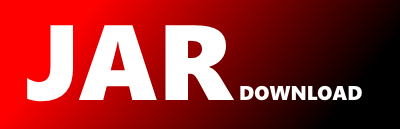
com.azure.resourcemanager.hybridcompute.models.Disk Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-hybridcompute Show documentation
Show all versions of azure-resourcemanager-hybridcompute Show documentation
This package contains Microsoft Azure SDK for HybridCompute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. The Hybrid Compute Management Client. Package tag package-preview-2024-07.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.hybridcompute.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Describes a disk on the machine.
*/
@Fluent
public final class Disk implements JsonSerializable {
/*
* The path of the disk.
*/
private String path;
/*
* The type of the disk.
*/
private String diskType;
/*
* The generated ID of the disk.
*/
private String generatedId;
/*
* The ID of the disk.
*/
private String id;
/*
* The name of the disk.
*/
private String name;
/*
* The size of the disk, in bytes
*/
private Long maxSizeInBytes;
/*
* The amount of space used on the disk, in bytes
*/
private Long usedSpaceInBytes;
/**
* Creates an instance of Disk class.
*/
public Disk() {
}
/**
* Get the path property: The path of the disk.
*
* @return the path value.
*/
public String path() {
return this.path;
}
/**
* Set the path property: The path of the disk.
*
* @param path the path value to set.
* @return the Disk object itself.
*/
public Disk withPath(String path) {
this.path = path;
return this;
}
/**
* Get the diskType property: The type of the disk.
*
* @return the diskType value.
*/
public String diskType() {
return this.diskType;
}
/**
* Set the diskType property: The type of the disk.
*
* @param diskType the diskType value to set.
* @return the Disk object itself.
*/
public Disk withDiskType(String diskType) {
this.diskType = diskType;
return this;
}
/**
* Get the generatedId property: The generated ID of the disk.
*
* @return the generatedId value.
*/
public String generatedId() {
return this.generatedId;
}
/**
* Set the generatedId property: The generated ID of the disk.
*
* @param generatedId the generatedId value to set.
* @return the Disk object itself.
*/
public Disk withGeneratedId(String generatedId) {
this.generatedId = generatedId;
return this;
}
/**
* Get the id property: The ID of the disk.
*
* @return the id value.
*/
public String id() {
return this.id;
}
/**
* Set the id property: The ID of the disk.
*
* @param id the id value to set.
* @return the Disk object itself.
*/
public Disk withId(String id) {
this.id = id;
return this;
}
/**
* Get the name property: The name of the disk.
*
* @return the name value.
*/
public String name() {
return this.name;
}
/**
* Set the name property: The name of the disk.
*
* @param name the name value to set.
* @return the Disk object itself.
*/
public Disk withName(String name) {
this.name = name;
return this;
}
/**
* Get the maxSizeInBytes property: The size of the disk, in bytes.
*
* @return the maxSizeInBytes value.
*/
public Long maxSizeInBytes() {
return this.maxSizeInBytes;
}
/**
* Set the maxSizeInBytes property: The size of the disk, in bytes.
*
* @param maxSizeInBytes the maxSizeInBytes value to set.
* @return the Disk object itself.
*/
public Disk withMaxSizeInBytes(Long maxSizeInBytes) {
this.maxSizeInBytes = maxSizeInBytes;
return this;
}
/**
* Get the usedSpaceInBytes property: The amount of space used on the disk, in bytes.
*
* @return the usedSpaceInBytes value.
*/
public Long usedSpaceInBytes() {
return this.usedSpaceInBytes;
}
/**
* Set the usedSpaceInBytes property: The amount of space used on the disk, in bytes.
*
* @param usedSpaceInBytes the usedSpaceInBytes value to set.
* @return the Disk object itself.
*/
public Disk withUsedSpaceInBytes(Long usedSpaceInBytes) {
this.usedSpaceInBytes = usedSpaceInBytes;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("path", this.path);
jsonWriter.writeStringField("diskType", this.diskType);
jsonWriter.writeStringField("generatedId", this.generatedId);
jsonWriter.writeStringField("id", this.id);
jsonWriter.writeStringField("name", this.name);
jsonWriter.writeNumberField("maxSizeInBytes", this.maxSizeInBytes);
jsonWriter.writeNumberField("usedSpaceInBytes", this.usedSpaceInBytes);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Disk from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Disk if the JsonReader was pointing to an instance of it, or null if it was pointing to
* JSON null.
* @throws IOException If an error occurs while reading the Disk.
*/
public static Disk fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Disk deserializedDisk = new Disk();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("path".equals(fieldName)) {
deserializedDisk.path = reader.getString();
} else if ("diskType".equals(fieldName)) {
deserializedDisk.diskType = reader.getString();
} else if ("generatedId".equals(fieldName)) {
deserializedDisk.generatedId = reader.getString();
} else if ("id".equals(fieldName)) {
deserializedDisk.id = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedDisk.name = reader.getString();
} else if ("maxSizeInBytes".equals(fieldName)) {
deserializedDisk.maxSizeInBytes = reader.getNullable(JsonReader::getLong);
} else if ("usedSpaceInBytes".equals(fieldName)) {
deserializedDisk.usedSpaceInBytes = reader.getNullable(JsonReader::getLong);
} else {
reader.skipChildren();
}
}
return deserializedDisk;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy