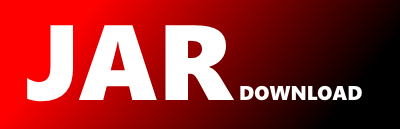
com.azure.resourcemanager.hybridcompute.fluent.models.MachineInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-hybridcompute Show documentation
Show all versions of azure-resourcemanager-hybridcompute Show documentation
This package contains Microsoft Azure SDK for HybridCompute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. The Hybrid Compute Management Client. Package tag package-preview-2024-07.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.hybridcompute.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.Resource;
import com.azure.core.management.SystemData;
import com.azure.core.management.exception.ManagementError;
import com.azure.resourcemanager.hybridcompute.models.AgentConfiguration;
import com.azure.resourcemanager.hybridcompute.models.AgentUpgrade;
import com.azure.resourcemanager.hybridcompute.models.ArcKindEnum;
import com.azure.resourcemanager.hybridcompute.models.CloudMetadata;
import com.azure.resourcemanager.hybridcompute.models.Identity;
import com.azure.resourcemanager.hybridcompute.models.LocationData;
import com.azure.resourcemanager.hybridcompute.models.MachineExtensionInstanceView;
import com.azure.resourcemanager.hybridcompute.models.OSProfile;
import com.azure.resourcemanager.hybridcompute.models.ServiceStatuses;
import com.azure.resourcemanager.hybridcompute.models.StatusTypes;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
import java.util.UUID;
/**
* Describes a hybrid machine.
*/
@Fluent
public final class MachineInner extends Resource {
/*
* Hybrid Compute Machine properties
*/
@JsonProperty(value = "properties")
private MachinePropertiesInner innerProperties;
/*
* The list of extensions affiliated to the machine
*/
@JsonProperty(value = "resources", access = JsonProperty.Access.WRITE_ONLY)
private List resources;
/*
* Identity for the resource.
*/
@JsonProperty(value = "identity")
private Identity identity;
/*
* Indicates which kind of Arc machine placement on-premises, such as HCI, SCVMM or VMware etc.
*/
@JsonProperty(value = "kind")
private ArcKindEnum kind;
/*
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*/
@JsonProperty(value = "systemData", access = JsonProperty.Access.WRITE_ONLY)
private SystemData systemData;
/**
* Creates an instance of MachineInner class.
*/
public MachineInner() {
}
/**
* Get the innerProperties property: Hybrid Compute Machine properties.
*
* @return the innerProperties value.
*/
private MachinePropertiesInner innerProperties() {
return this.innerProperties;
}
/**
* Get the resources property: The list of extensions affiliated to the machine.
*
* @return the resources value.
*/
public List resources() {
return this.resources;
}
/**
* Get the identity property: Identity for the resource.
*
* @return the identity value.
*/
public Identity identity() {
return this.identity;
}
/**
* Set the identity property: Identity for the resource.
*
* @param identity the identity value to set.
* @return the MachineInner object itself.
*/
public MachineInner withIdentity(Identity identity) {
this.identity = identity;
return this;
}
/**
* Get the kind property: Indicates which kind of Arc machine placement on-premises, such as HCI, SCVMM or VMware
* etc.
*
* @return the kind value.
*/
public ArcKindEnum kind() {
return this.kind;
}
/**
* Set the kind property: Indicates which kind of Arc machine placement on-premises, such as HCI, SCVMM or VMware
* etc.
*
* @param kind the kind value to set.
* @return the MachineInner object itself.
*/
public MachineInner withKind(ArcKindEnum kind) {
this.kind = kind;
return this;
}
/**
* Get the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* {@inheritDoc}
*/
@Override
public MachineInner withLocation(String location) {
super.withLocation(location);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MachineInner withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Get the locationData property: Metadata pertaining to the geographic location of the resource.
*
* @return the locationData value.
*/
public LocationData locationData() {
return this.innerProperties() == null ? null : this.innerProperties().locationData();
}
/**
* Set the locationData property: Metadata pertaining to the geographic location of the resource.
*
* @param locationData the locationData value to set.
* @return the MachineInner object itself.
*/
public MachineInner withLocationData(LocationData locationData) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withLocationData(locationData);
return this;
}
/**
* Get the agentConfiguration property: Configurable properties that the user can set locally via the azcmagent
* config command, or remotely via ARM.
*
* @return the agentConfiguration value.
*/
public AgentConfiguration agentConfiguration() {
return this.innerProperties() == null ? null : this.innerProperties().agentConfiguration();
}
/**
* Get the serviceStatuses property: Statuses of dependent services that are reported back to ARM.
*
* @return the serviceStatuses value.
*/
public ServiceStatuses serviceStatuses() {
return this.innerProperties() == null ? null : this.innerProperties().serviceStatuses();
}
/**
* Set the serviceStatuses property: Statuses of dependent services that are reported back to ARM.
*
* @param serviceStatuses the serviceStatuses value to set.
* @return the MachineInner object itself.
*/
public MachineInner withServiceStatuses(ServiceStatuses serviceStatuses) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withServiceStatuses(serviceStatuses);
return this;
}
/**
* Get the cloudMetadata property: The metadata of the cloud environment (Azure/GCP/AWS/OCI...).
*
* @return the cloudMetadata value.
*/
public CloudMetadata cloudMetadata() {
return this.innerProperties() == null ? null : this.innerProperties().cloudMetadata();
}
/**
* Set the cloudMetadata property: The metadata of the cloud environment (Azure/GCP/AWS/OCI...).
*
* @param cloudMetadata the cloudMetadata value to set.
* @return the MachineInner object itself.
*/
public MachineInner withCloudMetadata(CloudMetadata cloudMetadata) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withCloudMetadata(cloudMetadata);
return this;
}
/**
* Get the agentUpgrade property: The info of the machine w.r.t Agent Upgrade.
*
* @return the agentUpgrade value.
*/
public AgentUpgrade agentUpgrade() {
return this.innerProperties() == null ? null : this.innerProperties().agentUpgrade();
}
/**
* Set the agentUpgrade property: The info of the machine w.r.t Agent Upgrade.
*
* @param agentUpgrade the agentUpgrade value to set.
* @return the MachineInner object itself.
*/
public MachineInner withAgentUpgrade(AgentUpgrade agentUpgrade) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withAgentUpgrade(agentUpgrade);
return this;
}
/**
* Get the osProfile property: Specifies the operating system settings for the hybrid machine.
*
* @return the osProfile value.
*/
public OSProfile osProfile() {
return this.innerProperties() == null ? null : this.innerProperties().osProfile();
}
/**
* Set the osProfile property: Specifies the operating system settings for the hybrid machine.
*
* @param osProfile the osProfile value to set.
* @return the MachineInner object itself.
*/
public MachineInner withOsProfile(OSProfile osProfile) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withOsProfile(osProfile);
return this;
}
/**
* Get the licenseProfile property: Specifies the License related properties for a machine.
*
* @return the licenseProfile value.
*/
public LicenseProfileMachineInstanceViewInner licenseProfile() {
return this.innerProperties() == null ? null : this.innerProperties().licenseProfile();
}
/**
* Set the licenseProfile property: Specifies the License related properties for a machine.
*
* @param licenseProfile the licenseProfile value to set.
* @return the MachineInner object itself.
*/
public MachineInner withLicenseProfile(LicenseProfileMachineInstanceViewInner licenseProfile) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withLicenseProfile(licenseProfile);
return this;
}
/**
* Get the provisioningState property: The provisioning state, which only appears in the response.
*
* @return the provisioningState value.
*/
public String provisioningState() {
return this.innerProperties() == null ? null : this.innerProperties().provisioningState();
}
/**
* Get the status property: The status of the hybrid machine agent.
*
* @return the status value.
*/
public StatusTypes status() {
return this.innerProperties() == null ? null : this.innerProperties().status();
}
/**
* Get the lastStatusChange property: The time of the last status change.
*
* @return the lastStatusChange value.
*/
public OffsetDateTime lastStatusChange() {
return this.innerProperties() == null ? null : this.innerProperties().lastStatusChange();
}
/**
* Get the errorDetails property: Details about the error state.
*
* @return the errorDetails value.
*/
public List errorDetails() {
return this.innerProperties() == null ? null : this.innerProperties().errorDetails();
}
/**
* Get the agentVersion property: The hybrid machine agent full version.
*
* @return the agentVersion value.
*/
public String agentVersion() {
return this.innerProperties() == null ? null : this.innerProperties().agentVersion();
}
/**
* Get the vmId property: Specifies the hybrid machine unique ID.
*
* @return the vmId value.
*/
public UUID vmId() {
return this.innerProperties() == null ? null : this.innerProperties().vmId();
}
/**
* Set the vmId property: Specifies the hybrid machine unique ID.
*
* @param vmId the vmId value to set.
* @return the MachineInner object itself.
*/
public MachineInner withVmId(UUID vmId) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withVmId(vmId);
return this;
}
/**
* Get the displayName property: Specifies the hybrid machine display name.
*
* @return the displayName value.
*/
public String displayName() {
return this.innerProperties() == null ? null : this.innerProperties().displayName();
}
/**
* Get the machineFqdn property: Specifies the hybrid machine FQDN.
*
* @return the machineFqdn value.
*/
public String machineFqdn() {
return this.innerProperties() == null ? null : this.innerProperties().machineFqdn();
}
/**
* Get the clientPublicKey property: Public Key that the client provides to be used during initial resource
* onboarding.
*
* @return the clientPublicKey value.
*/
public String clientPublicKey() {
return this.innerProperties() == null ? null : this.innerProperties().clientPublicKey();
}
/**
* Set the clientPublicKey property: Public Key that the client provides to be used during initial resource
* onboarding.
*
* @param clientPublicKey the clientPublicKey value to set.
* @return the MachineInner object itself.
*/
public MachineInner withClientPublicKey(String clientPublicKey) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withClientPublicKey(clientPublicKey);
return this;
}
/**
* Get the osName property: The Operating System running on the hybrid machine.
*
* @return the osName value.
*/
public String osName() {
return this.innerProperties() == null ? null : this.innerProperties().osName();
}
/**
* Get the osVersion property: The version of Operating System running on the hybrid machine.
*
* @return the osVersion value.
*/
public String osVersion() {
return this.innerProperties() == null ? null : this.innerProperties().osVersion();
}
/**
* Get the osType property: The type of Operating System (windows/linux).
*
* @return the osType value.
*/
public String osType() {
return this.innerProperties() == null ? null : this.innerProperties().osType();
}
/**
* Set the osType property: The type of Operating System (windows/linux).
*
* @param osType the osType value to set.
* @return the MachineInner object itself.
*/
public MachineInner withOsType(String osType) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withOsType(osType);
return this;
}
/**
* Get the vmUuid property: Specifies the Arc Machine's unique SMBIOS ID.
*
* @return the vmUuid value.
*/
public UUID vmUuid() {
return this.innerProperties() == null ? null : this.innerProperties().vmUuid();
}
/**
* Get the extensions property: Machine Extensions information (deprecated field).
*
* @return the extensions value.
*/
public List extensions() {
return this.innerProperties() == null ? null : this.innerProperties().extensions();
}
/**
* Set the extensions property: Machine Extensions information (deprecated field).
*
* @param extensions the extensions value to set.
* @return the MachineInner object itself.
*/
public MachineInner withExtensions(List extensions) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withExtensions(extensions);
return this;
}
/**
* Get the osSku property: Specifies the Operating System product SKU.
*
* @return the osSku value.
*/
public String osSku() {
return this.innerProperties() == null ? null : this.innerProperties().osSku();
}
/**
* Get the osEdition property: The edition of the Operating System.
*
* @return the osEdition value.
*/
public String osEdition() {
return this.innerProperties() == null ? null : this.innerProperties().osEdition();
}
/**
* Get the domainName property: Specifies the Windows domain name.
*
* @return the domainName value.
*/
public String domainName() {
return this.innerProperties() == null ? null : this.innerProperties().domainName();
}
/**
* Get the adFqdn property: Specifies the AD fully qualified display name.
*
* @return the adFqdn value.
*/
public String adFqdn() {
return this.innerProperties() == null ? null : this.innerProperties().adFqdn();
}
/**
* Get the dnsFqdn property: Specifies the DNS fully qualified display name.
*
* @return the dnsFqdn value.
*/
public String dnsFqdn() {
return this.innerProperties() == null ? null : this.innerProperties().dnsFqdn();
}
/**
* Get the privateLinkScopeResourceId property: The resource id of the private link scope this machine is assigned
* to, if any.
*
* @return the privateLinkScopeResourceId value.
*/
public String privateLinkScopeResourceId() {
return this.innerProperties() == null ? null : this.innerProperties().privateLinkScopeResourceId();
}
/**
* Set the privateLinkScopeResourceId property: The resource id of the private link scope this machine is assigned
* to, if any.
*
* @param privateLinkScopeResourceId the privateLinkScopeResourceId value to set.
* @return the MachineInner object itself.
*/
public MachineInner withPrivateLinkScopeResourceId(String privateLinkScopeResourceId) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withPrivateLinkScopeResourceId(privateLinkScopeResourceId);
return this;
}
/**
* Get the parentClusterResourceId property: The resource id of the parent cluster (Azure HCI) this machine is
* assigned to, if any.
*
* @return the parentClusterResourceId value.
*/
public String parentClusterResourceId() {
return this.innerProperties() == null ? null : this.innerProperties().parentClusterResourceId();
}
/**
* Set the parentClusterResourceId property: The resource id of the parent cluster (Azure HCI) this machine is
* assigned to, if any.
*
* @param parentClusterResourceId the parentClusterResourceId value to set.
* @return the MachineInner object itself.
*/
public MachineInner withParentClusterResourceId(String parentClusterResourceId) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withParentClusterResourceId(parentClusterResourceId);
return this;
}
/**
* Get the mssqlDiscovered property: Specifies whether any MS SQL instance is discovered on the machine.
*
* @return the mssqlDiscovered value.
*/
public String mssqlDiscovered() {
return this.innerProperties() == null ? null : this.innerProperties().mssqlDiscovered();
}
/**
* Set the mssqlDiscovered property: Specifies whether any MS SQL instance is discovered on the machine.
*
* @param mssqlDiscovered the mssqlDiscovered value to set.
* @return the MachineInner object itself.
*/
public MachineInner withMssqlDiscovered(String mssqlDiscovered) {
if (this.innerProperties() == null) {
this.innerProperties = new MachinePropertiesInner();
}
this.innerProperties().withMssqlDiscovered(mssqlDiscovered);
return this;
}
/**
* Get the detectedProperties property: Detected properties from the machine.
*
* @return the detectedProperties value.
*/
public Map detectedProperties() {
return this.innerProperties() == null ? null : this.innerProperties().detectedProperties();
}
/**
* Get the networkProfile property: Information about the network the machine is on.
*
* @return the networkProfile value.
*/
public NetworkProfileInner networkProfile() {
return this.innerProperties() == null ? null : this.innerProperties().networkProfile();
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
if (resources() != null) {
resources().forEach(e -> e.validate());
}
if (identity() != null) {
identity().validate();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy