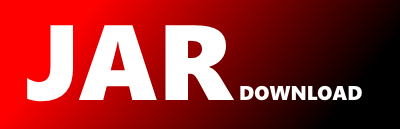
com.azure.resourcemanager.mediaservices.models.ContentKeyPolicyFairPlayConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Specifies a configuration for FairPlay licenses.
*/
@Fluent
public final class ContentKeyPolicyFairPlayConfiguration extends ContentKeyPolicyConfiguration {
/*
* The discriminator for derived types.
*/
private String odataType = "#Microsoft.Media.ContentKeyPolicyFairPlayConfiguration";
/*
* The key that must be used as FairPlay Application Secret key. This needs to be base64 encoded.
*/
private byte[] ask;
/*
* The password encrypting FairPlay certificate in PKCS 12 (pfx) format.
*/
private String fairPlayPfxPassword;
/*
* The Base64 representation of FairPlay certificate in PKCS 12 (pfx) format (including private key).
*/
private String fairPlayPfx;
/*
* The rental and lease key type.
*/
private ContentKeyPolicyFairPlayRentalAndLeaseKeyType rentalAndLeaseKeyType;
/*
* The rental duration. Must be greater than or equal to 0.
*/
private long rentalDuration;
/*
* Offline rental policy
*/
private ContentKeyPolicyFairPlayOfflineRentalConfiguration offlineRentalConfiguration;
/**
* Creates an instance of ContentKeyPolicyFairPlayConfiguration class.
*/
public ContentKeyPolicyFairPlayConfiguration() {
}
/**
* Get the odataType property: The discriminator for derived types.
*
* @return the odataType value.
*/
@Override
public String odataType() {
return this.odataType;
}
/**
* Get the ask property: The key that must be used as FairPlay Application Secret key. This needs to be base64
* encoded.
*
* @return the ask value.
*/
public byte[] ask() {
return CoreUtils.clone(this.ask);
}
/**
* Set the ask property: The key that must be used as FairPlay Application Secret key. This needs to be base64
* encoded.
*
* @param ask the ask value to set.
* @return the ContentKeyPolicyFairPlayConfiguration object itself.
*/
public ContentKeyPolicyFairPlayConfiguration withAsk(byte[] ask) {
this.ask = CoreUtils.clone(ask);
return this;
}
/**
* Get the fairPlayPfxPassword property: The password encrypting FairPlay certificate in PKCS 12 (pfx) format.
*
* @return the fairPlayPfxPassword value.
*/
public String fairPlayPfxPassword() {
return this.fairPlayPfxPassword;
}
/**
* Set the fairPlayPfxPassword property: The password encrypting FairPlay certificate in PKCS 12 (pfx) format.
*
* @param fairPlayPfxPassword the fairPlayPfxPassword value to set.
* @return the ContentKeyPolicyFairPlayConfiguration object itself.
*/
public ContentKeyPolicyFairPlayConfiguration withFairPlayPfxPassword(String fairPlayPfxPassword) {
this.fairPlayPfxPassword = fairPlayPfxPassword;
return this;
}
/**
* Get the fairPlayPfx property: The Base64 representation of FairPlay certificate in PKCS 12 (pfx) format
* (including private key).
*
* @return the fairPlayPfx value.
*/
public String fairPlayPfx() {
return this.fairPlayPfx;
}
/**
* Set the fairPlayPfx property: The Base64 representation of FairPlay certificate in PKCS 12 (pfx) format
* (including private key).
*
* @param fairPlayPfx the fairPlayPfx value to set.
* @return the ContentKeyPolicyFairPlayConfiguration object itself.
*/
public ContentKeyPolicyFairPlayConfiguration withFairPlayPfx(String fairPlayPfx) {
this.fairPlayPfx = fairPlayPfx;
return this;
}
/**
* Get the rentalAndLeaseKeyType property: The rental and lease key type.
*
* @return the rentalAndLeaseKeyType value.
*/
public ContentKeyPolicyFairPlayRentalAndLeaseKeyType rentalAndLeaseKeyType() {
return this.rentalAndLeaseKeyType;
}
/**
* Set the rentalAndLeaseKeyType property: The rental and lease key type.
*
* @param rentalAndLeaseKeyType the rentalAndLeaseKeyType value to set.
* @return the ContentKeyPolicyFairPlayConfiguration object itself.
*/
public ContentKeyPolicyFairPlayConfiguration
withRentalAndLeaseKeyType(ContentKeyPolicyFairPlayRentalAndLeaseKeyType rentalAndLeaseKeyType) {
this.rentalAndLeaseKeyType = rentalAndLeaseKeyType;
return this;
}
/**
* Get the rentalDuration property: The rental duration. Must be greater than or equal to 0.
*
* @return the rentalDuration value.
*/
public long rentalDuration() {
return this.rentalDuration;
}
/**
* Set the rentalDuration property: The rental duration. Must be greater than or equal to 0.
*
* @param rentalDuration the rentalDuration value to set.
* @return the ContentKeyPolicyFairPlayConfiguration object itself.
*/
public ContentKeyPolicyFairPlayConfiguration withRentalDuration(long rentalDuration) {
this.rentalDuration = rentalDuration;
return this;
}
/**
* Get the offlineRentalConfiguration property: Offline rental policy.
*
* @return the offlineRentalConfiguration value.
*/
public ContentKeyPolicyFairPlayOfflineRentalConfiguration offlineRentalConfiguration() {
return this.offlineRentalConfiguration;
}
/**
* Set the offlineRentalConfiguration property: Offline rental policy.
*
* @param offlineRentalConfiguration the offlineRentalConfiguration value to set.
* @return the ContentKeyPolicyFairPlayConfiguration object itself.
*/
public ContentKeyPolicyFairPlayConfiguration
withOfflineRentalConfiguration(ContentKeyPolicyFairPlayOfflineRentalConfiguration offlineRentalConfiguration) {
this.offlineRentalConfiguration = offlineRentalConfiguration;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
if (ask() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property ask in model ContentKeyPolicyFairPlayConfiguration"));
}
if (fairPlayPfxPassword() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property fairPlayPfxPassword in model ContentKeyPolicyFairPlayConfiguration"));
}
if (fairPlayPfx() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property fairPlayPfx in model ContentKeyPolicyFairPlayConfiguration"));
}
if (rentalAndLeaseKeyType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property rentalAndLeaseKeyType in model ContentKeyPolicyFairPlayConfiguration"));
}
if (offlineRentalConfiguration() != null) {
offlineRentalConfiguration().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(ContentKeyPolicyFairPlayConfiguration.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeBinaryField("ask", this.ask);
jsonWriter.writeStringField("fairPlayPfxPassword", this.fairPlayPfxPassword);
jsonWriter.writeStringField("fairPlayPfx", this.fairPlayPfx);
jsonWriter.writeStringField("rentalAndLeaseKeyType",
this.rentalAndLeaseKeyType == null ? null : this.rentalAndLeaseKeyType.toString());
jsonWriter.writeLongField("rentalDuration", this.rentalDuration);
jsonWriter.writeStringField("@odata.type", this.odataType);
jsonWriter.writeJsonField("offlineRentalConfiguration", this.offlineRentalConfiguration);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ContentKeyPolicyFairPlayConfiguration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ContentKeyPolicyFairPlayConfiguration if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ContentKeyPolicyFairPlayConfiguration.
*/
public static ContentKeyPolicyFairPlayConfiguration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ContentKeyPolicyFairPlayConfiguration deserializedContentKeyPolicyFairPlayConfiguration
= new ContentKeyPolicyFairPlayConfiguration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("ask".equals(fieldName)) {
deserializedContentKeyPolicyFairPlayConfiguration.ask = reader.getBinary();
} else if ("fairPlayPfxPassword".equals(fieldName)) {
deserializedContentKeyPolicyFairPlayConfiguration.fairPlayPfxPassword = reader.getString();
} else if ("fairPlayPfx".equals(fieldName)) {
deserializedContentKeyPolicyFairPlayConfiguration.fairPlayPfx = reader.getString();
} else if ("rentalAndLeaseKeyType".equals(fieldName)) {
deserializedContentKeyPolicyFairPlayConfiguration.rentalAndLeaseKeyType
= ContentKeyPolicyFairPlayRentalAndLeaseKeyType.fromString(reader.getString());
} else if ("rentalDuration".equals(fieldName)) {
deserializedContentKeyPolicyFairPlayConfiguration.rentalDuration = reader.getLong();
} else if ("@odata.type".equals(fieldName)) {
deserializedContentKeyPolicyFairPlayConfiguration.odataType = reader.getString();
} else if ("offlineRentalConfiguration".equals(fieldName)) {
deserializedContentKeyPolicyFairPlayConfiguration.offlineRentalConfiguration
= ContentKeyPolicyFairPlayOfflineRentalConfiguration.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedContentKeyPolicyFairPlayConfiguration;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy