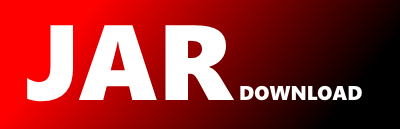
com.azure.resourcemanager.mediaservices.models.JobOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* Describes all the properties of a JobOutput.
*/
@Fluent
public class JobOutput implements JsonSerializable {
/*
* The discriminator for derived types.
*/
private String odataType = "JobOutput";
/*
* If the JobOutput is in the Error state, it contains the details of the error.
*/
private JobError error;
/*
* A preset used to override the preset in the corresponding transform output.
*/
private Preset presetOverride;
/*
* Describes the state of the JobOutput.
*/
private JobState state;
/*
* If the JobOutput is in a Processing state, this contains the Job completion percentage. The value is an estimate
* and not intended to be used to predict Job completion times. To determine if the JobOutput is complete, use the
* State property.
*/
private Integer progress;
/*
* A label that is assigned to a JobOutput in order to help uniquely identify it. This is useful when your Transform
* has more than one TransformOutput, whereby your Job has more than one JobOutput. In such cases, when you submit
* the Job, you will add two or more JobOutputs, in the same order as TransformOutputs in the Transform.
* Subsequently, when you retrieve the Job, either through events or on a GET request, you can use the label to
* easily identify the JobOutput. If a label is not provided, a default value of '{presetName}_{outputIndex}' will
* be used, where the preset name is the name of the preset in the corresponding TransformOutput and the output
* index is the relative index of the this JobOutput within the Job. Note that this index is the same as the
* relative index of the corresponding TransformOutput within its Transform.
*/
private String label;
/*
* The UTC date and time at which this Job Output began processing.
*/
private OffsetDateTime startTime;
/*
* The UTC date and time at which this Job Output finished processing.
*/
private OffsetDateTime endTime;
/**
* Creates an instance of JobOutput class.
*/
public JobOutput() {
}
/**
* Get the odataType property: The discriminator for derived types.
*
* @return the odataType value.
*/
public String odataType() {
return this.odataType;
}
/**
* Get the error property: If the JobOutput is in the Error state, it contains the details of the error.
*
* @return the error value.
*/
public JobError error() {
return this.error;
}
/**
* Set the error property: If the JobOutput is in the Error state, it contains the details of the error.
*
* @param error the error value to set.
* @return the JobOutput object itself.
*/
JobOutput withError(JobError error) {
this.error = error;
return this;
}
/**
* Get the presetOverride property: A preset used to override the preset in the corresponding transform output.
*
* @return the presetOverride value.
*/
public Preset presetOverride() {
return this.presetOverride;
}
/**
* Set the presetOverride property: A preset used to override the preset in the corresponding transform output.
*
* @param presetOverride the presetOverride value to set.
* @return the JobOutput object itself.
*/
public JobOutput withPresetOverride(Preset presetOverride) {
this.presetOverride = presetOverride;
return this;
}
/**
* Get the state property: Describes the state of the JobOutput.
*
* @return the state value.
*/
public JobState state() {
return this.state;
}
/**
* Set the state property: Describes the state of the JobOutput.
*
* @param state the state value to set.
* @return the JobOutput object itself.
*/
JobOutput withState(JobState state) {
this.state = state;
return this;
}
/**
* Get the progress property: If the JobOutput is in a Processing state, this contains the Job completion
* percentage. The value is an estimate and not intended to be used to predict Job completion times. To determine if
* the JobOutput is complete, use the State property.
*
* @return the progress value.
*/
public Integer progress() {
return this.progress;
}
/**
* Set the progress property: If the JobOutput is in a Processing state, this contains the Job completion
* percentage. The value is an estimate and not intended to be used to predict Job completion times. To determine if
* the JobOutput is complete, use the State property.
*
* @param progress the progress value to set.
* @return the JobOutput object itself.
*/
JobOutput withProgress(Integer progress) {
this.progress = progress;
return this;
}
/**
* Get the label property: A label that is assigned to a JobOutput in order to help uniquely identify it. This is
* useful when your Transform has more than one TransformOutput, whereby your Job has more than one JobOutput. In
* such cases, when you submit the Job, you will add two or more JobOutputs, in the same order as TransformOutputs
* in the Transform. Subsequently, when you retrieve the Job, either through events or on a GET request, you can use
* the label to easily identify the JobOutput. If a label is not provided, a default value of
* '{presetName}_{outputIndex}' will be used, where the preset name is the name of the preset in the corresponding
* TransformOutput and the output index is the relative index of the this JobOutput within the Job. Note that this
* index is the same as the relative index of the corresponding TransformOutput within its Transform.
*
* @return the label value.
*/
public String label() {
return this.label;
}
/**
* Set the label property: A label that is assigned to a JobOutput in order to help uniquely identify it. This is
* useful when your Transform has more than one TransformOutput, whereby your Job has more than one JobOutput. In
* such cases, when you submit the Job, you will add two or more JobOutputs, in the same order as TransformOutputs
* in the Transform. Subsequently, when you retrieve the Job, either through events or on a GET request, you can use
* the label to easily identify the JobOutput. If a label is not provided, a default value of
* '{presetName}_{outputIndex}' will be used, where the preset name is the name of the preset in the corresponding
* TransformOutput and the output index is the relative index of the this JobOutput within the Job. Note that this
* index is the same as the relative index of the corresponding TransformOutput within its Transform.
*
* @param label the label value to set.
* @return the JobOutput object itself.
*/
public JobOutput withLabel(String label) {
this.label = label;
return this;
}
/**
* Get the startTime property: The UTC date and time at which this Job Output began processing.
*
* @return the startTime value.
*/
public OffsetDateTime startTime() {
return this.startTime;
}
/**
* Set the startTime property: The UTC date and time at which this Job Output began processing.
*
* @param startTime the startTime value to set.
* @return the JobOutput object itself.
*/
JobOutput withStartTime(OffsetDateTime startTime) {
this.startTime = startTime;
return this;
}
/**
* Get the endTime property: The UTC date and time at which this Job Output finished processing.
*
* @return the endTime value.
*/
public OffsetDateTime endTime() {
return this.endTime;
}
/**
* Set the endTime property: The UTC date and time at which this Job Output finished processing.
*
* @param endTime the endTime value to set.
* @return the JobOutput object itself.
*/
JobOutput withEndTime(OffsetDateTime endTime) {
this.endTime = endTime;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (error() != null) {
error().validate();
}
if (presetOverride() != null) {
presetOverride().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("@odata.type", this.odataType);
jsonWriter.writeJsonField("presetOverride", this.presetOverride);
jsonWriter.writeStringField("label", this.label);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of JobOutput from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of JobOutput if the JsonReader was pointing to an instance of it, or null if it was pointing
* to JSON null.
* @throws IOException If an error occurs while reading the JobOutput.
*/
public static JobOutput fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
String discriminatorValue = null;
try (JsonReader readerToUse = reader.bufferObject()) {
readerToUse.nextToken(); // Prepare for reading
while (readerToUse.nextToken() != JsonToken.END_OBJECT) {
String fieldName = readerToUse.getFieldName();
readerToUse.nextToken();
if ("@odata.type".equals(fieldName)) {
discriminatorValue = readerToUse.getString();
break;
} else {
readerToUse.skipChildren();
}
}
// Use the discriminator value to determine which subtype should be deserialized.
if ("#Microsoft.Media.JobOutputAsset".equals(discriminatorValue)) {
return JobOutputAsset.fromJson(readerToUse.reset());
} else {
return fromJsonKnownDiscriminator(readerToUse.reset());
}
}
});
}
static JobOutput fromJsonKnownDiscriminator(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
JobOutput deserializedJobOutput = new JobOutput();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("@odata.type".equals(fieldName)) {
deserializedJobOutput.odataType = reader.getString();
} else if ("error".equals(fieldName)) {
deserializedJobOutput.error = JobError.fromJson(reader);
} else if ("presetOverride".equals(fieldName)) {
deserializedJobOutput.presetOverride = Preset.fromJson(reader);
} else if ("state".equals(fieldName)) {
deserializedJobOutput.state = JobState.fromString(reader.getString());
} else if ("progress".equals(fieldName)) {
deserializedJobOutput.progress = reader.getNullable(JsonReader::getInt);
} else if ("label".equals(fieldName)) {
deserializedJobOutput.label = reader.getString();
} else if ("startTime".equals(fieldName)) {
deserializedJobOutput.startTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("endTime".equals(fieldName)) {
deserializedJobOutput.endTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedJobOutput;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy