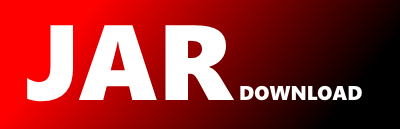
com.azure.resourcemanager.mediaservices.fluent.MediaservicesClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.mediaservices.fluent.models.EdgePoliciesInner;
import com.azure.resourcemanager.mediaservices.fluent.models.MediaServiceInner;
import com.azure.resourcemanager.mediaservices.models.ListEdgePoliciesInput;
import com.azure.resourcemanager.mediaservices.models.MediaServiceUpdate;
import com.azure.resourcemanager.mediaservices.models.SyncStorageKeysInput;
/** An instance of this class provides access to all the operations defined in MediaservicesClient. */
public interface MediaservicesClient {
/**
* List Media Services accounts in the resource group.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a collection of MediaService items as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByResourceGroup(String resourceGroupName);
/**
* List Media Services accounts in the resource group.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a collection of MediaService items as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByResourceGroup(String resourceGroupName, Context context);
/**
* Get the details of a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the details of a Media Services account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MediaServiceInner getByResourceGroup(String resourceGroupName, String accountName);
/**
* Get the details of a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the details of a Media Services account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getByResourceGroupWithResponse(
String resourceGroupName, String accountName, Context context);
/**
* Creates or updates a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of a Media Services account.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MediaServiceInner> beginCreateOrUpdate(
String resourceGroupName, String accountName, MediaServiceInner parameters);
/**
* Creates or updates a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of a Media Services account.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MediaServiceInner> beginCreateOrUpdate(
String resourceGroupName, String accountName, MediaServiceInner parameters, Context context);
/**
* Creates or updates a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a Media Services account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MediaServiceInner createOrUpdate(String resourceGroupName, String accountName, MediaServiceInner parameters);
/**
* Creates or updates a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a Media Services account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MediaServiceInner createOrUpdate(
String resourceGroupName, String accountName, MediaServiceInner parameters, Context context);
/**
* Deletes a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String accountName);
/**
* Deletes a Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response deleteWithResponse(String resourceGroupName, String accountName, Context context);
/**
* Updates an existing Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of a Media Services account.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MediaServiceInner> beginUpdate(
String resourceGroupName, String accountName, MediaServiceUpdate parameters);
/**
* Updates an existing Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of a Media Services account.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MediaServiceInner> beginUpdate(
String resourceGroupName, String accountName, MediaServiceUpdate parameters, Context context);
/**
* Updates an existing Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a Media Services account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MediaServiceInner update(String resourceGroupName, String accountName, MediaServiceUpdate parameters);
/**
* Updates an existing Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a Media Services account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MediaServiceInner update(
String resourceGroupName, String accountName, MediaServiceUpdate parameters, Context context);
/**
* Synchronizes storage account keys for a storage account associated with the Media Service account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void syncStorageKeys(String resourceGroupName, String accountName, SyncStorageKeysInput parameters);
/**
* Synchronizes storage account keys for a storage account associated with the Media Service account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response syncStorageKeysWithResponse(
String resourceGroupName, String accountName, SyncStorageKeysInput parameters, Context context);
/**
* List all the media edge policies associated with the Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
EdgePoliciesInner listEdgePolicies(String resourceGroupName, String accountName, ListEdgePoliciesInput parameters);
/**
* List all the media edge policies associated with the Media Services account.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param parameters The request parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response listEdgePoliciesWithResponse(
String resourceGroupName, String accountName, ListEdgePoliciesInput parameters, Context context);
/**
* List Media Services accounts in the subscription.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a collection of MediaService items as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list();
/**
* List Media Services accounts in the subscription.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a collection of MediaService items as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(Context context);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy