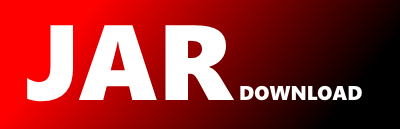
com.azure.resourcemanager.eventgrid.models.CustomDomainConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-eventgrid Show documentation
Show all versions of azure-resourcemanager-eventgrid Show documentation
This package contains Microsoft Azure SDK for EventGrid Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure EventGrid Management Client. Package tag package-2021-10-preview.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.eventgrid.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* A custom domain configuration that allows users to publish to their own domain name.
*/
@Fluent
public final class CustomDomainConfiguration {
/*
* Fully Qualified Domain Name (FQDN) for the custom domain.
*/
@JsonProperty(value = "fullyQualifiedDomainName", required = true)
private String fullyQualifiedDomainName;
/*
* Validation state for the custom domain. This is a read only property and is initially set to 'Pending' and will be updated to 'Approved' by Event Grid only after ownership of the domain name has been successfully validated.
*/
@JsonProperty(value = "validationState")
private CustomDomainValidationState validationState;
/*
* Identity info for accessing the certificate for the custom domain. This identity info must match an identity that has been set on the namespace.
*/
@JsonProperty(value = "identity")
private CustomDomainIdentity identity;
/*
* The URL for the certificate that is used for publishing to the custom domain. We currently support certificates stored in Azure Key Vault only. While certificate URL can be either
* versioned URL of the following format https://{key-vault-name}.vault.azure.net/certificates/{certificate-name}/{version-id}, or unversioned URL of the following format (e.g.,
* https://contosovault.vault.azure.net/certificates/contosocert, we support unversioned certificate URL only (e.g., https://contosovault.vault.azure.net/certificates/contosocert)
*/
@JsonProperty(value = "certificateUrl")
private String certificateUrl;
/*
* Expected DNS TXT record name. Event Grid will check for a TXT record with this name in the DNS record set of the custom domain name to prove ownership over the domain.
* The values under this TXT record must contain the expected TXT record value.
*/
@JsonProperty(value = "expectedTxtRecordName")
private String expectedTxtRecordName;
/*
* Expected DNS TXT record value. Event Grid will check for a TXT record with this value in the DNS record set of the custom domain name to prove ownership over the domain.
*/
@JsonProperty(value = "expectedTxtRecordValue")
private String expectedTxtRecordValue;
/**
* Creates an instance of CustomDomainConfiguration class.
*/
public CustomDomainConfiguration() {
}
/**
* Get the fullyQualifiedDomainName property: Fully Qualified Domain Name (FQDN) for the custom domain.
*
* @return the fullyQualifiedDomainName value.
*/
public String fullyQualifiedDomainName() {
return this.fullyQualifiedDomainName;
}
/**
* Set the fullyQualifiedDomainName property: Fully Qualified Domain Name (FQDN) for the custom domain.
*
* @param fullyQualifiedDomainName the fullyQualifiedDomainName value to set.
* @return the CustomDomainConfiguration object itself.
*/
public CustomDomainConfiguration withFullyQualifiedDomainName(String fullyQualifiedDomainName) {
this.fullyQualifiedDomainName = fullyQualifiedDomainName;
return this;
}
/**
* Get the validationState property: Validation state for the custom domain. This is a read only property and is
* initially set to 'Pending' and will be updated to 'Approved' by Event Grid only after ownership of the domain
* name has been successfully validated.
*
* @return the validationState value.
*/
public CustomDomainValidationState validationState() {
return this.validationState;
}
/**
* Set the validationState property: Validation state for the custom domain. This is a read only property and is
* initially set to 'Pending' and will be updated to 'Approved' by Event Grid only after ownership of the domain
* name has been successfully validated.
*
* @param validationState the validationState value to set.
* @return the CustomDomainConfiguration object itself.
*/
public CustomDomainConfiguration withValidationState(CustomDomainValidationState validationState) {
this.validationState = validationState;
return this;
}
/**
* Get the identity property: Identity info for accessing the certificate for the custom domain. This identity info
* must match an identity that has been set on the namespace.
*
* @return the identity value.
*/
public CustomDomainIdentity identity() {
return this.identity;
}
/**
* Set the identity property: Identity info for accessing the certificate for the custom domain. This identity info
* must match an identity that has been set on the namespace.
*
* @param identity the identity value to set.
* @return the CustomDomainConfiguration object itself.
*/
public CustomDomainConfiguration withIdentity(CustomDomainIdentity identity) {
this.identity = identity;
return this;
}
/**
* Get the certificateUrl property: The URL for the certificate that is used for publishing to the custom domain. We
* currently support certificates stored in Azure Key Vault only. While certificate URL can be either
* versioned URL of the following format
* https://{key-vault-name}.vault.azure.net/certificates/{certificate-name}/{version-id}, or unversioned URL of the
* following format (e.g.,
* https://contosovault.vault.azure.net/certificates/contosocert, we support unversioned certificate URL only (e.g.,
* https://contosovault.vault.azure.net/certificates/contosocert).
*
* @return the certificateUrl value.
*/
public String certificateUrl() {
return this.certificateUrl;
}
/**
* Set the certificateUrl property: The URL for the certificate that is used for publishing to the custom domain. We
* currently support certificates stored in Azure Key Vault only. While certificate URL can be either
* versioned URL of the following format
* https://{key-vault-name}.vault.azure.net/certificates/{certificate-name}/{version-id}, or unversioned URL of the
* following format (e.g.,
* https://contosovault.vault.azure.net/certificates/contosocert, we support unversioned certificate URL only (e.g.,
* https://contosovault.vault.azure.net/certificates/contosocert).
*
* @param certificateUrl the certificateUrl value to set.
* @return the CustomDomainConfiguration object itself.
*/
public CustomDomainConfiguration withCertificateUrl(String certificateUrl) {
this.certificateUrl = certificateUrl;
return this;
}
/**
* Get the expectedTxtRecordName property: Expected DNS TXT record name. Event Grid will check for a TXT record with
* this name in the DNS record set of the custom domain name to prove ownership over the domain.
* The values under this TXT record must contain the expected TXT record value.
*
* @return the expectedTxtRecordName value.
*/
public String expectedTxtRecordName() {
return this.expectedTxtRecordName;
}
/**
* Set the expectedTxtRecordName property: Expected DNS TXT record name. Event Grid will check for a TXT record with
* this name in the DNS record set of the custom domain name to prove ownership over the domain.
* The values under this TXT record must contain the expected TXT record value.
*
* @param expectedTxtRecordName the expectedTxtRecordName value to set.
* @return the CustomDomainConfiguration object itself.
*/
public CustomDomainConfiguration withExpectedTxtRecordName(String expectedTxtRecordName) {
this.expectedTxtRecordName = expectedTxtRecordName;
return this;
}
/**
* Get the expectedTxtRecordValue property: Expected DNS TXT record value. Event Grid will check for a TXT record
* with this value in the DNS record set of the custom domain name to prove ownership over the domain.
*
* @return the expectedTxtRecordValue value.
*/
public String expectedTxtRecordValue() {
return this.expectedTxtRecordValue;
}
/**
* Set the expectedTxtRecordValue property: Expected DNS TXT record value. Event Grid will check for a TXT record
* with this value in the DNS record set of the custom domain name to prove ownership over the domain.
*
* @param expectedTxtRecordValue the expectedTxtRecordValue value to set.
* @return the CustomDomainConfiguration object itself.
*/
public CustomDomainConfiguration withExpectedTxtRecordValue(String expectedTxtRecordValue) {
this.expectedTxtRecordValue = expectedTxtRecordValue;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (fullyQualifiedDomainName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property fullyQualifiedDomainName in model CustomDomainConfiguration"));
}
if (identity() != null) {
identity().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(CustomDomainConfiguration.class);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy