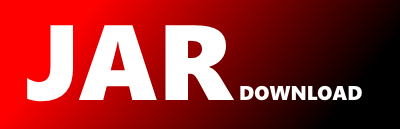
com.azure.resourcemanager.desktopvirtualization.models.ScalingPlanPooledSchedulePatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
Show all versions of azure-resourcemanager-desktopvirtualization Show documentation
This package contains Microsoft Azure SDK for DesktopVirtualization Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-04.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.desktopvirtualization.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.ProxyResource;
import com.azure.core.management.SystemData;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.desktopvirtualization.fluent.models.ScalingPlanPooledScheduleProperties;
import java.io.IOException;
import java.util.List;
/**
* ScalingPlanPooledSchedule properties that can be patched.
*/
@Fluent
public final class ScalingPlanPooledSchedulePatch extends ProxyResource {
/*
* Detailed properties for ScalingPlanPooledSchedule
*/
private ScalingPlanPooledScheduleProperties innerProperties;
/*
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*/
private SystemData systemData;
/*
* The type of the resource.
*/
private String type;
/*
* The name of the resource.
*/
private String name;
/*
* Fully qualified resource Id for the resource.
*/
private String id;
/**
* Creates an instance of ScalingPlanPooledSchedulePatch class.
*/
public ScalingPlanPooledSchedulePatch() {
}
/**
* Get the innerProperties property: Detailed properties for ScalingPlanPooledSchedule.
*
* @return the innerProperties value.
*/
private ScalingPlanPooledScheduleProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* Get the type property: The type of the resource.
*
* @return the type value.
*/
@Override
public String type() {
return this.type;
}
/**
* Get the name property: The name of the resource.
*
* @return the name value.
*/
@Override
public String name() {
return this.name;
}
/**
* Get the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
@Override
public String id() {
return this.id;
}
/**
* Get the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @return the daysOfWeek value.
*/
public List daysOfWeek() {
return this.innerProperties() == null ? null : this.innerProperties().daysOfWeek();
}
/**
* Set the daysOfWeek property: Set of days of the week on which this schedule is active.
*
* @param daysOfWeek the daysOfWeek value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withDaysOfWeek(List daysOfWeek) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withDaysOfWeek(daysOfWeek);
return this;
}
/**
* Get the rampUpStartTime property: Starting time for ramp up period.
*
* @return the rampUpStartTime value.
*/
public Time rampUpStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpStartTime();
}
/**
* Set the rampUpStartTime property: Starting time for ramp up period.
*
* @param rampUpStartTime the rampUpStartTime value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampUpStartTime(Time rampUpStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampUpStartTime(rampUpStartTime);
return this;
}
/**
* Get the rampUpLoadBalancingAlgorithm property: Load balancing algorithm for ramp up period.
*
* @return the rampUpLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm rampUpLoadBalancingAlgorithm() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpLoadBalancingAlgorithm();
}
/**
* Set the rampUpLoadBalancingAlgorithm property: Load balancing algorithm for ramp up period.
*
* @param rampUpLoadBalancingAlgorithm the rampUpLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch
withRampUpLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm rampUpLoadBalancingAlgorithm) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampUpLoadBalancingAlgorithm(rampUpLoadBalancingAlgorithm);
return this;
}
/**
* Get the rampUpMinimumHostsPct property: Minimum host percentage for ramp up period.
*
* @return the rampUpMinimumHostsPct value.
*/
public Integer rampUpMinimumHostsPct() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpMinimumHostsPct();
}
/**
* Set the rampUpMinimumHostsPct property: Minimum host percentage for ramp up period.
*
* @param rampUpMinimumHostsPct the rampUpMinimumHostsPct value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampUpMinimumHostsPct(Integer rampUpMinimumHostsPct) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampUpMinimumHostsPct(rampUpMinimumHostsPct);
return this;
}
/**
* Get the rampUpCapacityThresholdPct property: Capacity threshold for ramp up period.
*
* @return the rampUpCapacityThresholdPct value.
*/
public Integer rampUpCapacityThresholdPct() {
return this.innerProperties() == null ? null : this.innerProperties().rampUpCapacityThresholdPct();
}
/**
* Set the rampUpCapacityThresholdPct property: Capacity threshold for ramp up period.
*
* @param rampUpCapacityThresholdPct the rampUpCapacityThresholdPct value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampUpCapacityThresholdPct(Integer rampUpCapacityThresholdPct) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampUpCapacityThresholdPct(rampUpCapacityThresholdPct);
return this;
}
/**
* Get the peakStartTime property: Starting time for peak period.
*
* @return the peakStartTime value.
*/
public Time peakStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().peakStartTime();
}
/**
* Set the peakStartTime property: Starting time for peak period.
*
* @param peakStartTime the peakStartTime value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withPeakStartTime(Time peakStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withPeakStartTime(peakStartTime);
return this;
}
/**
* Get the peakLoadBalancingAlgorithm property: Load balancing algorithm for peak period.
*
* @return the peakLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm peakLoadBalancingAlgorithm() {
return this.innerProperties() == null ? null : this.innerProperties().peakLoadBalancingAlgorithm();
}
/**
* Set the peakLoadBalancingAlgorithm property: Load balancing algorithm for peak period.
*
* @param peakLoadBalancingAlgorithm the peakLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch
withPeakLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm peakLoadBalancingAlgorithm) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withPeakLoadBalancingAlgorithm(peakLoadBalancingAlgorithm);
return this;
}
/**
* Get the rampDownStartTime property: Starting time for ramp down period.
*
* @return the rampDownStartTime value.
*/
public Time rampDownStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownStartTime();
}
/**
* Set the rampDownStartTime property: Starting time for ramp down period.
*
* @param rampDownStartTime the rampDownStartTime value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampDownStartTime(Time rampDownStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownStartTime(rampDownStartTime);
return this;
}
/**
* Get the rampDownLoadBalancingAlgorithm property: Load balancing algorithm for ramp down period.
*
* @return the rampDownLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm rampDownLoadBalancingAlgorithm() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownLoadBalancingAlgorithm();
}
/**
* Set the rampDownLoadBalancingAlgorithm property: Load balancing algorithm for ramp down period.
*
* @param rampDownLoadBalancingAlgorithm the rampDownLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch
withRampDownLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm rampDownLoadBalancingAlgorithm) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownLoadBalancingAlgorithm(rampDownLoadBalancingAlgorithm);
return this;
}
/**
* Get the rampDownMinimumHostsPct property: Minimum host percentage for ramp down period.
*
* @return the rampDownMinimumHostsPct value.
*/
public Integer rampDownMinimumHostsPct() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownMinimumHostsPct();
}
/**
* Set the rampDownMinimumHostsPct property: Minimum host percentage for ramp down period.
*
* @param rampDownMinimumHostsPct the rampDownMinimumHostsPct value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampDownMinimumHostsPct(Integer rampDownMinimumHostsPct) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownMinimumHostsPct(rampDownMinimumHostsPct);
return this;
}
/**
* Get the rampDownCapacityThresholdPct property: Capacity threshold for ramp down period.
*
* @return the rampDownCapacityThresholdPct value.
*/
public Integer rampDownCapacityThresholdPct() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownCapacityThresholdPct();
}
/**
* Set the rampDownCapacityThresholdPct property: Capacity threshold for ramp down period.
*
* @param rampDownCapacityThresholdPct the rampDownCapacityThresholdPct value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampDownCapacityThresholdPct(Integer rampDownCapacityThresholdPct) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownCapacityThresholdPct(rampDownCapacityThresholdPct);
return this;
}
/**
* Get the rampDownForceLogoffUsers property: Should users be logged off forcefully from hosts.
*
* @return the rampDownForceLogoffUsers value.
*/
public Boolean rampDownForceLogoffUsers() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownForceLogoffUsers();
}
/**
* Set the rampDownForceLogoffUsers property: Should users be logged off forcefully from hosts.
*
* @param rampDownForceLogoffUsers the rampDownForceLogoffUsers value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampDownForceLogoffUsers(Boolean rampDownForceLogoffUsers) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownForceLogoffUsers(rampDownForceLogoffUsers);
return this;
}
/**
* Get the rampDownStopHostsWhen property: Specifies when to stop hosts during ramp down period.
*
* @return the rampDownStopHostsWhen value.
*/
public StopHostsWhen rampDownStopHostsWhen() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownStopHostsWhen();
}
/**
* Set the rampDownStopHostsWhen property: Specifies when to stop hosts during ramp down period.
*
* @param rampDownStopHostsWhen the rampDownStopHostsWhen value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampDownStopHostsWhen(StopHostsWhen rampDownStopHostsWhen) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownStopHostsWhen(rampDownStopHostsWhen);
return this;
}
/**
* Get the rampDownWaitTimeMinutes property: Number of minutes to wait to stop hosts during ramp down period.
*
* @return the rampDownWaitTimeMinutes value.
*/
public Integer rampDownWaitTimeMinutes() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownWaitTimeMinutes();
}
/**
* Set the rampDownWaitTimeMinutes property: Number of minutes to wait to stop hosts during ramp down period.
*
* @param rampDownWaitTimeMinutes the rampDownWaitTimeMinutes value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampDownWaitTimeMinutes(Integer rampDownWaitTimeMinutes) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownWaitTimeMinutes(rampDownWaitTimeMinutes);
return this;
}
/**
* Get the rampDownNotificationMessage property: Notification message for users during ramp down period.
*
* @return the rampDownNotificationMessage value.
*/
public String rampDownNotificationMessage() {
return this.innerProperties() == null ? null : this.innerProperties().rampDownNotificationMessage();
}
/**
* Set the rampDownNotificationMessage property: Notification message for users during ramp down period.
*
* @param rampDownNotificationMessage the rampDownNotificationMessage value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withRampDownNotificationMessage(String rampDownNotificationMessage) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withRampDownNotificationMessage(rampDownNotificationMessage);
return this;
}
/**
* Get the offPeakStartTime property: Starting time for off-peak period.
*
* @return the offPeakStartTime value.
*/
public Time offPeakStartTime() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakStartTime();
}
/**
* Set the offPeakStartTime property: Starting time for off-peak period.
*
* @param offPeakStartTime the offPeakStartTime value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch withOffPeakStartTime(Time offPeakStartTime) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withOffPeakStartTime(offPeakStartTime);
return this;
}
/**
* Get the offPeakLoadBalancingAlgorithm property: Load balancing algorithm for off-peak period.
*
* @return the offPeakLoadBalancingAlgorithm value.
*/
public SessionHostLoadBalancingAlgorithm offPeakLoadBalancingAlgorithm() {
return this.innerProperties() == null ? null : this.innerProperties().offPeakLoadBalancingAlgorithm();
}
/**
* Set the offPeakLoadBalancingAlgorithm property: Load balancing algorithm for off-peak period.
*
* @param offPeakLoadBalancingAlgorithm the offPeakLoadBalancingAlgorithm value to set.
* @return the ScalingPlanPooledSchedulePatch object itself.
*/
public ScalingPlanPooledSchedulePatch
withOffPeakLoadBalancingAlgorithm(SessionHostLoadBalancingAlgorithm offPeakLoadBalancingAlgorithm) {
if (this.innerProperties() == null) {
this.innerProperties = new ScalingPlanPooledScheduleProperties();
}
this.innerProperties().withOffPeakLoadBalancingAlgorithm(offPeakLoadBalancingAlgorithm);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("properties", this.innerProperties);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScalingPlanPooledSchedulePatch from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScalingPlanPooledSchedulePatch if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ScalingPlanPooledSchedulePatch.
*/
public static ScalingPlanPooledSchedulePatch fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScalingPlanPooledSchedulePatch deserializedScalingPlanPooledSchedulePatch
= new ScalingPlanPooledSchedulePatch();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedScalingPlanPooledSchedulePatch.id = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedScalingPlanPooledSchedulePatch.name = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedScalingPlanPooledSchedulePatch.type = reader.getString();
} else if ("properties".equals(fieldName)) {
deserializedScalingPlanPooledSchedulePatch.innerProperties
= ScalingPlanPooledScheduleProperties.fromJson(reader);
} else if ("systemData".equals(fieldName)) {
deserializedScalingPlanPooledSchedulePatch.systemData = SystemData.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedScalingPlanPooledSchedulePatch;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy