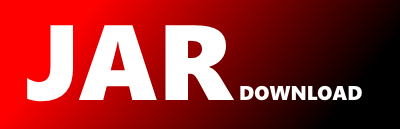
com.azure.resourcemanager.mediaservices.implementation.StreamingLocatorsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.implementation;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.mediaservices.fluent.StreamingLocatorsClient;
import com.azure.resourcemanager.mediaservices.fluent.models.ListContentKeysResponseInner;
import com.azure.resourcemanager.mediaservices.fluent.models.ListPathsResponseInner;
import com.azure.resourcemanager.mediaservices.fluent.models.StreamingLocatorInner;
import com.azure.resourcemanager.mediaservices.models.ListContentKeysResponse;
import com.azure.resourcemanager.mediaservices.models.ListPathsResponse;
import com.azure.resourcemanager.mediaservices.models.StreamingLocator;
import com.azure.resourcemanager.mediaservices.models.StreamingLocators;
public final class StreamingLocatorsImpl implements StreamingLocators {
private static final ClientLogger LOGGER = new ClientLogger(StreamingLocatorsImpl.class);
private final StreamingLocatorsClient innerClient;
private final com.azure.resourcemanager.mediaservices.MediaServicesManager serviceManager;
public StreamingLocatorsImpl(StreamingLocatorsClient innerClient,
com.azure.resourcemanager.mediaservices.MediaServicesManager serviceManager) {
this.innerClient = innerClient;
this.serviceManager = serviceManager;
}
public PagedIterable list(String resourceGroupName, String accountName) {
PagedIterable inner = this.serviceClient().list(resourceGroupName, accountName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new StreamingLocatorImpl(inner1, this.manager()));
}
public PagedIterable list(String resourceGroupName, String accountName, String filter,
Integer top, String orderby, Context context) {
PagedIterable inner
= this.serviceClient().list(resourceGroupName, accountName, filter, top, orderby, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new StreamingLocatorImpl(inner1, this.manager()));
}
public Response getWithResponse(String resourceGroupName, String accountName,
String streamingLocatorName, Context context) {
Response inner
= this.serviceClient().getWithResponse(resourceGroupName, accountName, streamingLocatorName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new StreamingLocatorImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public StreamingLocator get(String resourceGroupName, String accountName, String streamingLocatorName) {
StreamingLocatorInner inner = this.serviceClient().get(resourceGroupName, accountName, streamingLocatorName);
if (inner != null) {
return new StreamingLocatorImpl(inner, this.manager());
} else {
return null;
}
}
public Response deleteWithResponse(String resourceGroupName, String accountName, String streamingLocatorName,
Context context) {
return this.serviceClient().deleteWithResponse(resourceGroupName, accountName, streamingLocatorName, context);
}
public void delete(String resourceGroupName, String accountName, String streamingLocatorName) {
this.serviceClient().delete(resourceGroupName, accountName, streamingLocatorName);
}
public Response listContentKeysWithResponse(String resourceGroupName, String accountName,
String streamingLocatorName, Context context) {
Response inner = this.serviceClient()
.listContentKeysWithResponse(resourceGroupName, accountName, streamingLocatorName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new ListContentKeysResponseImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public ListContentKeysResponse listContentKeys(String resourceGroupName, String accountName,
String streamingLocatorName) {
ListContentKeysResponseInner inner
= this.serviceClient().listContentKeys(resourceGroupName, accountName, streamingLocatorName);
if (inner != null) {
return new ListContentKeysResponseImpl(inner, this.manager());
} else {
return null;
}
}
public Response listPathsWithResponse(String resourceGroupName, String accountName,
String streamingLocatorName, Context context) {
Response inner
= this.serviceClient().listPathsWithResponse(resourceGroupName, accountName, streamingLocatorName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new ListPathsResponseImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public ListPathsResponse listPaths(String resourceGroupName, String accountName, String streamingLocatorName) {
ListPathsResponseInner inner
= this.serviceClient().listPaths(resourceGroupName, accountName, streamingLocatorName);
if (inner != null) {
return new ListPathsResponseImpl(inner, this.manager());
} else {
return null;
}
}
public StreamingLocator getById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String accountName = ResourceManagerUtils.getValueFromIdByName(id, "mediaServices");
if (accountName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'mediaServices'.", id)));
}
String streamingLocatorName = ResourceManagerUtils.getValueFromIdByName(id, "streamingLocators");
if (streamingLocatorName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'streamingLocators'.", id)));
}
return this.getWithResponse(resourceGroupName, accountName, streamingLocatorName, Context.NONE).getValue();
}
public Response getByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String accountName = ResourceManagerUtils.getValueFromIdByName(id, "mediaServices");
if (accountName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'mediaServices'.", id)));
}
String streamingLocatorName = ResourceManagerUtils.getValueFromIdByName(id, "streamingLocators");
if (streamingLocatorName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'streamingLocators'.", id)));
}
return this.getWithResponse(resourceGroupName, accountName, streamingLocatorName, context);
}
public void deleteById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String accountName = ResourceManagerUtils.getValueFromIdByName(id, "mediaServices");
if (accountName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'mediaServices'.", id)));
}
String streamingLocatorName = ResourceManagerUtils.getValueFromIdByName(id, "streamingLocators");
if (streamingLocatorName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'streamingLocators'.", id)));
}
this.deleteWithResponse(resourceGroupName, accountName, streamingLocatorName, Context.NONE);
}
public Response deleteByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String accountName = ResourceManagerUtils.getValueFromIdByName(id, "mediaServices");
if (accountName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'mediaServices'.", id)));
}
String streamingLocatorName = ResourceManagerUtils.getValueFromIdByName(id, "streamingLocators");
if (streamingLocatorName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'streamingLocators'.", id)));
}
return this.deleteWithResponse(resourceGroupName, accountName, streamingLocatorName, context);
}
private StreamingLocatorsClient serviceClient() {
return this.innerClient;
}
private com.azure.resourcemanager.mediaservices.MediaServicesManager manager() {
return this.serviceManager;
}
public StreamingLocatorImpl define(String name) {
return new StreamingLocatorImpl(name, this.manager());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy