phase.
* @return the next definition stage.
*/
WithCreate withPeakStartVMOnConnect(SetStartVMOnConnect peakStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify peakActionOnDisconnect.
*/
interface WithPeakActionOnDisconnect {
/**
* Specifies the peakActionOnDisconnect property: Action to be taken after a user disconnect during the peak
* period..
*
* @param peakActionOnDisconnect Action to be taken after a user disconnect during the peak period.
* @return the next definition stage.
*/
WithCreate withPeakActionOnDisconnect(SessionHandlingOperation peakActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify peakMinutesToWaitOnDisconnect.
*/
interface WithPeakMinutesToWaitOnDisconnect {
/**
* Specifies the peakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the
* desired session handling action when a user disconnects during the peak period..
*
* @param peakMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the peak period.
* @return the next definition stage.
*/
WithCreate withPeakMinutesToWaitOnDisconnect(Integer peakMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify peakActionOnLogoff.
*/
interface WithPeakActionOnLogoff {
/**
* Specifies the peakActionOnLogoff property: Action to be taken after a logoff during the peak period..
*
* @param peakActionOnLogoff Action to be taken after a logoff during the peak period.
* @return the next definition stage.
*/
WithCreate withPeakActionOnLogoff(SessionHandlingOperation peakActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify peakMinutesToWaitOnLogoff.
*/
interface WithPeakMinutesToWaitOnLogoff {
/**
* Specifies the peakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the peak period..
*
* @param peakMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the peak period.
* @return the next definition stage.
*/
WithCreate withPeakMinutesToWaitOnLogoff(Integer peakMinutesToWaitOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampDownStartTime.
*/
interface WithRampDownStartTime {
/**
* Specifies the rampDownStartTime property: Starting time for ramp down period..
*
* @param rampDownStartTime Starting time for ramp down period.
* @return the next definition stage.
*/
WithCreate withRampDownStartTime(Time rampDownStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampDownStartVMOnConnect.
*/
interface WithRampDownStartVMOnConnect {
/**
* Specifies the rampDownStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the ramp down phase..
*
* @param rampDownStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during
* the ramp down phase.
* @return the next definition stage.
*/
WithCreate withRampDownStartVMOnConnect(SetStartVMOnConnect rampDownStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampDownActionOnDisconnect.
*/
interface WithRampDownActionOnDisconnect {
/**
* Specifies the rampDownActionOnDisconnect property: Action to be taken after a user disconnect during the
* ramp down period..
*
* @param rampDownActionOnDisconnect Action to be taken after a user disconnect during the ramp down period.
* @return the next definition stage.
*/
WithCreate withRampDownActionOnDisconnect(SessionHandlingOperation rampDownActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify
* rampDownMinutesToWaitOnDisconnect.
*/
interface WithRampDownMinutesToWaitOnDisconnect {
/**
* Specifies the rampDownMinutesToWaitOnDisconnect property: The time in minutes to wait before performing
* the desired session handling action when a user disconnects during the ramp down period..
*
* @param rampDownMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp down period.
* @return the next definition stage.
*/
WithCreate withRampDownMinutesToWaitOnDisconnect(Integer rampDownMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampDownActionOnLogoff.
*/
interface WithRampDownActionOnLogoff {
/**
* Specifies the rampDownActionOnLogoff property: Action to be taken after a logoff during the ramp down
* period..
*
* @param rampDownActionOnLogoff Action to be taken after a logoff during the ramp down period.
* @return the next definition stage.
*/
WithCreate withRampDownActionOnLogoff(SessionHandlingOperation rampDownActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify rampDownMinutesToWaitOnLogoff.
*/
interface WithRampDownMinutesToWaitOnLogoff {
/**
* Specifies the rampDownMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the ramp down period..
*
* @param rampDownMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp down period.
* @return the next definition stage.
*/
WithCreate withRampDownMinutesToWaitOnLogoff(Integer rampDownMinutesToWaitOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify offPeakStartTime.
*/
interface WithOffPeakStartTime {
/**
* Specifies the offPeakStartTime property: Starting time for off-peak period..
*
* @param offPeakStartTime Starting time for off-peak period.
* @return the next definition stage.
*/
WithCreate withOffPeakStartTime(Time offPeakStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify offPeakStartVMOnConnect.
*/
interface WithOffPeakStartVMOnConnect {
/**
* Specifies the offPeakStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the off-peak phase..
*
* @param offPeakStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during
* the off-peak phase.
* @return the next definition stage.
*/
WithCreate withOffPeakStartVMOnConnect(SetStartVMOnConnect offPeakStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify offPeakActionOnDisconnect.
*/
interface WithOffPeakActionOnDisconnect {
/**
* Specifies the offPeakActionOnDisconnect property: Action to be taken after a user disconnect during the
* off-peak period..
*
* @param offPeakActionOnDisconnect Action to be taken after a user disconnect during the off-peak period.
* @return the next definition stage.
*/
WithCreate withOffPeakActionOnDisconnect(SessionHandlingOperation offPeakActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify offPeakMinutesToWaitOnDisconnect.
*/
interface WithOffPeakMinutesToWaitOnDisconnect {
/**
* Specifies the offPeakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing
* the desired session handling action when a user disconnects during the off-peak period..
*
* @param offPeakMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the off-peak period.
* @return the next definition stage.
*/
WithCreate withOffPeakMinutesToWaitOnDisconnect(Integer offPeakMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify offPeakActionOnLogoff.
*/
interface WithOffPeakActionOnLogoff {
/**
* Specifies the offPeakActionOnLogoff property: Action to be taken after a logoff during the off-peak
* period..
*
* @param offPeakActionOnLogoff Action to be taken after a logoff during the off-peak period.
* @return the next definition stage.
*/
WithCreate withOffPeakActionOnLogoff(SessionHandlingOperation offPeakActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule definition allowing to specify offPeakMinutesToWaitOnLogoff.
*/
interface WithOffPeakMinutesToWaitOnLogoff {
/**
* Specifies the offPeakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the off-peak period..
*
* @param offPeakMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the off-peak period.
* @return the next definition stage.
*/
WithCreate withOffPeakMinutesToWaitOnLogoff(Integer offPeakMinutesToWaitOnLogoff);
}
}
/**
* Begins update for the ScalingPlanPersonalSchedule resource.
*
* @return the stage of resource update.
*/
ScalingPlanPersonalSchedule.Update update();
/**
* The template for ScalingPlanPersonalSchedule update.
*/
interface Update extends UpdateStages.WithDaysOfWeek, UpdateStages.WithRampUpStartTime,
UpdateStages.WithRampUpAutoStartHosts, UpdateStages.WithRampUpStartVMOnConnect,
UpdateStages.WithRampUpActionOnDisconnect, UpdateStages.WithRampUpMinutesToWaitOnDisconnect,
UpdateStages.WithRampUpActionOnLogoff, UpdateStages.WithRampUpMinutesToWaitOnLogoff,
UpdateStages.WithPeakStartTime, UpdateStages.WithPeakStartVMOnConnect, UpdateStages.WithPeakActionOnDisconnect,
UpdateStages.WithPeakMinutesToWaitOnDisconnect, UpdateStages.WithPeakActionOnLogoff,
UpdateStages.WithPeakMinutesToWaitOnLogoff, UpdateStages.WithRampDownStartTime,
UpdateStages.WithRampDownStartVMOnConnect, UpdateStages.WithRampDownActionOnDisconnect,
UpdateStages.WithRampDownMinutesToWaitOnDisconnect, UpdateStages.WithRampDownActionOnLogoff,
UpdateStages.WithRampDownMinutesToWaitOnLogoff, UpdateStages.WithOffPeakStartTime,
UpdateStages.WithOffPeakStartVMOnConnect, UpdateStages.WithOffPeakActionOnDisconnect,
UpdateStages.WithOffPeakMinutesToWaitOnDisconnect, UpdateStages.WithOffPeakActionOnLogoff,
UpdateStages.WithOffPeakMinutesToWaitOnLogoff {
/**
* Executes the update request.
*
* @return the updated resource.
*/
ScalingPlanPersonalSchedule apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
ScalingPlanPersonalSchedule apply(Context context);
}
/**
* The ScalingPlanPersonalSchedule update stages.
*/
interface UpdateStages {
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify daysOfWeek.
*/
interface WithDaysOfWeek {
/**
* Specifies the daysOfWeek property: Set of days of the week on which this schedule is active..
*
* @param daysOfWeek Set of days of the week on which this schedule is active.
* @return the next definition stage.
*/
Update withDaysOfWeek(List daysOfWeek);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampUpStartTime.
*/
interface WithRampUpStartTime {
/**
* Specifies the rampUpStartTime property: Starting time for ramp up period..
*
* @param rampUpStartTime Starting time for ramp up period.
* @return the next definition stage.
*/
Update withRampUpStartTime(Time rampUpStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampUpAutoStartHosts.
*/
interface WithRampUpAutoStartHosts {
/**
* Specifies the rampUpAutoStartHosts property: The desired startup behavior during the ramp up period for
* personal vms in the hostpool..
*
* @param rampUpAutoStartHosts The desired startup behavior during the ramp up period for personal vms in
* the hostpool.
* @return the next definition stage.
*/
Update withRampUpAutoStartHosts(StartupBehavior rampUpAutoStartHosts);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampUpStartVMOnConnect.
*/
interface WithRampUpStartVMOnConnect {
/**
* Specifies the rampUpStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the ramp up phase. If this is disabled, session hosts must be turned on using
* rampUpAutoStartHosts or by turning them on manually..
*
* @param rampUpStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during
* the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by
* turning them on manually.
* @return the next definition stage.
*/
Update withRampUpStartVMOnConnect(SetStartVMOnConnect rampUpStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampUpActionOnDisconnect.
*/
interface WithRampUpActionOnDisconnect {
/**
* Specifies the rampUpActionOnDisconnect property: Action to be taken after a user disconnect during the
* ramp up period..
*
* @param rampUpActionOnDisconnect Action to be taken after a user disconnect during the ramp up period.
* @return the next definition stage.
*/
Update withRampUpActionOnDisconnect(SessionHandlingOperation rampUpActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampUpMinutesToWaitOnDisconnect.
*/
interface WithRampUpMinutesToWaitOnDisconnect {
/**
* Specifies the rampUpMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the
* desired session handling action when a user disconnects during the ramp up period..
*
* @param rampUpMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the ramp up period.
* @return the next definition stage.
*/
Update withRampUpMinutesToWaitOnDisconnect(Integer rampUpMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampUpActionOnLogoff.
*/
interface WithRampUpActionOnLogoff {
/**
* Specifies the rampUpActionOnLogoff property: Action to be taken after a logoff during the ramp up
* period..
*
* @param rampUpActionOnLogoff Action to be taken after a logoff during the ramp up period.
* @return the next definition stage.
*/
Update withRampUpActionOnLogoff(SessionHandlingOperation rampUpActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampUpMinutesToWaitOnLogoff.
*/
interface WithRampUpMinutesToWaitOnLogoff {
/**
* Specifies the rampUpMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the ramp up period..
*
* @param rampUpMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp up period.
* @return the next definition stage.
*/
Update withRampUpMinutesToWaitOnLogoff(Integer rampUpMinutesToWaitOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify peakStartTime.
*/
interface WithPeakStartTime {
/**
* Specifies the peakStartTime property: Starting time for peak period..
*
* @param peakStartTime Starting time for peak period.
* @return the next definition stage.
*/
Update withPeakStartTime(Time peakStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify peakStartVMOnConnect.
*/
interface WithPeakStartVMOnConnect {
/**
* Specifies the peakStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the peak phase..
*
* @param peakStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during the
* peak phase.
* @return the next definition stage.
*/
Update withPeakStartVMOnConnect(SetStartVMOnConnect peakStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify peakActionOnDisconnect.
*/
interface WithPeakActionOnDisconnect {
/**
* Specifies the peakActionOnDisconnect property: Action to be taken after a user disconnect during the peak
* period..
*
* @param peakActionOnDisconnect Action to be taken after a user disconnect during the peak period.
* @return the next definition stage.
*/
Update withPeakActionOnDisconnect(SessionHandlingOperation peakActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify peakMinutesToWaitOnDisconnect.
*/
interface WithPeakMinutesToWaitOnDisconnect {
/**
* Specifies the peakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the
* desired session handling action when a user disconnects during the peak period..
*
* @param peakMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the peak period.
* @return the next definition stage.
*/
Update withPeakMinutesToWaitOnDisconnect(Integer peakMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify peakActionOnLogoff.
*/
interface WithPeakActionOnLogoff {
/**
* Specifies the peakActionOnLogoff property: Action to be taken after a logoff during the peak period..
*
* @param peakActionOnLogoff Action to be taken after a logoff during the peak period.
* @return the next definition stage.
*/
Update withPeakActionOnLogoff(SessionHandlingOperation peakActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify peakMinutesToWaitOnLogoff.
*/
interface WithPeakMinutesToWaitOnLogoff {
/**
* Specifies the peakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the peak period..
*
* @param peakMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the peak period.
* @return the next definition stage.
*/
Update withPeakMinutesToWaitOnLogoff(Integer peakMinutesToWaitOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampDownStartTime.
*/
interface WithRampDownStartTime {
/**
* Specifies the rampDownStartTime property: Starting time for ramp down period..
*
* @param rampDownStartTime Starting time for ramp down period.
* @return the next definition stage.
*/
Update withRampDownStartTime(Time rampDownStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampDownStartVMOnConnect.
*/
interface WithRampDownStartVMOnConnect {
/**
* Specifies the rampDownStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the ramp down phase..
*
* @param rampDownStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during
* the ramp down phase.
* @return the next definition stage.
*/
Update withRampDownStartVMOnConnect(SetStartVMOnConnect rampDownStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampDownActionOnDisconnect.
*/
interface WithRampDownActionOnDisconnect {
/**
* Specifies the rampDownActionOnDisconnect property: Action to be taken after a user disconnect during the
* ramp down period..
*
* @param rampDownActionOnDisconnect Action to be taken after a user disconnect during the ramp down period.
* @return the next definition stage.
*/
Update withRampDownActionOnDisconnect(SessionHandlingOperation rampDownActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampDownMinutesToWaitOnDisconnect.
*/
interface WithRampDownMinutesToWaitOnDisconnect {
/**
* Specifies the rampDownMinutesToWaitOnDisconnect property: The time in minutes to wait before performing
* the desired session handling action when a user disconnects during the ramp down period..
*
* @param rampDownMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp down period.
* @return the next definition stage.
*/
Update withRampDownMinutesToWaitOnDisconnect(Integer rampDownMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampDownActionOnLogoff.
*/
interface WithRampDownActionOnLogoff {
/**
* Specifies the rampDownActionOnLogoff property: Action to be taken after a logoff during the ramp down
* period..
*
* @param rampDownActionOnLogoff Action to be taken after a logoff during the ramp down period.
* @return the next definition stage.
*/
Update withRampDownActionOnLogoff(SessionHandlingOperation rampDownActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify rampDownMinutesToWaitOnLogoff.
*/
interface WithRampDownMinutesToWaitOnLogoff {
/**
* Specifies the rampDownMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the ramp down period..
*
* @param rampDownMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp down period.
* @return the next definition stage.
*/
Update withRampDownMinutesToWaitOnLogoff(Integer rampDownMinutesToWaitOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify offPeakStartTime.
*/
interface WithOffPeakStartTime {
/**
* Specifies the offPeakStartTime property: Starting time for off-peak period..
*
* @param offPeakStartTime Starting time for off-peak period.
* @return the next definition stage.
*/
Update withOffPeakStartTime(Time offPeakStartTime);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify offPeakStartVMOnConnect.
*/
interface WithOffPeakStartVMOnConnect {
/**
* Specifies the offPeakStartVMOnConnect property: The desired configuration of Start VM On Connect for the
* hostpool during the off-peak phase..
*
* @param offPeakStartVMOnConnect The desired configuration of Start VM On Connect for the hostpool during
* the off-peak phase.
* @return the next definition stage.
*/
Update withOffPeakStartVMOnConnect(SetStartVMOnConnect offPeakStartVMOnConnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify offPeakActionOnDisconnect.
*/
interface WithOffPeakActionOnDisconnect {
/**
* Specifies the offPeakActionOnDisconnect property: Action to be taken after a user disconnect during the
* off-peak period..
*
* @param offPeakActionOnDisconnect Action to be taken after a user disconnect during the off-peak period.
* @return the next definition stage.
*/
Update withOffPeakActionOnDisconnect(SessionHandlingOperation offPeakActionOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify offPeakMinutesToWaitOnDisconnect.
*/
interface WithOffPeakMinutesToWaitOnDisconnect {
/**
* Specifies the offPeakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing
* the desired session handling action when a user disconnects during the off-peak period..
*
* @param offPeakMinutesToWaitOnDisconnect The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the off-peak period.
* @return the next definition stage.
*/
Update withOffPeakMinutesToWaitOnDisconnect(Integer offPeakMinutesToWaitOnDisconnect);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify offPeakActionOnLogoff.
*/
interface WithOffPeakActionOnLogoff {
/**
* Specifies the offPeakActionOnLogoff property: Action to be taken after a logoff during the off-peak
* period..
*
* @param offPeakActionOnLogoff Action to be taken after a logoff during the off-peak period.
* @return the next definition stage.
*/
Update withOffPeakActionOnLogoff(SessionHandlingOperation offPeakActionOnLogoff);
}
/**
* The stage of the ScalingPlanPersonalSchedule update allowing to specify offPeakMinutesToWaitOnLogoff.
*/
interface WithOffPeakMinutesToWaitOnLogoff {
/**
* Specifies the offPeakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the
* desired session handling action when a user logs off during the off-peak period..
*
* @param offPeakMinutesToWaitOnLogoff The time in minutes to wait before performing the desired session
* handling action when a user logs off during the off-peak period.
* @return the next definition stage.
*/
Update withOffPeakMinutesToWaitOnLogoff(Integer offPeakMinutesToWaitOnLogoff);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
ScalingPlanPersonalSchedule refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
ScalingPlanPersonalSchedule refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy