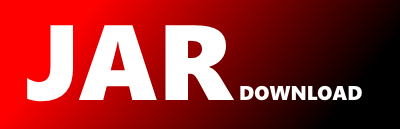
com.azure.resourcemanager.eventgrid.fluent.models.CaCertificateInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-eventgrid Show documentation
Show all versions of azure-resourcemanager-eventgrid Show documentation
This package contains Microsoft Azure SDK for EventGrid Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure EventGrid Management Client. Package tag package-2021-10-preview.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.eventgrid.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.ProxyResource;
import com.azure.core.management.SystemData;
import com.azure.resourcemanager.eventgrid.models.CaCertificateProvisioningState;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
/**
* The CA Certificate resource.
*/
@Fluent
public final class CaCertificateInner extends ProxyResource {
/*
* The properties of CA certificate.
*/
@JsonProperty(value = "properties")
private CaCertificateProperties innerProperties;
/*
* The system metadata relating to the CaCertificate resource.
*/
@JsonProperty(value = "systemData", access = JsonProperty.Access.WRITE_ONLY)
private SystemData systemData;
/**
* Creates an instance of CaCertificateInner class.
*/
public CaCertificateInner() {
}
/**
* Get the innerProperties property: The properties of CA certificate.
*
* @return the innerProperties value.
*/
private CaCertificateProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the systemData property: The system metadata relating to the CaCertificate resource.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* Get the description property: Description for the CA Certificate resource.
*
* @return the description value.
*/
public String description() {
return this.innerProperties() == null ? null : this.innerProperties().description();
}
/**
* Set the description property: Description for the CA Certificate resource.
*
* @param description the description value to set.
* @return the CaCertificateInner object itself.
*/
public CaCertificateInner withDescription(String description) {
if (this.innerProperties() == null) {
this.innerProperties = new CaCertificateProperties();
}
this.innerProperties().withDescription(description);
return this;
}
/**
* Get the encodedCertificate property: Base64 encoded PEM (Privacy Enhanced Mail) format certificate data.
*
* @return the encodedCertificate value.
*/
public String encodedCertificate() {
return this.innerProperties() == null ? null : this.innerProperties().encodedCertificate();
}
/**
* Set the encodedCertificate property: Base64 encoded PEM (Privacy Enhanced Mail) format certificate data.
*
* @param encodedCertificate the encodedCertificate value to set.
* @return the CaCertificateInner object itself.
*/
public CaCertificateInner withEncodedCertificate(String encodedCertificate) {
if (this.innerProperties() == null) {
this.innerProperties = new CaCertificateProperties();
}
this.innerProperties().withEncodedCertificate(encodedCertificate);
return this;
}
/**
* Get the issueTimeInUtc property: Certificate issue time in UTC. This is a read-only field.
*
* @return the issueTimeInUtc value.
*/
public OffsetDateTime issueTimeInUtc() {
return this.innerProperties() == null ? null : this.innerProperties().issueTimeInUtc();
}
/**
* Get the expiryTimeInUtc property: Certificate expiry time in UTC. This is a read-only field.
*
* @return the expiryTimeInUtc value.
*/
public OffsetDateTime expiryTimeInUtc() {
return this.innerProperties() == null ? null : this.innerProperties().expiryTimeInUtc();
}
/**
* Get the provisioningState property: Provisioning state of the CA Certificate resource.
*
* @return the provisioningState value.
*/
public CaCertificateProvisioningState provisioningState() {
return this.innerProperties() == null ? null : this.innerProperties().provisioningState();
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy