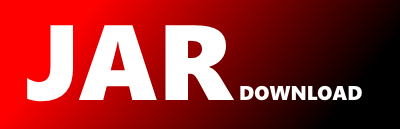
com.azure.resourcemanager.keyvault.models.AccessPolicy Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.keyvault.models;
import com.azure.core.annotation.Fluent;
import com.azure.resourcemanager.authorization.models.ActiveDirectoryGroup;
import com.azure.resourcemanager.authorization.models.ActiveDirectoryUser;
import com.azure.resourcemanager.authorization.models.ServicePrincipal;
import com.azure.resourcemanager.resources.fluentcore.arm.models.ChildResource;
import com.azure.resourcemanager.resources.fluentcore.model.Attachable;
import com.azure.resourcemanager.resources.fluentcore.model.HasInnerModel;
import com.azure.resourcemanager.resources.fluentcore.model.Settable;
import java.util.List;
/** An immutable client-side representation of a key vault access policy. */
@Fluent
public interface AccessPolicy extends ChildResource, HasInnerModel {
/**
* @return The Azure Active Directory tenant ID that should be used for authenticating requests to the key vault.
*/
String tenantId();
/** @return The object ID of a user or service principal in the Azure Active Directory tenant for the vault. */
String objectId();
/** @return Application ID of the client making request on behalf of a principal. */
String applicationId();
/** @return Permissions the identity has for keys and secrets. */
Permissions permissions();
/**************************************************************
* Fluent interfaces to attach an access policy
**************************************************************/
/**
* The entirety of an access policy definition.
*
* @param the return type of the final {@link Attachable#attach()}
*/
interface Definition extends DefinitionStages.Blank, DefinitionStages.WithAttach {
}
/** Grouping of access policy definition stages applicable as part of a key vault creation. */
interface DefinitionStages {
/**
* The first stage of an access policy definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends WithIdentity {
}
/**
* The access policy definition stage allowing the Active Directory identity to be specified.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithIdentity {
/**
* Specifies the object ID of the Active Directory identity this access policy is for.
*
* @param objectId the object ID of the AD identity
* @return the next stage of access policy definition
*/
WithAttach forObjectId(String objectId);
/**
* Specifies the Active Directory user this access policy is for.
*
* @param user the AD user object
* @return the next stage of access policy definition
*/
WithAttach forUser(ActiveDirectoryUser user);
/**
* Specifies the Active Directory user this access policy is for.
*
* @param userPrincipalName the user principal name of the AD user
* @return the next stage of access policy definition
*/
WithAttach forUser(String userPrincipalName);
/**
* Application ID of the client making request on behalf of a principal.
*
* @param applicationId the application ID
* @return the next stage of access policy definition
*/
WithAttach forApplicationId(String applicationId);
/**
* Specifies the Azure Active Directory tenant ID that should be used for authenticating requests to the key
* vault.
*
* @param tenantId the tenant ID for the key vault.
* @return the next stage of access policy definition
*/
WithAttach forTenantId(String tenantId);
/**
* Specifies the Active Directory group this access policy is for.
*
* @param activeDirectoryGroup the AD group object
* @return the next stage of access policy definition
*/
WithAttach forGroup(ActiveDirectoryGroup activeDirectoryGroup);
/**
* Specifies the Active Directory service principal this access policy is for.
*
* @param servicePrincipal the AD service principal object
* @return the next stage of access policy definition
*/
WithAttach forServicePrincipal(ServicePrincipal servicePrincipal);
/**
* Specifies the Active Directory service principal this access policy is for.
*
* @param servicePrincipalName the service principal name of the AD user
* @return the next stage of access policy definition
*/
WithAttach forServicePrincipal(String servicePrincipalName);
}
/**
* The access policy definition stage allowing permissions to be added.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithPermissions {
/**
* Allow all permissions for the AD identity to access keys.
*
* @return the next stage of access policy definition
*/
WithAttach allowKeyAllPermissions();
/**
* Allow a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowKeyPermissions(KeyPermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowKeyPermissions(List permissions);
/**
* Allow all permissions for the AD identity to access secrets.
*
* @return the next stage of access policy definition
*/
WithAttach allowSecretAllPermissions();
/**
* Allow a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowSecretPermissions(SecretPermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowSecretPermissions(List permissions);
/**
* Allow all permissions for the AD identity to access certificates.
*
* @return the next stage of access policy definition
*/
WithAttach allowCertificateAllPermissions();
/**
* Allow a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowCertificatePermissions(CertificatePermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowCertificatePermissions(List permissions);
/**
* Allow all permissions for the Ad identity to access storage.
*
* @return the next stage of access policy definition
*/
WithAttach allowStorageAllPermissions();
/**
* Allow a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowStoragePermissions(StoragePermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowStoragePermissions(List permissions);
}
/**
* The final stage of the access policy definition.
*
* At this stage, more permissions can be added or application ID can be specified, or the access policy
* definition can be attached to the parent key vault definition using {@link WithAttach#attach()}.
*
* @param the return type of {@link WithAttach#attach()}
*/
interface WithAttach extends Attachable.InUpdate, WithPermissions {
}
}
/**
* The entirety of an access policy definition as part of a key vault update.
*
* @param the return type of the final {@link UpdateDefinitionStages.WithAttach#attach()}
*/
interface UpdateDefinition
extends UpdateDefinitionStages.Blank, UpdateDefinitionStages.WithAttach {
}
/** Grouping of access policy definition stages applicable as part of a key vault update. */
interface UpdateDefinitionStages {
/**
* The first stage of an access policy definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends WithIdentity {
}
/**
* The access policy definition stage allowing the Active Directory identity to be specified.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithIdentity {
/**
* Specifies the object ID of the Active Directory identity this access policy is for.
*
* @param objectId the object ID of the AD identity
* @return the next stage of access policy definition
*/
WithAttach forObjectId(String objectId);
/**
* Specifies the Active Directory user this access policy is for.
*
* @param user the AD user object
* @return the next stage of access policy definition
*/
WithAttach forUser(ActiveDirectoryUser user);
/**
* Specifies the Active Directory user this access policy is for.
*
* @param userPrincipalName the user principal name of the AD user
* @return the next stage of access policy definition
*/
WithAttach forUser(String userPrincipalName);
/**
* Application ID of the client making request on behalf of a principal.
*
* @param applicationId the application ID
* @return the next stage of access policy definition
*/
WithAttach forApplicationId(String applicationId);
/**
* Specifies the Azure Active Directory tenant ID that should be used for authenticating requests to the key
* vault.
*
* @param tenantId the tenant ID for the key vault.
* @return the next stage of access policy definition
*/
WithAttach forTenantId(String tenantId);
/**
* Specifies the Active Directory group this access policy is for.
*
* @param activeDirectoryGroup the AD group object
* @return the next stage of access policy definition
*/
WithAttach forGroup(ActiveDirectoryGroup activeDirectoryGroup);
/**
* Specifies the Active Directory service principal this access policy is for.
*
* @param servicePrincipal the AD service principal object
* @return the next stage of access policy definition
*/
WithAttach forServicePrincipal(ServicePrincipal servicePrincipal);
/**
* Specifies the Active Directory service principal this access policy is for.
*
* @param servicePrincipalName the service principal name of the AD user
* @return the next stage of access policy definition
*/
WithAttach forServicePrincipal(String servicePrincipalName);
}
/**
* The access policy definition stage allowing permissions to be added.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithPermissions {
/**
* Allow all permissions for the AD identity to access keys.
*
* @return the next stage of access policy definition
*/
WithAttach allowKeyAllPermissions();
/**
* Allow a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowKeyPermissions(KeyPermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowKeyPermissions(List permissions);
/**
* Allow all permissions for the AD identity to access secrets.
*
* @return the next stage of access policy definition
*/
WithAttach allowSecretAllPermissions();
/**
* Allow a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowSecretPermissions(SecretPermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowSecretPermissions(List permissions);
/**
* Allow all permissions for the Ad identity to access storage.
*
* @return the next stage of access policy definition
*/
WithAttach allowStorageAllPermissions();
/**
* Allow all permissions for the AD identity to access certificates.
*
* @return the next stage of access policy definition
*/
WithAttach allowCertificateAllPermissions();
/**
* Allow a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowCertificatePermissions(CertificatePermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowCertificatePermissions(List permissions);
/**
* Allow a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowStoragePermissions(StoragePermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
WithAttach allowStoragePermissions(List permissions);
}
/**
* The final stage of the access policy definition.
*
* At this stage, more permissions can be added or application ID can be specified, or the access policy
* definition can be attached to the parent key vault update using {@link WithAttach#attach()}.
*
* @param the return type of {@link WithAttach#attach()}
*/
interface WithAttach extends Attachable.InDefinition, WithPermissions {
}
}
/** Grouping of all the key vault update stages. */
interface UpdateStages {
/** The access policy update stage allowing permissions to be added or removed. */
interface WithPermissions {
/**
* Allow all permissions for the AD identity to access keys.
*
* @return the next stage of access policy update
*/
Update allowKeyAllPermissions();
/**
* Allow a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy update
*/
Update allowKeyPermissions(KeyPermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy update
*/
Update allowKeyPermissions(List permissions);
/**
* Revoke all permissions for the AD identity to access keys.
*
* @return the next stage of access policy update
*/
Update disallowKeyAllPermissions();
/**
* Revoke a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions to revoke
* @return the next stage of access policy update
*/
Update disallowKeyPermissions(KeyPermissions... permissions);
/**
* Revoke a list of permissions for the AD identity to access keys.
*
* @param permissions the list of permissions to revoke
* @return the next stage of access policy update
*/
Update disallowKeyPermissions(List permissions);
/**
* Allow all permissions for the AD identity to access secrets.
*
* @return the next stage of access policy definition
*/
Update allowSecretAllPermissions();
/**
* Allow a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
Update allowSecretPermissions(SecretPermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
Update allowSecretPermissions(List permissions);
/**
* Revoke all permissions for the AD identity to access secrets.
*
* @return the next stage of access policy update
*/
Update disallowSecretAllPermissions();
/**
* Revoke a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions to revoke
* @return the next stage of access policy update
*/
Update disallowSecretPermissions(SecretPermissions... permissions);
/**
* Revoke a list of permissions for the AD identity to access secrets.
*
* @param permissions the list of permissions to revoke
* @return the next stage of access policy update
*/
Update disallowSecretPermissions(List permissions);
/**
* Allow all permissions for the AD identity to access certificates.
*
* @return the next stage of access policy update
*/
Update allowCertificateAllPermissions();
/**
* Allow a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy update
*/
Update allowCertificatePermissions(CertificatePermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy update
*/
Update allowCertificatePermissions(List permissions);
/**
* Revoke all permissions for the AD identity to access certificates.
*
* @return the next stage of access policy update
*/
Update disallowCertificateAllPermissions();
/**
* Revoke a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions to revoke
* @return the next stage of access policy update
*/
Update disallowCertificatePermissions(CertificatePermissions... permissions);
/**
* Revoke a list of permissions for the AD identity to access certificates.
*
* @param permissions the list of permissions to revoke
* @return the next stage of access policy update
*/
Update disallowCertificatePermissions(List permissions);
/**
* Allow all permissions for the Ad identity to access storage.
*
* @return the next stage of access policy definition
*/
Update allowStorageAllPermissions();
/**
* Allow a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
Update allowStoragePermissions(StoragePermissions... permissions);
/**
* Allow a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
Update allowStoragePermissions(List permissions);
/**
* Revoke all permissions for the Ad identity to access storage.
*
* @return the next stage of access policy definition
*/
Update disallowStorageAllPermissions();
/**
* Revoke a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
Update disallowStoragePermissions(StoragePermissions... permissions);
/**
* Revoke a list of permissions for the AD identity to access storage.
*
* @param permissions the list of permissions allowed
* @return the next stage of access policy definition
*/
Update disallowStoragePermissions(List permissions);
}
}
/** The entirety of an access policy update as part of a key vault update. */
interface Update extends UpdateStages.WithPermissions, Settable {
}
}