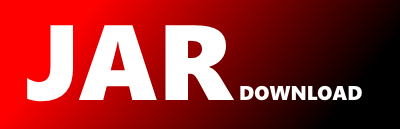
com.azure.resourcemanager.managementgroups.models.ManagementGroupDetails Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.managementgroups.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.List;
/** The details of a management group. */
@Fluent
public final class ManagementGroupDetails {
/*
* The version number of the object.
*/
@JsonProperty(value = "version")
private Integer version;
/*
* The date and time when this object was last updated.
*/
@JsonProperty(value = "updatedTime")
private OffsetDateTime updatedTime;
/*
* The identity of the principal or process that updated the object.
*/
@JsonProperty(value = "updatedBy")
private String updatedBy;
/*
* (Optional) The ID of the parent management group.
*/
@JsonProperty(value = "parent")
private ParentGroupInfo parent;
/*
* The path from the root to the current group.
*/
@JsonProperty(value = "path")
private List path;
/*
* The ancestors of the management group.
*/
@JsonProperty(value = "managementGroupAncestors")
private List managementGroupAncestors;
/*
* The ancestors of the management group displayed in reversed order, from immediate parent to the root.
*/
@JsonProperty(value = "managementGroupAncestorsChain")
private List managementGroupAncestorsChain;
/** Creates an instance of ManagementGroupDetails class. */
public ManagementGroupDetails() {
}
/**
* Get the version property: The version number of the object.
*
* @return the version value.
*/
public Integer version() {
return this.version;
}
/**
* Set the version property: The version number of the object.
*
* @param version the version value to set.
* @return the ManagementGroupDetails object itself.
*/
public ManagementGroupDetails withVersion(Integer version) {
this.version = version;
return this;
}
/**
* Get the updatedTime property: The date and time when this object was last updated.
*
* @return the updatedTime value.
*/
public OffsetDateTime updatedTime() {
return this.updatedTime;
}
/**
* Set the updatedTime property: The date and time when this object was last updated.
*
* @param updatedTime the updatedTime value to set.
* @return the ManagementGroupDetails object itself.
*/
public ManagementGroupDetails withUpdatedTime(OffsetDateTime updatedTime) {
this.updatedTime = updatedTime;
return this;
}
/**
* Get the updatedBy property: The identity of the principal or process that updated the object.
*
* @return the updatedBy value.
*/
public String updatedBy() {
return this.updatedBy;
}
/**
* Set the updatedBy property: The identity of the principal or process that updated the object.
*
* @param updatedBy the updatedBy value to set.
* @return the ManagementGroupDetails object itself.
*/
public ManagementGroupDetails withUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
/**
* Get the parent property: (Optional) The ID of the parent management group.
*
* @return the parent value.
*/
public ParentGroupInfo parent() {
return this.parent;
}
/**
* Set the parent property: (Optional) The ID of the parent management group.
*
* @param parent the parent value to set.
* @return the ManagementGroupDetails object itself.
*/
public ManagementGroupDetails withParent(ParentGroupInfo parent) {
this.parent = parent;
return this;
}
/**
* Get the path property: The path from the root to the current group.
*
* @return the path value.
*/
public List path() {
return this.path;
}
/**
* Set the path property: The path from the root to the current group.
*
* @param path the path value to set.
* @return the ManagementGroupDetails object itself.
*/
public ManagementGroupDetails withPath(List path) {
this.path = path;
return this;
}
/**
* Get the managementGroupAncestors property: The ancestors of the management group.
*
* @return the managementGroupAncestors value.
*/
public List managementGroupAncestors() {
return this.managementGroupAncestors;
}
/**
* Set the managementGroupAncestors property: The ancestors of the management group.
*
* @param managementGroupAncestors the managementGroupAncestors value to set.
* @return the ManagementGroupDetails object itself.
*/
public ManagementGroupDetails withManagementGroupAncestors(List managementGroupAncestors) {
this.managementGroupAncestors = managementGroupAncestors;
return this;
}
/**
* Get the managementGroupAncestorsChain property: The ancestors of the management group displayed in reversed
* order, from immediate parent to the root.
*
* @return the managementGroupAncestorsChain value.
*/
public List managementGroupAncestorsChain() {
return this.managementGroupAncestorsChain;
}
/**
* Set the managementGroupAncestorsChain property: The ancestors of the management group displayed in reversed
* order, from immediate parent to the root.
*
* @param managementGroupAncestorsChain the managementGroupAncestorsChain value to set.
* @return the ManagementGroupDetails object itself.
*/
public ManagementGroupDetails withManagementGroupAncestorsChain(
List managementGroupAncestorsChain) {
this.managementGroupAncestorsChain = managementGroupAncestorsChain;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (parent() != null) {
parent().validate();
}
if (path() != null) {
path().forEach(e -> e.validate());
}
if (managementGroupAncestorsChain() != null) {
managementGroupAncestorsChain().forEach(e -> e.validate());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy