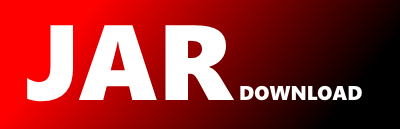
com.azure.resourcemanager.mediaservices.models.CommonEncryptionCenc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Class for envelope encryption scheme.
*/
@Fluent
public final class CommonEncryptionCenc implements JsonSerializable {
/*
* Representing supported protocols
*/
private EnabledProtocols enabledProtocols;
/*
* Representing which tracks should not be encrypted
*/
private List clearTracks;
/*
* Representing default content key for each encryption scheme and separate content keys for specific tracks
*/
private StreamingPolicyContentKeys contentKeys;
/*
* Configuration of DRMs for CommonEncryptionCenc encryption scheme
*/
private CencDrmConfiguration drm;
/*
* Optional configuration supporting ClearKey in CommonEncryptionCenc encryption scheme.
*/
private ClearKeyEncryptionConfiguration clearKeyEncryptionConfiguration;
/**
* Creates an instance of CommonEncryptionCenc class.
*/
public CommonEncryptionCenc() {
}
/**
* Get the enabledProtocols property: Representing supported protocols.
*
* @return the enabledProtocols value.
*/
public EnabledProtocols enabledProtocols() {
return this.enabledProtocols;
}
/**
* Set the enabledProtocols property: Representing supported protocols.
*
* @param enabledProtocols the enabledProtocols value to set.
* @return the CommonEncryptionCenc object itself.
*/
public CommonEncryptionCenc withEnabledProtocols(EnabledProtocols enabledProtocols) {
this.enabledProtocols = enabledProtocols;
return this;
}
/**
* Get the clearTracks property: Representing which tracks should not be encrypted.
*
* @return the clearTracks value.
*/
public List clearTracks() {
return this.clearTracks;
}
/**
* Set the clearTracks property: Representing which tracks should not be encrypted.
*
* @param clearTracks the clearTracks value to set.
* @return the CommonEncryptionCenc object itself.
*/
public CommonEncryptionCenc withClearTracks(List clearTracks) {
this.clearTracks = clearTracks;
return this;
}
/**
* Get the contentKeys property: Representing default content key for each encryption scheme and separate content
* keys for specific tracks.
*
* @return the contentKeys value.
*/
public StreamingPolicyContentKeys contentKeys() {
return this.contentKeys;
}
/**
* Set the contentKeys property: Representing default content key for each encryption scheme and separate content
* keys for specific tracks.
*
* @param contentKeys the contentKeys value to set.
* @return the CommonEncryptionCenc object itself.
*/
public CommonEncryptionCenc withContentKeys(StreamingPolicyContentKeys contentKeys) {
this.contentKeys = contentKeys;
return this;
}
/**
* Get the drm property: Configuration of DRMs for CommonEncryptionCenc encryption scheme.
*
* @return the drm value.
*/
public CencDrmConfiguration drm() {
return this.drm;
}
/**
* Set the drm property: Configuration of DRMs for CommonEncryptionCenc encryption scheme.
*
* @param drm the drm value to set.
* @return the CommonEncryptionCenc object itself.
*/
public CommonEncryptionCenc withDrm(CencDrmConfiguration drm) {
this.drm = drm;
return this;
}
/**
* Get the clearKeyEncryptionConfiguration property: Optional configuration supporting ClearKey in
* CommonEncryptionCenc encryption scheme.
*
* @return the clearKeyEncryptionConfiguration value.
*/
public ClearKeyEncryptionConfiguration clearKeyEncryptionConfiguration() {
return this.clearKeyEncryptionConfiguration;
}
/**
* Set the clearKeyEncryptionConfiguration property: Optional configuration supporting ClearKey in
* CommonEncryptionCenc encryption scheme.
*
* @param clearKeyEncryptionConfiguration the clearKeyEncryptionConfiguration value to set.
* @return the CommonEncryptionCenc object itself.
*/
public CommonEncryptionCenc
withClearKeyEncryptionConfiguration(ClearKeyEncryptionConfiguration clearKeyEncryptionConfiguration) {
this.clearKeyEncryptionConfiguration = clearKeyEncryptionConfiguration;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (enabledProtocols() != null) {
enabledProtocols().validate();
}
if (clearTracks() != null) {
clearTracks().forEach(e -> e.validate());
}
if (contentKeys() != null) {
contentKeys().validate();
}
if (drm() != null) {
drm().validate();
}
if (clearKeyEncryptionConfiguration() != null) {
clearKeyEncryptionConfiguration().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("enabledProtocols", this.enabledProtocols);
jsonWriter.writeArrayField("clearTracks", this.clearTracks, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("contentKeys", this.contentKeys);
jsonWriter.writeJsonField("drm", this.drm);
jsonWriter.writeJsonField("clearKeyEncryptionConfiguration", this.clearKeyEncryptionConfiguration);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of CommonEncryptionCenc from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of CommonEncryptionCenc if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the CommonEncryptionCenc.
*/
public static CommonEncryptionCenc fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
CommonEncryptionCenc deserializedCommonEncryptionCenc = new CommonEncryptionCenc();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("enabledProtocols".equals(fieldName)) {
deserializedCommonEncryptionCenc.enabledProtocols = EnabledProtocols.fromJson(reader);
} else if ("clearTracks".equals(fieldName)) {
List clearTracks = reader.readArray(reader1 -> TrackSelection.fromJson(reader1));
deserializedCommonEncryptionCenc.clearTracks = clearTracks;
} else if ("contentKeys".equals(fieldName)) {
deserializedCommonEncryptionCenc.contentKeys = StreamingPolicyContentKeys.fromJson(reader);
} else if ("drm".equals(fieldName)) {
deserializedCommonEncryptionCenc.drm = CencDrmConfiguration.fromJson(reader);
} else if ("clearKeyEncryptionConfiguration".equals(fieldName)) {
deserializedCommonEncryptionCenc.clearKeyEncryptionConfiguration
= ClearKeyEncryptionConfiguration.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedCommonEncryptionCenc;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy