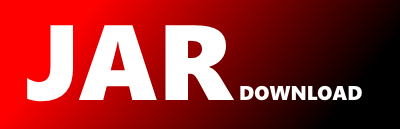
com.azure.resourcemanager.mediaservices.fluent.models.MediaServiceProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.resourcemanager.mediaservices.models.AccountEncryption;
import com.azure.resourcemanager.mediaservices.models.KeyDelivery;
import com.azure.resourcemanager.mediaservices.models.ProvisioningState;
import com.azure.resourcemanager.mediaservices.models.PublicNetworkAccess;
import com.azure.resourcemanager.mediaservices.models.StorageAccount;
import com.azure.resourcemanager.mediaservices.models.StorageAuthentication;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import java.util.UUID;
/** Properties of the Media Services account. */
@Fluent
public final class MediaServiceProperties {
/*
* The Media Services account ID.
*/
@JsonProperty(value = "mediaServiceId", access = JsonProperty.Access.WRITE_ONLY)
private UUID mediaServiceId;
/*
* The storage accounts for this resource.
*/
@JsonProperty(value = "storageAccounts")
private List storageAccounts;
/*
* The storageAuthentication property.
*/
@JsonProperty(value = "storageAuthentication")
private StorageAuthentication storageAuthentication;
/*
* The account encryption properties.
*/
@JsonProperty(value = "encryption")
private AccountEncryption encryption;
/*
* The Key Delivery properties for Media Services account.
*/
@JsonProperty(value = "keyDelivery")
private KeyDelivery keyDelivery;
/*
* Whether or not public network access is allowed for resources under the
* Media Services account.
*/
@JsonProperty(value = "publicNetworkAccess")
private PublicNetworkAccess publicNetworkAccess;
/*
* Provisioning state of the Media Services account.
*/
@JsonProperty(value = "provisioningState", access = JsonProperty.Access.WRITE_ONLY)
private ProvisioningState provisioningState;
/*
* The Private Endpoint Connections created for the Media Service account.
*/
@JsonProperty(value = "privateEndpointConnections", access = JsonProperty.Access.WRITE_ONLY)
private List privateEndpointConnections;
/**
* Get the mediaServiceId property: The Media Services account ID.
*
* @return the mediaServiceId value.
*/
public UUID mediaServiceId() {
return this.mediaServiceId;
}
/**
* Get the storageAccounts property: The storage accounts for this resource.
*
* @return the storageAccounts value.
*/
public List storageAccounts() {
return this.storageAccounts;
}
/**
* Set the storageAccounts property: The storage accounts for this resource.
*
* @param storageAccounts the storageAccounts value to set.
* @return the MediaServiceProperties object itself.
*/
public MediaServiceProperties withStorageAccounts(List storageAccounts) {
this.storageAccounts = storageAccounts;
return this;
}
/**
* Get the storageAuthentication property: The storageAuthentication property.
*
* @return the storageAuthentication value.
*/
public StorageAuthentication storageAuthentication() {
return this.storageAuthentication;
}
/**
* Set the storageAuthentication property: The storageAuthentication property.
*
* @param storageAuthentication the storageAuthentication value to set.
* @return the MediaServiceProperties object itself.
*/
public MediaServiceProperties withStorageAuthentication(StorageAuthentication storageAuthentication) {
this.storageAuthentication = storageAuthentication;
return this;
}
/**
* Get the encryption property: The account encryption properties.
*
* @return the encryption value.
*/
public AccountEncryption encryption() {
return this.encryption;
}
/**
* Set the encryption property: The account encryption properties.
*
* @param encryption the encryption value to set.
* @return the MediaServiceProperties object itself.
*/
public MediaServiceProperties withEncryption(AccountEncryption encryption) {
this.encryption = encryption;
return this;
}
/**
* Get the keyDelivery property: The Key Delivery properties for Media Services account.
*
* @return the keyDelivery value.
*/
public KeyDelivery keyDelivery() {
return this.keyDelivery;
}
/**
* Set the keyDelivery property: The Key Delivery properties for Media Services account.
*
* @param keyDelivery the keyDelivery value to set.
* @return the MediaServiceProperties object itself.
*/
public MediaServiceProperties withKeyDelivery(KeyDelivery keyDelivery) {
this.keyDelivery = keyDelivery;
return this;
}
/**
* Get the publicNetworkAccess property: Whether or not public network access is allowed for resources under the
* Media Services account.
*
* @return the publicNetworkAccess value.
*/
public PublicNetworkAccess publicNetworkAccess() {
return this.publicNetworkAccess;
}
/**
* Set the publicNetworkAccess property: Whether or not public network access is allowed for resources under the
* Media Services account.
*
* @param publicNetworkAccess the publicNetworkAccess value to set.
* @return the MediaServiceProperties object itself.
*/
public MediaServiceProperties withPublicNetworkAccess(PublicNetworkAccess publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
/**
* Get the provisioningState property: Provisioning state of the Media Services account.
*
* @return the provisioningState value.
*/
public ProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Get the privateEndpointConnections property: The Private Endpoint Connections created for the Media Service
* account.
*
* @return the privateEndpointConnections value.
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (storageAccounts() != null) {
storageAccounts().forEach(e -> e.validate());
}
if (encryption() != null) {
encryption().validate();
}
if (keyDelivery() != null) {
keyDelivery().validate();
}
if (privateEndpointConnections() != null) {
privateEndpointConnections().forEach(e -> e.validate());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy