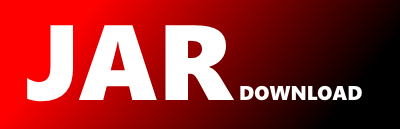
com.azure.resourcemanager.desktopvirtualization.models.ScalingPlanPersonalSchedulePatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPersonalScheduleProperties;
import java.io.IOException;
import java.util.List;
/**
* ScalingPlanPersonalSchedule properties that can be patched.
*/
@Fluent
public final class ScalingPlanPersonalSchedulePatch implements JsonSerializable {
/*
* Detailed properties for ScalingPlanPersonalSchedule
*/
private ScalingPlanPersonalScheduleProperties innerProperties;
/**
* Creates an instance of ScalingPlanPersonalSchedulePatch class.
*/
public ScalingPlanPersonalSchedulePatch() {
}
/**
* Get the innerProperties property: Detailed properties for ScalingPlanPersonalSchedule.
*
* @return the innerProperties value.
*/
private ScalingPlanPersonalScheduleProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @return the daysOfWeek value.
*/
public List daysOfWeek() {
return this.innerProperties() == null ? null : this.innerProperties().daysOfWeek();
}
/**
* Set the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @param daysOfWeek the daysOfWeek value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withDaysOfWeek(List daysOfWeek) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withDaysOfWeek(daysOfWeek);
return this;
}
/**
* Get the rampUpStartTime property: Starting time for ramp up period.
*
* @return the rampUpStartTime value.
*/
public Time rampUpStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpStartTime();
}
/**
* Set the rampUpStartTime property: Starting time for ramp up period.
*
* @param rampUpStartTime the rampUpStartTime value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampUpStartTime(Time rampUpStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampUpStartTime(rampUpStartTime);
return this;
}
/**
* Get the rampUpAutoStartHosts property: The desired startup behavior during the ramp up period for personal vms in
* the hostpool.
*
* @return the rampUpAutoStartHosts value.
*/
public StartupBehavior rampUpAutoStartHosts() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpAutoStartHosts();
}
/**
* Set the rampUpAutoStartHosts property: The desired startup behavior during the ramp up period for personal vms in
* the hostpool.
*
* @param rampUpAutoStartHosts the rampUpAutoStartHosts value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampUpAutoStartHosts(StartupBehavior rampUpAutoStartHosts) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampUpAutoStartHosts(rampUpAutoStartHosts);
return this;
}
/**
* Get the rampUpStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by turning
* them on manually.
*
* @return the rampUpStartVMOnConnect value.
*/
public SetStartVMOnConnect rampUpStartVMOnConnect() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpStartVMOnConnect();
}
/**
* Set the rampUpStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the ramp up phase. If this is disabled, session hosts must be turned on using rampUpAutoStartHosts or by turning
* them on manually.
*
* @param rampUpStartVMOnConnect the rampUpStartVMOnConnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampUpStartVMOnConnect(SetStartVMOnConnect rampUpStartVMOnConnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampUpStartVMOnConnect(rampUpStartVMOnConnect);
return this;
}
/**
* Get the rampUpActionOnDisconnect property: Action to be taken after a user disconnect during the ramp up period.
*
* @return the rampUpActionOnDisconnect value.
*/
public SessionHandlingOperation rampUpActionOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpActionOnDisconnect();
}
/**
* Set the rampUpActionOnDisconnect property: Action to be taken after a user disconnect during the ramp up period.
*
* @param rampUpActionOnDisconnect the rampUpActionOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withRampUpActionOnDisconnect(SessionHandlingOperation rampUpActionOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampUpActionOnDisconnect(rampUpActionOnDisconnect);
return this;
}
/**
* Get the rampUpMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp up period.
*
* @return the rampUpMinutesToWaitOnDisconnect value.
*/
public Integer rampUpMinutesToWaitOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpMinutesToWaitOnDisconnect();
}
/**
* Set the rampUpMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp up period.
*
* @param rampUpMinutesToWaitOnDisconnect the rampUpMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withRampUpMinutesToWaitOnDisconnect(Integer rampUpMinutesToWaitOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampUpMinutesToWaitOnDisconnect(rampUpMinutesToWaitOnDisconnect);
return this;
}
/**
* Get the rampUpActionOnLogoff property: Action to be taken after a logoff during the ramp up period.
*
* @return the rampUpActionOnLogoff value.
*/
public SessionHandlingOperation rampUpActionOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpActionOnLogoff();
}
/**
* Set the rampUpActionOnLogoff property: Action to be taken after a logoff during the ramp up period.
*
* @param rampUpActionOnLogoff the rampUpActionOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampUpActionOnLogoff(SessionHandlingOperation rampUpActionOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampUpActionOnLogoff(rampUpActionOnLogoff);
return this;
}
/**
* Get the rampUpMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp up period.
*
* @return the rampUpMinutesToWaitOnLogoff value.
*/
public Integer rampUpMinutesToWaitOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpMinutesToWaitOnLogoff();
}
/**
* Set the rampUpMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp up period.
*
* @param rampUpMinutesToWaitOnLogoff the rampUpMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampUpMinutesToWaitOnLogoff(Integer rampUpMinutesToWaitOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampUpMinutesToWaitOnLogoff(rampUpMinutesToWaitOnLogoff);
return this;
}
/**
* Get the peakStartTime property: Starting time for peak period.
*
* @return the peakStartTime value.
*/
public Time peakStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().peakStartTime();
}
/**
* Set the peakStartTime property: Starting time for peak period.
*
* @param peakStartTime the peakStartTime value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withPeakStartTime(Time peakStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withPeakStartTime(peakStartTime);
return this;
}
/**
* Get the peakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the peak phase.
*
* @return the peakStartVMOnConnect value.
*/
public SetStartVMOnConnect peakStartVMOnConnect() {
return this.innerProperties() == null ? null : this.innerProperties().peakStartVMOnConnect();
}
/**
* Set the peakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool during
* the peak phase.
*
* @param peakStartVMOnConnect the peakStartVMOnConnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withPeakStartVMOnConnect(SetStartVMOnConnect peakStartVMOnConnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withPeakStartVMOnConnect(peakStartVMOnConnect);
return this;
}
/**
* Get the peakActionOnDisconnect property: Action to be taken after a user disconnect during the peak period.
*
* @return the peakActionOnDisconnect value.
*/
public SessionHandlingOperation peakActionOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().peakActionOnDisconnect();
}
/**
* Set the peakActionOnDisconnect property: Action to be taken after a user disconnect during the peak period.
*
* @param peakActionOnDisconnect the peakActionOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withPeakActionOnDisconnect(SessionHandlingOperation peakActionOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withPeakActionOnDisconnect(peakActionOnDisconnect);
return this;
}
/**
* Get the peakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the peak period.
*
* @return the peakMinutesToWaitOnDisconnect value.
*/
public Integer peakMinutesToWaitOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().peakMinutesToWaitOnDisconnect();
}
/**
* Set the peakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired session
* handling action when a user disconnects during the peak period.
*
* @param peakMinutesToWaitOnDisconnect the peakMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withPeakMinutesToWaitOnDisconnect(Integer peakMinutesToWaitOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withPeakMinutesToWaitOnDisconnect(peakMinutesToWaitOnDisconnect);
return this;
}
/**
* Get the peakActionOnLogoff property: Action to be taken after a logoff during the peak period.
*
* @return the peakActionOnLogoff value.
*/
public SessionHandlingOperation peakActionOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().peakActionOnLogoff();
}
/**
* Set the peakActionOnLogoff property: Action to be taken after a logoff during the peak period.
*
* @param peakActionOnLogoff the peakActionOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withPeakActionOnLogoff(SessionHandlingOperation peakActionOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withPeakActionOnLogoff(peakActionOnLogoff);
return this;
}
/**
* Get the peakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the peak period.
*
* @return the peakMinutesToWaitOnLogoff value.
*/
public Integer peakMinutesToWaitOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().peakMinutesToWaitOnLogoff();
}
/**
* Set the peakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the peak period.
*
* @param peakMinutesToWaitOnLogoff the peakMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withPeakMinutesToWaitOnLogoff(Integer peakMinutesToWaitOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withPeakMinutesToWaitOnLogoff(peakMinutesToWaitOnLogoff);
return this;
}
/**
* Get the rampDownStartTime property: Starting time for ramp down period.
*
* @return the rampDownStartTime value.
*/
public Time rampDownStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownStartTime();
}
/**
* Set the rampDownStartTime property: Starting time for ramp down period.
*
* @param rampDownStartTime the rampDownStartTime value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampDownStartTime(Time rampDownStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampDownStartTime(rampDownStartTime);
return this;
}
/**
* Get the rampDownStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the ramp down phase.
*
* @return the rampDownStartVMOnConnect value.
*/
public SetStartVMOnConnect rampDownStartVMOnConnect() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownStartVMOnConnect();
}
/**
* Set the rampDownStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the ramp down phase.
*
* @param rampDownStartVMOnConnect the rampDownStartVMOnConnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampDownStartVMOnConnect(SetStartVMOnConnect rampDownStartVMOnConnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampDownStartVMOnConnect(rampDownStartVMOnConnect);
return this;
}
/**
* Get the rampDownActionOnDisconnect property: Action to be taken after a user disconnect during the ramp down
* period.
*
* @return the rampDownActionOnDisconnect value.
*/
public SessionHandlingOperation rampDownActionOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownActionOnDisconnect();
}
/**
* Set the rampDownActionOnDisconnect property: Action to be taken after a user disconnect during the ramp down
* period.
*
* @param rampDownActionOnDisconnect the rampDownActionOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withRampDownActionOnDisconnect(SessionHandlingOperation rampDownActionOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampDownActionOnDisconnect(rampDownActionOnDisconnect);
return this;
}
/**
* Get the rampDownMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp down period.
*
* @return the rampDownMinutesToWaitOnDisconnect value.
*/
public Integer rampDownMinutesToWaitOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownMinutesToWaitOnDisconnect();
}
/**
* Set the rampDownMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the ramp down period.
*
* @param rampDownMinutesToWaitOnDisconnect the rampDownMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withRampDownMinutesToWaitOnDisconnect(Integer rampDownMinutesToWaitOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampDownMinutesToWaitOnDisconnect(rampDownMinutesToWaitOnDisconnect);
return this;
}
/**
* Get the rampDownActionOnLogoff property: Action to be taken after a logoff during the ramp down period.
*
* @return the rampDownActionOnLogoff value.
*/
public SessionHandlingOperation rampDownActionOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownActionOnLogoff();
}
/**
* Set the rampDownActionOnLogoff property: Action to be taken after a logoff during the ramp down period.
*
* @param rampDownActionOnLogoff the rampDownActionOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withRampDownActionOnLogoff(SessionHandlingOperation rampDownActionOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampDownActionOnLogoff(rampDownActionOnLogoff);
return this;
}
/**
* Get the rampDownMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp down period.
*
* @return the rampDownMinutesToWaitOnLogoff value.
*/
public Integer rampDownMinutesToWaitOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownMinutesToWaitOnLogoff();
}
/**
* Set the rampDownMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the ramp down period.
*
* @param rampDownMinutesToWaitOnLogoff the rampDownMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withRampDownMinutesToWaitOnLogoff(Integer rampDownMinutesToWaitOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withRampDownMinutesToWaitOnLogoff(rampDownMinutesToWaitOnLogoff);
return this;
}
/**
* Get the offPeakStartTime property: Starting time for off-peak period.
*
* @return the offPeakStartTime value.
*/
public Time offPeakStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakStartTime();
}
/**
* Set the offPeakStartTime property: Starting time for off-peak period.
*
* @param offPeakStartTime the offPeakStartTime value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withOffPeakStartTime(Time offPeakStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withOffPeakStartTime(offPeakStartTime);
return this;
}
/**
* Get the offPeakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the off-peak phase.
*
* @return the offPeakStartVMOnConnect value.
*/
public SetStartVMOnConnect offPeakStartVMOnConnect() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakStartVMOnConnect();
}
/**
* Set the offPeakStartVMOnConnect property: The desired configuration of Start VM On Connect for the hostpool
* during the off-peak phase.
*
* @param offPeakStartVMOnConnect the offPeakStartVMOnConnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withOffPeakStartVMOnConnect(SetStartVMOnConnect offPeakStartVMOnConnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withOffPeakStartVMOnConnect(offPeakStartVMOnConnect);
return this;
}
/**
* Get the offPeakActionOnDisconnect property: Action to be taken after a user disconnect during the off-peak
* period.
*
* @return the offPeakActionOnDisconnect value.
*/
public SessionHandlingOperation offPeakActionOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakActionOnDisconnect();
}
/**
* Set the offPeakActionOnDisconnect property: Action to be taken after a user disconnect during the off-peak
* period.
*
* @param offPeakActionOnDisconnect the offPeakActionOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withOffPeakActionOnDisconnect(SessionHandlingOperation offPeakActionOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withOffPeakActionOnDisconnect(offPeakActionOnDisconnect);
return this;
}
/**
* Get the offPeakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the off-peak period.
*
* @return the offPeakMinutesToWaitOnDisconnect value.
*/
public Integer offPeakMinutesToWaitOnDisconnect() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakMinutesToWaitOnDisconnect();
}
/**
* Set the offPeakMinutesToWaitOnDisconnect property: The time in minutes to wait before performing the desired
* session handling action when a user disconnects during the off-peak period.
*
* @param offPeakMinutesToWaitOnDisconnect the offPeakMinutesToWaitOnDisconnect value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch
withOffPeakMinutesToWaitOnDisconnect(Integer offPeakMinutesToWaitOnDisconnect) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withOffPeakMinutesToWaitOnDisconnect(offPeakMinutesToWaitOnDisconnect);
return this;
}
/**
* Get the offPeakActionOnLogoff property: Action to be taken after a logoff during the off-peak period.
*
* @return the offPeakActionOnLogoff value.
*/
public SessionHandlingOperation offPeakActionOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakActionOnLogoff();
}
/**
* Set the offPeakActionOnLogoff property: Action to be taken after a logoff during the off-peak period.
*
* @param offPeakActionOnLogoff the offPeakActionOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withOffPeakActionOnLogoff(SessionHandlingOperation offPeakActionOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withOffPeakActionOnLogoff(offPeakActionOnLogoff);
return this;
}
/**
* Get the offPeakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the off-peak period.
*
* @return the offPeakMinutesToWaitOnLogoff value.
*/
public Integer offPeakMinutesToWaitOnLogoff() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakMinutesToWaitOnLogoff();
}
/**
* Set the offPeakMinutesToWaitOnLogoff property: The time in minutes to wait before performing the desired session
* handling action when a user logs off during the off-peak period.
*
* @param offPeakMinutesToWaitOnLogoff the offPeakMinutesToWaitOnLogoff value to set.
* @return the ScalingPlanPersonalSchedulePatch object itself.
*/
public ScalingPlanPersonalSchedulePatch withOffPeakMinutesToWaitOnLogoff(Integer offPeakMinutesToWaitOnLogoff) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPersonalScheduleProperties();
}
this.innerProperties().withOffPeakMinutesToWaitOnLogoff(offPeakMinutesToWaitOnLogoff);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("properties", this.innerProperties);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScalingPlanPersonalSchedulePatch from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScalingPlanPersonalSchedulePatch if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ScalingPlanPersonalSchedulePatch.
*/
public static ScalingPlanPersonalSchedulePatch fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScalingPlanPersonalSchedulePatch deserializedScalingPlanPersonalSchedulePatch
= new ScalingPlanPersonalSchedulePatch();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("properties".equals(fieldName)) {
deserializedScalingPlanPersonalSchedulePatch.innerProperties
= ScalingPlanPersonalScheduleProperties.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedScalingPlanPersonalSchedulePatch;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy