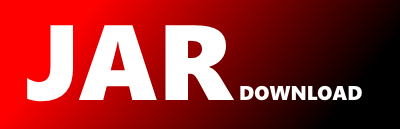
com.azure.resourcemanager.hybridcompute.models.MachineRunCommandInstanceView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-hybridcompute Show documentation
Show all versions of azure-resourcemanager-hybridcompute Show documentation
This package contains Microsoft Azure SDK for HybridCompute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. The Hybrid Compute Management Client. Package tag package-preview-2024-07.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.hybridcompute.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.List;
/**
* The instance view of a machine run command.
*/
@Fluent
public final class MachineRunCommandInstanceView implements JsonSerializable {
/*
* Script execution status.
*/
private ExecutionState executionState;
/*
* Communicate script configuration errors or execution messages.
*/
private String executionMessage;
/*
* Exit code returned from script execution.
*/
private Integer exitCode;
/*
* Script output stream.
*/
private String output;
/*
* Script error stream.
*/
private String error;
/*
* Script start time.
*/
private OffsetDateTime startTime;
/*
* Script end time.
*/
private OffsetDateTime endTime;
/*
* The status information.
*/
private List statuses;
/**
* Creates an instance of MachineRunCommandInstanceView class.
*/
public MachineRunCommandInstanceView() {
}
/**
* Get the executionState property: Script execution status.
*
* @return the executionState value.
*/
public ExecutionState executionState() {
return this.executionState;
}
/**
* Set the executionState property: Script execution status.
*
* @param executionState the executionState value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withExecutionState(ExecutionState executionState) {
this.executionState = executionState;
return this;
}
/**
* Get the executionMessage property: Communicate script configuration errors or execution messages.
*
* @return the executionMessage value.
*/
public String executionMessage() {
return this.executionMessage;
}
/**
* Set the executionMessage property: Communicate script configuration errors or execution messages.
*
* @param executionMessage the executionMessage value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withExecutionMessage(String executionMessage) {
this.executionMessage = executionMessage;
return this;
}
/**
* Get the exitCode property: Exit code returned from script execution.
*
* @return the exitCode value.
*/
public Integer exitCode() {
return this.exitCode;
}
/**
* Set the exitCode property: Exit code returned from script execution.
*
* @param exitCode the exitCode value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withExitCode(Integer exitCode) {
this.exitCode = exitCode;
return this;
}
/**
* Get the output property: Script output stream.
*
* @return the output value.
*/
public String output() {
return this.output;
}
/**
* Set the output property: Script output stream.
*
* @param output the output value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withOutput(String output) {
this.output = output;
return this;
}
/**
* Get the error property: Script error stream.
*
* @return the error value.
*/
public String error() {
return this.error;
}
/**
* Set the error property: Script error stream.
*
* @param error the error value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withError(String error) {
this.error = error;
return this;
}
/**
* Get the startTime property: Script start time.
*
* @return the startTime value.
*/
public OffsetDateTime startTime() {
return this.startTime;
}
/**
* Set the startTime property: Script start time.
*
* @param startTime the startTime value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withStartTime(OffsetDateTime startTime) {
this.startTime = startTime;
return this;
}
/**
* Get the endTime property: Script end time.
*
* @return the endTime value.
*/
public OffsetDateTime endTime() {
return this.endTime;
}
/**
* Set the endTime property: Script end time.
*
* @param endTime the endTime value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withEndTime(OffsetDateTime endTime) {
this.endTime = endTime;
return this;
}
/**
* Get the statuses property: The status information.
*
* @return the statuses value.
*/
public List statuses() {
return this.statuses;
}
/**
* Set the statuses property: The status information.
*
* @param statuses the statuses value to set.
* @return the MachineRunCommandInstanceView object itself.
*/
public MachineRunCommandInstanceView withStatuses(List statuses) {
this.statuses = statuses;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (statuses() != null) {
statuses().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("executionState",
this.executionState == null ? null : this.executionState.toString());
jsonWriter.writeStringField("executionMessage", this.executionMessage);
jsonWriter.writeNumberField("exitCode", this.exitCode);
jsonWriter.writeStringField("output", this.output);
jsonWriter.writeStringField("error", this.error);
jsonWriter.writeStringField("startTime",
this.startTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.startTime));
jsonWriter.writeStringField("endTime",
this.endTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.endTime));
jsonWriter.writeArrayField("statuses", this.statuses, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MachineRunCommandInstanceView from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MachineRunCommandInstanceView if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MachineRunCommandInstanceView.
*/
public static MachineRunCommandInstanceView fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MachineRunCommandInstanceView deserializedMachineRunCommandInstanceView
= new MachineRunCommandInstanceView();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("executionState".equals(fieldName)) {
deserializedMachineRunCommandInstanceView.executionState
= ExecutionState.fromString(reader.getString());
} else if ("executionMessage".equals(fieldName)) {
deserializedMachineRunCommandInstanceView.executionMessage = reader.getString();
} else if ("exitCode".equals(fieldName)) {
deserializedMachineRunCommandInstanceView.exitCode = reader.getNullable(JsonReader::getInt);
} else if ("output".equals(fieldName)) {
deserializedMachineRunCommandInstanceView.output = reader.getString();
} else if ("error".equals(fieldName)) {
deserializedMachineRunCommandInstanceView.error = reader.getString();
} else if ("startTime".equals(fieldName)) {
deserializedMachineRunCommandInstanceView.startTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("endTime".equals(fieldName)) {
deserializedMachineRunCommandInstanceView.endTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("statuses".equals(fieldName)) {
List statuses
= reader.readArray(reader1 -> ExtensionsResourceStatus.fromJson(reader1));
deserializedMachineRunCommandInstanceView.statuses = statuses;
} else {
reader.skipChildren();
}
}
return deserializedMachineRunCommandInstanceView;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy