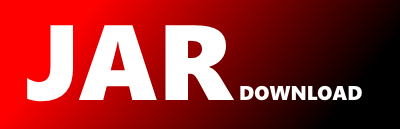
com.azure.resourcemanager.elasticsan.models.ScaleUpProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-elasticsan Show documentation
Show all versions of azure-resourcemanager-elasticsan Show documentation
This package contains Microsoft Azure SDK for ElasticSan Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-preview-2024-06.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.elasticsan.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Scale up properties on Elastic San Appliance.
*/
@Fluent
public final class ScaleUpProperties implements JsonSerializable {
/*
* Unused size on Elastic San appliance in TiB.
*/
private Long unusedSizeTiB;
/*
* Unit to increase Capacity Unit on Elastic San appliance in TiB.
*/
private Long increaseCapacityUnitByTiB;
/*
* Maximum scale up size on Elastic San appliance in TiB.
*/
private Long capacityUnitScaleUpLimitTiB;
/*
* Enable or Disable scale up setting on Elastic San Appliance.
*/
private AutoScalePolicyEnforcement autoScalePolicyEnforcement;
/**
* Creates an instance of ScaleUpProperties class.
*/
public ScaleUpProperties() {
}
/**
* Get the unusedSizeTiB property: Unused size on Elastic San appliance in TiB.
*
* @return the unusedSizeTiB value.
*/
public Long unusedSizeTiB() {
return this.unusedSizeTiB;
}
/**
* Set the unusedSizeTiB property: Unused size on Elastic San appliance in TiB.
*
* @param unusedSizeTiB the unusedSizeTiB value to set.
* @return the ScaleUpProperties object itself.
*/
public ScaleUpProperties withUnusedSizeTiB(Long unusedSizeTiB) {
this.unusedSizeTiB = unusedSizeTiB;
return this;
}
/**
* Get the increaseCapacityUnitByTiB property: Unit to increase Capacity Unit on Elastic San appliance in TiB.
*
* @return the increaseCapacityUnitByTiB value.
*/
public Long increaseCapacityUnitByTiB() {
return this.increaseCapacityUnitByTiB;
}
/**
* Set the increaseCapacityUnitByTiB property: Unit to increase Capacity Unit on Elastic San appliance in TiB.
*
* @param increaseCapacityUnitByTiB the increaseCapacityUnitByTiB value to set.
* @return the ScaleUpProperties object itself.
*/
public ScaleUpProperties withIncreaseCapacityUnitByTiB(Long increaseCapacityUnitByTiB) {
this.increaseCapacityUnitByTiB = increaseCapacityUnitByTiB;
return this;
}
/**
* Get the capacityUnitScaleUpLimitTiB property: Maximum scale up size on Elastic San appliance in TiB.
*
* @return the capacityUnitScaleUpLimitTiB value.
*/
public Long capacityUnitScaleUpLimitTiB() {
return this.capacityUnitScaleUpLimitTiB;
}
/**
* Set the capacityUnitScaleUpLimitTiB property: Maximum scale up size on Elastic San appliance in TiB.
*
* @param capacityUnitScaleUpLimitTiB the capacityUnitScaleUpLimitTiB value to set.
* @return the ScaleUpProperties object itself.
*/
public ScaleUpProperties withCapacityUnitScaleUpLimitTiB(Long capacityUnitScaleUpLimitTiB) {
this.capacityUnitScaleUpLimitTiB = capacityUnitScaleUpLimitTiB;
return this;
}
/**
* Get the autoScalePolicyEnforcement property: Enable or Disable scale up setting on Elastic San Appliance.
*
* @return the autoScalePolicyEnforcement value.
*/
public AutoScalePolicyEnforcement autoScalePolicyEnforcement() {
return this.autoScalePolicyEnforcement;
}
/**
* Set the autoScalePolicyEnforcement property: Enable or Disable scale up setting on Elastic San Appliance.
*
* @param autoScalePolicyEnforcement the autoScalePolicyEnforcement value to set.
* @return the ScaleUpProperties object itself.
*/
public ScaleUpProperties withAutoScalePolicyEnforcement(AutoScalePolicyEnforcement autoScalePolicyEnforcement) {
this.autoScalePolicyEnforcement = autoScalePolicyEnforcement;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("unusedSizeTiB", this.unusedSizeTiB);
jsonWriter.writeNumberField("increaseCapacityUnitByTiB", this.increaseCapacityUnitByTiB);
jsonWriter.writeNumberField("capacityUnitScaleUpLimitTiB", this.capacityUnitScaleUpLimitTiB);
jsonWriter.writeStringField("autoScalePolicyEnforcement",
this.autoScalePolicyEnforcement == null ? null : this.autoScalePolicyEnforcement.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScaleUpProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScaleUpProperties if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the ScaleUpProperties.
*/
public static ScaleUpProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScaleUpProperties deserializedScaleUpProperties = new ScaleUpProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("unusedSizeTiB".equals(fieldName)) {
deserializedScaleUpProperties.unusedSizeTiB = reader.getNullable(JsonReader::getLong);
} else if ("increaseCapacityUnitByTiB".equals(fieldName)) {
deserializedScaleUpProperties.increaseCapacityUnitByTiB = reader.getNullable(JsonReader::getLong);
} else if ("capacityUnitScaleUpLimitTiB".equals(fieldName)) {
deserializedScaleUpProperties.capacityUnitScaleUpLimitTiB = reader.getNullable(JsonReader::getLong);
} else if ("autoScalePolicyEnforcement".equals(fieldName)) {
deserializedScaleUpProperties.autoScalePolicyEnforcement
= AutoScalePolicyEnforcement.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedScaleUpProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy