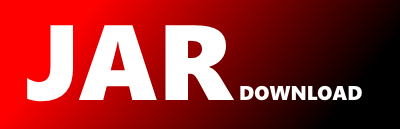
com.azure.resourcemanager.dynatrace.implementation.MonitorsClientImpl Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.dynatrace.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.Delete;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Headers;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.Patch;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.Put;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.dynatrace.fluent.MonitorsClient;
import com.azure.resourcemanager.dynatrace.fluent.models.AppServiceInfoInner;
import com.azure.resourcemanager.dynatrace.fluent.models.LinkableEnvironmentResponseInner;
import com.azure.resourcemanager.dynatrace.fluent.models.MarketplaceSaaSResourceDetailsResponseInner;
import com.azure.resourcemanager.dynatrace.fluent.models.MetricsStatusResponseInner;
import com.azure.resourcemanager.dynatrace.fluent.models.MonitorResourceInner;
import com.azure.resourcemanager.dynatrace.fluent.models.MonitoredResourceInner;
import com.azure.resourcemanager.dynatrace.fluent.models.SsoDetailsResponseInner;
import com.azure.resourcemanager.dynatrace.fluent.models.VMExtensionPayloadInner;
import com.azure.resourcemanager.dynatrace.fluent.models.VMInfoInner;
import com.azure.resourcemanager.dynatrace.models.AppServiceListResponse;
import com.azure.resourcemanager.dynatrace.models.LinkableEnvironmentListResponse;
import com.azure.resourcemanager.dynatrace.models.LinkableEnvironmentRequest;
import com.azure.resourcemanager.dynatrace.models.MarketplaceSaaSResourceDetailsRequest;
import com.azure.resourcemanager.dynatrace.models.MonitorResourceListResult;
import com.azure.resourcemanager.dynatrace.models.MonitorResourceUpdate;
import com.azure.resourcemanager.dynatrace.models.MonitoredResourceListResponse;
import com.azure.resourcemanager.dynatrace.models.SsoDetailsRequest;
import com.azure.resourcemanager.dynatrace.models.VMHostsListResponse;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in MonitorsClient.
*/
public final class MonitorsClientImpl implements MonitorsClient {
/**
* The proxy service used to perform REST calls.
*/
private final MonitorsService service;
/**
* The service client containing this operation class.
*/
private final DynatraceObservabilityImpl client;
/**
* Initializes an instance of MonitorsClientImpl.
*
* @param client the instance of the service client containing this operation class.
*/
MonitorsClientImpl(DynatraceObservabilityImpl client) {
this.service = RestProxy.create(MonitorsService.class, client.getHttpPipeline(), client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for DynatraceObservabilityMonitors to be used by the proxy service to
* perform REST calls.
*/
@Host("{$host}")
@ServiceInterface(name = "DynatraceObservabili")
public interface MonitorsService {
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}/listMonitoredResources")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listMonitoredResources(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}/getVMHostPayload")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getVMHostPayload(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getByResourceGroup(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}")
@ExpectedResponses({ 200, 201 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createOrUpdate(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@BodyParam("application/json") MonitorResourceInner resource, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Patch("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> update(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@BodyParam("application/json") MonitorResourceUpdate resource, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}")
@ExpectedResponses({ 200, 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> delete(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/providers/Dynatrace.Observability/monitors")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> list(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByResourceGroup(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/providers/Dynatrace.Observability/getMarketplaceSaaSResourceDetails")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ManagementException.class, code = { 404 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMarketplaceSaaSResourceDetails(
@HostParam("$host") String endpoint, @QueryParam("api-version") String apiVersion,
@PathParam("subscriptionId") String subscriptionId,
@BodyParam("application/json") MarketplaceSaaSResourceDetailsRequest request,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}/listHosts")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listHosts(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}/getMetricStatus")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMetricStatus(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}/listAppServices")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listAppServices(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}/getSSODetails")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ManagementException.class, code = { 401 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getSsoDetails(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@BodyParam("application/json") SsoDetailsRequest request, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Dynatrace.Observability/monitors/{monitorName}/listLinkableEnvironments")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listLinkableEnvironments(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("monitorName") String monitorName,
@BodyParam("application/json") LinkableEnvironmentRequest request, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listMonitoredResourcesNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listBySubscriptionIdNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByResourceGroupNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listHostsNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listAppServicesNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listLinkableEnvironmentsNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
}
/**
* List the resources currently being monitored by the Dynatrace monitor resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource along with {@link PagedResponse}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listMonitoredResourcesSinglePageAsync(String resourceGroupName,
String monitorName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listMonitoredResources(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* List the resources currently being monitored by the Dynatrace monitor resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource along with {@link PagedResponse}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listMonitoredResourcesSinglePageAsync(String resourceGroupName,
String monitorName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listMonitoredResources(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* List the resources currently being monitored by the Dynatrace monitor resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listMonitoredResourcesAsync(String resourceGroupName,
String monitorName) {
return new PagedFlux<>(() -> listMonitoredResourcesSinglePageAsync(resourceGroupName, monitorName),
nextLink -> listMonitoredResourcesNextSinglePageAsync(nextLink));
}
/**
* List the resources currently being monitored by the Dynatrace monitor resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listMonitoredResourcesAsync(String resourceGroupName, String monitorName,
Context context) {
return new PagedFlux<>(() -> listMonitoredResourcesSinglePageAsync(resourceGroupName, monitorName, context),
nextLink -> listMonitoredResourcesNextSinglePageAsync(nextLink, context));
}
/**
* List the resources currently being monitored by the Dynatrace monitor resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMonitoredResources(String resourceGroupName, String monitorName) {
return new PagedIterable<>(listMonitoredResourcesAsync(resourceGroupName, monitorName));
}
/**
* List the resources currently being monitored by the Dynatrace monitor resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMonitoredResources(String resourceGroupName, String monitorName,
Context context) {
return new PagedIterable<>(listMonitoredResourcesAsync(resourceGroupName, monitorName, context));
}
/**
* Returns the payload that needs to be passed in the request body for installing Dynatrace agent on a VM.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of payload to be passed while installing VM agent along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getVMHostPayloadWithResponseAsync(String resourceGroupName,
String monitorName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getVMHostPayload(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Returns the payload that needs to be passed in the request body for installing Dynatrace agent on a VM.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of payload to be passed while installing VM agent along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getVMHostPayloadWithResponseAsync(String resourceGroupName,
String monitorName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getVMHostPayload(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context);
}
/**
* Returns the payload that needs to be passed in the request body for installing Dynatrace agent on a VM.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of payload to be passed while installing VM agent on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getVMHostPayloadAsync(String resourceGroupName, String monitorName) {
return getVMHostPayloadWithResponseAsync(resourceGroupName, monitorName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Returns the payload that needs to be passed in the request body for installing Dynatrace agent on a VM.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of payload to be passed while installing VM agent along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getVMHostPayloadWithResponse(String resourceGroupName, String monitorName,
Context context) {
return getVMHostPayloadWithResponseAsync(resourceGroupName, monitorName, context).block();
}
/**
* Returns the payload that needs to be passed in the request body for installing Dynatrace agent on a VM.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of payload to be passed while installing VM agent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VMExtensionPayloadInner getVMHostPayload(String resourceGroupName, String monitorName) {
return getVMHostPayloadWithResponse(resourceGroupName, monitorName, Context.NONE).getValue();
}
/**
* Get a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a MonitorResource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getByResourceGroupWithResponseAsync(String resourceGroupName,
String monitorName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getByResourceGroup(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a MonitorResource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getByResourceGroupWithResponseAsync(String resourceGroupName,
String monitorName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getByResourceGroup(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context);
}
/**
* Get a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a MonitorResource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getByResourceGroupAsync(String resourceGroupName, String monitorName) {
return getByResourceGroupWithResponseAsync(resourceGroupName, monitorName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Get a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a MonitorResource along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getByResourceGroupWithResponse(String resourceGroupName, String monitorName,
Context context) {
return getByResourceGroupWithResponseAsync(resourceGroupName, monitorName, context).block();
}
/**
* Get a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a MonitorResource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MonitorResourceInner getByResourceGroup(String resourceGroupName, String monitorName) {
return getByResourceGroupWithResponse(resourceGroupName, monitorName, Context.NONE).getValue();
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateWithResponseAsync(String resourceGroupName,
String monitorName, MonitorResourceInner resource) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (resource == null) {
return Mono.error(new IllegalArgumentException("Parameter resource is required and cannot be null."));
} else {
resource.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createOrUpdate(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, resource, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateWithResponseAsync(String resourceGroupName,
String monitorName, MonitorResourceInner resource, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (resource == null) {
return Mono.error(new IllegalArgumentException("Parameter resource is required and cannot be null."));
} else {
resource.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createOrUpdate(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, resource, accept, context);
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of dynatrace Monitor Resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, MonitorResourceInner>
beginCreateOrUpdateAsync(String resourceGroupName, String monitorName, MonitorResourceInner resource) {
Mono>> mono
= createOrUpdateWithResponseAsync(resourceGroupName, monitorName, resource);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
MonitorResourceInner.class, MonitorResourceInner.class, this.client.getContext());
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of dynatrace Monitor Resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, MonitorResourceInner> beginCreateOrUpdateAsync(
String resourceGroupName, String monitorName, MonitorResourceInner resource, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= createOrUpdateWithResponseAsync(resourceGroupName, monitorName, resource, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
MonitorResourceInner.class, MonitorResourceInner.class, context);
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of dynatrace Monitor Resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MonitorResourceInner>
beginCreateOrUpdate(String resourceGroupName, String monitorName, MonitorResourceInner resource) {
return this.beginCreateOrUpdateAsync(resourceGroupName, monitorName, resource).getSyncPoller();
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of dynatrace Monitor Resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MonitorResourceInner> beginCreateOrUpdate(
String resourceGroupName, String monitorName, MonitorResourceInner resource, Context context) {
return this.beginCreateOrUpdateAsync(resourceGroupName, monitorName, resource, context).getSyncPoller();
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateAsync(String resourceGroupName, String monitorName,
MonitorResourceInner resource) {
return beginCreateOrUpdateAsync(resourceGroupName, monitorName, resource).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateAsync(String resourceGroupName, String monitorName,
MonitorResourceInner resource, Context context) {
return beginCreateOrUpdateAsync(resourceGroupName, monitorName, resource, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MonitorResourceInner createOrUpdate(String resourceGroupName, String monitorName,
MonitorResourceInner resource) {
return createOrUpdateAsync(resourceGroupName, monitorName, resource).block();
}
/**
* Create a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MonitorResourceInner createOrUpdate(String resourceGroupName, String monitorName,
MonitorResourceInner resource, Context context) {
return createOrUpdateAsync(resourceGroupName, monitorName, resource, context).block();
}
/**
* Update a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> updateWithResponseAsync(String resourceGroupName, String monitorName,
MonitorResourceUpdate resource) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (resource == null) {
return Mono.error(new IllegalArgumentException("Parameter resource is required and cannot be null."));
} else {
resource.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.update(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, resource, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Update a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> updateWithResponseAsync(String resourceGroupName, String monitorName,
MonitorResourceUpdate resource, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (resource == null) {
return Mono.error(new IllegalArgumentException("Parameter resource is required and cannot be null."));
} else {
resource.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.update(this.client.getEndpoint(), this.client.getApiVersion(), this.client.getSubscriptionId(),
resourceGroupName, monitorName, resource, accept, context);
}
/**
* Update a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono updateAsync(String resourceGroupName, String monitorName,
MonitorResourceUpdate resource) {
return updateWithResponseAsync(resourceGroupName, monitorName, resource)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Update a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response updateWithResponse(String resourceGroupName, String monitorName,
MonitorResourceUpdate resource, Context context) {
return updateWithResponseAsync(resourceGroupName, monitorName, resource, context).block();
}
/**
* Update a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param resource The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return dynatrace Monitor Resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MonitorResourceInner update(String resourceGroupName, String monitorName, MonitorResourceUpdate resource) {
return updateWithResponse(resourceGroupName, monitorName, resource, Context.NONE).getValue();
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteWithResponseAsync(String resourceGroupName, String monitorName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.delete(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteWithResponseAsync(String resourceGroupName, String monitorName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.delete(this.client.getEndpoint(), this.client.getApiVersion(), this.client.getSubscriptionId(),
resourceGroupName, monitorName, accept, context);
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String monitorName) {
Mono>> mono = deleteWithResponseAsync(resourceGroupName, monitorName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String monitorName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = deleteWithResponseAsync(resourceGroupName, monitorName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String monitorName) {
return this.beginDeleteAsync(resourceGroupName, monitorName).getSyncPoller();
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String monitorName,
Context context) {
return this.beginDeleteAsync(resourceGroupName, monitorName, context).getSyncPoller();
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String monitorName) {
return beginDeleteAsync(resourceGroupName, monitorName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String monitorName, Context context) {
return beginDeleteAsync(resourceGroupName, monitorName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String monitorName) {
deleteAsync(resourceGroupName, monitorName).block();
}
/**
* Delete a MonitorResource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String monitorName, Context context) {
deleteAsync(resourceGroupName, monitorName, context).block();
}
/**
* List all MonitorResource by subscriptionId.
*
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync() {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.list(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* List all MonitorResource by subscriptionId.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync(Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.list(this.client.getEndpoint(), this.client.getApiVersion(), this.client.getSubscriptionId(), accept,
context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* List all MonitorResource by subscriptionId.
*
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync() {
return new PagedFlux<>(() -> listSinglePageAsync(),
nextLink -> listBySubscriptionIdNextSinglePageAsync(nextLink));
}
/**
* List all MonitorResource by subscriptionId.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync(Context context) {
return new PagedFlux<>(() -> listSinglePageAsync(context),
nextLink -> listBySubscriptionIdNextSinglePageAsync(nextLink, context));
}
/**
* List all MonitorResource by subscriptionId.
*
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list() {
return new PagedIterable<>(listAsync());
}
/**
* List all MonitorResource by subscriptionId.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list(Context context) {
return new PagedIterable<>(listAsync(context));
}
/**
* List MonitorResource resources by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupSinglePageAsync(String resourceGroupName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listByResourceGroup(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* List MonitorResource resources by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupSinglePageAsync(String resourceGroupName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listByResourceGroup(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* List MonitorResource resources by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByResourceGroupAsync(String resourceGroupName) {
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName),
nextLink -> listByResourceGroupNextSinglePageAsync(nextLink));
}
/**
* List MonitorResource resources by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByResourceGroupAsync(String resourceGroupName, Context context) {
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName, context),
nextLink -> listByResourceGroupNextSinglePageAsync(nextLink, context));
}
/**
* List MonitorResource resources by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByResourceGroup(String resourceGroupName) {
return new PagedIterable<>(listByResourceGroupAsync(resourceGroupName));
}
/**
* List MonitorResource resources by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByResourceGroup(String resourceGroupName, Context context) {
return new PagedIterable<>(listByResourceGroupAsync(resourceGroupName, context));
}
/**
* Get Marketplace SaaS resource details of a tenant under a specific subscription.
*
* @param request Tenant Id.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 404.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return marketplace SaaS resource details of a tenant under a specific subscription along with {@link Response}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
getMarketplaceSaaSResourceDetailsWithResponseAsync(MarketplaceSaaSResourceDetailsRequest request) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (request == null) {
return Mono.error(new IllegalArgumentException("Parameter request is required and cannot be null."));
} else {
request.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getMarketplaceSaaSResourceDetails(this.client.getEndpoint(),
this.client.getApiVersion(), this.client.getSubscriptionId(), request, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get Marketplace SaaS resource details of a tenant under a specific subscription.
*
* @param request Tenant Id.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 404.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return marketplace SaaS resource details of a tenant under a specific subscription along with {@link Response}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
getMarketplaceSaaSResourceDetailsWithResponseAsync(MarketplaceSaaSResourceDetailsRequest request,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (request == null) {
return Mono.error(new IllegalArgumentException("Parameter request is required and cannot be null."));
} else {
request.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMarketplaceSaaSResourceDetails(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), request, accept, context);
}
/**
* Get Marketplace SaaS resource details of a tenant under a specific subscription.
*
* @param request Tenant Id.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 404.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return marketplace SaaS resource details of a tenant under a specific subscription on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono
getMarketplaceSaaSResourceDetailsAsync(MarketplaceSaaSResourceDetailsRequest request) {
return getMarketplaceSaaSResourceDetailsWithResponseAsync(request)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Get Marketplace SaaS resource details of a tenant under a specific subscription.
*
* @param request Tenant Id.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 404.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return marketplace SaaS resource details of a tenant under a specific subscription along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response
getMarketplaceSaaSResourceDetailsWithResponse(MarketplaceSaaSResourceDetailsRequest request, Context context) {
return getMarketplaceSaaSResourceDetailsWithResponseAsync(request, context).block();
}
/**
* Get Marketplace SaaS resource details of a tenant under a specific subscription.
*
* @param request Tenant Id.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 404.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return marketplace SaaS resource details of a tenant under a specific subscription.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MarketplaceSaaSResourceDetailsResponseInner
getMarketplaceSaaSResourceDetails(MarketplaceSaaSResourceDetailsRequest request) {
return getMarketplaceSaaSResourceDetailsWithResponse(request, Context.NONE).getValue();
}
/**
* List the VM/VMSS resources currently being monitored by the Dynatrace resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listHostsSinglePageAsync(String resourceGroupName, String monitorName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listHosts(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(),
res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* List the VM/VMSS resources currently being monitored by the Dynatrace resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listHostsSinglePageAsync(String resourceGroupName, String monitorName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listHosts(this.client.getEndpoint(), this.client.getApiVersion(), this.client.getSubscriptionId(),
resourceGroupName, monitorName, accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* List the VM/VMSS resources currently being monitored by the Dynatrace resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listHostsAsync(String resourceGroupName, String monitorName) {
return new PagedFlux<>(() -> listHostsSinglePageAsync(resourceGroupName, monitorName),
nextLink -> listHostsNextSinglePageAsync(nextLink));
}
/**
* List the VM/VMSS resources currently being monitored by the Dynatrace resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listHostsAsync(String resourceGroupName, String monitorName, Context context) {
return new PagedFlux<>(() -> listHostsSinglePageAsync(resourceGroupName, monitorName, context),
nextLink -> listHostsNextSinglePageAsync(nextLink, context));
}
/**
* List the VM/VMSS resources currently being monitored by the Dynatrace resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listHosts(String resourceGroupName, String monitorName) {
return new PagedIterable<>(listHostsAsync(resourceGroupName, monitorName));
}
/**
* List the VM/VMSS resources currently being monitored by the Dynatrace resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listHosts(String resourceGroupName, String monitorName, Context context) {
return new PagedIterable<>(listHostsAsync(resourceGroupName, monitorName, context));
}
/**
* Get metric status.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Name of the Monitor resource.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return metric status along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMetricStatusWithResponseAsync(String resourceGroupName,
String monitorName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getMetricStatus(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get metric status.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Name of the Monitor resource.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return metric status along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMetricStatusWithResponseAsync(String resourceGroupName,
String monitorName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMetricStatus(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context);
}
/**
* Get metric status.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Name of the Monitor resource.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return metric status on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getMetricStatusAsync(String resourceGroupName, String monitorName) {
return getMetricStatusWithResponseAsync(resourceGroupName, monitorName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Get metric status.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Name of the Monitor resource.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return metric status along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getMetricStatusWithResponse(String resourceGroupName,
String monitorName, Context context) {
return getMetricStatusWithResponseAsync(resourceGroupName, monitorName, context).block();
}
/**
* Get metric status.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Name of the Monitor resource.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return metric status.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MetricsStatusResponseInner getMetricStatus(String resourceGroupName, String monitorName) {
return getMetricStatusWithResponse(resourceGroupName, monitorName, Context.NONE).getValue();
}
/**
* Gets list of App Services with Dynatrace PaaS OneAgent enabled.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of App Services with Dynatrace PaaS OneAgent enabled along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listAppServicesSinglePageAsync(String resourceGroupName,
String monitorName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listAppServices(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets list of App Services with Dynatrace PaaS OneAgent enabled.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of App Services with Dynatrace PaaS OneAgent enabled along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listAppServicesSinglePageAsync(String resourceGroupName,
String monitorName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listAppServices(this.client.getEndpoint(), this.client.getApiVersion(), this.client.getSubscriptionId(),
resourceGroupName, monitorName, accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Gets list of App Services with Dynatrace PaaS OneAgent enabled.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of App Services with Dynatrace PaaS OneAgent enabled as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAppServicesAsync(String resourceGroupName, String monitorName) {
return new PagedFlux<>(() -> listAppServicesSinglePageAsync(resourceGroupName, monitorName),
nextLink -> listAppServicesNextSinglePageAsync(nextLink));
}
/**
* Gets list of App Services with Dynatrace PaaS OneAgent enabled.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of App Services with Dynatrace PaaS OneAgent enabled as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAppServicesAsync(String resourceGroupName, String monitorName,
Context context) {
return new PagedFlux<>(() -> listAppServicesSinglePageAsync(resourceGroupName, monitorName, context),
nextLink -> listAppServicesNextSinglePageAsync(nextLink, context));
}
/**
* Gets list of App Services with Dynatrace PaaS OneAgent enabled.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of App Services with Dynatrace PaaS OneAgent enabled as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listAppServices(String resourceGroupName, String monitorName) {
return new PagedIterable<>(listAppServicesAsync(resourceGroupName, monitorName));
}
/**
* Gets list of App Services with Dynatrace PaaS OneAgent enabled.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of App Services with Dynatrace PaaS OneAgent enabled as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listAppServices(String resourceGroupName, String monitorName,
Context context) {
return new PagedIterable<>(listAppServicesAsync(resourceGroupName, monitorName, context));
}
/**
* Gets the SSO configuration details from the partner.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the get sso details request.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 401.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SSO configuration details from the partner along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getSsoDetailsWithResponseAsync(String resourceGroupName,
String monitorName, SsoDetailsRequest request) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (request != null) {
request.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getSsoDetails(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, request, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets the SSO configuration details from the partner.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the get sso details request.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 401.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SSO configuration details from the partner along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getSsoDetailsWithResponseAsync(String resourceGroupName,
String monitorName, SsoDetailsRequest request, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (request != null) {
request.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getSsoDetails(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, request, accept, context);
}
/**
* Gets the SSO configuration details from the partner.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 401.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SSO configuration details from the partner on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getSsoDetailsAsync(String resourceGroupName, String monitorName) {
final SsoDetailsRequest request = null;
return getSsoDetailsWithResponseAsync(resourceGroupName, monitorName, request)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets the SSO configuration details from the partner.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the get sso details request.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 401.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SSO configuration details from the partner along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getSsoDetailsWithResponse(String resourceGroupName, String monitorName,
SsoDetailsRequest request, Context context) {
return getSsoDetailsWithResponseAsync(resourceGroupName, monitorName, request, context).block();
}
/**
* Gets the SSO configuration details from the partner.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws ManagementException thrown if the request is rejected by server on status code 401.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SSO configuration details from the partner.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public SsoDetailsResponseInner getSsoDetails(String resourceGroupName, String monitorName) {
final SsoDetailsRequest request = null;
return getSsoDetailsWithResponse(resourceGroupName, monitorName, request, Context.NONE).getValue();
}
/**
* Gets all the Dynatrace environments that a user can link a azure resource to.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the linkable environment request.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Dynatrace environments that a user can link a azure resource to along with {@link PagedResponse}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listLinkableEnvironmentsSinglePageAsync(
String resourceGroupName, String monitorName, LinkableEnvironmentRequest request) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (request == null) {
return Mono.error(new IllegalArgumentException("Parameter request is required and cannot be null."));
} else {
request.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listLinkableEnvironments(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, request, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets all the Dynatrace environments that a user can link a azure resource to.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the linkable environment request.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Dynatrace environments that a user can link a azure resource to along with {@link PagedResponse}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listLinkableEnvironmentsSinglePageAsync(
String resourceGroupName, String monitorName, LinkableEnvironmentRequest request, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (monitorName == null) {
return Mono.error(new IllegalArgumentException("Parameter monitorName is required and cannot be null."));
}
if (request == null) {
return Mono.error(new IllegalArgumentException("Parameter request is required and cannot be null."));
} else {
request.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listLinkableEnvironments(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), resourceGroupName, monitorName, request, accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Gets all the Dynatrace environments that a user can link a azure resource to.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the linkable environment request.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Dynatrace environments that a user can link a azure resource to as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listLinkableEnvironmentsAsync(String resourceGroupName,
String monitorName, LinkableEnvironmentRequest request) {
return new PagedFlux<>(() -> listLinkableEnvironmentsSinglePageAsync(resourceGroupName, monitorName, request),
nextLink -> listLinkableEnvironmentsNextSinglePageAsync(nextLink));
}
/**
* Gets all the Dynatrace environments that a user can link a azure resource to.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the linkable environment request.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Dynatrace environments that a user can link a azure resource to as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listLinkableEnvironmentsAsync(String resourceGroupName,
String monitorName, LinkableEnvironmentRequest request, Context context) {
return new PagedFlux<>(
() -> listLinkableEnvironmentsSinglePageAsync(resourceGroupName, monitorName, request, context),
nextLink -> listLinkableEnvironmentsNextSinglePageAsync(nextLink, context));
}
/**
* Gets all the Dynatrace environments that a user can link a azure resource to.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the linkable environment request.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Dynatrace environments that a user can link a azure resource to as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listLinkableEnvironments(String resourceGroupName,
String monitorName, LinkableEnvironmentRequest request) {
return new PagedIterable<>(listLinkableEnvironmentsAsync(resourceGroupName, monitorName, request));
}
/**
* Gets all the Dynatrace environments that a user can link a azure resource to.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param monitorName Monitor resource name.
* @param request The details of the linkable environment request.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the Dynatrace environments that a user can link a azure resource to as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listLinkableEnvironments(String resourceGroupName,
String monitorName, LinkableEnvironmentRequest request, Context context) {
return new PagedIterable<>(listLinkableEnvironmentsAsync(resourceGroupName, monitorName, request, context));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource along with {@link PagedResponse}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listMonitoredResourcesNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listMonitoredResourcesNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of all the resources being monitored by Dynatrace monitor resource along with {@link PagedResponse}
* on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listMonitoredResourcesNextSinglePageAsync(String nextLink,
Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listMonitoredResourcesNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listBySubscriptionIdNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listBySubscriptionIdNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listBySubscriptionIdNextSinglePageAsync(String nextLink,
Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listBySubscriptionIdNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listByResourceGroupNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a MonitorResource list operation along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupNextSinglePageAsync(String nextLink,
Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listByResourceGroupNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listHostsNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listHostsNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(),
res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list VM Host Operation along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listHostsNextSinglePageAsync(String nextLink, Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listHostsNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list App Services Operation along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listAppServicesNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listAppServicesNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response of a list App Services Operation along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listAppServicesNextSinglePageAsync(String nextLink,
Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listAppServicesNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for getting all the linkable environments along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listLinkableEnvironmentsNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listLinkableEnvironmentsNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for getting all the linkable environments along with {@link PagedResponse} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listLinkableEnvironmentsNextSinglePageAsync(String nextLink, Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listLinkableEnvironmentsNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy