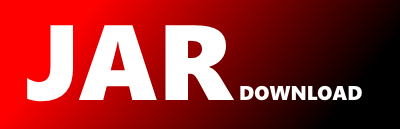
com.azure.resourcemanager.mediaservices.fluent.models.StreamingPolicyProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.mediaservices.models.CommonEncryptionCbcs;
import com.azure.resourcemanager.mediaservices.models.CommonEncryptionCenc;
import com.azure.resourcemanager.mediaservices.models.EnvelopeEncryption;
import com.azure.resourcemanager.mediaservices.models.NoEncryption;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* Class to specify properties of Streaming Policy.
*/
@Fluent
public final class StreamingPolicyProperties implements JsonSerializable {
/*
* Creation time of Streaming Policy
*/
private OffsetDateTime created;
/*
* Default ContentKey used by current Streaming Policy
*/
private String defaultContentKeyPolicyName;
/*
* Configuration of EnvelopeEncryption
*/
private EnvelopeEncryption envelopeEncryption;
/*
* Configuration of CommonEncryptionCenc
*/
private CommonEncryptionCenc commonEncryptionCenc;
/*
* Configuration of CommonEncryptionCbcs
*/
private CommonEncryptionCbcs commonEncryptionCbcs;
/*
* Configurations of NoEncryption
*/
private NoEncryption noEncryption;
/**
* Creates an instance of StreamingPolicyProperties class.
*/
public StreamingPolicyProperties() {
}
/**
* Get the created property: Creation time of Streaming Policy.
*
* @return the created value.
*/
public OffsetDateTime created() {
return this.created;
}
/**
* Get the defaultContentKeyPolicyName property: Default ContentKey used by current Streaming Policy.
*
* @return the defaultContentKeyPolicyName value.
*/
public String defaultContentKeyPolicyName() {
return this.defaultContentKeyPolicyName;
}
/**
* Set the defaultContentKeyPolicyName property: Default ContentKey used by current Streaming Policy.
*
* @param defaultContentKeyPolicyName the defaultContentKeyPolicyName value to set.
* @return the StreamingPolicyProperties object itself.
*/
public StreamingPolicyProperties withDefaultContentKeyPolicyName(String defaultContentKeyPolicyName) {
this.defaultContentKeyPolicyName = defaultContentKeyPolicyName;
return this;
}
/**
* Get the envelopeEncryption property: Configuration of EnvelopeEncryption.
*
* @return the envelopeEncryption value.
*/
public EnvelopeEncryption envelopeEncryption() {
return this.envelopeEncryption;
}
/**
* Set the envelopeEncryption property: Configuration of EnvelopeEncryption.
*
* @param envelopeEncryption the envelopeEncryption value to set.
* @return the StreamingPolicyProperties object itself.
*/
public StreamingPolicyProperties withEnvelopeEncryption(EnvelopeEncryption envelopeEncryption) {
this.envelopeEncryption = envelopeEncryption;
return this;
}
/**
* Get the commonEncryptionCenc property: Configuration of CommonEncryptionCenc.
*
* @return the commonEncryptionCenc value.
*/
public CommonEncryptionCenc commonEncryptionCenc() {
return this.commonEncryptionCenc;
}
/**
* Set the commonEncryptionCenc property: Configuration of CommonEncryptionCenc.
*
* @param commonEncryptionCenc the commonEncryptionCenc value to set.
* @return the StreamingPolicyProperties object itself.
*/
public StreamingPolicyProperties withCommonEncryptionCenc(CommonEncryptionCenc commonEncryptionCenc) {
this.commonEncryptionCenc = commonEncryptionCenc;
return this;
}
/**
* Get the commonEncryptionCbcs property: Configuration of CommonEncryptionCbcs.
*
* @return the commonEncryptionCbcs value.
*/
public CommonEncryptionCbcs commonEncryptionCbcs() {
return this.commonEncryptionCbcs;
}
/**
* Set the commonEncryptionCbcs property: Configuration of CommonEncryptionCbcs.
*
* @param commonEncryptionCbcs the commonEncryptionCbcs value to set.
* @return the StreamingPolicyProperties object itself.
*/
public StreamingPolicyProperties withCommonEncryptionCbcs(CommonEncryptionCbcs commonEncryptionCbcs) {
this.commonEncryptionCbcs = commonEncryptionCbcs;
return this;
}
/**
* Get the noEncryption property: Configurations of NoEncryption.
*
* @return the noEncryption value.
*/
public NoEncryption noEncryption() {
return this.noEncryption;
}
/**
* Set the noEncryption property: Configurations of NoEncryption.
*
* @param noEncryption the noEncryption value to set.
* @return the StreamingPolicyProperties object itself.
*/
public StreamingPolicyProperties withNoEncryption(NoEncryption noEncryption) {
this.noEncryption = noEncryption;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (envelopeEncryption() != null) {
envelopeEncryption().validate();
}
if (commonEncryptionCenc() != null) {
commonEncryptionCenc().validate();
}
if (commonEncryptionCbcs() != null) {
commonEncryptionCbcs().validate();
}
if (noEncryption() != null) {
noEncryption().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("defaultContentKeyPolicyName", this.defaultContentKeyPolicyName);
jsonWriter.writeJsonField("envelopeEncryption", this.envelopeEncryption);
jsonWriter.writeJsonField("commonEncryptionCenc", this.commonEncryptionCenc);
jsonWriter.writeJsonField("commonEncryptionCbcs", this.commonEncryptionCbcs);
jsonWriter.writeJsonField("noEncryption", this.noEncryption);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StreamingPolicyProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StreamingPolicyProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the StreamingPolicyProperties.
*/
public static StreamingPolicyProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StreamingPolicyProperties deserializedStreamingPolicyProperties = new StreamingPolicyProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("created".equals(fieldName)) {
deserializedStreamingPolicyProperties.created = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("defaultContentKeyPolicyName".equals(fieldName)) {
deserializedStreamingPolicyProperties.defaultContentKeyPolicyName = reader.getString();
} else if ("envelopeEncryption".equals(fieldName)) {
deserializedStreamingPolicyProperties.envelopeEncryption = EnvelopeEncryption.fromJson(reader);
} else if ("commonEncryptionCenc".equals(fieldName)) {
deserializedStreamingPolicyProperties.commonEncryptionCenc = CommonEncryptionCenc.fromJson(reader);
} else if ("commonEncryptionCbcs".equals(fieldName)) {
deserializedStreamingPolicyProperties.commonEncryptionCbcs = CommonEncryptionCbcs.fromJson(reader);
} else if ("noEncryption".equals(fieldName)) {
deserializedStreamingPolicyProperties.noEncryption = NoEncryption.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedStreamingPolicyProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy