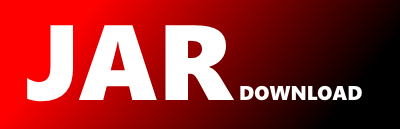
com.azure.resourcemanager.mediaservices.models.MetricSpecification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
/** A metric emitted by service. */
@Fluent
public final class MetricSpecification {
/*
* The metric name.
*/
@JsonProperty(value = "name", access = JsonProperty.Access.WRITE_ONLY)
private String name;
/*
* The metric display name.
*/
@JsonProperty(value = "displayName", access = JsonProperty.Access.WRITE_ONLY)
private String displayName;
/*
* The metric display description.
*/
@JsonProperty(value = "displayDescription", access = JsonProperty.Access.WRITE_ONLY)
private String displayDescription;
/*
* The metric unit
*/
@JsonProperty(value = "unit", access = JsonProperty.Access.WRITE_ONLY)
private MetricUnit unit;
/*
* The metric aggregation type
*/
@JsonProperty(value = "aggregationType", access = JsonProperty.Access.WRITE_ONLY)
private MetricAggregationType aggregationType;
/*
* The metric lock aggregation type
*/
@JsonProperty(value = "lockAggregationType", access = JsonProperty.Access.WRITE_ONLY)
private MetricAggregationType lockAggregationType;
/*
* Supported aggregation types.
*/
@JsonProperty(value = "supportedAggregationTypes")
private List supportedAggregationTypes;
/*
* The metric dimensions.
*/
@JsonProperty(value = "dimensions", access = JsonProperty.Access.WRITE_ONLY)
private List dimensions;
/*
* Indicates whether regional MDM account is enabled.
*/
@JsonProperty(value = "enableRegionalMdmAccount", access = JsonProperty.Access.WRITE_ONLY)
private Boolean enableRegionalMdmAccount;
/*
* The source MDM account.
*/
@JsonProperty(value = "sourceMdmAccount", access = JsonProperty.Access.WRITE_ONLY)
private String sourceMdmAccount;
/*
* The source MDM namespace.
*/
@JsonProperty(value = "sourceMdmNamespace", access = JsonProperty.Access.WRITE_ONLY)
private String sourceMdmNamespace;
/*
* The supported time grain types.
*/
@JsonProperty(value = "supportedTimeGrainTypes", access = JsonProperty.Access.WRITE_ONLY)
private List supportedTimeGrainTypes;
/**
* Get the name property: The metric name.
*
* @return the name value.
*/
public String name() {
return this.name;
}
/**
* Get the displayName property: The metric display name.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Get the displayDescription property: The metric display description.
*
* @return the displayDescription value.
*/
public String displayDescription() {
return this.displayDescription;
}
/**
* Get the unit property: The metric unit.
*
* @return the unit value.
*/
public MetricUnit unit() {
return this.unit;
}
/**
* Get the aggregationType property: The metric aggregation type.
*
* @return the aggregationType value.
*/
public MetricAggregationType aggregationType() {
return this.aggregationType;
}
/**
* Get the lockAggregationType property: The metric lock aggregation type.
*
* @return the lockAggregationType value.
*/
public MetricAggregationType lockAggregationType() {
return this.lockAggregationType;
}
/**
* Get the supportedAggregationTypes property: Supported aggregation types.
*
* @return the supportedAggregationTypes value.
*/
public List supportedAggregationTypes() {
return this.supportedAggregationTypes;
}
/**
* Set the supportedAggregationTypes property: Supported aggregation types.
*
* @param supportedAggregationTypes the supportedAggregationTypes value to set.
* @return the MetricSpecification object itself.
*/
public MetricSpecification withSupportedAggregationTypes(List supportedAggregationTypes) {
this.supportedAggregationTypes = supportedAggregationTypes;
return this;
}
/**
* Get the dimensions property: The metric dimensions.
*
* @return the dimensions value.
*/
public List dimensions() {
return this.dimensions;
}
/**
* Get the enableRegionalMdmAccount property: Indicates whether regional MDM account is enabled.
*
* @return the enableRegionalMdmAccount value.
*/
public Boolean enableRegionalMdmAccount() {
return this.enableRegionalMdmAccount;
}
/**
* Get the sourceMdmAccount property: The source MDM account.
*
* @return the sourceMdmAccount value.
*/
public String sourceMdmAccount() {
return this.sourceMdmAccount;
}
/**
* Get the sourceMdmNamespace property: The source MDM namespace.
*
* @return the sourceMdmNamespace value.
*/
public String sourceMdmNamespace() {
return this.sourceMdmNamespace;
}
/**
* Get the supportedTimeGrainTypes property: The supported time grain types.
*
* @return the supportedTimeGrainTypes value.
*/
public List supportedTimeGrainTypes() {
return this.supportedTimeGrainTypes;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (dimensions() != null) {
dimensions().forEach(e -> e.validate());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy