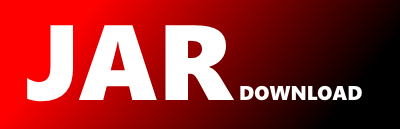
com.azure.resourcemanager.eventgrid.fluent.models.VerifiedPartnerProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-eventgrid Show documentation
Show all versions of azure-resourcemanager-eventgrid Show documentation
This package contains Microsoft Azure SDK for EventGrid Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure EventGrid Management Client. Package tag package-2021-10-preview.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.eventgrid.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.resourcemanager.eventgrid.models.PartnerDetails;
import com.azure.resourcemanager.eventgrid.models.VerifiedPartnerProvisioningState;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.UUID;
/**
* Properties of the verified partner.
*/
@Fluent
public final class VerifiedPartnerProperties {
/*
* ImmutableId of the corresponding partner registration.
*/
@JsonProperty(value = "partnerRegistrationImmutableId")
private UUID partnerRegistrationImmutableId;
/*
* Official name of the Partner.
*/
@JsonProperty(value = "organizationName")
private String organizationName;
/*
* Display name of the verified partner.
*/
@JsonProperty(value = "partnerDisplayName")
private String partnerDisplayName;
/*
* Details of the partner topic scenario.
*/
@JsonProperty(value = "partnerTopicDetails")
private PartnerDetails partnerTopicDetails;
/*
* Details of the partner destination scenario.
*/
@JsonProperty(value = "partnerDestinationDetails")
private PartnerDetails partnerDestinationDetails;
/*
* Provisioning state of the verified partner.
*/
@JsonProperty(value = "provisioningState")
private VerifiedPartnerProvisioningState provisioningState;
/**
* Creates an instance of VerifiedPartnerProperties class.
*/
public VerifiedPartnerProperties() {
}
/**
* Get the partnerRegistrationImmutableId property: ImmutableId of the corresponding partner registration.
*
* @return the partnerRegistrationImmutableId value.
*/
public UUID partnerRegistrationImmutableId() {
return this.partnerRegistrationImmutableId;
}
/**
* Set the partnerRegistrationImmutableId property: ImmutableId of the corresponding partner registration.
*
* @param partnerRegistrationImmutableId the partnerRegistrationImmutableId value to set.
* @return the VerifiedPartnerProperties object itself.
*/
public VerifiedPartnerProperties withPartnerRegistrationImmutableId(UUID partnerRegistrationImmutableId) {
this.partnerRegistrationImmutableId = partnerRegistrationImmutableId;
return this;
}
/**
* Get the organizationName property: Official name of the Partner.
*
* @return the organizationName value.
*/
public String organizationName() {
return this.organizationName;
}
/**
* Set the organizationName property: Official name of the Partner.
*
* @param organizationName the organizationName value to set.
* @return the VerifiedPartnerProperties object itself.
*/
public VerifiedPartnerProperties withOrganizationName(String organizationName) {
this.organizationName = organizationName;
return this;
}
/**
* Get the partnerDisplayName property: Display name of the verified partner.
*
* @return the partnerDisplayName value.
*/
public String partnerDisplayName() {
return this.partnerDisplayName;
}
/**
* Set the partnerDisplayName property: Display name of the verified partner.
*
* @param partnerDisplayName the partnerDisplayName value to set.
* @return the VerifiedPartnerProperties object itself.
*/
public VerifiedPartnerProperties withPartnerDisplayName(String partnerDisplayName) {
this.partnerDisplayName = partnerDisplayName;
return this;
}
/**
* Get the partnerTopicDetails property: Details of the partner topic scenario.
*
* @return the partnerTopicDetails value.
*/
public PartnerDetails partnerTopicDetails() {
return this.partnerTopicDetails;
}
/**
* Set the partnerTopicDetails property: Details of the partner topic scenario.
*
* @param partnerTopicDetails the partnerTopicDetails value to set.
* @return the VerifiedPartnerProperties object itself.
*/
public VerifiedPartnerProperties withPartnerTopicDetails(PartnerDetails partnerTopicDetails) {
this.partnerTopicDetails = partnerTopicDetails;
return this;
}
/**
* Get the partnerDestinationDetails property: Details of the partner destination scenario.
*
* @return the partnerDestinationDetails value.
*/
public PartnerDetails partnerDestinationDetails() {
return this.partnerDestinationDetails;
}
/**
* Set the partnerDestinationDetails property: Details of the partner destination scenario.
*
* @param partnerDestinationDetails the partnerDestinationDetails value to set.
* @return the VerifiedPartnerProperties object itself.
*/
public VerifiedPartnerProperties withPartnerDestinationDetails(PartnerDetails partnerDestinationDetails) {
this.partnerDestinationDetails = partnerDestinationDetails;
return this;
}
/**
* Get the provisioningState property: Provisioning state of the verified partner.
*
* @return the provisioningState value.
*/
public VerifiedPartnerProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Set the provisioningState property: Provisioning state of the verified partner.
*
* @param provisioningState the provisioningState value to set.
* @return the VerifiedPartnerProperties object itself.
*/
public VerifiedPartnerProperties withProvisioningState(VerifiedPartnerProvisioningState provisioningState) {
this.provisioningState = provisioningState;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (partnerTopicDetails() != null) {
partnerTopicDetails().validate();
}
if (partnerDestinationDetails() != null) {
partnerDestinationDetails().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy