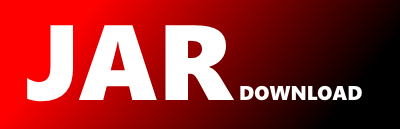
com.azure.resourcemanager.hybridcompute.models.LicenseDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-hybridcompute Show documentation
Show all versions of azure-resourcemanager-hybridcompute Show documentation
This package contains Microsoft Azure SDK for HybridCompute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. The Hybrid Compute Management Client. Package tag package-preview-2024-07.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.hybridcompute.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
/**
* Describes the properties of a License.
*/
@Fluent
public final class LicenseDetails {
/*
* Describes the state of the license.
*/
@JsonProperty(value = "state")
private LicenseState state;
/*
* Describes the license target server.
*/
@JsonProperty(value = "target")
private LicenseTarget target;
/*
* Describes the edition of the license. The values are either Standard or Datacenter.
*/
@JsonProperty(value = "edition")
private LicenseEdition edition;
/*
* Describes the license core type (pCore or vCore).
*/
@JsonProperty(value = "type")
private LicenseCoreType type;
/*
* Describes the number of processors.
*/
@JsonProperty(value = "processors")
private Integer processors;
/*
* Describes the number of assigned licenses.
*/
@JsonProperty(value = "assignedLicenses", access = JsonProperty.Access.WRITE_ONLY)
private Integer assignedLicenses;
/*
* Describes the immutable id.
*/
@JsonProperty(value = "immutableId", access = JsonProperty.Access.WRITE_ONLY)
private String immutableId;
/*
* A list of volume license details.
*/
@JsonProperty(value = "volumeLicenseDetails")
private List volumeLicenseDetails;
/**
* Creates an instance of LicenseDetails class.
*/
public LicenseDetails() {
}
/**
* Get the state property: Describes the state of the license.
*
* @return the state value.
*/
public LicenseState state() {
return this.state;
}
/**
* Set the state property: Describes the state of the license.
*
* @param state the state value to set.
* @return the LicenseDetails object itself.
*/
public LicenseDetails withState(LicenseState state) {
this.state = state;
return this;
}
/**
* Get the target property: Describes the license target server.
*
* @return the target value.
*/
public LicenseTarget target() {
return this.target;
}
/**
* Set the target property: Describes the license target server.
*
* @param target the target value to set.
* @return the LicenseDetails object itself.
*/
public LicenseDetails withTarget(LicenseTarget target) {
this.target = target;
return this;
}
/**
* Get the edition property: Describes the edition of the license. The values are either Standard or Datacenter.
*
* @return the edition value.
*/
public LicenseEdition edition() {
return this.edition;
}
/**
* Set the edition property: Describes the edition of the license. The values are either Standard or Datacenter.
*
* @param edition the edition value to set.
* @return the LicenseDetails object itself.
*/
public LicenseDetails withEdition(LicenseEdition edition) {
this.edition = edition;
return this;
}
/**
* Get the type property: Describes the license core type (pCore or vCore).
*
* @return the type value.
*/
public LicenseCoreType type() {
return this.type;
}
/**
* Set the type property: Describes the license core type (pCore or vCore).
*
* @param type the type value to set.
* @return the LicenseDetails object itself.
*/
public LicenseDetails withType(LicenseCoreType type) {
this.type = type;
return this;
}
/**
* Get the processors property: Describes the number of processors.
*
* @return the processors value.
*/
public Integer processors() {
return this.processors;
}
/**
* Set the processors property: Describes the number of processors.
*
* @param processors the processors value to set.
* @return the LicenseDetails object itself.
*/
public LicenseDetails withProcessors(Integer processors) {
this.processors = processors;
return this;
}
/**
* Get the assignedLicenses property: Describes the number of assigned licenses.
*
* @return the assignedLicenses value.
*/
public Integer assignedLicenses() {
return this.assignedLicenses;
}
/**
* Get the immutableId property: Describes the immutable id.
*
* @return the immutableId value.
*/
public String immutableId() {
return this.immutableId;
}
/**
* Get the volumeLicenseDetails property: A list of volume license details.
*
* @return the volumeLicenseDetails value.
*/
public List volumeLicenseDetails() {
return this.volumeLicenseDetails;
}
/**
* Set the volumeLicenseDetails property: A list of volume license details.
*
* @param volumeLicenseDetails the volumeLicenseDetails value to set.
* @return the LicenseDetails object itself.
*/
public LicenseDetails withVolumeLicenseDetails(List volumeLicenseDetails) {
this.volumeLicenseDetails = volumeLicenseDetails;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (volumeLicenseDetails() != null) {
volumeLicenseDetails().forEach(e -> e.validate());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy