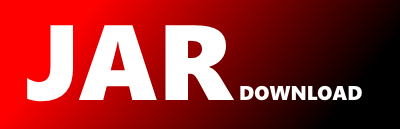
com.azure.resourcemanager.mediaservices.models.AssetTrack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.util.Context;
import com.azure.resourcemanager.mediaservices.fluent.models.AssetTrackInner;
/**
* An immutable client-side representation of AssetTrack.
*/
public interface AssetTrack {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the track property: Detailed information about a track in the asset.
*
* @return the track value.
*/
TrackBase track();
/**
* Gets the provisioningState property: Provisioning state of the asset track.
*
* @return the provisioningState value.
*/
ProvisioningState provisioningState();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.mediaservices.fluent.models.AssetTrackInner object.
*
* @return the inner object.
*/
AssetTrackInner innerModel();
/**
* The entirety of the AssetTrack definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The AssetTrack definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the AssetTrack definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the AssetTrack definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, accountName, assetName.
*
* @param resourceGroupName The name of the resource group within the Azure subscription.
* @param accountName The Media Services account name.
* @param assetName The Asset name.
* @return the next definition stage.
*/
WithCreate withExistingAsset(String resourceGroupName, String accountName, String assetName);
}
/**
* The stage of the AssetTrack definition which contains all the minimum required properties for the resource to
* be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithTrack {
/**
* Executes the create request.
*
* @return the created resource.
*/
AssetTrack create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
AssetTrack create(Context context);
}
/**
* The stage of the AssetTrack definition allowing to specify track.
*/
interface WithTrack {
/**
* Specifies the track property: Detailed information about a track in the asset..
*
* @param track Detailed information about a track in the asset.
* @return the next definition stage.
*/
WithCreate withTrack(TrackBase track);
}
}
/**
* Begins update for the AssetTrack resource.
*
* @return the stage of resource update.
*/
AssetTrack.Update update();
/**
* The template for AssetTrack update.
*/
interface Update extends UpdateStages.WithTrack {
/**
* Executes the update request.
*
* @return the updated resource.
*/
AssetTrack apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
AssetTrack apply(Context context);
}
/**
* The AssetTrack update stages.
*/
interface UpdateStages {
/**
* The stage of the AssetTrack update allowing to specify track.
*/
interface WithTrack {
/**
* Specifies the track property: Detailed information about a track in the asset..
*
* @param track Detailed information about a track in the asset.
* @return the next definition stage.
*/
Update withTrack(TrackBase track);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
AssetTrack refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
AssetTrack refresh(Context context);
/**
* Update the track data
*
* Update the track data. Call this API after any changes are made to the track data stored in the asset container.
* For example, you have modified the WebVTT captions file in the Azure blob storage container for the asset,
* viewers will not see the new version of the captions unless this API is called. Note, the changes may not be
* reflected immediately. CDN cache may also need to be purged if applicable.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void updateTrackData();
/**
* Update the track data
*
* Update the track data. Call this API after any changes are made to the track data stored in the asset container.
* For example, you have modified the WebVTT captions file in the Azure blob storage container for the asset,
* viewers will not see the new version of the captions unless this API is called. Note, the changes may not be
* reflected immediately. CDN cache may also need to be purged if applicable.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void updateTrackData(Context context);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy