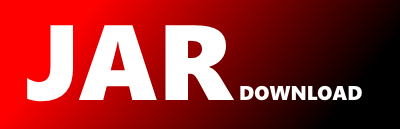
com.azure.resourcemanager.eventgrid.fluent.models.PermissionBindingInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-eventgrid Show documentation
Show all versions of azure-resourcemanager-eventgrid Show documentation
This package contains Microsoft Azure SDK for EventGrid Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure EventGrid Management Client. Package tag package-2021-10-preview.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.eventgrid.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.ProxyResource;
import com.azure.core.management.SystemData;
import com.azure.resourcemanager.eventgrid.models.PermissionBindingProvisioningState;
import com.azure.resourcemanager.eventgrid.models.PermissionType;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* The Permission binding resource.
*/
@Fluent
public final class PermissionBindingInner extends ProxyResource {
/*
* The properties of permission binding.
*/
@JsonProperty(value = "properties")
private PermissionBindingProperties innerProperties;
/*
* The system metadata relating to the PermissionBinding resource.
*/
@JsonProperty(value = "systemData", access = JsonProperty.Access.WRITE_ONLY)
private SystemData systemData;
/**
* Creates an instance of PermissionBindingInner class.
*/
public PermissionBindingInner() {
}
/**
* Get the innerProperties property: The properties of permission binding.
*
* @return the innerProperties value.
*/
private PermissionBindingProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the systemData property: The system metadata relating to the PermissionBinding resource.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* Get the description property: Description for the Permission Binding resource.
*
* @return the description value.
*/
public String description() {
return this.innerProperties() == null ? null : this.innerProperties().description();
}
/**
* Set the description property: Description for the Permission Binding resource.
*
* @param description the description value to set.
* @return the PermissionBindingInner object itself.
*/
public PermissionBindingInner withDescription(String description) {
if (this.innerProperties() == null) {
this.innerProperties = new PermissionBindingProperties();
}
this.innerProperties().withDescription(description);
return this;
}
/**
* Get the topicSpaceName property: The name of the Topic Space resource that the permission is bound to.
* The Topic space needs to be a resource under the same namespace the permission binding is a part of.
*
* @return the topicSpaceName value.
*/
public String topicSpaceName() {
return this.innerProperties() == null ? null : this.innerProperties().topicSpaceName();
}
/**
* Set the topicSpaceName property: The name of the Topic Space resource that the permission is bound to.
* The Topic space needs to be a resource under the same namespace the permission binding is a part of.
*
* @param topicSpaceName the topicSpaceName value to set.
* @return the PermissionBindingInner object itself.
*/
public PermissionBindingInner withTopicSpaceName(String topicSpaceName) {
if (this.innerProperties() == null) {
this.innerProperties = new PermissionBindingProperties();
}
this.innerProperties().withTopicSpaceName(topicSpaceName);
return this;
}
/**
* Get the permission property: The allowed permission.
*
* @return the permission value.
*/
public PermissionType permission() {
return this.innerProperties() == null ? null : this.innerProperties().permission();
}
/**
* Set the permission property: The allowed permission.
*
* @param permission the permission value to set.
* @return the PermissionBindingInner object itself.
*/
public PermissionBindingInner withPermission(PermissionType permission) {
if (this.innerProperties() == null) {
this.innerProperties = new PermissionBindingProperties();
}
this.innerProperties().withPermission(permission);
return this;
}
/**
* Get the clientGroupName property: The name of the client group resource that the permission is bound to.
* The client group needs to be a resource under the same namespace the permission binding is a part of.
*
* @return the clientGroupName value.
*/
public String clientGroupName() {
return this.innerProperties() == null ? null : this.innerProperties().clientGroupName();
}
/**
* Set the clientGroupName property: The name of the client group resource that the permission is bound to.
* The client group needs to be a resource under the same namespace the permission binding is a part of.
*
* @param clientGroupName the clientGroupName value to set.
* @return the PermissionBindingInner object itself.
*/
public PermissionBindingInner withClientGroupName(String clientGroupName) {
if (this.innerProperties() == null) {
this.innerProperties = new PermissionBindingProperties();
}
this.innerProperties().withClientGroupName(clientGroupName);
return this;
}
/**
* Get the provisioningState property: Provisioning state of the PermissionBinding resource.
*
* @return the provisioningState value.
*/
public PermissionBindingProvisioningState provisioningState() {
return this.innerProperties() == null ? null : this.innerProperties().provisioningState();
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy